GLLibPlayer : SoundPlayer
[GLLibPlayer]
sound player
More...Functions | |
void | run () |
Implementation of the Runnable interface. | |
static long | Snd_DurationGet (int channel) throws Exception |
get duration of a sound | |
static void | Snd_ForceExecOnThreadOnGamePause () |
When the game receive an interupt, it can be important to force execution of the sound thread. | |
static final void | Snd_FreeChannel (int channel) |
Free sound channel from the player asigned to it. | |
Object | Snd_GetChannelPlayer (int channel) |
static int | Snd_GetChannelVolume (int channel) throws Exception |
Get the current volume of a channel. | |
static int | Snd_GetCurrentSoundIndex (int nChannel) |
Get the index of the sound loaded on the channel. | |
static void | Snd_Init (int nbSoundSlot) throws Exception |
Allocate sound player and resources. | |
static boolean | Snd_IsPlaying (int channel) throws Exception |
Return true if a sound is currently playing on channel. | |
static void | Snd_LoadSound (byte[] soundData, int nMIME, int index, boolean bCacheThisSound) throws Exception |
Load a sound file/resource from data package. | |
static void | Snd_LoadSound (byte[] soundData, int nMIME, int index) throws Exception |
Load a sound file/resource from data package. | |
static void | Snd_LoadSound (String dataFileName, int resourceIndex, boolean bCacheThisSound) throws Exception |
Load a sound file/resource from data package. | |
static void | Snd_LoadSound (String dataFileName, int resourceIndex) throws Exception |
Load a sound file/resource from data package. | |
static long | Snd_MediaTimeGet (int channel) |
get current media time | |
static long | Snd_MediaTimeSet (int channel, long time) |
set current media time | |
static void | Snd_MidiPlayNote (int channel, int MIDIChannel, int note, int volume) throws Exception |
play a note on a midi channel. | |
static boolean | Snd_MidiSetChannelVolume (int channel, int MIDIChannel, int volume) throws Exception |
adjust volume of a midi channel | |
static final void | Snd_Pause (int channel) |
Pause sound on a channel. | |
static void | Snd_Play (int channel, int index, int loop, int volume, int priority) |
Play a sound on a channel. | |
static void | Snd_PrepareSound (int channel, int index, int priority) |
Prepare a sound to be played on a channel. | |
static void | Snd_Quit () throws Exception |
Deallocate all sound ressources and players. | |
static int | Snd_RateGet (int channel) |
get rate of a sound | |
static int | Snd_RateGetMax (int channel) throws Exception |
get max rate of a sound | |
static int | Snd_RateGetMin (int channel) throws Exception |
get min rate of a sound | |
static boolean | Snd_RateSet (int channel, int rate) throws Exception |
set rate of a sound | |
static final void | Snd_Resume (int channel) |
Resume currently paused sound on a channel. | |
static void | Snd_SetMasterVolume (int volume) throws Exception |
Change master volume value. | |
static void | Snd_SetMediaDuration (int index, int duration) throws Exception |
Sets duration for the sound (Use with GLLibConfig.sound_useFakeMediaDuration == true). | |
static final void | Snd_Stop (int channel) |
Stop sound on a channel. | |
static void | Snd_StopAllSounds () |
Stop all sounds on all channel. | |
static int | Snd_TempoGet (int channel) throws Exception |
get tempo of a sound | |
static boolean | Snd_TempoSet (int channel, int tempo) throws Exception |
set tempo of a sound | |
static void | Snd_UnLoadSound (int index) throws Exception |
Unload a sound resource from memory. | |
static void | Snd_Update () |
Update sound engine status all sound ressource. | |
Variables | |
static final int | k_snd_priority_highest = 0 |
Sound priorities constant. | |
static final int | k_snd_priority_lowest = 15 |
Sound priorities constant. | |
static final int | k_snd_priority_normal = 7 |
Sound priorities constant. | |
static String[] | s_data_mimeType |
Sound Mime Container, to know the sound type of each slots. | |
static int | s_snd_masterVolume |
Sound engine master volume (0-100). The volume will be scaled to the phone range. | |
static int | s_snd_maxNbSoundSlot |
Sound maximum slot count for this game. The maximum number of sounds that can be loaded into memory. |
Detailed Description
sound playerGLLibPlayer does not need to be instanciated to use those function
Function Documentation
void run | ( | ) | [inherited] |
Implementation of the Runnable interface.
This function will be used only when the GLLibPlayer is used to play sounds. When the GLLibPlayer is instanciated to play anymations, this functions is doing nothing.
static long Snd_DurationGet | ( | int | channel | ) | throws Exception [static, package, inherited] |
get duration of a sound
- Parameters:
-
channel - channel of the sound
- Note:
- will only work on MIDP20 device with GLLibConfig.sound_useJSR135=true
- Returns:
- duration of the sound
static void Snd_ForceExecOnThreadOnGamePause | ( | ) | [static, package, inherited] |
When the game receive an interupt, it can be important to force execution of the sound thread.
Its going to work only if GLLib.s_game_isPaused is True.
static final void Snd_FreeChannel | ( | int | channel | ) | [static, package, inherited] |
Free sound channel from the player asigned to it.
- Parameters:
-
channel Channel to prepare the sound on.
Object Snd_GetChannelPlayer | ( | int | channel | ) | [package, inherited] |
static int Snd_GetChannelVolume | ( | int | channel | ) | throws Exception [static, package, inherited] |
Get the current volume of a channel.
Scaled to (0-100) (API independent, Master Volume independent)
- Parameters:
-
channel whose current volume we want
- Returns:
- the volume with which this channel is playing (0-100)
- Note:
- Not implemented for Nokia API
static int Snd_GetCurrentSoundIndex | ( | int | nChannel | ) | [static, package, inherited] |
Get the index of the sound loaded on the channel.
- Parameters:
-
nChannel Channel to query for the loaded sound index.
- Returns:
- The sound index of the channel. or -1 if there is no sound loaded.
- Note:
- The dummy sound are never loaded and will always return -1.
static void Snd_Init | ( | int | nbSoundSlot | ) | throws Exception [static, package, inherited] |
Allocate sound player and resources.
Init sound container.
- Parameters:
-
nbSoundSlot number of sounds file you want to have in memory.
- Note:
- This call does not load the sound files (mid,wav,mp3...) in memory.
Also this is not the number of sound your phone can play at once ( Channels ).
static boolean Snd_IsPlaying | ( | int | channel | ) | throws Exception [static, protected, inherited] |
Return true if a sound is currently playing on channel.
- Parameters:
-
channel Channel where the sound is suposed to play.
- Note:
- dummy sound are always NOT playing.
static void Snd_LoadSound | ( | byte[] | soundData, | |
int | nMIME, | |||
int | index, | |||
boolean | bCacheThisSound | |||
) | throws Exception [static, package, inherited] |
Load a sound file/resource from data package.
- Parameters:
-
soundData Byte array containing the sound data to be loaded. nMIME MIME type of the data in soundData. index Index of the sound, to ask to play/stop/pause/free... it. bCacheThisSound this sound will be cached. Works ONLY if sound_useCachedPlayers is true.
- Warning:
- index Must be smaller than the Slot count when you initialized the player.
static void Snd_LoadSound | ( | byte[] | soundData, | |
int | nMIME, | |||
int | index | |||
) | throws Exception [static, package, inherited] |
Load a sound file/resource from data package.
- Parameters:
-
soundData Byte array containing the sound data to be loaded. nMIME MIME type of the data in soundData. index Index of the sound, to ask to play/stop/pause/free... it.
- Warning:
- index Must be smaller than the Slot count when you initialized the player.
static void Snd_LoadSound | ( | String | dataFileName, | |
int | resourceIndex, | |||
boolean | bCacheThisSound | |||
) | throws Exception [static, package, inherited] |
Load a sound file/resource from data package.
- Parameters:
-
dataFileName Path+filename of the file to load the sound from. resourceIndex Resource index of sound to load in the dataFileName resource file. bCacheThisSound this sound will be cached. Works ONLY if sound_useCachedPlayers is true.
- Warning:
- resourceIndex is the index in the resource pack BUT it will be also the index to play/stop the sound. So make sure that all of your sound are at the begining of a pack, because if your sound are at the end of a resource pack with resourceIndex ranging from 100 to 110, you will have to init the sound library with 120 slot or you will have out of bound exception.
- Note:
- If resourceIndex is invalid no error is raised because some version of the game have less sound than others, doing this, we can keep the code unchanged for each version.
This function is using GLLib package management.
- See also:
- GLLib.Pack_ReadData
static void Snd_LoadSound | ( | String | dataFileName, | |
int | resourceIndex | |||
) | throws Exception [static, package, inherited] |
Load a sound file/resource from data package.
- Parameters:
-
dataFileName Path+filename of the file to load the sound from. resourceIndex Resource index of sound to load in the dataFileName resource file.
- Warning:
- resourceIndex is the index in the resource pack BUT it will be also the index to play/stop the sound. So make sure that all of your sound are at the begining of a pack, because if your sound are at the end of a resource pack with resourceIndex ranging from 100 to 110, you will have to init the sound library with 120 slot or you will have out of bound exception.
- Note:
- If resourceIndex is invalid no error is raised because some version of the game have less sound than others, doing this, we can keep the code unchanged for each version.
This function is using GLLib package management.
- See also:
- GLLib.Pack_ReadData
static long Snd_MediaTimeGet | ( | int | channel | ) | [static, package, inherited] |
get current media time
- Parameters:
-
channel - channel on which the midi sound is currently playing
- Returns:
- current media time or -1 if unknown or not recoverable
static long Snd_MediaTimeSet | ( | int | channel, | |
long | time | |||
) | [static, package, inherited] |
set current media time
- Parameters:
-
channel - channel on which the midi sound is currently playing time - time to set media on
- Returns:
- current media time or -1 if unknown or not recoverable or failed
static void Snd_MidiPlayNote | ( | int | channel, | |
int | MIDIChannel, | |||
int | note, | |||
int | volume | |||
) | throws Exception [static, package, inherited] |
play a note on a midi channel.
a clean implementation should issue a note off( volume=0) event to finish playing a note
- Parameters:
-
channel - channel on which the midi sound is currently playing MIDIChannel - midi channel to play note on [0-15] note - the note to play volume - volume to set [0=note off, 126]
- Note:
- will only work on MIDP20 device with GLLibConfig.sound_useJSR135=true
static boolean Snd_MidiSetChannelVolume | ( | int | channel, | |
int | MIDIChannel, | |||
int | volume | |||
) | throws Exception [static, package, inherited] |
adjust volume of a midi channel
- Parameters:
-
channel - channel on which the midi sound is currently playing MIDIChannel - midi channel to alter [0-15] volume - volume to set [0=off, 126]
- Returns:
- true if it was able to set volume
- Note:
- will only work on MIDP20 device with GLLibConfig.sound_useJSR135=true
this command may be overridde by the midi file itself if it issues a setvolume comand itself later
static final void Snd_Pause | ( | int | channel | ) | [static, package, inherited] |
Pause sound on a channel.
If this channel is not currently playing a sound, nothing will be done.
- Parameters:
-
channel Channel of the sound to pause.
static void Snd_Play | ( | int | channel, | |
int | index, | |||
int | loop, | |||
int | volume, | |||
int | priority | |||
) | [static, package, inherited] |
Play a sound on a channel.
If the sound was not prepared it will be done before playing.
- Parameters:
-
channel Channel to play the sound on. index Index of BGM to play. loop Number of time to play this sound. (0:infinite) volume Volume of BGM (0-100). priority Priority of this sound. (0:biggest - 15:lowest)
- See also:
- Snd_prepareSound
static void Snd_PrepareSound | ( | int | channel, | |
int | index, | |||
int | priority | |||
) | [static, package, inherited] |
Prepare a sound to be played on a channel.
If there is allready a sound prepared, this call will be ignored if the new priority is lower than the current priority. If the new priority is higher of equal, this new request will be executed.
- Parameters:
-
channel Channel to prepare the sound on. index Index of the sound to be played. priority Priority of this request. (0:highest - 15:lowest)
static void Snd_Quit | ( | ) | throws Exception [static, package, inherited] |
Deallocate all sound ressources and players.
- Note:
- All sound loaded with Snd_loadSound are going to be freed from memory.
static int Snd_RateGet | ( | int | channel | ) | [static, package, inherited] |
get rate of a sound
- Parameters:
-
channel - channel of the sound to alter the rate
- Note:
- will only work on MIDP20 device with GLLibConfig.sound_useJSR135=true
- Returns:
- current sound rate (-1 if unable to get it)
static int Snd_RateGetMax | ( | int | channel | ) | throws Exception [static, package, inherited] |
get max rate of a sound
- Parameters:
-
channel - channel of the sound
- Note:
- will only work on MIDP20 device with GLLibConfig.sound_useJSR135=true
- Returns:
- max rate value
static int Snd_RateGetMin | ( | int | channel | ) | throws Exception [static, package, inherited] |
get min rate of a sound
- Parameters:
-
channel - channel of the sound
- Note:
- will only work on MIDP20 device with GLLibConfig.sound_useJSR135=true
- Returns:
- min rate value
static boolean Snd_RateSet | ( | int | channel, | |
int | rate | |||
) | throws Exception [static, package, inherited] |
set rate of a sound
- Parameters:
-
channel - channel of the sound to alter the rate rate - rate to set (100000 = 100 % speed)
- Note:
- will only work on MIDP20 device with GLLibConfig.sound_useJSR135=true
- Returns:
- true if it was able to set rate
static final void Snd_Resume | ( | int | channel | ) | [static, package, inherited] |
Resume currently paused sound on a channel.
If there is no paused sound, nothing will be done.
- Parameters:
-
channel Channel of the sound to resume.
static void Snd_SetMasterVolume | ( | int | volume | ) | throws Exception [static, package, inherited] |
Change master volume value.
- Parameters:
-
volume The new volume value. (0 - 100)
- Note:
- It may not be used until the next Snd_play.
static void Snd_SetMediaDuration | ( | int | index, | |
int | duration | |||
) | throws Exception [static, package, inherited] |
Sets duration for the sound (Use with GLLibConfig.sound_useFakeMediaDuration == true).
- Parameters:
-
index Sound slot index duration duration.
- Note:
- Use with GLLibConfig.sound_useFakeMediaDuration == true
static final void Snd_Stop | ( | int | channel | ) | [static, package, inherited] |
Stop sound on a channel.
If this channel is not currently playing a sound, nothing will be done.
- Parameters:
-
channel Channel of the sound to stop.
static void Snd_StopAllSounds | ( | ) | [static, package, inherited] |
Stop all sounds on all channel.
static int Snd_TempoGet | ( | int | channel | ) | throws Exception [static, package, inherited] |
get tempo of a sound
- Parameters:
-
channel - channel of the sound to get the tempo
- Note:
- will only work on MIDP20 device with GLLibConfig.sound_useJSR135=true
- Returns:
- tempo or -1 if unable to get it
static boolean Snd_TempoSet | ( | int | channel, | |
int | tempo | |||
) | throws Exception [static, package, inherited] |
set tempo of a sound
- Parameters:
-
channel - channel of the sound to alter the tempo tempo - tempo to set (120000 = 120 beats per minute)
- Note:
- will only work on MIDP20 device with GLLibConfig.sound_useJSR135=true
it is usually better to use Snd_SetRate instead of altering the tempo of the sound
- Returns:
- true if it was able to set tempo
static void Snd_UnLoadSound | ( | int | index | ) | throws Exception [static, package, inherited] |
Unload a sound resource from memory.
No exception will thrown if the sound is allready unloaded or if the index is invalid.
- Parameters:
-
index Index of sound to unload.
static void Snd_Update | ( | ) | [static, package, inherited] |
Update sound engine status all sound ressource.
This call HAS to be called at every game loop.
- Note:
- If your device is using the Threaded system, this call will do nothing. It is a good idea to keep calling it any way to prevent errors/bugs with unthreaded devices.
Variable Documentation
final int k_snd_priority_highest = 0 [static, package, inherited] |
Sound priorities constant.
final int k_snd_priority_lowest = 15 [static, package, inherited] |
Sound priorities constant.
final int k_snd_priority_normal = 7 [static, package, inherited] |
Sound priorities constant.
String [] s_data_mimeType [static, package, inherited] |
Sound Mime Container, to know the sound type of each slots.
int s_snd_masterVolume [static, package, inherited] |
Sound engine master volume (0-100). The volume will be scaled to the phone range.
int s_snd_maxNbSoundSlot [static, package, inherited] |
Sound maximum slot count for this game. The maximum number of sounds that can be loaded into memory.
Generated on Tue Sep 23 23:05:31 2008 for GLLib by
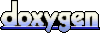