GLLib : Math Utils
[GLLib]
mathematic function
More...Functions | |
static String | ConvertFixedPointToString (int value, int precision) |
Converts a GLLib Fixed Point number to a string in base 10. | |
static final long | Math_Abs (long a) |
static final int | Math_Abs (int a) |
static int | Math_Atan (int dx, int dy) |
Arctangent. | |
static int | Math_AtanSlow (int dx, int dy) |
Arctangent, very slow but accurate method, it find angle by dichotomy. | |
static void | Math_Bezier2D (int x1, int y1, int x2, int y2, int x3, int y3, int interp) |
Three control point 2D Bezier interpolation. | |
static void | Math_Bezier3D (int x1, int y1, int z1, int x2, int y2, int z2, int x3, int y3, int z3, int interp) |
Three control point 3D Bezier interpolation. | |
static int | Math_Cos (int angle) |
Cosinus. | |
static int | Math_DegreeToFixedPointAngle (int a) |
Convert a degree angle into a fixed point angle. | |
static int | Math_Det (int x1, int y1, int x2, int y2) |
Compute the determinant of 2 2Dvector. | |
static int | Math_DistPointLine (int x0, int y0, int x1, int y1, int x2, int y2) throws Exception |
Compute the distance from a point to a segment/line. | |
static int | Math_Div10 (int a) |
Divide by 10 a number, with result in fixed point. | |
static int | Math_DotProduct (int x1, int y1, int x2, int y2) |
Compute the dot product of 2 2Dvector. | |
static int | Math_FixedPoint_Add (int summand1, int summand2) |
Addition for Fixed Point. | |
static int | Math_FixedPoint_DegreesToAngleFixedPoint (int angle) |
Convert an angle from degrees to fixed point angle. | |
static int | Math_FixedPoint_DegreesToRadians (int angle) |
Convert an angle from degrees to radians (both fixed point and not, since this is just a ratio). | |
static int | Math_FixedPoint_Det (int x1, int y1, int x2, int y2) |
Compute the determinant of 2 2Dvector in Fixed Point. | |
static int | Math_FixedPoint_Divide (int dividend, int divisor) |
Division for Fixed Point. | |
static int | Math_FixedPoint_DotProduct (int x1, int y1, int x2, int y2) |
Compute the dot product of 2 2Dvector in Fixed Point. | |
static int | Math_FixedPoint_LineCircleIntersect (int in_x1, int in_y1, int in_x2, int in_y2, int in_circleX, int in_circleY, int in_radius) |
Find intersect points between a line and a circle. | |
static int | Math_FixedPoint_LineRectangleIntersect (int in_x1, int in_y1, int in_x2, int in_y2, int in_rectX, int in_rectY, int in_rectW, int in_rectH) |
Find intersect points between a line and a rectangle. | |
static int | Math_FixedPoint_Multiply (int multiplicand, int multiplier) |
Multiplication for Fixed Point. | |
static int | Math_FixedPoint_Norm (int x, int y) |
Nnormal of a vector in Fixed Point. | |
static int | Math_FixedPoint_NormPow (int x, int y) |
Compute pow of the normal of a vector in Fixed Point. | |
static int | Math_FixedPoint_PointLineDistance (int in_lineX1, int in_lineY1, int in_lineX2, int in_lineY2, int in_pointX, int in_pointY) |
Compute the distance from a point to a segment/line. | |
static int | Math_FixedPoint_RadiansToAngleFixedPoint (int angle) |
Convert an angle from radians to fixed point angle. | |
static int | Math_FixedPoint_RadiansToDegrees (int angle) |
Convert an angle from radians to degrees (both fixed point and not, since this is just a ratio). | |
static int | Math_FixedPoint_Round (int value) |
Rounds value to closest whole number in Fixed Point. | |
static int | Math_FixedPoint_Sqrt (long value) |
Square Root for Long Fixed Point values. | |
static int | Math_FixedPoint_Sqrt (int value) |
Square Root for Integer Fixed Point values. | |
static int | Math_FixedPoint_Square (int value) |
Square for Fixed Point. | |
static int | Math_FixedPoint_Subtract (int minuend, int subtrahend) |
Subtraction for Fixed Point. | |
static int | Math_FixedPointAdjust (int a) |
Adjust a fixed point in base 8 to this fixed point base. | |
static int | Math_FixedPointAngleToDegree (int a) |
Convert a fixed point angle into a degree angle. | |
static int | Math_FixedPointToInt (int a) |
Convert a fixed point value to an int value. | |
static void | Math_Init (String packName, int cos, int sqrt) throws Exception |
Math initialisation, by reading specified table from a package. | |
static int | Math_IntToFixedPoint (int a) |
Convert a fixed point value to an int value. | |
static int | Math_Log2 (int a) |
return log base 2 | |
static final long | Math_Max (long a, long b) |
static final int | Math_Max (int a, int b) |
static final long | Math_Min (long a, long b) |
static final int | Math_Min (int a, int b) |
static int | Math_Norm (int x, int y, int iter) |
Norm following newton law approximation. | |
static int | Math_NormPow (int x1, int y1) |
Compute pow of the normal of a vector. | |
static void | Math_QuickSort (int array[]) |
Quicksort an array of integer. | |
static int[] | Math_QuickSortIndices (int data[], int nbItemPerValue, int itemNb) |
Get an array of indice corresponding to the sorting of an array of data. | |
static int[] | Math_QuickSortIndices (int data[]) |
Get an array of indice corresponding to the sorting of an array of data. | |
static void | Math_Quit () |
Free all math arrays. | |
static final int | Math_Rand () |
Create a random number. | |
static int | Math_Rand (int a, int b) |
Create a random int inside the interval [a, b[. | |
static final void | Math_RandSetSeed (long seed) |
set math random seed | |
static boolean | Math_RectIntersect (int Ax0, int Ay0, int Ax1, int Ay1, int Bx0, int By0, int Bx1, int By1) |
Tell if 2 axis aligned rectangle intersect. | |
static boolean | Math_SameSign (int a, int b) |
Test 2 numbers to see if they are both of the same sign. | |
static int | Math_SegmentIntersect (int x1, int y1, int x2, int y2, int x3, int y3, int x4, int y4) |
Tell if 2 segment intersect themselves. | |
static int | Math_Sin (int a) |
Sinus. | |
static int | Math_Sqrt (long val) |
Square Root slow. | |
static int | Math_Sqrt (int x) |
Square Root. | |
static int | Math_Sqrt_FixedPoint (int val, int precisionLoop) |
Square Root for Fixed Point. | |
static int | Math_Tan (int angle) |
Tangent. | |
Variables | |
static final int | Math_Angle180 = Math_DegreeToFixedPointAngle(180) |
180 in fixed point | |
static final int | Math_Angle270 = Math_DegreeToFixedPointAngle(270) |
270 in fixed point | |
static final int | Math_Angle360 = Math_DegreeToFixedPointAngle(360) |
360 in fixed point | |
static final int | Math_Angle90 = Math_DegreeToFixedPointAngle(90) |
90 in fixed point | |
static final int | Math_AngleMUL = 1 << GLLibConfig.math_angleFixedPointBase |
angle fixed point multiplier eg one degree in fixed point base.(not equal to Math_DegreeToFixedPointAngle(1)) | |
static final int | Math_FixedPoint_E = 1459366444 >> (29 - GLLibConfig.math_fixedPointBase) |
static final int | Math_FixedPoint_PI = 1686629713 >> (29 - GLLibConfig.math_fixedPointBase) |
static final int | MATH_INTERSECT_NO_INTERSECT = 0 |
static final int | MATH_INTERSECT_ONE_POINT = (1 << 1) |
static final int | MATH_INTERSECT_TWO_POINTS = (1 << 2) |
static final int | MATH_SEGMENTINTERSECT_COLLINEAR = -1 |
return value of Math_segmentIntersect if there is no or full intersection because both segment are colinear | |
static final int | MATH_SEGMENTINTERSECT_DO_INTERSECT = 1 |
return value of Math_segmentIntersect if there is an intersection | |
static final int | MATH_SEGMENTINTERSECT_DONT_INTERSECT = 0 |
return value of Math_segmentIntersect if there is no intersection | |
static int | s_math_bezierX |
x coordinate of bezier interpolated value through Math_Bezier2D or Math_Bezier3D | |
static int | s_math_bezierY |
y coordinate of bezier interpolated value through Math_Bezier2D or Math_Bezier3D | |
static int | s_math_bezierZ |
z coordinate of bezier interpolated value through Math_Bezier3D | |
static int | s_Math_distPointLineX |
x coordinate of orthogonal projection of point on a segment using Math_distPointLine | |
static int | s_Math_distPointLineY |
y coordinate of orthogonal projection of point on a segment using Math_distPointLine | |
static final int | s_math_F_05 = (s_math_F_1>>1) |
Fixed point value for number 0.5. | |
static final int | s_math_F_1 = 1 << GLLibConfig.math_fixedPointBase |
Fixed point value for number 1. | |
static int | s_Math_intersectPoints [][] = new int[2][2] |
static int | s_Math_intersectX |
x coordinate of intersection point of 2 segment using Math_segmentIntersect | |
static int | s_Math_intersectY |
y coordinate of intersection point of 2 segment using Math_segmentIntersect | |
static java.util.Random | s_math_random |
Random number generator. |
Detailed Description
mathematic function
Function Documentation
static String ConvertFixedPointToString | ( | int | value, | |
int | precision | |||
) | [static, package, inherited] |
Converts a GLLib Fixed Point number to a string in base 10.
- Parameters:
-
value Fixed Point value to be converted. precision Number or of decimals to print.
- Returns:
- String representaion of Fixed Point number in base 10
static final long Math_Abs | ( | long | a | ) | [static, package, inherited] |
static final int Math_Abs | ( | int | a | ) | [static, package, inherited] |
static int Math_Atan | ( | int | dx, | |
int | dy | |||
) | [static, package, inherited] |
Arctangent.
- Note:
- this function will create a cache to speed up calculation if GLLibConfig.math_AtanUseCacheTable is set to true, but will also consume up to ((1<<math_fixedPointBase)+1)*4 byte
- Parameters:
-
dx,dy Slope of the angle.
- Returns:
- Arctangent of the slope.
- Note:
- This is not the real atan func as found in common device, it will return an angle in [0, 1] and not in [0, PI/2].
static int Math_AtanSlow | ( | int | dx, | |
int | dy | |||
) | [static, package, inherited] |
Arctangent, very slow but accurate method, it find angle by dichotomy.
- Parameters:
-
dx,dy Slope of the angle.
- Returns:
- Arctangent of the slope.
- Note:
- This is not the real atan func as found in common device, it will return an angle in [0, 1] and not in [0, PI/2].
static void Math_Bezier2D | ( | int | x1, | |
int | y1, | |||
int | x2, | |||
int | y2, | |||
int | x3, | |||
int | y3, | |||
int | interp | |||
) | [static, package, inherited] |
Three control point 2D Bezier interpolation.
- Parameters:
-
x1,y1 First control point. x2,y2 Second control point. x3,y3 Third control point. interp Interpolation value (ranges from 0 to s_math_F_1).
- Returns:
- Result returned in s_math_bezierX, s_math_bezierY.
static void Math_Bezier3D | ( | int | x1, | |
int | y1, | |||
int | z1, | |||
int | x2, | |||
int | y2, | |||
int | z2, | |||
int | x3, | |||
int | y3, | |||
int | z3, | |||
int | interp | |||
) | [static, package, inherited] |
Three control point 3D Bezier interpolation.
- Parameters:
-
x1,y1,z1 First control point. x2,y2,z2 Second control point. x3,y3,z3 Third control point. interp Interpolation value (ranges from 0 to s_math_F_1).
- Returns:
- Result returned in s_math_bezierX, s_math_bezierY, s_math_bezierZ.
static int Math_Cos | ( | int | angle | ) | [static, package, inherited] |
Cosinus.
- Parameters:
-
angle - angle in fixed point 0=0 (1<<math_angleFixedPointBase)=360.
- Returns:
- Cosinus value of the angle in fixed Point
static int Math_DegreeToFixedPointAngle | ( | int | a | ) | [static, package, inherited] |
Convert a degree angle into a fixed point angle.
- Parameters:
-
an angle in degrees.
- Returns:
- angle in fixed point.
static int Math_Det | ( | int | x1, | |
int | y1, | |||
int | x2, | |||
int | y2 | |||
) | [static, package, inherited] |
Compute the determinant of 2 2Dvector.
- Parameters:
-
x1,y1 X & Y value of the first vector. x2,y2 X & Y value of the second vector.
- Returns:
- The determinant of the 2 vectors.
static int Math_DistPointLine | ( | int | x0, | |
int | y0, | |||
int | x1, | |||
int | y1, | |||
int | x2, | |||
int | y2 | |||
) | throws Exception [static, package, inherited] |
Compute the distance from a point to a segment/line.
Calculate coordinate of orthogonal projection of point on segment as well.
- Parameters:
-
(x0,y0)(x1,y1) Coordinate of segment. (x2,y2) Coordinate of point.
- Returns:
- Distance from point to segment (orthogonal projection) in fixed point.
- Note:
- Coordinates of projected point are saved in (s_Math_distPointLineX, s_Math_distPointLineY).
static int Math_Div10 | ( | int | a | ) | [static, package, inherited] |
Divide by 10 a number, with result in fixed point.
Faster than classical division.
- Parameters:
-
a Number to divide by 10.
- Returns:
- Fixed point value of the number divided by 10.
static int Math_DotProduct | ( | int | x1, | |
int | y1, | |||
int | x2, | |||
int | y2 | |||
) | [static, package, inherited] |
Compute the dot product of 2 2Dvector.
- Parameters:
-
x1,y1 X & Y value of the first vector. x2,y2 X & Y value of the second vector.
- Returns:
- The dot product of the 2 vectors.
static int Math_FixedPoint_Add | ( | int | summand1, | |
int | summand2 | |||
) | [static, package, inherited] |
Addition for Fixed Point.
- Parameters:
-
summand1 Number to be added in Fixed Point. summand2 Number to be added in Fixed Point.
- Returns:
- Sum of summand1 and summand2 in Fixed Point.
- Note:
- An assert will indicate if the result overflows Integer.MAX_VALUE.
static int Math_FixedPoint_DegreesToAngleFixedPoint | ( | int | angle | ) | [static, package, inherited] |
Convert an angle from degrees to fixed point angle.
- Parameters:
-
angle in degrees to be converted.
- Returns:
- angle in Fixed Point.
static int Math_FixedPoint_DegreesToRadians | ( | int | angle | ) | [static, package, inherited] |
Convert an angle from degrees to radians (both fixed point and not, since this is just a ratio).
- Parameters:
-
angle in degrees to be converted into radians.
- Returns:
- angle in radians. (same precision as input param)
static int Math_FixedPoint_Det | ( | int | x1, | |
int | y1, | |||
int | x2, | |||
int | y2 | |||
) | [static, package, inherited] |
Compute the determinant of 2 2Dvector in Fixed Point.
- Parameters:
-
x1,y1 X & Y value of the first vector in Fixed Point. x2,y2 X & Y value of the second vector in Fixed Point.
- Returns:
- The determinant of the 2 vectors in Fixed Point.
static int Math_FixedPoint_Divide | ( | int | dividend, | |
int | divisor | |||
) | [static, package, inherited] |
Division for Fixed Point.
- Parameters:
-
dividend Number to be divided in Fixed Point. divisor Number to be divided by in Fixed Point.
- Returns:
- Quotient of dividend and divisor in Fixed Point.
- Note:
- An assert will indicate a division by zero.
static int Math_FixedPoint_DotProduct | ( | int | x1, | |
int | y1, | |||
int | x2, | |||
int | y2 | |||
) | [static, package, inherited] |
Compute the dot product of 2 2Dvector in Fixed Point.
- Parameters:
-
x1,y1 X & Y value of the first vector in Fixed Point. x2,y2 X & Y value of the second vector in Fixed Point.
- Returns:
- The dot product of the 2 vectors in Fixed Point.
static int Math_FixedPoint_LineCircleIntersect | ( | int | in_x1, | |
int | in_y1, | |||
int | in_x2, | |||
int | in_y2, | |||
int | in_circleX, | |||
int | in_circleY, | |||
int | in_radius | |||
) | [static, package, inherited] |
Find intersect points between a line and a circle.
- Parameters:
-
(in_x1,in_y1) first point on the line. (in_x2,in_y2) second point on the line. (in_circleX,in_circleY) center coordinates of circle. (in_radius) radius of the circle.
- Returns:
- MATH_INTERSECT_NO_INTERSECT if no intersect between line and circle. MATH_INTERSECT_ONE_POINT if only one intersect point between line and circle (Tangeant). MATH_INTERSECT_TWO_POINTS if two intersect ponts between line and circle.
- Note:
- Coordinates of intersection points are saved in the array s_Math_intersectPoints[][] First index indicates point: 0 = first point and 1 = second point Second index indicates x or y coordinate: 0 = x coordinate and 1 = y coordinate
static int Math_FixedPoint_LineRectangleIntersect | ( | int | in_x1, | |
int | in_y1, | |||
int | in_x2, | |||
int | in_y2, | |||
int | in_rectX, | |||
int | in_rectY, | |||
int | in_rectW, | |||
int | in_rectH | |||
) | [static, package, inherited] |
Find intersect points between a line and a rectangle.
- Parameters:
-
(in_x1,in_y1) first point on the line. (in_x2,in_y2) second point on the line. (in_rectX,in_rectY) coordinates of top-left corner of rectangle. (in_rectW,in_rectH) Width and Height of rectangle.
- Returns:
- MATH_INTERSECT_NO_INTERSECT if no intersect between line and rectangle. MATH_INTERSECT_ONE_POINT if only one intersect point between line and rectangle . MATH_INTERSECT_TWO_POINTS if two intersect points between line and circle.
- Note:
- Coordinates of intersection points are saved in the array s_Math_intersectPoints[][] First index indicates point: 0 = first point and 1 = second point Second index indicates x or y coordinate: 0 = x coordinate and 1 = y coordinate
static int Math_FixedPoint_Multiply | ( | int | multiplicand, | |
int | multiplier | |||
) | [static, package, inherited] |
Multiplication for Fixed Point.
- Parameters:
-
multiplicand Number to be mutiplied in Fixed Point. multiplier Number to be multiplied by in Fixed Point.
- Returns:
- Product of multiplicand and multiplier in Fixed Point.
- Note:
- An assert will indicate if the result overflows Integer.MAX_VALUE.
static int Math_FixedPoint_Norm | ( | int | x, | |
int | y | |||
) | [static, package, inherited] |
Nnormal of a vector in Fixed Point.
- Parameters:
-
x,y X & Y value of the vector in Fixed Point.
- Returns:
- norm of vector (x,y).
static int Math_FixedPoint_NormPow | ( | int | x, | |
int | y | |||
) | [static, package, inherited] |
Compute pow of the normal of a vector in Fixed Point.
- Parameters:
-
x,y X & Y value of the vector in Fixed Point.
- Returns:
- The pow of the normal of the vector in Fixed Point.
- Note:
- An assert will indicate if the result overflows Integer.MAX_VALUE.
static int Math_FixedPoint_PointLineDistance | ( | int | in_lineX1, | |
int | in_lineY1, | |||
int | in_lineX2, | |||
int | in_lineY2, | |||
int | in_pointX, | |||
int | in_pointY | |||
) | [static, package, inherited] |
Compute the distance from a point to a segment/line.
Calculate coordinate of orthogonal projection of point on segment as well.
- Parameters:
-
(in_lineX1,in_lineY1)(in_lineX2,in_lineY2) Coordinate of segment. (in_pointX,in_pointY) Coordinate of point.
- Returns:
- Distance from point to segment (orthogonal projection) in fixed point.
- Note:
- Coordinates of intersection points in fixed Point are saved in (s_Math_distPointLineX, s_Math_distPointLineY).
static int Math_FixedPoint_RadiansToAngleFixedPoint | ( | int | angle | ) | [static, package, inherited] |
Convert an angle from radians to fixed point angle.
- Parameters:
-
angle in radians to be converted.
- Returns:
- angle in Fixed Point.
static int Math_FixedPoint_RadiansToDegrees | ( | int | angle | ) | [static, package, inherited] |
Convert an angle from radians to degrees (both fixed point and not, since this is just a ratio).
- Parameters:
-
angle in radians to be converted into degrees.
- Returns:
- angle in degress. (same precision as input param)
static int Math_FixedPoint_Round | ( | int | value | ) | [static, package, inherited] |
Rounds value to closest whole number in Fixed Point.
- Parameters:
-
value Value to be rounded in Fixed Point.
- Returns:
- Rounded value in Fixed Point.
static int Math_FixedPoint_Sqrt | ( | long | value | ) | [static, package, inherited] |
Square Root for Long Fixed Point values.
- Parameters:
-
value Number to get square root of.
- Returns:
- Square root of val in Fixed Point.
- Note:
- Iterative process, precise but can be slow, use with caution.
static int Math_FixedPoint_Sqrt | ( | int | value | ) | [static, package, inherited] |
Square Root for Integer Fixed Point values.
- Parameters:
-
value Number to get square root of.
- Returns:
- Square root of val in Fixed Point.
- Note:
- Iterative process, precise but can be slow, use with caution.
static int Math_FixedPoint_Square | ( | int | value | ) | [static, package, inherited] |
Square for Fixed Point.
- Parameters:
-
value Number to be squared in Fixed Point.
- Returns:
- Squared value in Fixed Point.
static int Math_FixedPoint_Subtract | ( | int | minuend, | |
int | subtrahend | |||
) | [static, package, inherited] |
Subtraction for Fixed Point.
- Parameters:
-
minuend Number to be subtracted from in Fixed Point. subtrahend Number to be subtracted in Fixed Point.
- Returns:
- Difference of minuend and subtrahend in Fixed Point.
- Note:
- An assert will indicate if the result overflows Integer.MAX_VALUE.
static int Math_FixedPointAdjust | ( | int | a | ) | [static, package, inherited] |
Adjust a fixed point in base 8 to this fixed point base.
- Parameters:
-
a Value to adjsut.
- Returns:
- The value ajusted.
static int Math_FixedPointAngleToDegree | ( | int | a | ) | [static, package, inherited] |
Convert a fixed point angle into a degree angle.
- Parameters:
-
an angle in fixed point.
- Returns:
- angle in degrees.
static int Math_FixedPointToInt | ( | int | a | ) | [static, package, inherited] |
Convert a fixed point value to an int value.
- Parameters:
-
a Fixed point value to convert to int.
- Returns:
- Int value of a.
static void Math_Init | ( | String | packName, | |
int | cos, | |||
int | sqrt | |||
) | throws Exception [static, package, inherited] |
Math initialisation, by reading specified table from a package.
- Parameters:
-
packName Name of the pack witch contains math table. cos Index of cosine table in the pack. Use -1 if no table but all call to Math_Cos & Math_Sin will fail. sqrt Index of sqrt table in the pack. Use -1 if no table but all call to Math_sqrt will fail.
- Returns:
- True if everything was loaded as requested.
static int Math_IntToFixedPoint | ( | int | a | ) | [static, package, inherited] |
Convert a fixed point value to an int value.
- Parameters:
-
a Value to convert to fixed point.
- Returns:
- Fixed point value of a.
static int Math_Log2 | ( | int | a | ) | [static, package, inherited] |
return log base 2
- Parameters:
-
a value to calculate log base 2
- Returns:
- log base 2 of value
static final long Math_Max | ( | long | a, | |
long | b | |||
) | [static, package, inherited] |
static final int Math_Max | ( | int | a, | |
int | b | |||
) | [static, package, inherited] |
static final long Math_Min | ( | long | a, | |
long | b | |||
) | [static, package, inherited] |
static final int Math_Min | ( | int | a, | |
int | b | |||
) | [static, package, inherited] |
static int Math_Norm | ( | int | x, | |
int | y, | |||
int | iter | |||
) | [static, package, inherited] |
Norm following newton law approximation.
Sqrt(n) is provided by iteration of xk = (xk + n / xk) / 2. The more iteration (k++), the better is the approximation.
- Parameters:
-
x,y X and Y coordinate of vector to get the norm from. iter Number of iteration to perform, more iteration gives a better result however more iteration are more cpu intensive.
- Returns:
- norm of vector (x,y).
static int Math_NormPow | ( | int | x1, | |
int | y1 | |||
) | [static, package, inherited] |
Compute pow of the normal of a vector.
- Parameters:
-
x1,y1 X & Y value of the vector.
- Returns:
- The pow of the normal of the vector.
static void Math_QuickSort | ( | int | array[] | ) | [static, package, inherited] |
Quicksort an array of integer.
- Parameters:
-
array Array of data to sort.
static int [] Math_QuickSortIndices | ( | int | data[], | |
int | nbItemPerValue, | |||
int | itemNb | |||
) | [static, package, inherited] |
Get an array of indice corresponding to the sorting of an array of data.
Example: data={12, 3, 8} return {1, 2, 0} so that data[1] <= data[2] <= data[0].
- Parameters:
-
data Array of data to scale. nbItemPerValue If data is an array of values, how many item are needed per value. itemNb If data is an array of values, which item shoudl be considered for sorting.
- Returns:
- Array of indices, so that data[indices] are sorted.
static int [] Math_QuickSortIndices | ( | int | data[] | ) | [static, package, inherited] |
Get an array of indice corresponding to the sorting of an array of data.
Example: data={12, 3, 8} return {1, 2, 0} so that data[1] <= data[2] <= data[0].
- Parameters:
-
data Array of data to scale.
- Returns:
- Array of indices, so that data[indices] are sorted.
static void Math_Quit | ( | ) | [static, package, inherited] |
Free all math arrays.
static final int Math_Rand | ( | ) | [static, package, inherited] |
Create a random number.
- Returns:
- a random int.
static int Math_Rand | ( | int | a, | |
int | b | |||
) | [static, package, inherited] |
Create a random int inside the interval [a, b[.
- Parameters:
-
a,b Interval for the random number to generate.
- Returns:
- A number between [a, b[.
- Note:
- if a=b then return value is a (or b)
static final void Math_RandSetSeed | ( | long | seed | ) | [static, package, inherited] |
set math random seed
- Parameters:
-
seed seed to use for random number generator.
static boolean Math_RectIntersect | ( | int | Ax0, | |
int | Ay0, | |||
int | Ax1, | |||
int | Ay1, | |||
int | Bx0, | |||
int | By0, | |||
int | Bx1, | |||
int | By1 | |||
) | [static, package, inherited] |
Tell if 2 axis aligned rectangle intersect.
- Parameters:
-
(Ax0,Ay0)(Ax1,Ay1) top left and bottom right coordinate of first rectangle (Bx0,By0)(Bx1,By1) top left and bottom right coordinate of second rectangle
- Returns:
- true if intersect, false if not
static boolean Math_SameSign | ( | int | a, | |
int | b | |||
) | [static, package, inherited] |
Test 2 numbers to see if they are both of the same sign.
- Parameters:
-
a,b Numbers to compare.
- Returns:
- True if both numbers are positive.
True if both numbers are negative.
False Otherwise.
static int Math_SegmentIntersect | ( | int | x1, | |
int | y1, | |||
int | x2, | |||
int | y2, | |||
int | x3, | |||
int | y3, | |||
int | x4, | |||
int | y4 | |||
) | [static, package, inherited] |
Tell if 2 segment intersect themselves.
- Parameters:
-
(x1,y1)(x2,y2) Coordinate of first segment. (x3,y3)(x4,y4) Coordinate of second segment.
- Returns:
- MATH_SEGMENTINTERSECT_DO_INTERSECT if intersect.
- MATH_SEGMENTINTERSECT_DONT_INTERSECT if no intersection.
- MATH_SEGMENTINTERSECT_COLLINEAR if two segment are colinear (full or no intersection).
- Note:
- Coordinates of intersection point are saved in (s_Math_intersectX, s_Math_intersectY).
- Warning:
- This function can generate some Overflows if large value are used. Be carefull when using this code with FixedPoint values, the boundaries can change with the value of GLLibConfig.math_fixedPointBase.
static int Math_Sin | ( | int | a | ) | [static, package, inherited] |
Sinus.
- Parameters:
-
a Angle in fixed point 0=0 (1<<math_angleFixedPointBase)=360.
- Returns:
- Sinus value of the angle.
static int Math_Sqrt | ( | long | val | ) | [static, package, inherited] |
Square Root slow.
- Parameters:
-
val Number to get square root of.
- Returns:
- Square root of val.
- Note:
- long version is way slower than int function
static int Math_Sqrt | ( | int | x | ) | [static, package, inherited] |
Square Root.
- Parameters:
-
x Number to get square root of.
- Returns:
- Square root of x.
- Note:
- Integer Square Root function. Contributors include Arne Steinarson for the basic approximation idea, Dann Corbit and Mathew Hendry for the first cut at the algorithm, Lawrence Kirby for the rearrangement, improvments and range optimization, Paul Hsieh for the round-then-adjust idea, and Tim Tyler, for the Java port.
static int Math_Sqrt_FixedPoint | ( | int | val, | |
int | precisionLoop | |||
) | [static, package, inherited] |
Square Root for Fixed Point.
- Parameters:
-
val Number to get square root of. precisionLoop Number of time to go through the algo to get good presicion in the result. Good value are [10-30] 15 is usualy good.
- Returns:
- Square root of val in Fixed Point.
- Note:
- Iterative process, can be slow, use with caution.
static int Math_Tan | ( | int | angle | ) | [static, package, inherited] |
Tangent.
- Parameters:
-
angle - Angle in fixed point 0=0 256=360
- Returns:
- Tangent value of the angle.
Variable Documentation
final int Math_Angle180 = Math_DegreeToFixedPointAngle(180) [static, package, inherited] |
180 in fixed point
final int Math_Angle270 = Math_DegreeToFixedPointAngle(270) [static, package, inherited] |
270 in fixed point
final int Math_Angle360 = Math_DegreeToFixedPointAngle(360) [static, package, inherited] |
360 in fixed point
final int Math_Angle90 = Math_DegreeToFixedPointAngle(90) [static, package, inherited] |
90 in fixed point
final int Math_AngleMUL = 1 << GLLibConfig.math_angleFixedPointBase [static, package, inherited] |
angle fixed point multiplier eg one degree in fixed point base.(not equal to Math_DegreeToFixedPointAngle(1))
final int Math_FixedPoint_E = 1459366444 >> (29 - GLLibConfig.math_fixedPointBase) [static, package, inherited] |
final int Math_FixedPoint_PI = 1686629713 >> (29 - GLLibConfig.math_fixedPointBase) [static, package, inherited] |
final int MATH_INTERSECT_NO_INTERSECT = 0 [static, package, inherited] |
final int MATH_INTERSECT_ONE_POINT = (1 << 1) [static, package, inherited] |
final int MATH_INTERSECT_TWO_POINTS = (1 << 2) [static, package, inherited] |
final int MATH_SEGMENTINTERSECT_COLLINEAR = -1 [static, package, inherited] |
return value of Math_segmentIntersect if there is no or full intersection because both segment are colinear
final int MATH_SEGMENTINTERSECT_DO_INTERSECT = 1 [static, package, inherited] |
return value of Math_segmentIntersect if there is an intersection
final int MATH_SEGMENTINTERSECT_DONT_INTERSECT = 0 [static, package, inherited] |
return value of Math_segmentIntersect if there is no intersection
int s_math_bezierX [static, package, inherited] |
x coordinate of bezier interpolated value through Math_Bezier2D or Math_Bezier3D
int s_math_bezierY [static, package, inherited] |
y coordinate of bezier interpolated value through Math_Bezier2D or Math_Bezier3D
int s_math_bezierZ [static, package, inherited] |
z coordinate of bezier interpolated value through Math_Bezier3D
int s_Math_distPointLineX [static, package, inherited] |
x coordinate of orthogonal projection of point on a segment using Math_distPointLine
int s_Math_distPointLineY [static, package, inherited] |
y coordinate of orthogonal projection of point on a segment using Math_distPointLine
final int s_math_F_05 = (s_math_F_1>>1) [static, package, inherited] |
Fixed point value for number 0.5.
final int s_math_F_1 = 1 << GLLibConfig.math_fixedPointBase [static, package, inherited] |
Fixed point value for number 1.
int s_Math_intersectPoints[][] = new int[2][2] [static, package, inherited] |
int s_Math_intersectX [static, package, inherited] |
x coordinate of intersection point of 2 segment using Math_segmentIntersect
int s_Math_intersectY [static, package, inherited] |
y coordinate of intersection point of 2 segment using Math_segmentIntersect
java.util.Random s_math_random [static, package, inherited] |
Random number generator.
Generated on Tue Sep 23 23:05:30 2008 for GLLib by
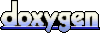