GLLib : File/Package System
[GLLib]
handle data file access (read only)
More...Functions | |
static String | GetMIME (int idx) |
Get the MIME type as a String for a MIME index. | |
static int | GetNBData () |
Get the number of data in current data file. | |
InputStream | GetResourceAsStream (String s) |
static void | Pack_Close () |
Close a pack file. | |
static void | Pack_LoadMIME (String filename) |
Load the MIME type from a MIME Pack. | |
static void | Pack_Open (String filename) |
Open a pack given its path and filename. | |
static int | Pack_PositionAtData (int idx) |
Position the pack pointer to the begining of data idx. | |
static int | Pack_Read () |
Read a single byte from the current stream. | |
static int | Pack_Read16 () |
Read one unsigned short from the current stream. | |
static int | Pack_Read32 () |
Read one int from the current stream. | |
static Object | Pack_ReadArray (int idx) |
Read an array or multiarray from the stream. | |
static byte[] | Pack_ReadData (int idx) |
Read and return the data at idx. | |
static int | Pack_ReadFully (byte[] array, int offset, int length) |
Read into a byte array. | |
static void | Pack_ReleaseBinaryCache (String filename) |
Release cache data of a pack and subpack. | |
static void | Pack_Seek (int addr) |
Set the current offset to addr byte from the beginning. | |
static void | Pack_Skip (int nb) |
Skip ahead in the current stream. | |
Variables | |
static byte[][] | MIME_type |
MIME type array. Stored as UTF-8. | |
static int | s_pack_lastDataReadMimeType |
Mime type of last data that have been read through Pack_Read. |
Detailed Description
handle data file access (read only)Packages are composed of at least one data file (subpack).
The first data file (first subpack) contain extra information about all the data stored within the pack.
Extra information for 1st subpack, at the very beginning of the file:
- 2 byte : Number of data within the pack. How many data are store within this pack (including all subpack)).
- 2 byte : Number of subpacks. How many subpacks compose this pack.
- 2 byte * number of subpack (fat table): List the starting data number of each subpack.
Example with 3 sub pack we could have : 0, 8, 15 :- Subpack0 contain data(0) to data(7).
- Subpack1 contain data(8), data(14).
- Subpack2 contain data(15) to data(nb of data).
Then each subpack (including the first one) contain:
- 4 byte * number of data in this subpack + 1 : offset table. This offset is the offset of each data from the begining of this file to the data. The +1 is for an extra value which represent the end of the file, this is used to calulate the size of each data within the subpack (size of data) = (offset of next data) - (offset of the data).
Finaly, for each data inside the subpack:
- 1 byte : mime type of the data except if file is a dummy file (eg size = 0)
- x byte : the data
There is also one "special" pack, which contain the MIME type if some were defined:
- 1 byte : Mime type count.
- For each mime type:
- 1 byte : length of mime type byte array.
- length of mime type byte : byte array encoded as UTF-8 representing the mime type.
Function Documentation
static String GetMIME | ( | int | idx | ) | [static, package, inherited] |
Get the MIME type as a String for a MIME index.
- Parameters:
-
idx Idex to get MIME type.
- Returns:
- String containing the requested MIME type or empty string.
static int GetNBData | ( | ) | [static, package, inherited] |
Get the number of data in current data file.
- Returns:
- the number of data
InputStream GetResourceAsStream | ( | String | s | ) | [inherited] |
static void Pack_Close | ( | ) | [static, package, inherited] |
Close a pack file.
Nothing will be done if there are no pack currently open.
- Note:
- This is the only way to free the memory comsumed by a pack loaded in memory. GLLib.Gc() will be called by this function.
static void Pack_LoadMIME | ( | String | filename | ) | [static, package, inherited] |
Load the MIME type from a MIME Pack.
- Parameters:
-
filename Filename of the pack containing the MIME type.
static void Pack_Open | ( | String | filename | ) | [static, package, inherited] |
Open a pack given its path and filename.
Example: Pack_Open("/0");
- Parameters:
-
filename Filename of the package to open.
- Note:
- This load the whole pack in memory watch for phone constraint.
static int Pack_PositionAtData | ( | int | idx | ) | [static, package, inherited] |
Position the pack pointer to the begining of data idx.
If the data requested is not in this subpack, the correct subpack will be opened. This can be usefull if you want to read/stream/parse the pack-stream by yourself.
- Returns:
- Length of the requested data.
- Note:
- s_pack_lastDataReadMimeType is now valid for the requested data.
static int Pack_Read | ( | ) | [static, package, inherited] |
Read a single byte from the current stream.
- Returns:
- one byte as a int, or -1 if EOF.
- Exceptions:
-
Exception if error occured.
static int Pack_Read16 | ( | ) | [static, package, inherited] |
Read one unsigned short from the current stream.
LittleEndian Format.
- Returns:
- Return unsigned short value as an int.
- Exceptions:
-
Exception if error occured.
static int Pack_Read32 | ( | ) | [static, package, inherited] |
Read one int from the current stream.
LittleEndian Format.
- Returns:
- Return int value.
- Exceptions:
-
Exception if error occured.
static Object Pack_ReadArray | ( | int | idx | ) | [static, package, inherited] |
Read an array or multiarray from the stream.
ID is encoded as:
- bit 0-1 = type of data array (ARRAY_BYTE, ARRAY_SHORT, ARRAY_INT).
- bit 2 = 1 if multidimension array, 0 otherwise.
- bit 3 = encoding for nb of component in array (0=byte, 1 = short).
- bit 4-5 = if multidimensional array . dimension of array else real padding of data inside array.
- bit 6-7 = unused.
- Parameters:
-
idx Index of the array/multiarray to read.
- Returns:
- Array or multiarray as an Object.
- Exceptions:
-
Exception if error occured.
static byte [] Pack_ReadData | ( | int | idx | ) | [static, package, inherited] |
Read and return the data at idx.
If needed, the system, will seek to the data, or even open another subpack.
- Parameters:
-
idx Index of the data to read in this package.
- Returns:
- A byte array containing the data requested.
static int Pack_ReadFully | ( | byte[] | array, | |
int | offset, | |||
int | length | |||
) | [static, package, inherited] |
Read into a byte array.
- Parameters:
-
array Array to store data read, array must be non null. offset Offset to store byte in array. length Number of byte to read.
- Returns:
- Number of byte read, must be length or exception.
static void Pack_ReleaseBinaryCache | ( | String | filename | ) | [static, package, inherited] |
Release cache data of a pack and subpack.
- Parameters:
-
filename Filename of the package to release.
static void Pack_Seek | ( | int | addr | ) | [static, package, inherited] |
Set the current offset to addr byte from the beginning.
- Parameters:
-
addr Offset to seek to from the begining of the current stream.
static void Pack_Skip | ( | int | nb | ) | [static, package, inherited] |
Skip ahead in the current stream.
Move forward the current offset in the stream.
- Parameters:
-
nb Number of byte to skip in data file.
- Exceptions:
-
Exception if error occured.
Variable Documentation
byte [][] MIME_type [static, package, inherited] |
MIME type array. Stored as UTF-8.
int s_pack_lastDataReadMimeType [static, package, inherited] |
Mime type of last data that have been read through Pack_Read.
Generated on Tue Sep 23 23:05:30 2008 for GLLib by
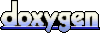