![]() |
CoffeeLake Intel(R) Firmware Support Package (FSP) Integration Guide
|
GpioConfig.h
Go to the documentation of this file.
45 UINT32 PadMode : 5;
51 UINT32 HostSoftPadOwn : 2;
54 Can choose between In, In with inversion, Out, both In and Out, both In with inversion and out or disabling both.
57 UINT32 Direction : 6;
64 UINT32 OutputState : 2;
71 UINT32 InterruptConfig : 9;
77 UINT32 PowerConfig : 8;
83 UINT32 ElectricalConfig : 9;
89 UINT32 LockConfig : 4;
94 UINT32 OtherSettings : 9;
197 GpioIntEdge = (0x3 << 5), ///< Set interrupt as edge triggered (type of edge depends on input inversion)
202 #define B_GPIO_INT_CONFIG_INT_SOURCE_MASK 0x1F ///< Mask for GPIO_INT_CONFIG for interrupt source
283 #define B_GPIO_ELECTRICAL_CONFIG_TERMINATION_MASK 0x1F ///< Mask for GPIO_ELECTRICAL_CONFIG for termination value
294 functions which allow to unlock a GPIO pad. If possible each GPIO lib function will try to unlock
312 #define B_GPIO_LOCK_CONFIG_PAD_CONF_LOCK_MASK 0x3 ///< Mask for GPIO_LOCK_CONFIG for Pad Configuration Lock
313 #define B_GPIO_LOCK_CONFIG_OUTPUT_LOCK_MASK 0xC ///< Mask for GPIO_LOCK_CONFIG for Pad Output Lock
GPIO_RESET_CONFIG
GPIO Power Configuration GPIO_RESET_CONFIG allows to set GPIO Reset type (PADCFG_DW0.PadRstCfg) which will be used to reset certain GPIO settings.
Definition: GpioConfig.h:211
GPIO_OUTPUT_STATE
GPIO Output State This field is relevant only if output is enabled.
Definition: GpioConfig.h:163
Deep Sleep Well Reset (DSW_PWROK) GPP: not applicable GPD: PadRstCfg = 00b = "Powergood" Pad settings...
Definition: GpioConfig.h:257
Native function controls pads termination This setting is applicable only to some native modes...
Definition: GpioConfig.h:280
GPIO_OTHER_CONFIG
Other GPIO Configuration GPIO_OTHER_CONFIG is used for less often settings and for future extensions ...
Definition: GpioConfig.h:323
GPIO_ELECTRICAL_CONFIG
GPIO Electrical Configuration Configuration options for GPIO termination setting. ...
Definition: GpioConfig.h:264
Resume Reset (RSMRST) GPP: PadRstCfg = 00b = "Powergood" GPD: PadRstCfg = 11b = "Resume Reset" Pad se...
Definition: GpioConfig.h:224
GPIO_HOSTSW_OWN
Host Software Pad Ownership modes This setting affects GPIO interrupt status registers.
Definition: GpioConfig.h:128
GPIO_LOCK_CONFIG
GPIO LockConfiguration Set GPIO configuration lock and output state lock.
Definition: GpioConfig.h:297
Set interrupt as edge triggered (type of edge depends on input inversion)
Definition: GpioConfig.h:197
GPIO_INT_CONFIG
GPIO interrupt configuration This setting is applicable only if pad is in GPIO mode and has input ena...
Definition: GpioConfig.h:189
Platform Reset (PLTRST) PadRstCfg = 10b = "GPIO Reset" Pad settings will reset on: ...
Definition: GpioConfig.h:245
Host Deep Reset PadRstCfg = 01b = "Deep GPIO Reset" Pad settings will reset on:
Definition: GpioConfig.h:235
GPIO_PAD_MODE
GPIO Pad Mode Refer to GPIO documentation on native functions available for certain pad...
Definition: GpioConfig.h:113
Generated on Wed Aug 22 2018 17:48:55 for CoffeeLake Intel(R) Firmware Support Package (FSP) Integration Guide by
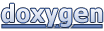