XPlayer core
[XPlayer]
The base class for XPlayer communication with the Gameloft server.
More...Classes | |
class | XPlayer |
Detailed Description
The base class for XPlayer communication with the Gameloft server.
Important:
Make sure the API connects to the correct URL and gameserver!!! Ask interactivity team for the correct urls.
Control the set of features offered by the api using the Configuration class.
The api consists of two parts: XPlayer Highscores and XPlayer Multiplayer.
XPlayer Highscores
Provides services for registering a nickname, logging in, sending highscores, viewing leaderboard(s), rating and recommending games, viewing stats.
The main mechanism for passing requests to the Gameloft server is by using HTTP transactions. Every action that results in contacting the server has a pair of asynchronous functions, a send function and a handle.
The send function builds up the HTTP message with the request and initiates the transaction (sends that message to the server).
The handle manages the connection and any events that might occur, for example a response from the server, a timeout or an error. It sets an error code indicating the status of the current connection which can be viewed by the means of the getLastError()
function provided.
This means that the usual cycle of a request is the following:
- Initiate the transaction by sending the request.
-
While the result from the server is pending and a timeout has not occured, call the handle and check the error code returned with
getLastError()
; - Analysing the error code returned, determine a correct response (if the result is still pending, wait some more, if timedout or some other error display a meaningful message, etc.)
Following is a short example of a generic game rate sending cycle:
Common practice is to use a 4-step request process.
- First we have a SET step which initiates the request.
- Second is a WAIT step for response waiting.
- Third is the PROCESS step which determines the outcome of the request. It can either end successfully or return some error.
- Last is the DISPLAY step in which the results are displayed switch (_online_substate) { case OnlineSubstate.SET: { The user's rate is sent to the server. sendRateGame(rate); We go to the WAIT step of the request. setOnlineSubstate(OnlineSubstate.WAIT); break; }
case OnlineSubstate.WAIT: { ...// Here there should be a test on a keypress event which if true would call the cancel() method of the XPlayer class, canceling any request. This would provide the user with an option to cancel the transaction.
We call the handle handleRateGame(); and get the error code returned by it. _errCode = getLastError();
If the connection is not ongoing (it has finished, either successfully or abnormally), we move on to PROCESS. Otherwise the result is still pending we keep waiting. if (_errCode != XPlayer.Error.ERROR_PENDING) { cleanup(); setOnlineSubstate(OnlineSubstate.PROCESS); } break; }
The request finished somehow so we must determine the way it did. We'll therefore check the error code returned. case OnlineSubstate.PROCESS: { switch (_errCode) { case XPlayer.Error.ERROR_NONE: { ...// No error - do anything that might be needed here and then go to displaying the result. setOnlineSubstate(OnlineSubstate.DISPLAY); break; }
case XPlayer.Error.ERROR_CONNECTION: { ...// A connection error occured - treat the error here, for example inform the user of its appearance and then go to a new state. We should also provide the user the means to retry the request. break; }
default: { ...// Some other error occured. Also, an error message should be displayed and an option to retry provided. break; } } break; }
case OnlineSubstate.DISPLAY: { ...// Display a message about the rating completing successfully, etc. Provide the means to go to the next game state. break; } }
SCORE SENDING FUNCTIONS:
Depending on the game, different score sending functions can be used.
-
If the game has supplemental data attached to each leaderboad, use the supplemental data functions. Posting a score to a leaderboard that has supplemental data will FAIL if any of the supplemental data fields are missing from the message!! ALL supplemental data for a given level MUST be present in the post score message for this level or it will fail. So for leaderboards with supplemental data use the
sendHighscoreWithSupplementalData()
function. -
If the game has multiple leaderboads, all the scores can be sent using a single http request, with or without supplemental data. When multiple scores are to be sent, the procedure is: reset the multiple score buffer, add the scores in the buffer and call send.
- If the game only has one leaderboard, don't send the level parameter! Call this functions with level = -1.
XPlayer Multiplayer
Provides services for creating a multiplayer game, joining a game, listing the available games and playing a multiplayer game.
The communication with the server is performed using TCP streams.
The communication protocol is based on requests, similar to the HTTP requests from the Highscore XPlayer. There are some messages though that arrive to the client without a previous request being made.
The messages exchanged between the server and the client are:
- Connection messages - used to establish and terminate the physical connection.
- Game messages - requests , push messages and in game messages.
The connection messages and the game request messages work in a similar way to the requests in the Highscore XPlayer, with send and handle functions corresponding to each function. These functios are to be used the same way as the HTTP requests from XPlayer Highscore, even though the communication lies on TCP in this case. Look at the example above for sample use.
The push messages are sent by the server to inform the client that something has happened on the server side: some player just joined the current game, some player left, the game has started or somebody finished the game. To make sure the push messages are processed, the game must call mpHandleUpdates()
on every cycle while being in a state where push messages are expected to be received (in session waiting start, in game playing).
The in game messages are sent by the directly by the caller class and they are forwarded by the server to all the other clients that are engaged in a multiplayer game with the current player. The api does not do any processing of those messages. To make sure the in game messages are received and stored in the in game message queue, the game must call mpHandleGameData()
on every cycle while the multiplayer game is in progress.
Here is how it works:
Before performing any multiplayer actions, the player must establish a connection and login on the multiplayer server. This is achieved using the mpSendEstablishConnection()/mpHandleEstablishConnection()
and mpSendLogin()/mpHandleLogin()
functions. According to the design of the game, the connection establish/login operations can be performed when a multiplayer menu is accesses, with disconnection occuring when the menu is left, or they can be performed before any multiplayer game, with disconnections occuring when the game is finished.
After connection and login, the player can choose to go to quick game, create a new game or list the available games. The quick game option returns the first available game on the server if any, create game makes a new game on the multiplayer server and list games returns a list of all the games in the server that can be joined.
If a player creates a game, he is the master of the game and can kick out a player that joined or start the game when enough players have joined. If the master leaves the session, the game is terminated and everybody else in the game is kicked out.
A game can be joined using the name of the game. The name can be chosen from the sessions list.
After enough players ( 2 usually ) are in a game, the master can choose to start the game. The other player will receive the start response as a push message.
After the game is started, the players can send game specific messages to each other.
The game is finished when everybody finishes the game and sends a finish message, or when some players have left and the game cannot go on.
- Author:
- Gameloft Interactivity Team
- Version:
- 1.0.9
- Copyright:
- Gameloft SA � 2005
Generated on Tue Sep 23 23:05:31 2008 for GLLib by
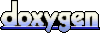