GLLib Class Reference
[GLLib]
The GLLib class is the main class to do a game creation at Gameloft.
More...
Inherits Canvas, and Runnable.
void | keyPressed (int keyCode) |
Key pressed method. | |
void | keyReleased (int keyCode) |
Key released. | |
static void | Game_KeyClearKeyCode () |
clear all the key code . no key input will be valid until you define new key association using Game_keySetKeyCode | |
static void | Game_KeySetKeyCode (boolean gameAction, int keyCode, int key) |
set the keycode for a specific key | |
static int | IsAnyKeyDown () |
Is there any key that is being held. | |
static boolean | IsKeyDown (int keyFlag) |
Query a key to know if its down/pressed. | |
static boolean | IsKeyUp (int keyFlag) |
Query a key to know if its up/unpressed. | |
static void | ResetAKey (int keyFlag) |
Reset one key to unpressed state. | |
static void | ResetKey () |
Reset all keys to unpressed. | |
static int | WasAnyKeyPressed () |
Was there any key just pressed. | |
static int | WasAnyKeyReleased () |
Was there any key just released. | |
static boolean | WasKeyPressed (int keyFlag) |
Was there a key just pressed. | |
static boolean | WasKeyReleased (int keyFlag) |
Was there a key just released. | |
Public Member Functions | |
void | Game_Run () throws Exception |
Function to be implemented in every game. | |
InputStream | GetResourceAsStream (String s) |
GLLib (Object application, Object display) | |
Constructor. | |
void | hideNotify () |
Override of Canvas.hideNotify(). | |
void | paint (Graphics _g) |
Standard rendering function. | |
void | run () |
Game engine main loop/thread. | |
void | showNotify () |
Override of Canvas.showNotify(). | |
void | sizeChanged (int w, int h) |
Called when the drawable area has been changed. | |
Static Public Member Functions | |
static final void | drawPartialRGB (Graphics g, int screenWidth, int screenHeight, int rgbData[], int scanlength, int srcX, int srcY, int x, int y, int width, int height, boolean processAlpha) |
Same as drawPartialRGB but it's useful when you draw in a back buffer that is bigger than the screen. | |
static final void | drawPartialRGB (Graphics g, int rgbData[], int scanlength, int srcX, int srcY, int x, int y, int width, int height, boolean processAlpha) |
Same as drawRGB but it draws safely (without drawing outside the screen). | |
static void | LZMA_Inflate (byte[] compressDat) throws Exception |
static void | Vibrate (int duration) |
Make the phone vibrate or flash the back light is the phone has this functionnality. | |
Static Public Attributes | |
static javax.microedition.lcdui.Graphics | g = null |
Graphics context where all rendering operation will happen. | |
Protected Member Functions | |
Image | GetSoftwareDoubleBuffer () |
Graphics | GetSoftwareDoubleBufferGraphics () |
void | Init () |
Initialize and start the game engine. | |
void | Pause () |
Pause the game engine. | |
void | Quit () |
Request to quit the game engine. | |
void | Resume () |
Resume the game engine. | |
void | SetupDisplay () |
Setup the display. | |
void | UnInit () |
Quit game engine, free all memory and object. | |
Static Protected Member Functions | |
static void | SetupDefaultKey () |
Setup the default key association for this device. | |
Package Functions | |
void | CheckAndDumpConfig () |
abstract void | Game_update () throws Exception |
Function to be implemented in every game. | |
String | Text_GetLanguageAsString (int languageCode) |
get language code as a string | |
int | Text_GetPhoneDefaultLangage () |
get phone default langage, if unable to get it, return GLLang.EN (considered as default language) | |
Static Package Functions | |
[static initializer] | |
static void | Assert (boolean test, String errMessage) |
Assertion test. | |
static final void | ClipRect (int x, int y, int width, int height) |
Intersects the current clip with the specified rectangle. | |
static String | ConvertFixedPointToString (int value, int precision) |
Converts a GLLib Fixed Point number to a string in base 10. | |
static final void | CopyArea (int x_src, int y_src, int width, int height, int x_dest, int y_dest, int anchor) |
Copies the contents of a rectangular area (x_src, y_src, width, height) to a destination area, whose anchor point identified by anchor is located at (x_dest, y_dest). | |
static void | Dbg (String log) |
Log a debug string on the console. | |
static final void | DrawArc (int x, int y, int width, int height, int startAngle, int arcAngle) |
Draws the outline of a circular or elliptical arc covering the specified rectangle, using the current color and stroke style. | |
static final void | DrawChar (char character, int x, int y, int anchor) |
Draws the specified character using the current font and color. | |
static final void | DrawChars (char[] data, int offset, int length, int x, int y, int anchor) |
Draws the specified characters using the current font and color. | |
static final void | DrawImage (javax.microedition.lcdui.Image img, int x, int y, int anchor) |
Draws the specified image by using the anchor point. | |
static final void | DrawLine (int x1, int y1, int x2, int y2) |
Draws a line between the coordinates (x1,y1) and (x2,y2) using the current color and stroke style. | |
static final void | DrawRect (int x, int y, int width, int height) |
Draws the outline of the specified rectangle using the current color and stroke style. | |
static final void | DrawRegion (javax.microedition.lcdui.Image src, int x_src, int y_src, int width, int height, int transform, int x_dest, int y_dest, int anchor) |
Copies a region of the specified source image to a location within the destination, possibly transforming (rotating and reflecting) the image data using the chosen transform function. | |
static final void | DrawRGB (int[] rgbData, int offset, int scanlength, int x, int y, int width, int height, boolean processAlpha) |
Renders a series of device-independent RGB+transparency values in a specified region. | |
static final void | DrawRoundRect (int x, int y, int width, int height, int arcWidth, int arcHeight) |
Draws the outline of the specified rounded corner rectangle using the current color and stroke style. | |
static final void | DrawString (String str, int x, int y, int anchor) |
Draws the specified String using the current font and color. | |
static final void | DrawSubstring (String str, int offset, int len, int x, int y, int anchor) |
Draws the specified String using the current font and color. | |
static final void | FillArc (int x, int y, int width, int height, int startAngle, int arcAngle) |
Fills a circular or elliptical arc covering the specified rectangle. | |
static final void | FillRect (int x, int y, int width, int height) |
Fills the specified rectangle with the current color. | |
static final void | FillRoundRect (int x, int y, int width, int height, int arcWidth, int arcHeight) |
Fills the specified rounded corner rectangle with the current color. | |
static final void | FillTriangle (int x1, int y1, int x2, int y2, int x3, int y3) |
Fills the specified triangle will the current color. | |
static final void | Gc () |
call the garbage collector to free memory | |
static final int | GetBlueComponent () |
Gets the blue component of the current color. | |
static final int | GetClipHeight () |
Gets the height of the current clipping area. | |
static final int | GetClipWidth () |
Gets the width of the current clipping area. | |
static final int | GetClipX () |
Gets the X offset of the current clipping area, relative to the coordinate system origin of this graphics context. | |
static final int | GetClipY () |
Gets the Y offset of the current clipping area, relative to the coordinate system origin of this graphics context. | |
static final int | GetColor () |
Gets the current color. | |
static final int | GetDisplayColor (int color) |
Gets the color that will be displayed if the specified color is requested. | |
static final javax.microedition.lcdui.Font | GetFont () |
Gets the current font. | |
static final long | GetFrameTime () |
get time for this frame (real time when this frame started) | |
static final int | GetGrayScale () |
Gets the current grayscale value of the color being used for rendering operations. | |
static final int | GetGreenComponent () |
Gets the green component of the current color. | |
static String | GetMIME (int idx) |
Get the MIME type as a String for a MIME index. | |
static int | GetNBData () |
Get the number of data in current data file. | |
static final long | GetRealTime () |
get real time at the moment the fucntion is called | |
static final int | GetRedComponent () |
Gets the red component of the current color. | |
static final int | GetScreenHeight () |
get screen height, (if screen orientation changes, it will return the new screen height automatically | |
static final int | GetScreenWidth () |
get screen width, (if screen orientation changes, it will return the new screen width automatically | |
static final int | GetStrokeStyle () |
Gets the stroke style used for drawing operations. | |
static final int | GetTranslateX () |
Gets the X coordinate of the translated origin of this graphics context. | |
static final int | GetTranslateY () |
Gets the Y coordinate of the translated origin of this graphics context. | |
static void | InitSharedRms (String strVendor, String strMidletName) |
Sets vendor name and midlet names - used for shared rms read/write. | |
static final long | Math_Abs (long a) |
static final int | Math_Abs (int a) |
static int | Math_Atan (int dx, int dy) |
Arctangent. | |
static int | Math_AtanSlow (int dx, int dy) |
Arctangent, very slow but accurate method, it find angle by dichotomy. | |
static void | Math_Bezier2D (int x1, int y1, int x2, int y2, int x3, int y3, int interp) |
Three control point 2D Bezier interpolation. | |
static void | Math_Bezier3D (int x1, int y1, int z1, int x2, int y2, int z2, int x3, int y3, int z3, int interp) |
Three control point 3D Bezier interpolation. | |
static int | Math_Cos (int angle) |
Cosinus. | |
static int | Math_DegreeToFixedPointAngle (int a) |
Convert a degree angle into a fixed point angle. | |
static int | Math_Det (int x1, int y1, int x2, int y2) |
Compute the determinant of 2 2Dvector. | |
static int | Math_DistPointLine (int x0, int y0, int x1, int y1, int x2, int y2) throws Exception |
Compute the distance from a point to a segment/line. | |
static int | Math_Div10 (int a) |
Divide by 10 a number, with result in fixed point. | |
static int | Math_DotProduct (int x1, int y1, int x2, int y2) |
Compute the dot product of 2 2Dvector. | |
static int | Math_FixedPoint_Add (int summand1, int summand2) |
Addition for Fixed Point. | |
static int | Math_FixedPoint_DegreesToAngleFixedPoint (int angle) |
Convert an angle from degrees to fixed point angle. | |
static int | Math_FixedPoint_DegreesToRadians (int angle) |
Convert an angle from degrees to radians (both fixed point and not, since this is just a ratio). | |
static int | Math_FixedPoint_Det (int x1, int y1, int x2, int y2) |
Compute the determinant of 2 2Dvector in Fixed Point. | |
static int | Math_FixedPoint_Divide (int dividend, int divisor) |
Division for Fixed Point. | |
static int | Math_FixedPoint_DotProduct (int x1, int y1, int x2, int y2) |
Compute the dot product of 2 2Dvector in Fixed Point. | |
static int | Math_FixedPoint_LineCircleIntersect (int in_x1, int in_y1, int in_x2, int in_y2, int in_circleX, int in_circleY, int in_radius) |
Find intersect points between a line and a circle. | |
static int | Math_FixedPoint_LineRectangleIntersect (int in_x1, int in_y1, int in_x2, int in_y2, int in_rectX, int in_rectY, int in_rectW, int in_rectH) |
Find intersect points between a line and a rectangle. | |
static int | Math_FixedPoint_Multiply (int multiplicand, int multiplier) |
Multiplication for Fixed Point. | |
static int | Math_FixedPoint_Norm (int x, int y) |
Nnormal of a vector in Fixed Point. | |
static int | Math_FixedPoint_NormPow (int x, int y) |
Compute pow of the normal of a vector in Fixed Point. | |
static int | Math_FixedPoint_PointLineDistance (int in_lineX1, int in_lineY1, int in_lineX2, int in_lineY2, int in_pointX, int in_pointY) |
Compute the distance from a point to a segment/line. | |
static int | Math_FixedPoint_RadiansToAngleFixedPoint (int angle) |
Convert an angle from radians to fixed point angle. | |
static int | Math_FixedPoint_RadiansToDegrees (int angle) |
Convert an angle from radians to degrees (both fixed point and not, since this is just a ratio). | |
static int | Math_FixedPoint_Round (int value) |
Rounds value to closest whole number in Fixed Point. | |
static int | Math_FixedPoint_Sqrt (long value) |
Square Root for Long Fixed Point values. | |
static int | Math_FixedPoint_Sqrt (int value) |
Square Root for Integer Fixed Point values. | |
static int | Math_FixedPoint_Square (int value) |
Square for Fixed Point. | |
static int | Math_FixedPoint_Subtract (int minuend, int subtrahend) |
Subtraction for Fixed Point. | |
static int | Math_FixedPointAdjust (int a) |
Adjust a fixed point in base 8 to this fixed point base. | |
static int | Math_FixedPointAngleToDegree (int a) |
Convert a fixed point angle into a degree angle. | |
static int | Math_FixedPointToInt (int a) |
Convert a fixed point value to an int value. | |
static void | Math_Init (String packName, int cos, int sqrt) throws Exception |
Math initialisation, by reading specified table from a package. | |
static int | Math_IntToFixedPoint (int a) |
Convert a fixed point value to an int value. | |
static int | Math_Log2 (int a) |
return log base 2 | |
static final long | Math_Max (long a, long b) |
static final int | Math_Max (int a, int b) |
static final long | Math_Min (long a, long b) |
static final int | Math_Min (int a, int b) |
static int | Math_Norm (int x, int y, int iter) |
Norm following newton law approximation. | |
static int | Math_NormPow (int x1, int y1) |
Compute pow of the normal of a vector. | |
static void | Math_QuickSort (int array[]) |
Quicksort an array of integer. | |
static int[] | Math_QuickSortIndices (int data[], int nbItemPerValue, int itemNb) |
Get an array of indice corresponding to the sorting of an array of data. | |
static int[] | Math_QuickSortIndices (int data[]) |
Get an array of indice corresponding to the sorting of an array of data. | |
static void | Math_Quit () |
Free all math arrays. | |
static final int | Math_Rand () |
Create a random number. | |
static int | Math_Rand (int a, int b) |
Create a random int inside the interval [a, b[. | |
static final void | Math_RandSetSeed (long seed) |
set math random seed | |
static boolean | Math_RectIntersect (int Ax0, int Ay0, int Ax1, int Ay1, int Bx0, int By0, int Bx1, int By1) |
Tell if 2 axis aligned rectangle intersect. | |
static boolean | Math_SameSign (int a, int b) |
Test 2 numbers to see if they are both of the same sign. | |
static int | Math_SegmentIntersect (int x1, int y1, int x2, int y2, int x3, int y3, int x4, int y4) |
Tell if 2 segment intersect themselves. | |
static int | Math_Sin (int a) |
Sinus. | |
static int | Math_Sqrt (long val) |
Square Root slow. | |
static int | Math_Sqrt (int x) |
Square Root. | |
static int | Math_Sqrt_FixedPoint (int val, int precisionLoop) |
Square Root for Fixed Point. | |
static int | Math_Tan (int angle) |
Tangent. | |
static void | Mem_ArrayCopy (Object src, int src_position, Object dst, int dst_position, int length) throws Exception |
Copies an array from the specified source array, beginning at the specified position, to the specified position of the destination array. | |
static int | Mem_GetArray (byte[] src, int src_off, byte[] dst) throws Exception |
fill destination array with content of src array at specified offset | |
static byte | Mem_GetByte (byte[] src, int src_off) |
get a byte value from the specified array at specified offset | |
static int | Mem_GetInt (byte[] src, int src_off) |
get an int value from the specified array at specified offset | |
static long | Mem_GetLong (byte[] src, int src_off) |
get a long value from the specified array at specified offset | |
static short | Mem_GetShort (byte[] src, int src_off) |
get a short value from the specified array at specified offset | |
static Object | Mem_ReadArray (InputStream is) |
Read an array/multiarray, from an inputStream. | |
static int | Mem_SetArray (byte[] dst, int dst_off, byte[] src) throws Exception |
copies whole content of src array in dst array at specified offset | |
static int | Mem_SetByte (byte[] dst, int dst_off, byte src) |
set a byte value in a byte array at a given offset | |
static int | Mem_SetInt (byte[] dst, int dst_off, int src) |
set a int value in a byte array at a given offset | |
static int | Mem_SetLong (byte[] dst, int dst_off, long src) |
set a long value in a byte array at a given offset | |
static int | Mem_SetShort (byte[] dst, int dst_off, short src) |
set a short value in a byte array at a given offset | |
static void | Pack_Close () |
Close a pack file. | |
static void | Pack_CloseShared () throws Exception |
Closes current pack, previously opened with Pack_OpenShared. | |
static void | Pack_LoadMIME (String filename) |
Load the MIME type from a MIME Pack. | |
static void | Pack_Open (String filename) |
Open a pack given its path and filename. | |
static void | Pack_OpenShared (String strName, String strVendor, String strMidletName) throws Exception |
Opens a shared rms pack. | |
static int | Pack_PositionAtData (int idx) |
Position the pack pointer to the begining of data idx. | |
static int | Pack_Read () |
Read a single byte from the current stream. | |
static int | Pack_Read16 () |
Read one unsigned short from the current stream. | |
static int | Pack_Read32 () |
Read one int from the current stream. | |
static Object | Pack_ReadArray (int idx) |
Read an array or multiarray from the stream. | |
static byte[] | Pack_ReadData (int idx) |
Read and return the data at idx. | |
static int | Pack_ReadFully (byte[] array, int offset, int length) |
Read into a byte array. | |
static void | Pack_ReleaseBinaryCache (String filename) |
Release cache data of a pack and subpack. | |
static void | Pack_Seek (int addr) |
Set the current offset to addr byte from the beginning. | |
static void | Pack_Skip (int nb) |
Skip ahead in the current stream. | |
static final boolean | PlatformRequest (String url) |
Performs platformRequest. | |
static void | Print (String log) |
print a string in out stream, does not append character return at the end of line (as opposed to Dbg) | |
static void | Profiler_BeginNamedEvent (String name) |
Mark the beginning of an event. | |
static void | Profiler_Draw () |
Draw profiler events to screen. | |
static void | Profiler_End () |
End capturing events. | |
static void | Profiler_EndNamedEvent () |
Mark the end of an event. | |
static void | Profiler_Start () |
Start capturing events. | |
static byte[] | Rms_Read (String strName) |
Reads data from the recordstore. | |
static byte[] | Rms_ReadShared (String strName, String strVendor, String strMidletName) |
Read data from shared recordstore. | |
static void | Rms_Write (String strName, byte[] data) |
Writes data to the recordstore. | |
static void | Rms_WriteShared (String strName, String strVendor, String strMidletName, byte[] data) |
Write data to shared recordstore. | |
static void | SavePack (String packName, String rmsName) |
Reads a resource, and writes it into the the recordstore - used for DEBUG purposes. | |
static final void | SetClip (int x, int y, int width, int height) |
Sets the current clip to the rectangle specified by the given coordinates. | |
static final void | setColor (int red, int green, int blue) |
Sets the current color to the specified RGB values. | |
static final void | SetColor (int RGB) |
Sets the current color to the specified RGB values. | |
static final void | SetCurrentGraphics (javax.microedition.lcdui.Image img) |
set current graphics context -. | |
static final void | SetCurrentGraphics (javax.microedition.lcdui.Graphics graphics) |
set current graphics context -. | |
static final void | SetFont (javax.microedition.lcdui.Font font) |
Sets the font for all subsequent text rendering operations. | |
static final void | SetGrayScale (int value) |
Sets the current grayscale to be used for all subsequent rendering operations. | |
static final void | SetStrokeStyle (int style) |
Sets the stroke style used for drawing lines, arcs, rectangles, and rounded rectangles. | |
static int | Stream_Read (InputStream is) throws Exception |
static int | Stream_Read16 (InputStream is) throws Exception |
static int | Stream_Read32 (InputStream is) throws Exception |
static int | Stream_ReadFully (InputStream is, byte[] array, int offset, int length) |
static void | Text_BuildStringCache () |
cache all the text into string Array | |
static void | Text_FreeAll () |
free all the data-array used by the text | |
static String | Text_FromUTF8 (byte[] src, int offset, int len) |
get a string from an UTF-8 encoded byte array. | |
static final int | Text_GetNbString () |
get nb of string in current text pack | |
static String | Text_GetString (int index) |
get a string given it's index | |
static void | Text_LoadTextFromPack (String filename, int index1, int index2) |
open and load text from two text packages inside a pack file | |
static void | Text_LoadTextFromPack (String filename, int index) |
static final void | Text_SetEncoding (String encoding) |
set current text encoding | |
static final void | Translate (int x, int y) |
Translates the origin of the graphics context to the point (x, y) in the current coordinate system. | |
static void | Warning (String message) |
display a warning | |
Static Package Attributes | |
static final int | Align = SpecPos + kNumFullDistances - kEndPosModelIndex |
static final int | BOTTOM = 32 |
Constant for positioning the anchor point of text and images below the text or image Constant for positioning the anchor point of text and images below the text or image. | |
static final int | DOTTED = 1 |
Constant for the DOTTED stroke style. | |
static final int | HCENTER = 1 |
Constant for centering text and images horizontally around the anchor point. | |
static int | inputIndex |
static final int | IsMatch = 0 |
static final int | IsRep = IsMatch + (kNumStates << kNumPosBitsMax) |
static final int | IsRep0Long = IsRepG2 + kNumStates |
static final int | IsRepG0 = IsRep + kNumStates |
static final int | IsRepG1 = IsRepG0 + kNumStates |
static final int | IsRepG2 = IsRepG1 + kNumStates |
static final int | kAlignTableSize = (1 << kNumAlignBits) |
static final int | kBitModelTotal = (1 << kNumBitModelTotalBits) |
static final int | kEndPosModelIndex = 14 |
static final int | kLenNumHighBits = 8 |
static final int | kLenNumHighSymbols = (1 << kLenNumHighBits) |
static final int | kLenNumLowBits = 3 |
static final int | kLenNumLowSymbols = (1 << kLenNumLowBits) |
static final int | kLenNumMidBits = 3 |
static final int | kLenNumMidSymbols = (1 << kLenNumMidBits) |
static final int | kMatchMinLen = 2 |
static final int | kNumAlignBits = 4 |
static final int | kNumBitModelTotalBits = 11 |
static final int | kNumFullDistances = (1 << (kEndPosModelIndex >> 1)) |
static final int | kNumLenProbs = LenHigh + kLenNumHighSymbols |
static final int | kNumLenToPosStates = 4 |
static final int | kNumMoveBits = 5 |
static final int | kNumPosBitsMax = 4 |
static final int | kNumPosSlotBits = 6 |
static final int | kNumPosStatesMax = (1 << kNumPosBitsMax) |
static final int | kNumStates = 12 |
static final int | kNumTopBits = 24 |
static final int | kStartPosModelIndex = 4 |
static final int | kTopValue = (1 << kNumTopBits) |
static final int | LEFT = 4 |
Constant for positioning the anchor point of text and images to the left of the text or image. | |
static final int | LenChoice = 0 |
static final int | LenChoice2 = LenChoice + 1 |
static final int | LenCoder = Align + kAlignTableSize |
static final int | LenHigh = LenMid + (kNumPosStatesMax << kLenNumMidBits) |
static final int | LenLow = LenChoice2 + 1 |
static final int | LenMid = LenLow + (kNumPosStatesMax << kLenNumLowBits) |
static final int | Literal = RepLenCoder + kNumLenProbs |
static final int | LZMA_BASE_SIZE = 1846 |
static final int | LZMA_LIT_SIZE = 768 |
static final int | LZMA_RESULT_DATA_ERROR = 1 |
static final int | LZMA_RESULT_NOT_ENOUGH_MEM = 2 |
static final int | LZMA_RESULT_OK = 0 |
static byte[] | m_Buffer |
static long | m_Code |
static int | m_current_keys_pressed |
Current keys pressed. | |
static int | m_current_keys_released |
Current keys released. | |
static int | m_current_keys_state |
Current keys state. | |
static int | m_ExtraBytes |
static int | m_inSize |
static int | m_keys_pressed |
Previous frame keys pressed. | |
static int | m_keys_released |
Previous frame keys released. | |
static int | m_keys_state |
Previous frame keys state. | |
static int | m_last_key_pressed = -9999 |
Previous key pressed. | |
static byte[] | m_outStream |
static long | m_Range |
static final int | Math_Angle180 = Math_DegreeToFixedPointAngle(180) |
180 in fixed point | |
static final int | Math_Angle270 = Math_DegreeToFixedPointAngle(270) |
270 in fixed point | |
static final int | Math_Angle360 = Math_DegreeToFixedPointAngle(360) |
360 in fixed point | |
static final int | Math_Angle90 = Math_DegreeToFixedPointAngle(90) |
90 in fixed point | |
static final int | Math_AngleMUL = 1 << GLLibConfig.math_angleFixedPointBase |
angle fixed point multiplier eg one degree in fixed point base.(not equal to Math_DegreeToFixedPointAngle(1)) | |
static final int | Math_FixedPoint_E = 1459366444 >> (29 - GLLibConfig.math_fixedPointBase) |
static final int | Math_FixedPoint_PI = 1686629713 >> (29 - GLLibConfig.math_fixedPointBase) |
static final int | MATH_INTERSECT_NO_INTERSECT = 0 |
static final int | MATH_INTERSECT_ONE_POINT = (1 << 1) |
static final int | MATH_INTERSECT_TWO_POINTS = (1 << 2) |
static final int | MATH_SEGMENTINTERSECT_COLLINEAR = -1 |
return value of Math_segmentIntersect if there is no or full intersection because both segment are colinear | |
static final int | MATH_SEGMENTINTERSECT_DO_INTERSECT = 1 |
return value of Math_segmentIntersect if there is an intersection | |
static final int | MATH_SEGMENTINTERSECT_DONT_INTERSECT = 0 |
return value of Math_segmentIntersect if there is no intersection | |
static byte[][] | MIME_type |
MIME type array. Stored as UTF-8. | |
static final int | PosSlot = IsRep0Long + (kNumStates << kNumPosBitsMax) |
static final int | PROFILER_MAX_EVENTS = 200 |
Maximum number of events. | |
static final int | RepLenCoder = LenCoder + kNumLenProbs |
static final int | RIGHT = 8 |
Constant for positioning the anchor point of text and images to the right of the text or image. | |
static javax.microedition.midlet.MIDlet | s_application |
Reference to the application. Usually its a reference to a midlet (or IApplication for doja). | |
static Display | s_display |
reference to the display | |
static int | s_game_currentFrameNB |
Current frame number. Increased every frame. | |
static int | s_game_FPSAverage |
Average fps * 100. | |
static int | s_game_frameDT |
Delta time between the previous frame and this one. | |
static boolean | s_game_interruptNotify |
Interrupt notifier. Set to true when an interrupt occured. | |
static boolean | s_game_isPaused |
Pause state of the game. True if the game is paused. | |
static int | s_game_keyEventIndex |
Key indice of the key which had an event. -1 if no event. | |
static int | s_game_keyJustPressed |
Key indice of the key that was just pressed. -1 if no key was just pressed. | |
static long | s_game_keyPressedTime |
Time of the last key press. | |
static long | s_game_lastFrameTime |
Similar to s_game_timeWhenFrameStart but internally used for GLLibConfig.useFakeInterruptHandling. | |
static int | s_game_state |
Current game state. | |
static long | s_game_timeWhenFrameStart |
Current time when the frame started. | |
static int | s_game_totalExecutionTime |
Total game execution time. | |
static GLLib | s_gllib_instance |
The only instance of this GLLib class. | |
static int | s_keyLastKeyPressUntranslatedCode = -9999 |
Untranslated value of the last keycode. Used before passing through Game_TranslateKeyCode. | |
static int | s_keyLastKeyStates |
Last key state obtained through getKeyStates(). | |
static byte | s_keyState [] |
Current key states bufferised. | |
static byte | s_keyStateRT [] |
RealTime key buffer. User should use s_keyState instead. | |
static int | s_math_bezierX |
x coordinate of bezier interpolated value through Math_Bezier2D or Math_Bezier3D | |
static int | s_math_bezierY |
y coordinate of bezier interpolated value through Math_Bezier2D or Math_Bezier3D | |
static int | s_math_bezierZ |
z coordinate of bezier interpolated value through Math_Bezier3D | |
static int | s_Math_distPointLineX |
x coordinate of orthogonal projection of point on a segment using Math_distPointLine | |
static int | s_Math_distPointLineY |
y coordinate of orthogonal projection of point on a segment using Math_distPointLine | |
static final int | s_math_F_05 = (s_math_F_1>>1) |
Fixed point value for number 0.5. | |
static final int | s_math_F_1 = 1 << GLLibConfig.math_fixedPointBase |
Fixed point value for number 1. | |
static int | s_Math_intersectPoints [][] = new int[2][2] |
static int | s_Math_intersectX |
x coordinate of intersection point of 2 segment using Math_segmentIntersect | |
static int | s_Math_intersectY |
y coordinate of intersection point of 2 segment using Math_segmentIntersect | |
static java.util.Random | s_math_random |
Random number generator. | |
static int | s_pack_lastDataReadMimeType |
Mime type of last data that have been read through Pack_Read. | |
static final int | SOLID = 0 |
Constant for the SOLID stroke style. | |
static final int | SpecPos = PosSlot + (kNumLenToPosStates << kNumPosSlotBits) |
static String | text_encoding = "UTF-8" |
encoding for text (UTF-8 by default) | |
static int | text_nbString |
number of string in this text pack | |
static final int | tkNumMoveBits = 5 |
static final int | TOP = 16 |
Constant for positioning the anchor point of text and images above the text or image. | |
static final int | TRANS_MIRROR = 2 |
causes the specified image region to be reflected about its vertical center. | |
static final int | TRANS_MIRROR_ROT180 = 1 |
causes the specified image region to be reflected about its vertical center and then rotated clockwise by 180 degrees. | |
static final int | TRANS_MIRROR_ROT270 = 4 |
causes the specified image region to be reflected about its vertical center and then rotated clockwise by 270 degrees. | |
static final int | TRANS_MIRROR_ROT90 = 7 |
causes the specified image region to be reflected about its vertical center and then rotated clockwise by 90 degrees. | |
static final int | TRANS_NONE = 0 |
No transform is applied. | |
static final int | TRANS_ROT180 = 3 |
causes the specified image region to be rotated clockwise by 180 degrees. | |
static final int | TRANS_ROT270 = 6 |
causes the specified image region to be rotated clockwise by 270 degrees. | |
static final int | TRANS_ROT90 = 5 |
causes the specified image region to be rotated clockwise by 90 degrees. | |
static final int | VCENTER = 2 |
Constant for centering text and images images vertically around the anchor point. |
Detailed Description
The GLLib class is the main class to do a game creation at Gameloft.The GLLib main function is to be the hearth of your game, it impletements Runnable by starting a Thread. The GLLib provides the developer with methods to handle game key events by doing the mapping to your device. Package management for your resources is also provided by the GLLib class. You have acces also to basic math support.
Member Function Documentation
static void LZMA_Inflate | ( | byte[] | compressDat | ) | throws Exception [static] |
Member Data Documentation
final int Align = SpecPos + kNumFullDistances - kEndPosModelIndex [static, package] |
int inputIndex [static, package] |
final int IsMatch = 0 [static, package] |
final int IsRep = IsMatch + (kNumStates << kNumPosBitsMax) [static, package] |
final int IsRep0Long = IsRepG2 + kNumStates [static, package] |
final int IsRepG0 = IsRep + kNumStates [static, package] |
final int IsRepG1 = IsRepG0 + kNumStates [static, package] |
final int IsRepG2 = IsRepG1 + kNumStates [static, package] |
final int kAlignTableSize = (1 << kNumAlignBits) [static, package] |
final int kBitModelTotal = (1 << kNumBitModelTotalBits) [static, package] |
final int kEndPosModelIndex = 14 [static, package] |
final int kLenNumHighBits = 8 [static, package] |
final int kLenNumHighSymbols = (1 << kLenNumHighBits) [static, package] |
final int kLenNumLowBits = 3 [static, package] |
final int kLenNumLowSymbols = (1 << kLenNumLowBits) [static, package] |
final int kLenNumMidBits = 3 [static, package] |
final int kLenNumMidSymbols = (1 << kLenNumMidBits) [static, package] |
final int kMatchMinLen = 2 [static, package] |
final int kNumAlignBits = 4 [static, package] |
final int kNumBitModelTotalBits = 11 [static, package] |
final int kNumFullDistances = (1 << (kEndPosModelIndex >> 1)) [static, package] |
final int kNumLenProbs = LenHigh + kLenNumHighSymbols [static, package] |
final int kNumLenToPosStates = 4 [static, package] |
final int kNumMoveBits = 5 [static, package] |
final int kNumPosBitsMax = 4 [static, package] |
final int kNumPosSlotBits = 6 [static, package] |
final int kNumPosStatesMax = (1 << kNumPosBitsMax) [static, package] |
final int kNumStates = 12 [static, package] |
final int kNumTopBits = 24 [static, package] |
final int kStartPosModelIndex = 4 [static, package] |
final int kTopValue = (1 << kNumTopBits) [static, package] |
final int LenChoice = 0 [static, package] |
final int LenChoice2 = LenChoice + 1 [static, package] |
final int LenCoder = Align + kAlignTableSize [static, package] |
final int LenHigh = LenMid + (kNumPosStatesMax << kLenNumMidBits) [static, package] |
final int LenLow = LenChoice2 + 1 [static, package] |
final int LenMid = LenLow + (kNumPosStatesMax << kLenNumLowBits) [static, package] |
final int Literal = RepLenCoder + kNumLenProbs [static, package] |
final int LZMA_BASE_SIZE = 1846 [static, package] |
final int LZMA_LIT_SIZE = 768 [static, package] |
final int LZMA_RESULT_DATA_ERROR = 1 [static, package] |
final int LZMA_RESULT_NOT_ENOUGH_MEM = 2 [static, package] |
final int LZMA_RESULT_OK = 0 [static, package] |
byte [] m_Buffer [static, package] |
long m_Code [static, package] |
int m_ExtraBytes [static, package] |
int m_inSize [static, package] |
byte [] m_outStream [static, package] |
long m_Range [static, package] |
final int PosSlot = IsRep0Long + (kNumStates << kNumPosBitsMax) [static, package] |
final int RepLenCoder = LenCoder + kNumLenProbs [static, package] |
final int SpecPos = PosSlot + (kNumLenToPosStates << kNumPosSlotBits) [static, package] |
final int tkNumMoveBits = 5 [static, package] |
Generated on Tue Sep 23 23:05:32 2008 for GLLib by
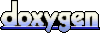