PxArticulationJoint Class Reference
[Physics]
a joint between two links in an articulation.
More...
#include <PxArticulationJoint.h>
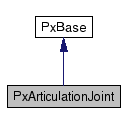
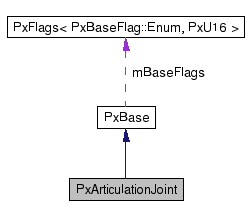
Public Member Functions | |
virtual void | setParentPose (const PxTransform &pose)=0 |
set the joint pose in the parent frame | |
virtual PxTransform | getParentPose () const =0 |
get the joint pose in the parent frame | |
virtual void | setChildPose (const PxTransform &pose)=0 |
set the joint pose in the child frame | |
virtual PxTransform | getChildPose () const =0 |
get the joint pose in the child frame | |
virtual void | setTargetOrientation (const PxQuat &orientation)=0 |
set the target drive | |
virtual PxQuat | getTargetOrientation () const =0 |
get the target drive position | |
virtual void | setTargetVelocity (const PxVec3 &velocity)=0 |
set the target drive velocity | |
virtual PxVec3 | getTargetVelocity () const =0 |
get the target drive velocity | |
virtual void | setDriveType (PxArticulationJointDriveType::Enum driveType)=0 |
set the drive type | |
virtual PxArticulationJointDriveType::Enum | getDriveType () const =0 |
get the drive type | |
virtual void | setStiffness (PxReal spring)=0 |
set the drive strength of the joint acceleration spring. | |
virtual PxReal | getStiffness () const =0 |
get the drive strength of the joint acceleration spring | |
virtual void | setDamping (PxReal damping)=0 |
set the damping of the joint acceleration spring | |
virtual PxReal | getDamping () const =0 |
get the damping of the joint acceleration spring | |
virtual void | setInternalCompliance (PxReal compliance)=0 |
set the internal compliance | |
virtual PxReal | getInternalCompliance () const =0 |
get the internal compliance | |
virtual void | setExternalCompliance (PxReal compliance)=0 |
get the drive external compliance | |
virtual PxReal | getExternalCompliance () const =0 |
get the drive external compliance | |
virtual void | setSwingLimit (PxReal zLimit, PxReal yLimit)=0 |
set the extents of the cone limit. The extents are measured in the frame of the parent. | |
virtual void | getSwingLimit (PxReal &zLimit, PxReal &yLimit) const =0 |
get the extents for the swing limit cone | |
virtual void | setTangentialStiffness (PxReal spring)=0 |
set the tangential spring for the limit cone Range: ([0, PX_MAX_F32), [0, PX_MAX_F32)) Default: (0.0, 0.0) | |
virtual PxReal | getTangentialStiffness () const =0 |
get the tangential spring for the swing limit cone | |
virtual void | setTangentialDamping (PxReal damping)=0 |
set the tangential damping for the limit cone Range: ([0, PX_MAX_F32), [0, PX_MAX_F32)) Default: (0.0, 0.0) | |
virtual PxReal | getTangentialDamping () const =0 |
get the tangential damping for the swing limit cone | |
virtual void | setSwingLimitContactDistance (PxReal contactDistance)=0 |
set the contact distance for the swing limit | |
virtual PxReal | getSwingLimitContactDistance () const =0 |
get the contact distance for the swing limit | |
virtual void | setSwingLimitEnabled (bool enabled)=0 |
set the flag which enables the swing limit | |
virtual bool | getSwingLimitEnabled () const =0 |
get the flag which enables the swing limit | |
virtual void | setTwistLimit (PxReal lower, PxReal upper)=0 |
set the bounds of the twistLimit | |
virtual void | getTwistLimit (PxReal &lower, PxReal &upper) const =0 |
get the bounds of the twistLimit | |
virtual void | setTwistLimitEnabled (bool enabled)=0 |
set the flag which enables the twist limit | |
virtual bool | getTwistLimitEnabled () const =0 |
get the twistLimitEnabled flag | |
virtual void | setTwistLimitContactDistance (PxReal contactDistance)=0 |
set the contact distance for the swing limit | |
virtual PxReal | getTwistLimitContactDistance () const =0 |
get the contact distance for the swing limit | |
virtual const char * | getConcreteTypeName () const |
Returns string name of dynamic type. | |
Protected Member Functions | |
PX_INLINE | PxArticulationJoint (PxType concreteType, PxBaseFlags baseFlags) |
PX_INLINE | PxArticulationJoint (PxBaseFlags baseFlags) |
virtual | ~PxArticulationJoint () |
virtual bool | isKindOf (const char *name) const |
Returns whether a given type name matches with the type of this instance. |
Detailed Description
a joint between two links in an articulation.The joint model is very similar to a PxSphericalJoint with swing and twist limits, and an implicit drive model.
- See also:
- PxArticulation PxArticulationLink
Constructor & Destructor Documentation
PX_INLINE PxArticulationJoint::PxArticulationJoint | ( | PxType | concreteType, | |
PxBaseFlags | baseFlags | |||
) | [inline, protected] |
PX_INLINE PxArticulationJoint::PxArticulationJoint | ( | PxBaseFlags | baseFlags | ) | [inline, protected] |
virtual PxArticulationJoint::~PxArticulationJoint | ( | ) | [inline, protected, virtual] |
Member Function Documentation
virtual PxTransform PxArticulationJoint::getChildPose | ( | ) | const [pure virtual] |
get the joint pose in the child frame
- Returns:
- the joint pose in the child frame
- See also:
- setChildPose()
virtual const char* PxArticulationJoint::getConcreteTypeName | ( | ) | const [inline, virtual] |
Returns string name of dynamic type.
- Returns:
- Class name of most derived type of this object.
Implements PxBase.
virtual PxReal PxArticulationJoint::getDamping | ( | ) | const [pure virtual] |
virtual PxArticulationJointDriveType::Enum PxArticulationJoint::getDriveType | ( | ) | const [pure virtual] |
virtual PxReal PxArticulationJoint::getExternalCompliance | ( | ) | const [pure virtual] |
get the drive external compliance
- Returns:
- the compliance to external forces
- See also:
- setExternalCompliance()
virtual PxReal PxArticulationJoint::getInternalCompliance | ( | ) | const [pure virtual] |
get the internal compliance
- Returns:
- the compliance to internal forces
- See also:
- setInternalCompliance()
virtual PxTransform PxArticulationJoint::getParentPose | ( | ) | const [pure virtual] |
get the joint pose in the parent frame
- Returns:
- the joint pose in the parent frame
- See also:
- setParentPose()
virtual PxReal PxArticulationJoint::getStiffness | ( | ) | const [pure virtual] |
get the drive strength of the joint acceleration spring
- Returns:
- the spring strength of the joint
- See also:
- setStiffness()
virtual void PxArticulationJoint::getSwingLimit | ( | PxReal & | zLimit, | |
PxReal & | yLimit | |||
) | const [pure virtual] |
get the extents for the swing limit cone
- Parameters:
-
[out] zLimit the allowed extent of rotation around the z-axis [out] yLimit the allowed extent of rotation around the y-axis
- Note:
- Please note the order of zLimit and yLimit.
- See also:
- setSwingLimit()
virtual PxReal PxArticulationJoint::getSwingLimitContactDistance | ( | ) | const [pure virtual] |
get the contact distance for the swing limit
- Returns:
- the contact distance for the swing limit cone
- See also:
- setSwingLimitContactDistance()
virtual bool PxArticulationJoint::getSwingLimitEnabled | ( | ) | const [pure virtual] |
get the flag which enables the swing limit
- Returns:
- whether the swing limit is enabled
- See also:
- setSwingLimitEnabled()
virtual PxReal PxArticulationJoint::getTangentialDamping | ( | ) | const [pure virtual] |
get the tangential damping for the swing limit cone
- Returns:
- the tangential damping
- See also:
- setTangentialDamping()
virtual PxReal PxArticulationJoint::getTangentialStiffness | ( | ) | const [pure virtual] |
get the tangential spring for the swing limit cone
- Returns:
- the tangential spring
- See also:
- setTangentialStiffness()
virtual PxQuat PxArticulationJoint::getTargetOrientation | ( | ) | const [pure virtual] |
get the target drive position
- Returns:
- the joint drive target position
- See also:
- setTargetOrientation()
virtual PxVec3 PxArticulationJoint::getTargetVelocity | ( | ) | const [pure virtual] |
get the target drive velocity
- Returns:
- the target velocity for the joint
- See also:
- setTargetVelocity()
virtual void PxArticulationJoint::getTwistLimit | ( | PxReal & | lower, | |
PxReal & | upper | |||
) | const [pure virtual] |
get the bounds of the twistLimit
- Parameters:
-
[out] lower the lower extent of the twist limit [out] upper the upper extent of the twist limit
- See also:
- setTwistLimit()
virtual PxReal PxArticulationJoint::getTwistLimitContactDistance | ( | ) | const [pure virtual] |
get the contact distance for the swing limit
- Returns:
- the contact distance for the twist limit
- See also:
- setTwistLimitContactDistance()
virtual bool PxArticulationJoint::getTwistLimitEnabled | ( | ) | const [pure virtual] |
get the twistLimitEnabled flag
- Returns:
- whether the twist limit is enabled
- See also:
- setTwistLimitEnabled()
virtual bool PxArticulationJoint::isKindOf | ( | const char * | superClass | ) | const [inline, protected, virtual] |
Returns whether a given type name matches with the type of this instance.
Reimplemented from PxBase.
References PxBase::isKindOf().
virtual void PxArticulationJoint::setChildPose | ( | const PxTransform & | pose | ) | [pure virtual] |
set the joint pose in the child frame
- Parameters:
-
[in] pose the joint pose in the child frame Default: the identity matrix
- See also:
- getChildPose()
virtual void PxArticulationJoint::setDamping | ( | PxReal | damping | ) | [pure virtual] |
set the damping of the joint acceleration spring
The acceleration generated by the spring drive is proportional to this value and the difference between the angular velocity of the joint and the target drive velocity.
- Parameters:
-
[in] damping the damping of the joint drive Range: [0, PX_MAX_F32)
Default: 0.0
- See also:
- getDamping()
virtual void PxArticulationJoint::setDriveType | ( | PxArticulationJointDriveType::Enum | driveType | ) | [pure virtual] |
set the drive type
- Parameters:
-
[in] driveType the drive type for the joint Default: PxArticulationJointDriveType::eTARGET
- See also:
- getDriveType()
virtual void PxArticulationJoint::setExternalCompliance | ( | PxReal | compliance | ) | [pure virtual] |
get the drive external compliance
Compliance determines the extent to which the joint resists acceleration.
There are separate values for resistance to accelerations caused by external forces such as gravity and contact forces, and internal forces generated from other joints.
A low compliance means that forces have little effect, a compliance of 1 means the joint does not resist such forces at all.
- Parameters:
-
[in] compliance the compliance to external forces Range: (0, 1] Default: 0.0
- See also:
- getExternalCompliance()
virtual void PxArticulationJoint::setInternalCompliance | ( | PxReal | compliance | ) | [pure virtual] |
set the internal compliance
Compliance determines the extent to which the joint resists acceleration.
There are separate values for resistance to accelerations caused by external forces such as gravity and contact forces, and internal forces generated from other joints.
A low compliance means that forces have little effect, a compliance of 1 means the joint does not resist such forces at all.
- Parameters:
-
[in] compliance the compliance to internal forces Range: (0, 1] Default: 0.0
- See also:
- getInternalCompliance()
virtual void PxArticulationJoint::setParentPose | ( | const PxTransform & | pose | ) | [pure virtual] |
set the joint pose in the parent frame
- Parameters:
-
[in] pose the joint pose in the parent frame Default: the identity matrix
- See also:
- getParentPose()
virtual void PxArticulationJoint::setStiffness | ( | PxReal | spring | ) | [pure virtual] |
set the drive strength of the joint acceleration spring.
The acceleration generated by the spring drive is proportional to this value and the angle between the drive target position and the current position.
- Parameters:
-
[in] spring the spring strength of the joint Range: [0, PX_MAX_F32)
Default: 0.0
- See also:
- getStiffness()
virtual void PxArticulationJoint::setSwingLimit | ( | PxReal | zLimit, | |
PxReal | yLimit | |||
) | [pure virtual] |
set the extents of the cone limit. The extents are measured in the frame of the parent.
Note that very small or highly elliptical limit cones may result in jitter.
- Parameters:
-
[in] zLimit the allowed extent of rotation around the z-axis [in] yLimit the allowed extent of rotation around the y-axis Range: ( (0, Pi), (0, Pi) ) Default: (Pi/4, Pi/4)
- Note:
- Please note the order of zLimit and yLimit.
virtual void PxArticulationJoint::setSwingLimitContactDistance | ( | PxReal | contactDistance | ) | [pure virtual] |
set the contact distance for the swing limit
The contact distance should be less than either limit angle.
Range: [0, Pi] Default: 0.05 radians
- See also:
- getSwingLimitContactDistance()
virtual void PxArticulationJoint::setSwingLimitEnabled | ( | bool | enabled | ) | [pure virtual] |
set the flag which enables the swing limit
- Parameters:
-
[in] enabled whether the limit is enabled Default: false
- See also:
- getSwingLimitEnabled()
virtual void PxArticulationJoint::setTangentialDamping | ( | PxReal | damping | ) | [pure virtual] |
set the tangential damping for the limit cone Range: ([0, PX_MAX_F32), [0, PX_MAX_F32)) Default: (0.0, 0.0)
virtual void PxArticulationJoint::setTangentialStiffness | ( | PxReal | spring | ) | [pure virtual] |
set the tangential spring for the limit cone Range: ([0, PX_MAX_F32), [0, PX_MAX_F32)) Default: (0.0, 0.0)
virtual void PxArticulationJoint::setTargetOrientation | ( | const PxQuat & | orientation | ) | [pure virtual] |
set the target drive
This is the target position for the joint drive, measured in the parent constraint frame.
- Parameters:
-
[in] orientation the target orientation for the joint Range: a unit quaternion Default: the identity quaternion
- See also:
- getTargetOrientation()
virtual void PxArticulationJoint::setTargetVelocity | ( | const PxVec3 & | velocity | ) | [pure virtual] |
set the target drive velocity
This is the target velocity for the joint drive, measured in the parent constraint frame
- Parameters:
-
[in] velocity the target velocity for the joint Default: the zero vector
- See also:
- getTargetVelocity()
virtual void PxArticulationJoint::setTwistLimit | ( | PxReal | lower, | |
PxReal | upper | |||
) | [pure virtual] |
set the bounds of the twistLimit
- Parameters:
-
[in] lower the lower extent of the twist limit [in] upper the upper extent of the twist limit Range: (-Pi, Pi) Default: (-Pi/4, Pi/4)
- See also:
- getTwistLimit()
virtual void PxArticulationJoint::setTwistLimitContactDistance | ( | PxReal | contactDistance | ) | [pure virtual] |
set the contact distance for the swing limit
The contact distance should be less than half the distance between the upper and lower limits.
Range: [0, Pi) Default: 0.05 radians
- See also:
- getTwistLimitContactDistance()
virtual void PxArticulationJoint::setTwistLimitEnabled | ( | bool | enabled | ) | [pure virtual] |
set the flag which enables the twist limit
- Parameters:
-
[in] enabled whether the twist limit is enabled Default: false
- See also:
- getTwistLimitEnabled()
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com