PxVehicleDriveNW Class Reference
[Vehicle]
Data structure with instanced dynamics data and configuration data of a vehicle with up to PX_MAX_NB_WHEELS driven wheels.
More...
#include <PxVehicleDriveNW.h>
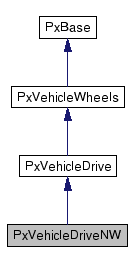
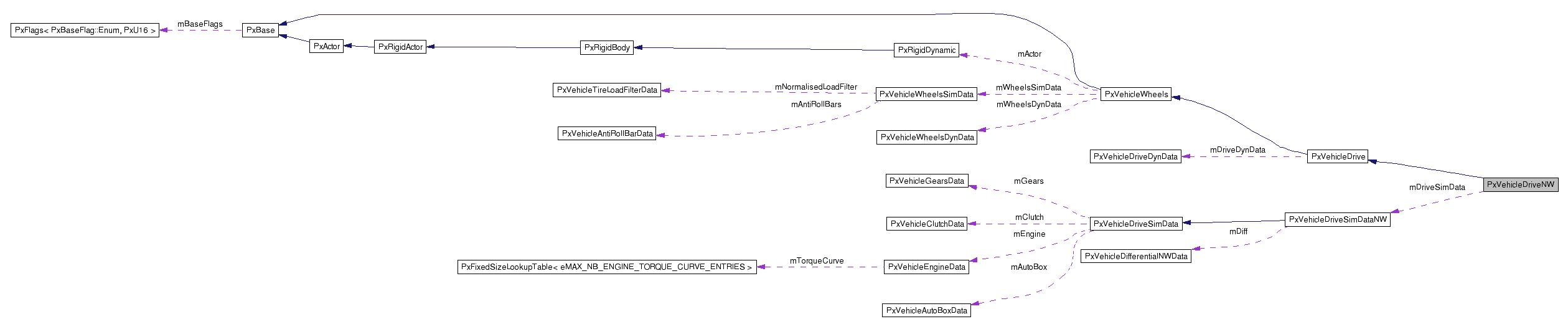
Public Member Functions | |
void | free () |
Deallocate a PxVehicleDriveNW instance. | |
void | setup (PxPhysics *physics, PxRigidDynamic *vehActor, const PxVehicleWheelsSimData &wheelsData, const PxVehicleDriveSimDataNW &driveData, const PxU32 nbWheels) |
Set up a vehicle using simulation data for the wheels and drive model. | |
void | setToRestState () |
Set a vehicle to its rest state. Aside from the rigid body transform, this will set the vehicle and rigid body to the state they were in immediately after setup or create. | |
PxVehicleDriveNW (PxBaseFlags baseFlags) | |
PxVehicleDriveNW () | |
~PxVehicleDriveNW () | |
virtual const char * | getConcreteTypeName () const |
Returns string name of dynamic type. | |
virtual bool | isKindOf (const char *name) const |
Returns whether a given type name matches with the type of this instance. | |
Static Public Member Functions | |
static PxVehicleDriveNW * | allocate (const PxU32 nbWheels) |
Allocate a PxVehicleDriveNW instance for a NWDrive vehicle with nbWheels. | |
static PxVehicleDriveNW * | create (PxPhysics *physics, PxRigidDynamic *vehActor, const PxVehicleWheelsSimData &wheelsData, const PxVehicleDriveSimDataNW &driveData, const PxU32 nbWheels) |
Allocate and set up a vehicle using simulation data for the wheels and drive model. | |
static PxVehicleDriveNW * | createObject (PxU8 *&address, PxDeserializationContext &context) |
static void | getBinaryMetaData (PxOutputStream &stream) |
Public Attributes | |
PxVehicleDriveSimDataNW | mDriveSimData |
Simulation data that describes the configuration of the vehicle's drive model. | |
Private Member Functions | |
bool | isValid () const |
Test if the instanced dynamics and configuration data has legal values. | |
Friends | |
class | PxVehicleUpdate |
Detailed Description
Data structure with instanced dynamics data and configuration data of a vehicle with up to PX_MAX_NB_WHEELS driven wheels.Constructor & Destructor Documentation
PxVehicleDriveNW::PxVehicleDriveNW | ( | PxBaseFlags | baseFlags | ) | [inline] |
PxVehicleDriveNW::PxVehicleDriveNW | ( | ) |
PxVehicleDriveNW::~PxVehicleDriveNW | ( | ) | [inline] |
Member Function Documentation
static PxVehicleDriveNW* PxVehicleDriveNW::allocate | ( | const PxU32 | nbWheels | ) | [static] |
Allocate a PxVehicleDriveNW instance for a NWDrive vehicle with nbWheels.
- Parameters:
-
[in] nbWheels is the number of wheels on the vehicle.
- Returns:
- The instantiated vehicle.
static PxVehicleDriveNW* PxVehicleDriveNW::create | ( | PxPhysics * | physics, | |
PxRigidDynamic * | vehActor, | |||
const PxVehicleWheelsSimData & | wheelsData, | |||
const PxVehicleDriveSimDataNW & | driveData, | |||
const PxU32 | nbWheels | |||
) | [static] |
Allocate and set up a vehicle using simulation data for the wheels and drive model.
- Parameters:
-
[in] physics is a PxPhysics instance that is needed to create special vehicle constraints that are maintained by the vehicle. [in] vehActor is a PxRigidDynamic instance that is used to represent the vehicle in the PhysX SDK. [in] wheelsData describes the configuration of all suspension/tires/wheels of the vehicle. The vehicle instance takes a copy of this data. [in] driveData describes the properties of the vehicle's drive model (gears/engine/clutch/differential/autobox). The vehicle instance takes a copy of this data. [in] nbWheels is the number of wheels on the vehicle.
- Note:
- It is assumed that the first shapes of the actor are the wheel shapes, followed by the chassis shapes. To break this assumption use PxVehicleWheelsSimData::setWheelShapeMapping.
- Returns:
- The instantiated vehicle.
static PxVehicleDriveNW* PxVehicleDriveNW::createObject | ( | PxU8 *& | address, | |
PxDeserializationContext & | context | |||
) | [static] |
void PxVehicleDriveNW::free | ( | ) |
static void PxVehicleDriveNW::getBinaryMetaData | ( | PxOutputStream & | stream | ) | [static] |
Reimplemented from PxVehicleDrive.
virtual const char* PxVehicleDriveNW::getConcreteTypeName | ( | ) | const [inline, virtual] |
Returns string name of dynamic type.
- Returns:
- Class name of most derived type of this object.
Reimplemented from PxVehicleDrive.
virtual bool PxVehicleDriveNW::isKindOf | ( | const char * | superClass | ) | const [inline, virtual] |
Returns whether a given type name matches with the type of this instance.
Reimplemented from PxVehicleDrive.
References PxBase::isKindOf().
bool PxVehicleDriveNW::isValid | ( | ) | const [private] |
Test if the instanced dynamics and configuration data has legal values.
Reimplemented from PxVehicleDrive.
void PxVehicleDriveNW::setToRestState | ( | ) |
Set a vehicle to its rest state. Aside from the rigid body transform, this will set the vehicle and rigid body to the state they were in immediately after setup or create.
- Note:
- Calling setToRestState invalidates the cached raycast hit planes under each wheel meaning that suspension line raycasts need to be performed at least once with PxVehicleSuspensionRaycasts before calling PxVehicleUpdates.
- See also:
- setup, create, PxVehicleSuspensionRaycasts, PxVehicleUpdates
Reimplemented from PxVehicleDrive.
void PxVehicleDriveNW::setup | ( | PxPhysics * | physics, | |
PxRigidDynamic * | vehActor, | |||
const PxVehicleWheelsSimData & | wheelsData, | |||
const PxVehicleDriveSimDataNW & | driveData, | |||
const PxU32 | nbWheels | |||
) |
Set up a vehicle using simulation data for the wheels and drive model.
- Parameters:
-
[in] physics is a PxPhysics instance that is needed to create special vehicle constraints that are maintained by the vehicle. [in] vehActor is a PxRigidDynamic instance that is used to represent the vehicle in the PhysX SDK. [in] wheelsData describes the configuration of all suspension/tires/wheels of the vehicle. The vehicle instance takes a copy of this data. [in] driveData describes the properties of the vehicle's drive model (gears/engine/clutch/differential/autobox). The vehicle instance takes a copy of this data. [in] nbWheels is the number of wheels on the vehicle.
- Note:
- It is assumed that the first shapes of the actor are the wheel shapes, followed by the chassis shapes. To break this assumption use PxVehicleWheelsSimData::setWheelShapeMapping.
Friends And Related Function Documentation
friend class PxVehicleUpdate [friend] |
Reimplemented from PxVehicleDrive.
Member Data Documentation
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com