GLLibPlayer Class Reference
[GLLibPlayer]
Generic GLLib Player.
More...
Inherits Runnable.
Public Member Functions | |
void | run () |
Implementation of the Runnable interface. | |
Public Attributes | |
int | curTime |
Sprite current time. Used for frame that stay on screen longer than a frame. | |
Static Public Attributes | |
static final int | WRAP_CLAMP = 0 |
wrappng parameter, specify that the tileset is to be repeated only once | |
static final int | WRAP_REPEAT = 1 |
wrappng parameter, specify that the tileset is to be repeated indefinitely | |
Static Protected Member Functions | |
static boolean | Snd_IsPlaying (int channel) throws Exception |
Return true if a sound is currently playing on channel. | |
Package Functions | |
final int | GetAnim () |
get animation | |
final int | GetDuration () |
Get the duration of the current frame of the current animation. | |
final int | GetFrame () |
get current frame nb | |
int | GetNbanim () |
Get the animation count of the current sprite. | |
int | GetNbFrame () |
Get the frame count of the current animation. | |
final ASprite | GetSprite () |
Get the current sprite. | |
final int | GetTransform () |
get current sprite transformation | |
GLLibPlayer (ASprite sprite, int x, int y) | |
Basic constructor. | |
GLLibPlayer () | |
Empty constructor. | |
boolean | IsAnimOver () |
Query the state of the current animation to see if its over. | |
void | Render () |
Render the curreny animation in GLLib curent graphic context. | |
void | Reset () |
Reset the current player. | |
void | SetAnim (int anim, int nbLoop) |
Set a new animation number to play. | |
final void | SetAnim (int anim) |
Set a new animation number to play, animation will loop forever. | |
int | SetFrame (int frame) |
Set a new frame position in the current animation. | |
final void | SetPos (int x, int y) |
Set new position of sprite. | |
void | SetSprite (ASprite sprite) |
Set a new ASprite reference in the player. | |
final void | SetTransform (int transform) |
set current sprite transformation | |
Object | Snd_GetChannelPlayer (int channel) |
void | Update (int DT) |
Update current animation time from 1 time unit. | |
final void | Update () |
Update current animation time from 1 time unit. | |
Static Package Functions | |
static long | Snd_DurationGet (int channel) throws Exception |
get duration of a sound | |
static void | Snd_ForceExecOnThreadOnGamePause () |
When the game receive an interupt, it can be important to force execution of the sound thread. | |
static final void | Snd_FreeChannel (int channel) |
Free sound channel from the player asigned to it. | |
static int | Snd_GetChannelVolume (int channel) throws Exception |
Get the current volume of a channel. | |
static int | Snd_GetCurrentSoundIndex (int nChannel) |
Get the index of the sound loaded on the channel. | |
static void | Snd_Init (int nbSoundSlot) throws Exception |
Allocate sound player and resources. | |
static void | Snd_LoadSound (byte[] soundData, int nMIME, int index, boolean bCacheThisSound) throws Exception |
Load a sound file/resource from data package. | |
static void | Snd_LoadSound (byte[] soundData, int nMIME, int index) throws Exception |
Load a sound file/resource from data package. | |
static void | Snd_LoadSound (String dataFileName, int resourceIndex, boolean bCacheThisSound) throws Exception |
Load a sound file/resource from data package. | |
static void | Snd_LoadSound (String dataFileName, int resourceIndex) throws Exception |
Load a sound file/resource from data package. | |
static long | Snd_MediaTimeGet (int channel) |
get current media time | |
static long | Snd_MediaTimeSet (int channel, long time) |
set current media time | |
static void | Snd_MidiPlayNote (int channel, int MIDIChannel, int note, int volume) throws Exception |
play a note on a midi channel. | |
static boolean | Snd_MidiSetChannelVolume (int channel, int MIDIChannel, int volume) throws Exception |
adjust volume of a midi channel | |
static final void | Snd_Pause (int channel) |
Pause sound on a channel. | |
static void | Snd_Play (int channel, int index, int loop, int volume, int priority) |
Play a sound on a channel. | |
static void | Snd_PrepareSound (int channel, int index, int priority) |
Prepare a sound to be played on a channel. | |
static void | Snd_Quit () throws Exception |
Deallocate all sound ressources and players. | |
static int | Snd_RateGet (int channel) |
get rate of a sound | |
static int | Snd_RateGetMax (int channel) throws Exception |
get max rate of a sound | |
static int | Snd_RateGetMin (int channel) throws Exception |
get min rate of a sound | |
static boolean | Snd_RateSet (int channel, int rate) throws Exception |
set rate of a sound | |
static final void | Snd_Resume (int channel) |
Resume currently paused sound on a channel. | |
static void | Snd_SetMasterVolume (int volume) throws Exception |
Change master volume value. | |
static void | Snd_SetMediaDuration (int index, int duration) throws Exception |
Sets duration for the sound (Use with GLLibConfig.sound_useFakeMediaDuration == true). | |
static final void | Snd_Stop (int channel) |
Stop sound on a channel. | |
static void | Snd_StopAllSounds () |
Stop all sounds on all channel. | |
static int | Snd_TempoGet (int channel) throws Exception |
get tempo of a sound | |
static boolean | Snd_TempoSet (int channel, int tempo) throws Exception |
set tempo of a sound | |
static void | Snd_UnLoadSound (int index) throws Exception |
Unload a sound resource from memory. | |
static void | Snd_Update () |
Update sound engine status all sound ressource. | |
static void | Tileset_Destroy (int nLayer, boolean bFreeBufferImage) |
Delete a layer from the player. | |
static void | Tileset_Destroy (int nLayer) |
Delete a layer from the player. | |
static void | Tileset_Draw (Graphics g, int dx, int dy, int nLayer) |
Draw a specific onto destination Graphics. | |
static void | Tileset_Draw (Graphics g, int nLayer) |
Draw a specific onto destination Graphics. | |
static final Graphics | Tileset_GetBufferGraphics (int p_iLayer, int p_iImage) |
Get the graphics context of one of the images being used as a buffer for this layer. | |
static final Graphics | Tileset_GetBufferGraphics (int p_iLayer) |
Get the graphics context of the image being used as a buffer for this layer. | |
static final Image | Tileset_GetBufferImage (int p_iLayer, int p_iImage) |
Get one of the images that is being used as a buffer for this layer. | |
static final Image | Tileset_GetBufferImage (int p_iLayer) |
Get the image that is the buffer for this layer. | |
static final int[] | Tileset_GetCamera (int nLayer) |
Get the camera position of a specific layer. | |
static final int | Tileset_GetCameraX (int nLayer) |
Get the camera X position of a specific layer. | |
static final int | Tileset_GetCameraY (int nLayer) |
Get the camera Y position of a specific layer. | |
static final int | Tileset_GetLayerHeight (int nLayer) |
Get the pixel height of a specific layer. | |
static final int | Tileset_GetLayerTileCountHeight (int nLayer) |
Get the tile count height of a specific layer. | |
static final int | Tileset_GetLayerTileCountWidth (int nLayer) |
Get the tile count width of a specific layer. | |
static final int | Tileset_GetLayerWidth (int nLayer) |
Get the pixel width of a specific layer. | |
static final int | Tileset_GetTile (int nLayer, int x, int y) |
Get value of a specific tile. | |
static final int | Tileset_GetTileFlags (int nLayer, int x, int y) |
Get the flags information of a specific tile. | |
static void | Tileset_Init (int nDestWidth, int nDestHeight, int nTileWidth, int nTileHeight) |
Initialize the GLLibPlayerTileSet engine. | |
static void | Tileset_LoadLayer (int nLayer, byte[] MapSizes, byte[] MapData, byte[] MapFlip, ASprite MapSprite, int iUseCB, int origin, int wrappingX, int wrappingY) |
Load a tileset layer into the player. | |
static void | Tileset_LoadLayer (int nLayer, byte[] MapSizes, byte[] MapData, byte[] MapFlip, ASprite MapSprite, boolean bUseCB, int origin, int wrappingX, int wrappingY) |
Load a tileset layer into the player. | |
static final void | Tileset_SetCamera (int nLayer, int x, int y) |
Set the camera position of a specific layer. | |
static void | Tileset_Update (int nLayer) |
Update a specific layer circular buffer (back buffer) but does nothing if this layer is not using a circular buffer. | |
Package Attributes | |
int | curFlags |
Sprite player current display flags. | |
int | posX |
Sprite player current X position. | |
int | posY |
Sprite player current Y position. | |
ASprite | sprite |
Sprite player current ASprite reference. | |
Static Package Attributes | |
static final int | k_snd_priority_highest = 0 |
Sound priorities constant. | |
static final int | k_snd_priority_lowest = 15 |
Sound priorities constant. | |
static final int | k_snd_priority_normal = 7 |
Sound priorities constant. | |
static String[] | s_data_mimeType |
Sound Mime Container, to know the sound type of each slots. | |
static int | s_snd_masterVolume |
Sound engine master volume (0-100). The volume will be scaled to the phone range. | |
static int | s_snd_maxNbSoundSlot |
Sound maximum slot count for this game. The maximum number of sounds that can be loaded into memory. |
Detailed Description
Generic GLLib Player.This class is used to Play ASprite animations and to play Musics/Sounds FX.
The Animation part of the player will use ASprite animation. You can have many instances of the player using the same ASprite to keep track of each animation state, progression and position.
The Sound part of the player is unique so it does not have to be instanciated. Once initiated, you can access it statically from everywhere in you code.
Generated on Tue Sep 23 23:05:32 2008 for GLLib by
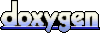