PxStrideIterator< T > Class Template Reference
[Foundation]
Iterator class for iterating over arrays of data that may be interleaved with other data.
More...
#include <PxStrideIterator.h>
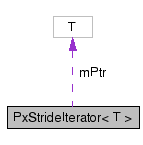
Public Member Functions | |
PX_INLINE | PxStrideIterator (T *ptr=NULL, PxU32 stride=sizeof(T)) |
Constructor. | |
PX_INLINE | PxStrideIterator (const PxStrideIterator< typename StripConst< T >::Type > &strideIterator) |
Copy constructor. | |
PX_INLINE T * | ptr () const |
Get pointer to element. | |
PX_INLINE PxU32 | stride () const |
Get stride. | |
PX_INLINE T & | operator* () const |
Indirection operator. | |
PX_INLINE T * | operator-> () const |
Dereferencing operator. | |
PX_INLINE T & | operator[] (unsigned int i) const |
Indexing operator. | |
PX_INLINE PxStrideIterator & | operator++ () |
Pre-increment operator. | |
PX_INLINE PxStrideIterator | operator++ (int) |
Post-increment operator. | |
PX_INLINE PxStrideIterator & | operator-- () |
Pre-decrement operator. | |
PX_INLINE PxStrideIterator | operator-- (int) |
Post-decrement operator. | |
PX_INLINE PxStrideIterator | operator+ (unsigned int i) const |
Addition operator. | |
PX_INLINE PxStrideIterator | operator- (unsigned int i) const |
Subtraction operator. | |
PX_INLINE PxStrideIterator & | operator+= (unsigned int i) |
Addition compound assignment operator. | |
PX_INLINE PxStrideIterator & | operator-= (unsigned int i) |
Subtraction compound assignment operator. | |
PX_INLINE int | operator- (const PxStrideIterator &other) const |
Iterator difference. | |
PX_INLINE bool | operator== (const PxStrideIterator &other) const |
Equality operator. | |
PX_INLINE bool | operator!= (const PxStrideIterator &other) const |
Inequality operator. | |
PX_INLINE bool | operator< (const PxStrideIterator &other) const |
Less than operator. | |
PX_INLINE bool | operator> (const PxStrideIterator &other) const |
Greater than operator. | |
PX_INLINE bool | operator<= (const PxStrideIterator &other) const |
Less or equal than operator. | |
PX_INLINE bool | operator>= (const PxStrideIterator &other) const |
Greater or equal than operator. | |
Private Member Functions | |
PX_INLINE bool | isCompatible (const PxStrideIterator &other) const |
Static Private Member Functions | |
static PX_INLINE T * | byteAdd (T *ptr, PxU32 bytes) |
static PX_INLINE T * | byteSub (T *ptr, PxU32 bytes) |
Private Attributes | |
T * | mPtr |
PxU32 | mStride |
Detailed Description
template<typename T>
class PxStrideIterator< T >
Iterator class for iterating over arrays of data that may be interleaved with other data.
This class is used for iterating over arrays of elements that may have a larger element to element offset, called the stride, than the size of the element itself (non-contiguous).
The template parameter T denotes the type of the element accessed. The stride itself is stored as a member field so multiple instances of a PxStrideIterator class can have different strides. This is useful for cases were the stride depends on runtime configuration.
The stride iterator can be used for index based access, e.g.:
PxStrideIterator<PxVec3> strideArray(...); for (unsigned i = 0; i < 10; ++i) { PxVec3& vec = strideArray[i]; ... }
PxStrideIterator<PxVec3> strideBegin(...); PxStrideIterator<PxVec3> strideEnd(strideBegin + 10); for (PxStrideIterator<PxVec3> it = strideBegin; it < strideEnd; ++it) { PxVec3& vec = *it; ... }
Two special cases:
- A stride of sizeof(T) represents a regular c array of type T.
- A stride of 0 can be used to describe re-occurrence of the same element multiple times.
Constructor & Destructor Documentation
PX_INLINE PxStrideIterator< T >::PxStrideIterator | ( | T * | ptr = NULL , |
|
PxU32 | stride = sizeof(T) | |||
) | [inline, explicit] |
Constructor.
Optionally takes a pointer to an element and a stride.
- Parameters:
-
[in] ptr pointer to element, defaults to NULL. [in] stride stride for accessing consecutive elements, defaults to the size of one element.
PX_INLINE PxStrideIterator< T >::PxStrideIterator | ( | const PxStrideIterator< typename StripConst< T >::Type > & | strideIterator | ) | [inline] |
Member Function Documentation
static PX_INLINE T* PxStrideIterator< T >::byteAdd | ( | T * | ptr, | |
PxU32 | bytes | |||
) | [inline, static, private] |
static PX_INLINE T* PxStrideIterator< T >::byteSub | ( | T * | ptr, | |
PxU32 | bytes | |||
) | [inline, static, private] |
PX_INLINE bool PxStrideIterator< T >::isCompatible | ( | const PxStrideIterator< T > & | other | ) | const [inline, private] |
PX_INLINE bool PxStrideIterator< T >::operator!= | ( | const PxStrideIterator< T > & | other | ) | const [inline] |
Inequality operator.
PX_INLINE T& PxStrideIterator< T >::operator* | ( | ) | const [inline] |
Indirection operator.
PX_INLINE PxStrideIterator PxStrideIterator< T >::operator+ | ( | unsigned int | i | ) | const [inline] |
Addition operator.
PX_INLINE PxStrideIterator PxStrideIterator< T >::operator++ | ( | int | ) | [inline] |
Post-increment operator.
PX_INLINE PxStrideIterator& PxStrideIterator< T >::operator++ | ( | ) | [inline] |
Pre-increment operator.
PX_INLINE PxStrideIterator& PxStrideIterator< T >::operator+= | ( | unsigned int | i | ) | [inline] |
Addition compound assignment operator.
PX_INLINE int PxStrideIterator< T >::operator- | ( | const PxStrideIterator< T > & | other | ) | const [inline] |
Iterator difference.
PX_INLINE PxStrideIterator PxStrideIterator< T >::operator- | ( | unsigned int | i | ) | const [inline] |
Subtraction operator.
PX_INLINE PxStrideIterator PxStrideIterator< T >::operator-- | ( | int | ) | [inline] |
Post-decrement operator.
PX_INLINE PxStrideIterator& PxStrideIterator< T >::operator-- | ( | ) | [inline] |
Pre-decrement operator.
PX_INLINE PxStrideIterator& PxStrideIterator< T >::operator-= | ( | unsigned int | i | ) | [inline] |
Subtraction compound assignment operator.
PX_INLINE T* PxStrideIterator< T >::operator-> | ( | ) | const [inline] |
Dereferencing operator.
PX_INLINE bool PxStrideIterator< T >::operator< | ( | const PxStrideIterator< T > & | other | ) | const [inline] |
Less than operator.
PX_INLINE bool PxStrideIterator< T >::operator<= | ( | const PxStrideIterator< T > & | other | ) | const [inline] |
Less or equal than operator.
PX_INLINE bool PxStrideIterator< T >::operator== | ( | const PxStrideIterator< T > & | other | ) | const [inline] |
Equality operator.
PX_INLINE bool PxStrideIterator< T >::operator> | ( | const PxStrideIterator< T > & | other | ) | const [inline] |
Greater than operator.
PX_INLINE bool PxStrideIterator< T >::operator>= | ( | const PxStrideIterator< T > & | other | ) | const [inline] |
Greater or equal than operator.
PX_INLINE T& PxStrideIterator< T >::operator[] | ( | unsigned int | i | ) | const [inline] |
Indexing operator.
PX_INLINE T* PxStrideIterator< T >::ptr | ( | ) | const [inline] |
PX_INLINE PxU32 PxStrideIterator< T >::stride | ( | ) | const [inline] |
Member Data Documentation
T* PxStrideIterator< T >::mPtr [private] |
Referenced by PxStrideIterator< const PxVec3 >::isCompatible(), PxStrideIterator< const PxVec3 >::operator!=(), PxStrideIterator< const PxVec3 >::operator-(), PxStrideIterator< const PxVec3 >::operator<(), PxStrideIterator< const PxVec3 >::operator<=(), PxStrideIterator< const PxVec3 >::operator==(), PxStrideIterator< const PxVec3 >::operator>(), and PxStrideIterator< const PxVec3 >::operator>=().
PxU32 PxStrideIterator< T >::mStride [private] |
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com