![]() |
CoffeeLake Intel(R) Firmware Support Package (FSP) Integration Guide
|
FspsUpd.h
Go to the documentation of this file.
72 /// The PCH_DEVICE_INTERRUPT_CONFIG block describes interrupt pin, IRQ and interrupt mode for PCH device.
117 UINT8 UnusedUpdSpace0[3];
145 UINT8 UnusedUpdSpace1[3];
173 UINT8 SataPortsEnable[8];
179 UINT8 SataPortsDevSlp[8];
185 UINT8 PortUsb20Enable[16];
191 UINT8 PortUsb30Enable[10];
201 UINT8 UnusedUpdSpace2[2];
209 UINT8 SerialIoDevMode[12];
227 UINT8 PxRcConfig[8];
281 UINT8 Usb2AfePetxiset[16];
287 UINT8 Usb2AfeTxiset[16];
293 UINT8 Usb2AfePredeemp[16];
299 UINT8 Usb2AfePehalfbit[16];
305 UINT8 Usb3HsioTxDeEmphEnable[10];
311 UINT8 Usb3HsioTxDeEmph[10];
317 UINT8 Usb3HsioTxDownscaleAmpEnable[10];
323 UINT8 Usb3HsioTxDownscaleAmp[10];
468 UINT8 PchPostMemRsvd[29];
636 UINT8 UnusedUpdSpace5[1];
641 UINT8 PcieRpSlotImplemented[24];
647 UINT8 PcieClkSrcUsage[16];
652 UINT8 PcieClkSrcClkReq[16];
657 UINT8 PcieRpAcsEnabled[24];
663 UINT8 PcieRpEnableCpm[24];
670 UINT16 PcieRpDetectTimeoutMs[24];
687 UINT8 UnusedUpdSpace6[3];
705 UINT8 PegDeEmphasis[4];
710 UINT8 PegSlotPowerLimitValue[4];
716 UINT8 PegSlotPowerLimitScale[4];
721 UINT16 PegPhysicalSlotNumber[4];
760 UINT32 VtdBaseAddress[3];
845 UINT8 SaPostMemProductionRsvd[35];
851 UINT8 PcieRootPortGen2PllL1CgDisable[24];
863 UINT8 Psi3Enable[5];
869 UINT8 Psi4Enable[5];
875 UINT8 ImonSlope[5];
881 UINT8 ImonOffset[5];
886 UINT8 VrConfigEnable[5];
892 UINT8 TdcEnable[5];
899 UINT8 TdcTimeWindow[5];
905 UINT8 TdcLock[5];
959 UINT16 TdcPowerLimit[5];
965 UINT16 AcLoadline[5];
969 UINT8 UnusedUpdSpace8[10];
975 UINT16 DcLoadline[5];
980 UINT16 Psi1Threshold[5];
985 UINT16 Psi2Threshold[5];
990 UINT16 Psi3Threshold[5];
995 UINT16 IccMax[5];
1000 UINT16 VrVoltageLimit[5];
1037 UINT8 UnusedUpdSpace9[6];
1105 UINT16 ImonSlope1[5];
1155 UINT8 ReservedCpuPostMemProduction[18];
1172 UINT8 PchWriteProtectionEnable[5];
1177 UINT8 PchReadProtectionEnable[5];
1183 UINT16 PchProtectedRangeLimit[5];
1188 UINT16 PchProtectedRangeBase[5];
1241 UINT8 PchUsbHsioFilterSel[10];
1245 UINT8 UnusedUpdSpace11[5];
1360 UINT8 UnusedUpdSpace13[3];
1385 UINT8 PcieRpHotPlug[24];
1390 UINT8 PcieRpPmSci[24];
1395 UINT8 PcieRpExtSync[24];
1400 UINT8 PcieRpTransmitterHalfSwing[24];
1405 UINT8 PcieRpClkReqDetect[24];
1410 UINT8 PcieRpAdvancedErrorReporting[24];
1415 UINT8 PcieRpUnsupportedRequestReport[24];
1420 UINT8 PcieRpFatalErrorReport[24];
1425 UINT8 PcieRpNoFatalErrorReport[24];
1430 UINT8 PcieRpCorrectableErrorReport[24];
1435 UINT8 PcieRpSystemErrorOnFatalError[24];
1440 UINT8 PcieRpSystemErrorOnNonFatalError[24];
1445 UINT8 PcieRpSystemErrorOnCorrectableError[24];
1450 UINT8 PcieRpMaxPayload[24];
1456 UINT8 PchUsbHsioRxTuningParameters[10];
1459 Mask for enabling tuning of HSIO Rx signals of USB3 ports. Bits: 0 - HsioCtrlAdaptOffsetCfgEnable,
1462 UINT8 PchUsbHsioRxTuningEnable[10];
1466 UINT8 UnusedUpdSpace14[4];
1472 UINT8 PcieRpPcieSpeed[24];
1478 UINT8 PcieRpGen3EqPh3Method[24];
1483 UINT8 PcieRpPhysicalSlotNumber[24];
1486 The root port completion timeout(see: PCH_PCIE_COMPLETION_TIMEOUT). Default is PchPcieCompletionTO_Default.
1488 UINT8 PcieRpCompletionTimeout[24];
1492 UINT8 UnusedUpdSpace15[106];
1498 UINT8 PcieRpAspm[24];
1504 UINT8 PcieRpL1Substates[24];
1509 UINT8 PcieRpLtrEnable[24];
1514 UINT8 PcieRpLtrConfigLock[24];
1519 UINT8 PcieEqPh3LaneParamCm[24];
1524 UINT8 PcieEqPh3LaneParamCp[24];
1529 UINT8 PcieSwEqCoeffListCm[5];
1534 UINT8 PcieSwEqCoeffListCp[5];
1587 UINT8 SerialIoSpiCsPolarity[3];
1605 Corresponds to the WOL Enable Override bit in the General PM Configuration B (GEN_PMCON_B) register.
1617 Determine if WLAN wake from Sx, corresponds to the HOST_WLAN_PP_EN bit in the PWRM_CFG3 register.
1691 UINT8 UnusedUpdSpace18[3];
1787 UINT8 SataPortsHotPlug[8];
1792 UINT8 SataPortsInterlockSw[8];
1797 UINT8 SataPortsExternal[8];
1802 UINT8 SataPortsSpinUp[8];
1807 UINT8 SataPortsSolidStateDrive[8];
1812 UINT8 SataPortsEnableDitoConfig[8];
1817 UINT8 SataPortsDmVal[8];
1822 UINT16 SataPortsDitoVal[8];
1827 UINT8 SataPortsZpOdd[8];
1904 UINT8 SataRstPcieEnable[3];
1909 UINT8 SataRstPcieStoragePort[3];
1914 UINT8 SataRstPcieDeviceResetDelay[3];
1950 UINT8 PchSerialIoI2cPadsTermination[6];
1964 UINT8 UnusedUpdSpace22[1];
1969 UINT8 SerialIoUartHwFlowCtrl[3];
2173 UINT8 PchMemoryPmsyncEnable[2];
2178 UINT8 PchMemoryC0TransmitEnable[2];
2183 UINT8 PchMemoryPinSelection[2];
2200 UINT8 Usb2OverCurrentPin[16];
2205 UINT8 Usb3OverCurrentPin[10];
2258 UINT64 BgpdtHash[4];
2346 ASPM configuration on the PCH side of the DMI/OPI Link. Default is <b>PchPcieAspmAutoConfig</b>
2353 UINT8 ReservedFspsUpd[1];
2401 UINT8 PegMaxPayload[4];
2451 UINT8 SaPostMemTestRsvd[11];
2901 UINT8 StateRatio[40];
2910 UINT8 StateRatioMax16[16];
2949 Package Long duration turbo mode power limit. Units are based on POWER_MGMT_CONFIG.CustomPowerUnit.
2955 Package Short duration turbo mode power limit. Units are based on POWER_MGMT_CONFIG.CustomPowerUnit.Valid
2979 Short term Power Limit value for custom cTDP level 1. Units are based on POWER_MGMT_CONFIG.CustomPowerUnit.Valid
2985 Long term Power Limit value for custom cTDP level 1. Units are based on POWER_MGMT_CONFIG.CustomPowerUnit.Valid
2991 Short term Power Limit value for custom cTDP level 2. Units are based on POWER_MGMT_CONFIG.CustomPowerUnit.Valid
2997 Long term Power Limit value for custom cTDP level 2. Units are based on POWER_MGMT_CONFIG.CustomPowerUnit.Valid
3003 Short term Power Limit value for custom cTDP level 3. Units are based on POWER_MGMT_CONFIG.CustomPowerUnit.Valid
3009 Long term Power Limit value for custom cTDP level 3. Units are based on POWER_MGMT_CONFIG.CustomPowerUnit.Valid
3126 UINT8 ReservedCpuPostMemTest[19];
3186 UINT16 PcieRpLtrMaxSnoopLatency[24];
3191 UINT16 PcieRpLtrMaxNoSnoopLatency[24];
3196 UINT8 PcieRpSnoopLatencyOverrideMode[24];
3201 UINT8 PcieRpSnoopLatencyOverrideMultiplier[24];
3206 UINT16 PcieRpSnoopLatencyOverrideValue[24];
3211 UINT8 PcieRpNonSnoopLatencyOverrideMode[24];
3216 UINT8 PcieRpNonSnoopLatencyOverrideMultiplier[24];
3221 UINT16 PcieRpNonSnoopLatencyOverrideValue[24];
3226 UINT8 PcieRpSlotPowerLimitScale[24];
3231 UINT16 PcieRpSlotPowerLimitValue[24];
3236 UINT8 PcieRpUptp[24];
3241 UINT8 PcieRpDptp[24];
3276 UINT8 UnusedUpdSpace24[17];
3292 UINT8 ReservedFspsTestUpd[12];
UINT32 PsysPowerLimit2Power
Offset 0x0894 - Platform PL2 power Platform PL2 power.
Definition: FspsUpd.h:3024
UINT8 ThreeCoreRatioLimit
Offset 0x07CD - 3-Core Ratio Limit 3-Core Ratio Limit: LFM to Fused, For overclocking part: LFM to 25...
Definition: FspsUpd.h:2471
UINT8 PchHdaAudioLinkDmic1
Offset 0x00FF - Enable HD Audio DMIC1 Link Enable/disable HD Audio DMIC1 link.
Definition: FspsUpd.h:347
UINT8 TcoIrqEnable
Offset 0x008B - Enable/Disable Tco IRQ Enable/disable TCO IRQ $EN_DIS.
Definition: FspsUpd.h:248
UINT8 PchHdaIDispLinkFrequency
Offset 0x036A - iDisp-Link Frequency iDisp-Link Freq (PCH_HDAUDIO_LINK_FREQUENCY enum): 4: 96MHz...
Definition: FspsUpd.h:1216
UINT16 CstateLatencyControl1Irtl
Offset 0x085A - Interrupt Response Time Limit of C-State LatencyContol1 Interrupt Response Time Limit...
Definition: FspsUpd.h:2926
UINT8 PchPmVrAlert
Offset 0x0151 - VRAlert# Pin When VRAlert# feature pin is enabled and its state is '0'...
Definition: FspsUpd.h:547
UINT8 PchTTLock
Offset 0x0715 - Thermal Throttle Lock Thermal Throttle Lock.
Definition: FspsUpd.h:2046
UINT32 Custom3PowerLimit2
Offset 0x088C - Long term Power Limit value for custom cTDP level 3 Long term Power Limit value for c...
Definition: FspsUpd.h:3012
UINT16 WatchDogTimerBios
Offset 0x015D - BIOS Timer 16 bits Value, Set BIOS watchdog timer.
Definition: FspsUpd.h:614
UINT8 SlowSlewRateForIa
Offset 0x02A4 - Slew Rate configuration for Deep Package C States for VR IA domain Slew Rate configur...
Definition: FspsUpd.h:939
UINT8 CridEnable
Offset 0x0200 - Enable/Disable SA CRID Enable: SA CRID, Disable (Default): SA CRID $EN_DIS...
Definition: FspsUpd.h:693
UINT32 BltBufferAddress
Offset 0x0234 - Blt Buffer Address Address of Blt buffer.
Definition: FspsUpd.h:833
UINT8 PchCnviMfUart1Type
Offset 0x014B - CNVi MfUart1 Type This option configures Uart type which connects to MfUart1 0:ISH Ua...
Definition: FspsUpd.h:511
UINT8 MeUnconfigOnRtcClear
Offset 0x07A8 - ME Unconfig on RTC clear 0: Disable ME Unconfig On Rtc Clear.
Definition: FspsUpd.h:2328
UINT8 ProcessorTraceEnable
Offset 0x07F2 - Enable or Disable Processor Trace feature Enable or Disable Processor Trace feature; ...
Definition: FspsUpd.h:2693
UINT16 PchT2Level
Offset 0x0711 - Thermal Throttling Custimized T2Level Value Custimized T2Level value.
Definition: FspsUpd.h:2027
UINT8 PchPmWoWlanDeepSxEnable
Offset 0x0673 - PCH Pm WoW lan DeepSx Enable Determine if WLAN wake from DeepSx, corresponds to the D...
Definition: FspsUpd.h:1627
UINT8 PchUsb2PhySusPgEnable
Offset 0x0148 - PCH USB2 PHY Power Gating enable 1: Will enable USB2 PHY SUS Well Power Gating...
Definition: FspsUpd.h:494
UINT8 SataSalpSupport
Offset 0x0041 - Enable SATA SALP Support Enable/disable SATA Aggressive Link Power Management...
Definition: FspsUpd.h:167
UINT8 PchLockDownRtcMemoryLock
Offset 0x0395 - RTC CMOS MEMORY LOCK Enable RTC lower and upper 128 byte Lock bits to lock Bytes 38h-...
Definition: FspsUpd.h:1380
UINT8 PchPmWolEnableOverride
Offset 0x0670 - PCH Pm Wol Enable Override Corresponds to the WOL Enable Override bit in the General ...
Definition: FspsUpd.h:1608
UINT8 UsbPdoProgramming
Offset 0x0114 - USB PDO Programming Enable/disable PDO programming for USB in PEI phase...
Definition: FspsUpd.h:420
UINT8 SataP0TDispFinit
Offset 0x0727 - Port 0 Alternate Fast Init Tdispatch Port 0 Alternate Fast Init Tdispatch.
Definition: FspsUpd.h:2145
UINT8 PsysPowerLimit2
Offset 0x07E9 - PL2 Enable Value PL2 Enable activates the PL2 value to limit average platform power...
Definition: FspsUpd.h:2639
UINT8 TTCrossThrottling
Offset 0x0717 - Enable PCH Cross Throttling Enable/Disable PCH Cross Throttling $EN_DIS.
Definition: FspsUpd.h:2058
UINT8 SataRstCpuAttachedStorage
Offset 0x0751 - PCH Sata Rst CPU Attached Storage CPU Attached Storage $EN_DIS.
Definition: FspsUpd.h:2225
UINT8 NumberOfEntries
Offset 0x07DB - Custom Ratio State Entries The number of custom ratio state entries, ranges from 0 to 40 for a valid custom ratio table.Sets the number of custom P-states.
Definition: FspsUpd.h:2560
UINT8 EnergyEfficientTurbo
Offset 0x0802 - Enable or Disable Energy Efficient Turbo Enable or Disable Energy Efficient Turbo...
Definition: FspsUpd.h:2731
UINT8 EdramTestMode
Offset 0x07B3 - EDRAM Test Mode Enable: PAM register will not be locked by RC, platform code should l...
Definition: FspsUpd.h:2382
UINT16 CstateLatencyControl2Irtl
Offset 0x085C - Interrupt Response Time Limit of C-State LatencyContol2 Interrupt Response Time Limit...
Definition: FspsUpd.h:2931
UINT8 PchLockDownBiosInterface
Offset 0x08C0 - Enable LOCKDOWN BIOS Interface Enable BIOS Interface Lock Down bit to prevent writes ...
Definition: FspsUpd.h:3162
UINT8 PchPmSlpStrchSusUp
Offset 0x0681 - PCH Pm Slp Strch Sus Up Enable SLP_X Stretching After SUS Well Power Up...
Definition: FspsUpd.h:1704
UINT16 FivrRfiFrequency
Offset 0x030F - FIVR RFI Frequency PCODE MMIO Mailbox: Set the desired RFI frequency, in increments of 100KHz.
Definition: FspsUpd.h:1062
UINT8 RemoteAssistance
Offset 0x015F - Remote Assistance Trigger Availablilty Enable/Disable.
Definition: FspsUpd.h:620
UINT8 C1e
Offset 0x080C - Enable or Disable Enhanced C-states Enable or Disable Enhanced C-states.
Definition: FspsUpd.h:2791
UINT8 PpmIrmSetting
Offset 0x0819 - Interrupt Redirection Mode Select Interrupt Redirection Mode Select.0: Fixed priority; 1: Round robin;2: Hash vector;4: PAIR with fixed priority;5: PAIR with round robin;6: PAIR with hash vector;7: No change.
Definition: FspsUpd.h:2869
UINT8 PchDmiAspmCtrl
Offset 0x07AB - Pch Dmi Aspm Ctrl ASPM configuration on the PCH side of the DMI/OPI Link...
Definition: FspsUpd.h:2349
UINT8 PchEspiLgmrEnable
Offset 0x014C - Espi Lgmr Memory Range decode This option enables or disables espi lgmr $EN_DIS...
Definition: FspsUpd.h:517
UINT8 MinRingRatioLimit
Offset 0x08A3 - Minimum Ring ratio limit override Minimum Ring ratio limit override.
Definition: FspsUpd.h:3102
UINT8 PchIshGp7GpioAssign
Offset 0x038D - Enable PCH ISH GP_7 GPIO pin assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1344
UINT8 DdiPortCDdc
Offset 0x022E - Enable or disable DDC of DDI port C 0=Disable, 1(Default)=Enable $EN_DIS.
Definition: FspsUpd.h:802
UINT8 SdCardPowerEnableActiveHigh
Offset 0x0147 - SdCard power enable polarity Choose SD_PWREN# polarity 0: Active low, 1: Active high.
Definition: FspsUpd.h:487
UINT8 IslVrCmd
Offset 0x0319 - Activates VR mailbox command for Intersil VR C-state issues.
Definition: FspsUpd.h:1099
UINT64 BiosGuardModulePtr
Offset 0x077D - BiosGuardModulePtr BiosGuardModulePtr default values.
Definition: FspsUpd.h:2268
UINT32 PowerLimit4
Offset 0x0870 - Package PL4 power limit Package PL4 power limit.
Definition: FspsUpd.h:2970
UINT8 SerialIoEnableDebugUartAfterPost
Offset 0x0707 - Enable Debug UART Controller Enable debug UART controller after post.
Definition: FspsUpd.h:1982
UINT32 ProcessorTraceMemLength
Offset 0x07FB - Memory region allocation for Processor Trace Length in bytes of memory region allocat...
Definition: FspsUpd.h:2705
UINT8 FastPkgCRampDisableSa
Offset 0x0302 - Disable Fast Slew Rate for Deep Package C States for VR SA domain Disable Fast Slew R...
Definition: FspsUpd.h:1014
UINT8 SixCoreRatioLimit
Offset 0x089B - 6-Core Ratio Limit 6-Core Ratio Limit: LFM to Fused, For overclocking part: LFM to 25...
Definition: FspsUpd.h:3051
UINT8 PsysPowerLimit1Time
Offset 0x07E8 - PL1 timewindow PL1 timewindow in seconds.
Definition: FspsUpd.h:2632
UINT8 PchLanEnable
Offset 0x00FC - Enable LAN Enable/disable LAN controller.
Definition: FspsUpd.h:329
UINT32 Custom1PowerLimit2
Offset 0x087C - Long term Power Limit value for custom cTDP level 1 Long term Power Limit value for c...
Definition: FspsUpd.h:2988
UINT8 PmcCpuC10GatePinEnable
Offset 0x07AA - Pmc Cpu C10 Gate Pin Enable Enable/Disable platform support for CPU_C10_GATE# pin to ...
Definition: FspsUpd.h:2343
UINT8 PchIshGp0GpioAssign
Offset 0x0386 - Enable PCH ISH GP_0 GPIO pin assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1302
UINT8 CstateLatencyControl3TimeUnit
Offset 0x0816 - TimeUnit for C-State Latency Control3 TimeUnit for C-State Latency Control3;Valid val...
Definition: FspsUpd.h:2852
UINT8 BiProcHot
Offset 0x0804 - Enable or Disable Bi-Directional PROCHOT# Enable or Disable Bi-Directional PROCHOT#; ...
Definition: FspsUpd.h:2743
UINT8 PchMemoryThrottlingEnable
Offset 0x072B - Enable Memory Thermal Throttling Enable Memory Thermal Throttling.
Definition: FspsUpd.h:2168
UINT8 PchLockDownBiosLock
Offset 0x0393 - Enable LOCKDOWN BIOS LOCK Enable the BIOS Lock feature and set EISS bit (D31:F5:RegDC...
Definition: FspsUpd.h:1367
UINT8 PchCrid
Offset 0x0394 - PCH Compatibility Revision ID This member describes whether or not the CRID feature o...
Definition: FspsUpd.h:1373
UINT8 SataLedEnable
Offset 0x0150 - SATA LED SATA LED indicating SATA controller activity.
Definition: FspsUpd.h:540
UINT32 PchHdaVerbTablePtr
Offset 0x008D - PCH HDA Verb Table Pointer Pointer to Array of pointers to Verb Table.
Definition: FspsUpd.h:258
UINT8 OneCoreRatioLimit
Offset 0x07CB - 1-Core Ratio Limit 1-Core Ratio Limit: LFM to Fused, For overclocking part: LFM to 25...
Definition: FspsUpd.h:2459
UINT8 SlpS0Override
Offset 0x067A - SLP_S0# Override Select 'Auto', it will be auto-configured according to probe type...
Definition: FspsUpd.h:1669
UINT8 PkgCStateUnDemotion
Offset 0x080E - Enable or Disable Package Cstate UnDemotion Enable or Disable Package Cstate UnDemoti...
Definition: FspsUpd.h:2803
UINT16 PchHdaResetWaitTimer
Offset 0x08BD - HD Audio Reset Wait Timer The delay timer after Azalia reset, the value is number of ...
Definition: FspsUpd.h:3150
UINT8 EnergyEfficientPState
Offset 0x0801 - Enable or Disable Energy Efficient P-state Enable or Disable Energy Efficient P-state...
Definition: FspsUpd.h:2724
UINT32 PcieRpDpcMask
Offset 0x010C - DPC for PCIE RP Mask Enable/disable Downstream Port Containment for PCIE Root Ports...
Definition: FspsUpd.h:407
UINT8 SataRstInterrupt
Offset 0x07A7 - SATA RST Interrupt Mode Allowes to choose which interrupts will be implemented by SAT...
Definition: FspsUpd.h:2320
UINT8 PchPmPwrCycDur
Offset 0x068A - PCH Pm Reset Power Cycle Duration Could be customized in the unit of second...
Definition: FspsUpd.h:1755
UINT32 PmcPowerButtonDebounce
Offset 0x0115 - Power button debounce configuration Debounce time for PWRBTN in microseconds.
Definition: FspsUpd.h:426
UINT8 CdynmaxClampEnable
Offset 0x07BC - Enable/Disable CdynmaxClamp Enable(Default): Enable CdynmaxClamp, Disable: Disable Cd...
Definition: FspsUpd.h:2419
UINT8 Custom1ConfigTdpControl
Offset 0x07DE - Custom Config Tdp Control Config Tdp Control (0/1/2) value for custom cTDP level 1...
Definition: FspsUpd.h:2576
UINT8 PchScsEmmcHs400RxStrobeDll1
Offset 0x06F7 - Rx Strobe Delay Control Rx Strobe Delay Control - Rx Strobe Delay DLL 1 (HS400 Mode)...
Definition: FspsUpd.h:1931
UINT16 CstateLatencyControl4Irtl
Offset 0x0860 - Interrupt Response Time Limit of C-State LatencyContol4 Interrupt Response Time Limit...
Definition: FspsUpd.h:2941
UINT8 PchPmSlpS3MinAssert
Offset 0x0676 - PCH Pm Slp S3 Min Assert SLP_S3 Minimum Assertion Width Policy.
Definition: FspsUpd.h:1644
UINT8 C1StateAutoDemotion
Offset 0x08A0 - Enable or Disable C1 Cstate Demotion Enable or Disable C1 Cstate Demotion.
Definition: FspsUpd.h:3083
UINT8 GtFreqMax
Offset 0x07BE - GT Frequency Limit 0xFF: Auto(Default), 2: 100 Mhz, 3: 150 Mhz, 4: 200 Mhz...
Definition: FspsUpd.h:2439
UINT8 PchPmSlpS4MinAssert
Offset 0x0677 - PCH Pm Slp S4 Min Assert SLP_S4 Minimum Assertion Width Policy.
Definition: FspsUpd.h:1649
UINT8 PmcModPhySusPgEnable
Offset 0x01FB - ModPHY SUS Power Domain Dynamic Gating Enable/Disable ModPHY SUS Power Domain Dynamic...
Definition: FspsUpd.h:677
UINT8 SkipPostBootSai
Offset 0x0A72 - Skip POSTBOOT SAI Deprecated $EN_DIS.
Definition: FspsUpd.h:3282
UINT8 EnableItbm
Offset 0x089E - Intel Turbo Boost Max Technology 3.0 Intel Turbo Boost Max Technology 3...
Definition: FspsUpd.h:3071
UINT8 PchPmSlpS0Vm075VSupport
Offset 0x0154 - SLP_S0 VM 0.75V Support SLP_S0 Voltage Margining 0.75V Support Policy.
Definition: FspsUpd.h:565
UINT8 PchHdaSndwBufferRcomp
Offset 0x0107 - Soundwire Clock Buffer GPIO RCOMP Setting 0: non-ACT - 50 Ohm driver impedance...
Definition: FspsUpd.h:395
UINT16 CstateLatencyControl3Irtl
Offset 0x085E - Interrupt Response Time Limit of C-State LatencyContol3 Interrupt Response Time Limit...
Definition: FspsUpd.h:2936
UINT32 GraphicsConfigPtr
Offset 0x0028 - Graphics Configuration Ptr Points to VBT.
Definition: FspsUpd.h:101
UINT8 PkgCStateLimit
Offset 0x0812 - Set the Max Pkg Cstate Set the Max Pkg Cstate.
Definition: FspsUpd.h:2828
UINT8 PchTTEnable
Offset 0x0713 - Enable The Thermal Throttle Enable the thermal throttle function. ...
Definition: FspsUpd.h:2033
UINT8 PchScsEmmcHs400TuningRequired
Offset 0x06F5 - Enable eMMC HS400 Training Deprecated.
Definition: FspsUpd.h:1920
UINT8 ManageabilityMode
Offset 0x0158 - Manageability Mode set by Mebx Enable/Disable.
Definition: FspsUpd.h:589
UINT8 SendVrMbxCmd
Offset 0x0303 - Enable VR specific mailbox command VR specific mailbox commands.
Definition: FspsUpd.h:1022
UINT8 CdClock
Offset 0x0217 - CdClock Frequency selection 0=337.5 Mhz, 1=450 Mhz, 2=540 Mhz, 3(Default)=675 Mhz 0: ...
Definition: FspsUpd.h:733
UINT8 XdciEnable
Offset 0x006C - Enable xDCI controller Enable/disable to xDCI controller.
Definition: FspsUpd.h:197
UINT8 Custom2PowerLimit1Time
Offset 0x07DF - Custom Short term Power Limit time window Short term Power Limit time window value fo...
Definition: FspsUpd.h:2582
UINT8 DisableD0I3SettingForHeci
Offset 0x08BC - D0I3 Setting for HECI Disable Test, 0: disable, 1: enable, Setting this option disabl...
Definition: FspsUpd.h:3145
UINT8 C1StateUnDemotion
Offset 0x08A1 - Enable or Disable C1 Cstate UnDemotion Enable or Disable C1 Cstate UnDemotion...
Definition: FspsUpd.h:3089
UINT8 DmiIot
Offset 0x07B5 - DMI IOT Control Enable: Enable DMI IOT Control, Disable(Default): Disable DMI IOT Con...
Definition: FspsUpd.h:2395
UINT8 RampDown
Offset 0x032A - Ramp Down Randomization time PCODE MMIO Mailbox: Acoustic Migitation Range...
Definition: FspsUpd.h:1133
UINT8 ConfigTdpBios
Offset 0x07E6 - Load Configurable TDP SSDT Configure whether to load Configurable TDP SSDT; 0: Disabl...
Definition: FspsUpd.h:2620
UINT8 PchDmiTsawEn
Offset 0x0718 - DMI Thermal Sensor Autonomous Width Enable DMI Thermal Sensor Autonomous Width Enable...
Definition: FspsUpd.h:2064
UINT32 Custom1PowerLimit1
Offset 0x0878 - Short term Power Limit value for custom cTDP level 1 Short term Power Limit value for...
Definition: FspsUpd.h:2982
UINT8 DmiExtSync
Offset 0x07B4 - DMI Extended Sync Control Enable: Enable DMI Extended Sync Control, Disable(Default): Disable DMI Extended Sync Control $EN_DIS.
Definition: FspsUpd.h:2389
UINT8 PchPmPciePllSsc
Offset 0x068B - PCH Pm Pcie Pll Ssc Specifies the Pcie Pll Spread Spectrum Percentage.
Definition: FspsUpd.h:1761
UINT8 PchLockDownGlobalSmi
Offset 0x08BF - Enable LOCKDOWN SMI Enable SMI_LOCK bit to prevent writes to the Global SMI Enable bi...
Definition: FspsUpd.h:3156
UINT8 FourCoreRatioLimit
Offset 0x07CE - 4-Core Ratio Limit 4-Core Ratio Limit: LFM to Fused, For overclocking part: LFM to 25...
Definition: FspsUpd.h:2477
UINT8 PeiGraphicsPeimInit
Offset 0x0218 - Enable/Disable PeiGraphicsPeimInit Enable: Enable PeiGraphicsPeimInit, Disable(Default): Disable PeiGraphicsPeimInit $EN_DIS.
Definition: FspsUpd.h:739
UINT32 MicrocodeRegionBase
Offset 0x0038 - MicrocodeRegionBase Memory Base of Microcode Updates.
Definition: FspsUpd.h:150
UINT8 PchPmDisableEnergyReport
Offset 0x0A5E - PCH Energy Reporting Disable/Enable PCH to CPU energy report feature.
Definition: FspsUpd.h:3259
UINT8 Custom1PowerLimit1Time
Offset 0x07DC - Custom Short term Power Limit time window Short term Power Limit time window value fo...
Definition: FspsUpd.h:2566
UINT8 SlpS0DisQForDebug
Offset 0x067B - S0ix Override Settings Select 'Auto', it will be auto-configured according to probe t...
Definition: FspsUpd.h:1680
UINT8 VoltageOptimization
Offset 0x07FF - Enable or Disable Voltage Optimization feature Enable or Disable Voltage Optimization...
Definition: FspsUpd.h:2711
UINT8 PchPmWoWlanEnable
Offset 0x0672 - PCH Pm WoW lan Enable Determine if WLAN wake from Sx, corresponds to the HOST_WLAN_PP...
Definition: FspsUpd.h:1620
UINT32 PcieRpDpcExtensionsMask
Offset 0x0110 - DPC Extensions PCIE RP Mask Enable/disable DPC Extensions for PCIE Root Ports...
Definition: FspsUpd.h:413
UINT8 PchXhciOcLock
Offset 0x0A60 - PCH USB OverCurrent mapping lock enable If this policy option is enabled then BIOS wi...
Definition: FspsUpd.h:3272
UINT8 PchPmDisableDsxAcPresentPulldown
Offset 0x0684 - PCH Pm Disable Dsx Ac Present Pulldown When Disable, PCH will internal pull down AC_P...
Definition: FspsUpd.h:1721
UINT8 McivrRfiFrequencyPrefix
Offset 0x030D - McIVR RFI Frequency Prefix PCODE MMIO Mailbox: McIVR RFI Frequency Adjustment Prefix...
Definition: FspsUpd.h:1049
UINT8 PchPmWolOvrWkSts
Offset 0x0689 - PCH Pm WOL_OVR_WK_STS Clear the WOL_OVR_WK_STS bit in the Power and Reset Status (PRS...
Definition: FspsUpd.h:1749
UINT8 DebugInterfaceLockEnable
Offset 0x07EF - Lock or Unlock debug interface features Lock or Unlock debug interface features; 0: D...
Definition: FspsUpd.h:2675
UINT8 PchHdaAudioLinkSndw2
Offset 0x0104 - Enable HD Audio SoundWire#2 Link Enable/disable HD Audio SNDW2 link.
Definition: FspsUpd.h:377
UINT8 SataPwrOptEnable
Offset 0x068D - PCH Sata Pwr Opt Enable SATA Power Optimizer on PCH side.
Definition: FspsUpd.h:1771
UINT8 SlowSlewRateForFivr
Offset 0x0314 - Slew Rate configuration for Deep Package C States for VR FIVR domain Slew Rate config...
Definition: FspsUpd.h:1088
UINT8 PchHdaAudioLinkSndw1
Offset 0x0103 - Enable HD Audio SoundWire#1 Link Enable/disable HD Audio SNDW1 link.
Definition: FspsUpd.h:371
UINT8 PchSirqMode
Offset 0x0709 - Serial IRQ Mode Select Serial IRQ Mode Select, 0: quiet mode, 1: continuous mode...
Definition: FspsUpd.h:1994
UINT8 PcieRpImrEnabled
Offset 0x066D - PCIE IMR Enables Isolated Memory Region for PCIe.
Definition: FspsUpd.h:1593
UINT8 SkipMpInit
Offset 0x030C - Deprecated DO NOT USE Skip Multi-Processor Initialization.
Definition: FspsUpd.h:1043
UINT8 PchIshUart0GpioAssign
Offset 0x0381 - Enable PCH ISH UART0 GPIO pins assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1272
UINT8 Custom1TurboActivationRatio
Offset 0x07DD - Custom Turbo Activation Ratio Turbo Activation Ratio for custom cTDP level 1...
Definition: FspsUpd.h:2571
UINT8 PowerLimit2
Offset 0x07D2 - Short Duration Turbo Mode Enable or Disable short duration Turbo Mode.
Definition: FspsUpd.h:2503
UINT8 SataRstHddUnlock
Offset 0x06E8 - PCH Sata Rst Hdd Unlock Indicates that the HDD password unlock in the OS is enabled...
Definition: FspsUpd.h:1880
UINT8 SataMode
Offset 0x0093 - SATA Mode Select SATA controller working mode.
Definition: FspsUpd.h:275
UINT8 PchHdaAudioLinkSsp0
Offset 0x0100 - Enable HD Audio SSP0 Link Enable/disable HD Audio SSP0/I2S link.
Definition: FspsUpd.h:353
UINT8 RaceToHalt
Offset 0x081C - Race To Halt Enable/Disable Race To Halt feature.
Definition: FspsUpd.h:2889
UINT8 SkipS3CdClockInit
Offset 0x0231 - Enable/Disable SkipS3CdClockInit Enable: Skip Full CD clock initializaton, Disable(Default): Initialize the full CD clock in S3 resume due to GOP absent $EN_DIS.
Definition: FspsUpd.h:821
UINT8 PchHdaAudioLinkSndw3
Offset 0x0105 - Enable HD Audio SoundWire#3 Link Enable/disable HD Audio SNDW3 link.
Definition: FspsUpd.h:383
UINT8 TStates
Offset 0x0803 - Enable or Disable T states Enable or Disable T states; 0: Disable; 1: Enable...
Definition: FspsUpd.h:2737
UINT8 ProcessorTraceOutputScheme
Offset 0x07F1 - Control on Processor Trace output scheme Control on Processor Trace output scheme; 0:...
Definition: FspsUpd.h:2687
Copyright (c) 2018, Intel Corporation.
UINT8 CstateLatencyControl2TimeUnit
Offset 0x0815 - TimeUnit for C-State Latency Control2 TimeUnit for C-State Latency Control2;Valid val...
Definition: FspsUpd.h:2846
UINT32 BltBufferSize
Offset 0x0238 - Blt Buffer Size Size of Blt Buffer, is equal to PixelWidth * PixelHeight * 4 bytes (t...
Definition: FspsUpd.h:839
UINT8 PchHotEnable
Offset 0x014F - PCHHOT# pin Enable PCHHOT# pin assertion when temperature is higher than PchHotLevel...
Definition: FspsUpd.h:534
UINT16 CstateLatencyControl5Irtl
Offset 0x0862 - Interrupt Response Time Limit of C-State LatencyContol5 Interrupt Response Time Limit...
Definition: FspsUpd.h:2946
UINT8 SevenCoreRatioLimit
Offset 0x089C - 7-Core Ratio Limit 7-Core Ratio Limit: LFM to Fused, For overclocking part: LFM to 25...
Definition: FspsUpd.h:3058
UINT8 TwoCoreRatioLimit
Offset 0x07CC - 2-Core Ratio Limit 2-Core Ratio Limit: LFM to Fused, For overclocking part: LFM to 25...
Definition: FspsUpd.h:2465
UINT8 PowerLimit3DutyCycle
Offset 0x07D5 - Package PL3 Duty Cycle Package PL3 Duty Cycle; Valid Range is 0 to 100...
Definition: FspsUpd.h:2519
UINT8 PchPmLanWakeFromDeepSx
Offset 0x0674 - PCH Pm Lan Wake From DeepSx Determine if enable LAN to wake from deep Sx...
Definition: FspsUpd.h:1633
UINT8 ConfigTdpLock
Offset 0x07E5 - ConfigTdp mode settings Lock Lock the ConfigTdp mode settings from runtime changes; 0...
Definition: FspsUpd.h:2614
UINT8 SataThermalSuggestedSetting
Offset 0x072A - Sata Thermal Throttling Suggested Setting Sata Thermal Throttling Suggested Setting...
Definition: FspsUpd.h:2162
UINT8 SgxSinitNvsData
Offset 0x079F - SgxSinitNvsData SgxSinitNvsData default values.
Definition: FspsUpd.h:2300
UINT8 ApIdleManner
Offset 0x07F0 - AP Idle Manner of waiting for SIPI AP Idle Manner of waiting for SIPI; 1: HALT loop; ...
Definition: FspsUpd.h:2681
UINT8 DmiTS0TW
Offset 0x071A - Thermal Sensor 0 Target Width DMT thermal sensor suggested representative values...
Definition: FspsUpd.h:2076
UINT8 MaxRatio
Offset 0x081D - Max P-State Ratio Max P-State Ratio, Valid Range 0 to 0x7F.
Definition: FspsUpd.h:2894
UINT8 MachineCheckEnable
Offset 0x07ED - Enable or Disable initialization of machine check registers Enable or Disable initial...
Definition: FspsUpd.h:2663
UINT32 Custom2PowerLimit2
Offset 0x0884 - Long term Power Limit value for custom cTDP level 2 Long term Power Limit value for c...
Definition: FspsUpd.h:3000
UINT8 SataEnable
Offset 0x0092 - Enable SATA Enable/disable SATA controller.
Definition: FspsUpd.h:269
UINT8 PchHdaIDispLinkTmode
Offset 0x036B - iDisp-Link T-mode iDisp-Link T-Mode (PCH_HDAUDIO_IDISP_TMODE enum): 0: 2T...
Definition: FspsUpd.h:1222
UINT8 PowerLimit4Lock
Offset 0x07D7 - Package PL4 Lock Package PL4 Lock Enable/Disable; 0: Disable ; 1: Enable $EN_DIS...
Definition: FspsUpd.h:2531
UINT8 PchPmLpcClockRun
Offset 0x0680 - PCH Pm Lpc Clock Run This member describes whether or not the LPC ClockRun feature of...
Definition: FspsUpd.h:1698
UINT32 ChipsetInitBinLen
Offset 0x0124 - Length of ChipsetInit Binary ChipsetInit Binary Length.
Definition: FspsUpd.h:462
UINT8 SataRstRaidDeviceId
Offset 0x06E0 - PCH Sata Rst Raid Device Id Enable RAID Alternate ID.
Definition: FspsUpd.h:1833
UINT8 AesEnable
Offset 0x0277 - Advanced Encryption Standard (AES) feature Enable or Disable Advanced Encryption Stan...
Definition: FspsUpd.h:857
UINT8 EightCoreRatioLimit
Offset 0x089D - 8-Core Ratio Limit 8-Core Ratio Limit: LFM to Fused, For overclocking part: LFM to 25...
Definition: FspsUpd.h:3065
UINT8 SerialIoUart0PinMuxing
Offset 0x0701 - PcdSerialIoUart0PinMuxing Select SerialIo Uart0 pin muxing.
Definition: FspsUpd.h:1960
UINT8 TccOffsetLock
Offset 0x07DA - Tcc Offset Lock Tcc Offset Lock for Runtime Average Temperature Limit (RATL) to lock ...
Definition: FspsUpd.h:2554
UINT8 PchScsEmmcHs400DriverStrength
Offset 0x06F9 - I/O Driver Strength Deprecated.
Definition: FspsUpd.h:1942
UINT8 NumOfDevIntConfig
Offset 0x007F - Number of DevIntConfig Entry Number of Device Interrupt Configuration Entry...
Definition: FspsUpd.h:220
UINT8 Hwp
Offset 0x07CF - Enable or Disable HWP Enable or Disable HWP(Hardware P states) Support.
Definition: FspsUpd.h:2484
UINT8 ProcHotLock
Offset 0x081A - Lock prochot configuration Lock prochot configuration Enable/Disable; 0: Disable; 1: ...
Definition: FspsUpd.h:2875
UINT8 DdiPortFHpd
Offset 0x022C - Enable or disable HPD of DDI port F 0=Disable, 1(Default)=Enable $EN_DIS.
Definition: FspsUpd.h:790
UINT8 PchIshPdtUnlock
Offset 0x038E - PCH ISH PDT Unlock Msg 0: False; 1: True.
Definition: FspsUpd.h:1350
UINT8 SataRstOromUiBanner
Offset 0x06E6 - PCH Sata Rst Orom Ui Banner OROM UI and BANNER.
Definition: FspsUpd.h:1869
UINT8 AutoThermalReporting
Offset 0x0808 - Enable or Disable Thermal Reporting Enable or Disable Thermal Reporting through ACPI ...
Definition: FspsUpd.h:2767
UINT8 ShowSpiController
Offset 0x0034 - Show SPI controller Enable/disable to show SPI controller.
Definition: FspsUpd.h:141
UINT8 DebugInterfaceEnable
Offset 0x0333 - Enable or Disable processor debug features Enable or Disable processor debug features...
Definition: FspsUpd.h:1149
UINT32 DevIntConfigPtr
Offset 0x007B - Address of PCH_DEVICE_INTERRUPT_CONFIG table.
Definition: FspsUpd.h:214
UINT8 DdiPortFDdc
Offset 0x0230 - Enable or disable DDC of DDI port F 0(Default)=Disable, 1=Enable $EN_DIS.
Definition: FspsUpd.h:814
UINT8 Heci3Enabled
Offset 0x014D - HECI3 state The HECI3 state from Mbp for reference in S3 path or when MbpHob is not i...
Definition: FspsUpd.h:524
UINT8 TccOffsetClamp
Offset 0x07D9 - Tcc Offset Clamp Enable/Disable Tcc Offset Clamp for Runtime Average Temperature Limi...
Definition: FspsUpd.h:2547
UINT8 Custom3TurboActivationRatio
Offset 0x07E3 - Custom Turbo Activation Ratio Turbo Activation Ratio for custom cTDP level 3...
Definition: FspsUpd.h:2603
UINT8 MonitorMwaitEnable
Offset 0x07EC - Enable or Disable Monitor /MWAIT instructions Enable or Disable Monitor /MWAIT instru...
Definition: FspsUpd.h:2657
UINT16 PchT0Level
Offset 0x070D - Thermal Throttling Custimized T0Level Value Custimized T0Level value.
Definition: FspsUpd.h:2017
UINT8 PchIshGp2GpioAssign
Offset 0x0388 - Enable PCH ISH GP_2 GPIO pin assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1314
UINT8 CstateLatencyControl5TimeUnit
Offset 0x0818 - TimeUnit for C-State Latency Control5 TimeUnit for C-State Latency Control5;Valid val...
Definition: FspsUpd.h:2863
UINT8 PsysOffset
Offset 0x02A1 - Platform Psys offset correction PCODE MMIO Mailbox: Platform Psys offset correction...
Definition: FspsUpd.h:917
UINT8 DdiPortBHpd
Offset 0x0229 - Enable or disable HPD of DDI port B 0=Disable, 1(Default)=Enable $EN_DIS.
Definition: FspsUpd.h:772
UINT8 PowerLimit3Lock
Offset 0x07D6 - Package PL3 Lock Package PL3 Lock Enable/Disable; 0: Disable ; 1: Enable $EN_DIS...
Definition: FspsUpd.h:2525
UINT8 DdiPortDDdc
Offset 0x022F - Enable or disable DDC of DDI port D 0=Disable, 1(Default)=Enable $EN_DIS.
Definition: FspsUpd.h:808
UINT8 SataRstOromUiDelay
Offset 0x06E7 - PCH Sata Rst Orom Ui Delay 00b: 2 secs; 01b: 4 secs; 10b: 6 secs; 11: 8 secs (see: PC...
Definition: FspsUpd.h:1874
UINT8 FiveCoreRatioLimit
Offset 0x089A - 5-Core Ratio Limit 5-Core Ratio Limit: LFM to Fused, For overclocking part: LFM to 25...
Definition: FspsUpd.h:3044
UINT8 TTSuggestedSetting
Offset 0x0716 - Thermal Throttling Suggested Setting Thermal Throttling Suggested Setting...
Definition: FspsUpd.h:2052
UINT8 PsysSlope
Offset 0x02A0 - Platform Psys slope correction PCODE MMIO Mailbox: Platform Psys slope correction...
Definition: FspsUpd.h:911
UINT8 DdiPortCHpd
Offset 0x022A - Enable or disable HPD of DDI port C 0=Disable, 1(Default)=Enable $EN_DIS.
Definition: FspsUpd.h:778
UINT8 PchIoApicEntry24_119
Offset 0x037D - Enable PCH Io Apic Entry 24-119 0: Disable; 1: Enable.
Definition: FspsUpd.h:1251
UINT8 CStatePreWake
Offset 0x080F - Enable or Disable CState-Pre wake Enable or Disable CState-Pre wake.
Definition: FspsUpd.h:2809
UINT8 FastPkgCRampDisableFivr
Offset 0x0313 - Disable Fast Slew Rate for Deep Package C States for VR FIVR domain Disable Fast Slew...
Definition: FspsUpd.h:1081
UINT8 PchPmSlpLanLowDc
Offset 0x0682 - PCH Pm Slp Lan Low Dc Enable/Disable SLP_LAN# Low on DC Power.
Definition: FspsUpd.h:1710
UINT8 CstCfgCtrIoMwaitRedirection
Offset 0x0811 - Enable or Disable IO to MWAIT redirection Enable or Disable IO to MWAIT redirection; ...
Definition: FspsUpd.h:2821
UINT8 PchHdaLinkFrequency
Offset 0x0369 - HD Audio Link Frequency HDA Link Freq (PCH_HDAUDIO_LINK_FREQUENCY enum): 0: 6MHz...
Definition: FspsUpd.h:1210
UINT8 PsysPowerLimit1
Offset 0x07E7 - PL1 Enable value PL1 Enable value to limit average platform power.
Definition: FspsUpd.h:2626
UINT8 ThermalMonitor
Offset 0x0809 - Enable or Disable Thermal Monitor Enable or Disable Thermal Monitor; 0: Disable; 1: E...
Definition: FspsUpd.h:2773
UINT8 DmiSuggestedSetting
Offset 0x0719 - DMI Thermal Sensor Suggested Setting DMT thermal sensor suggested representative valu...
Definition: FspsUpd.h:2070
UINT8 PchIshGp6GpioAssign
Offset 0x038C - Enable PCH ISH GP_6 GPIO pin assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1338
UINT8 PchHdaDspEnable
Offset 0x002D - Enable HD Audio DSP Enable/disable HD Audio DSP feature.
Definition: FspsUpd.h:113
UINT8 DmiTS1TW
Offset 0x071B - Thermal Sensor 1 Target Width Thermal Sensor 1 Target Width.
Definition: FspsUpd.h:2082
UINT8 AcousticNoiseMitigation
Offset 0x02A2 - Acoustic Noise Mitigation feature Enable or Disable Acoustic Noise Mitigation feature...
Definition: FspsUpd.h:925
UINT8 PchScsEmmcHs400TxDataDll
Offset 0x06F8 - Tx Data Delay Control Tx Data Delay Control 1 - Tx Data Delay (HS400 Mode)...
Definition: FspsUpd.h:1936
UINT8 ConfigTdpLevel
Offset 0x081B - Configuration for boot TDP selection Configuration for boot TDP selection; 0: TDP Nom...
Definition: FspsUpd.h:2881
UINT8 EcCmdProvisionEav
Offset 0x078D - EcCmdProvisionEav Ephemeral Authorization Value default values.
Definition: FspsUpd.h:2280
UINT32 BiosGuardAttr
Offset 0x0779 - BiosGuardAttr BiosGuardAttr default values.
Definition: FspsUpd.h:2263
UINT8 Device4Enable
Offset 0x002C - Enable Device 4 Enable/disable Device 4 $EN_DIS.
Definition: FspsUpd.h:107
UINT8 PchHdaAudioLinkHda
Offset 0x00FD - Enable HD Audio Link Enable/disable HD Audio Link.
Definition: FspsUpd.h:335
UINT8 PchTTState13Enable
Offset 0x0714 - PMSync State 13 When set to 1 and the programmed GPIO pin is a 1, then PMSync state 1...
Definition: FspsUpd.h:2040
UINT8 SlowSlewRateForGt
Offset 0x02A5 - Slew Rate configuration for Deep Package C States for VR GT domain Slew Rate configur...
Definition: FspsUpd.h:946
UINT8 PchUnlockGpioPads
Offset 0x08C1 - Unlock all GPIO pads Force all GPIO pads to be unlocked for debug purpose...
Definition: FspsUpd.h:3168
UINT8 Custom2TurboActivationRatio
Offset 0x07E0 - Custom Turbo Activation Ratio Turbo Activation Ratio for custom cTDP level 2...
Definition: FspsUpd.h:2587
UINT8 PchScsEmmcHs400DllDataValid
Offset 0x06F6 - Set HS400 Tuning Data Valid Set if HS400 Tuning Data Valid.
Definition: FspsUpd.h:1926
UINT8 CstateLatencyControl1TimeUnit
Offset 0x0814 - TimeUnit for C-State Latency Control1 TimeUnit for C-State Latency Control1;Valid val...
Definition: FspsUpd.h:2840
UINT8 PchHdaVcType
Offset 0x0368 - VC Type Virtual Channel Type Select: 0: VC0, 1: VC1.
Definition: FspsUpd.h:1204
UINT8 PchTsmicLock
Offset 0x070C - Thermal Device SMI Enable This locks down SMI Enable on Alert Thermal Sensor Trip...
Definition: FspsUpd.h:2012
UINT8 PowerLimit3Time
Offset 0x07D4 - Package PL3 time window Package PL3 time window range for this policy from 0 to 64ms...
Definition: FspsUpd.h:2514
UINT8 PchIshGp5GpioAssign
Offset 0x038B - Enable PCH ISH GP_5 GPIO pin assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1332
UINT8 PchHdaIDispCodecDisconnect
Offset 0x036D - iDisplay Audio Codec disconnection 0: Not disconnected, enumerable, 1: Disconnected SDI, not enumerable.
Definition: FspsUpd.h:1235
UINT16 WatchDogTimerOs
Offset 0x015B - OS Timer 16 bits Value, Set OS watchdog timer.
Definition: FspsUpd.h:608
UINT8 TetonGlacierSupport
Offset 0x0667 - Teton Glacier Support Enables support for the Teton Glacier card. ...
Definition: FspsUpd.h:1570
UINT8 PmgCstCfgCtrlLock
Offset 0x080B - Configure C-State Configuration Lock Configure C-State Configuration Lock; 0: Disable...
Definition: FspsUpd.h:2785
UINT8 PchIshGp1GpioAssign
Offset 0x0387 - Enable PCH ISH GP_1 GPIO pin assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1308
UINT8 PchHdaDspUaaCompliance
Offset 0x036C - Universal Audio Architecture compliance for DSP enabled system 0: Not-UAA Compliant (...
Definition: FspsUpd.h:1229
UINT8 HwpInterruptControl
Offset 0x0899 - Set HW P-State Interrupts Enabled for for MISC_PWR_MGMT Set HW P-State Interrupts Ena...
Definition: FspsUpd.h:3037
UINT8 DmiTS3TW
Offset 0x071D - Thermal Sensor 3 Target Width Thermal Sensor 3 Target Width.
Definition: FspsUpd.h:2094
UINT8 PowerLimit1Time
Offset 0x07D1 - Package Long duration turbo mode time Package Long duration turbo mode time window in...
Definition: FspsUpd.h:2497
UINT8 MlcSpatialPrefetcher
Offset 0x07EB - Enable or Disable MLC Spatial Prefetcher Enable or Disable MLC Spatial Prefetcher; 0:...
Definition: FspsUpd.h:2651
UINT8 TxtEnable
Offset 0x0305 - Enable or Disable TXT Enable or Disable TXT; 0: Disable; 1: Enable.
Definition: FspsUpd.h:1033
UINT8 Custom2ConfigTdpControl
Offset 0x07E1 - Custom Config Tdp Control Config Tdp Control (0/1/2) value for custom cTDP level 1...
Definition: FspsUpd.h:2592
UINT8 ScsSdCardEnabled
Offset 0x0033 - Enable SdCard Controller Enable/disable SD Card Controller.
Definition: FspsUpd.h:135
UINT8 EsataSpeedLimit
Offset 0x068E - PCH Sata eSATA Speed Limit When enabled, BIOS will configure the PxSCTL.SPD to 2 to limit the eSATA port speed.
Definition: FspsUpd.h:1777
UINT16 DeltaT12PowerCycleDelay
Offset 0x0232 - Delta T12 Power Cycle Delay required in ms Select the value for delay required...
Definition: FspsUpd.h:828
UINT8 PchPwrOptEnable
Offset 0x0347 - Enable Power Optimizer Enable DMI Power Optimizer on PCH side.
Definition: FspsUpd.h:1167
UINT32 PchPcieDeviceOverrideTablePtr
Offset 0x0754 - Pch PCIE device override table pointer The PCIe device table is being used to overrid...
Definition: FspsUpd.h:2245
UINT8 McivrRfiFrequencyAdjust
Offset 0x030E - McIVR RFI Frequency Adjustment PCODE MMIO Mailbox: Adjust the RFI frequency relative ...
Definition: FspsUpd.h:1055
UINT8 HdcControl
Offset 0x07D0 - Hardware Duty Cycle Control Hardware Duty Cycle Control configuration.
Definition: FspsUpd.h:2490
UINT8 Custom3PowerLimit1Time
Offset 0x07E2 - Custom Short term Power Limit time window Short term Power Limit time window value fo...
Definition: FspsUpd.h:2598
UINT8 PchPmPcieWakeFromDeepSx
Offset 0x0671 - PCH Pm Pcie Wake From DeepSx Determine if enable PCIe to wake from deep Sx...
Definition: FspsUpd.h:1614
UINT32 PsysPowerLimit1Power
Offset 0x0890 - Platform PL1 power Platform PL1 power.
Definition: FspsUpd.h:3018
UINT8 PchEnableComplianceMode
Offset 0x0734 - Enable xHCI Compliance Mode Compliance Mode can be enabled for testing through this o...
Definition: FspsUpd.h:2195
UINT8 SataSpeedLimit
Offset 0x068F - PCH Sata Speed Limit Indicates the maximum speed the SATA controller can support 0h: ...
Definition: FspsUpd.h:1782
UINT32 MicrocodeRegionSize
Offset 0x003C - MicrocodeRegionSize Size of Microcode Updates.
Definition: FspsUpd.h:155
UINT32 ChipsetInitBinPtr
Offset 0x0120 - Pointer of ChipsetInit Binary ChipsetInit Binary Pointer.
Definition: FspsUpd.h:457
UINT8 SataRstLegacyOrom
Offset 0x011A - PCH SATA use RST Legacy OROM Use PCH SATA RST Legacy OROM when CSM is Enabled $EN_DIS...
Definition: FspsUpd.h:438
UINT8 PchIshGp3GpioAssign
Offset 0x0389 - Enable PCH ISH GP_3 GPIO pin assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1320
UINT8 SataRstSmartStorage
Offset 0x06EB - PCH Sata Rst Smart Storage RST Smart Storage caching Bit.
Definition: FspsUpd.h:1899
UINT8 TurboPowerLimitLock
Offset 0x07D3 - Turbo settings Lock Lock all Turbo settings Enable/Disable; 0: Disable ...
Definition: FspsUpd.h:2509
UINT8 PchIshI2c2GpioAssign
Offset 0x0385 - Enable PCH ISH I2C2 GPIO pins assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1296
UINT8 DdiPortBDdc
Offset 0x022D - Enable or disable DDC of DDI port B 0=Disable, 1(Default)=Enable $EN_DIS.
Definition: FspsUpd.h:796
UINT32 * Data
Pointer to the data buffer. Its length is specified in the header.
Definition: FspsUpd.h:58
UINT8 PchIoApicId
Offset 0x037E - PCH Io Apic ID This member determines IOAPIC ID.
Definition: FspsUpd.h:1256
UINT8 PchPmSlpS0Vm070VSupport
Offset 0x0153 - SLP_S0 VM 0.70V Support SLP_S0 Voltage Margining 0.70V Support Policy.
Definition: FspsUpd.h:559
UINT8 Cx
Offset 0x080A - Enable or Disable CPU power states (C-states) Enable or Disable CPU power states (C-s...
Definition: FspsUpd.h:2779
UINT8 SataP1TDispFinit
Offset 0x0729 - Port 1 Alternate Fast Init Tdispatch Port 1 Alternate Fast Init Tdispatch.
Definition: FspsUpd.h:2156
UINT8 PavpEnable
Offset 0x0216 - Enable/Disable PavpEnable Enable(Default): Enable PavpEnable, Disable: Disable PavpEn...
Definition: FspsUpd.h:727
UINT8 SlpS0WithGbeSupport
Offset 0x01FC - SlpS0WithGbeSupport Enable/Disable SLP_S0 with GBE Support.
Definition: FspsUpd.h:683
UINT8 RampUp
Offset 0x0329 - Ramp Up Randomization time PCODE MMIO Mailbox: Acoustic Migitation Range...
Definition: FspsUpd.h:1126
UINT8 FastPkgCRampDisableGt
Offset 0x0301 - Disable Fast Slew Rate for Deep Package C States for VR GT domain Disable Fast Slew R...
Definition: FspsUpd.h:1007
UINT8 PchLanLtrEnable
Offset 0x038F - Enable PCH Lan LTR capabilty of PCH internal LAN 0: Disable; 1: Enable.
Definition: FspsUpd.h:1356
UINT8 PmSupport
Offset 0x07BB - Enable/Disable IGFX PmSupport Enable(Default): Enable IGFX PmSupport, Disable: Disable IGFX PmSupport $EN_DIS.
Definition: FspsUpd.h:2413
UINT8 PchUsbOverCurrentEnable
Offset 0x0149 - PCH USB OverCurrent mapping enable 1: Will program USB OC pin mapping in xHCI control...
Definition: FspsUpd.h:501
UINT8 PchHdaAudioLinkDmic0
Offset 0x00FE - Enable HD Audio DMIC0 Link Enable/disable HD Audio DMIC0 link.
Definition: FspsUpd.h:341
UINT8 PchSirqEnable
Offset 0x0708 - Enable Serial IRQ Determines if enable Serial IRQ.
Definition: FspsUpd.h:1988
UINT32 TraceHubMemBase
Offset 0x011B - Trace Hub Memory Base If Trace Hub is enabled and trace to memory is desired...
Definition: FspsUpd.h:445
UINT8 DdiPortDHpd
Offset 0x022B - Enable or disable HPD of DDI port D 0=Disable, 1(Default)=Enable $EN_DIS.
Definition: FspsUpd.h:784
UINT8 PchIshI2c0GpioAssign
Offset 0x0383 - Enable PCH ISH I2C0 GPIO pins assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1284
UINT8 McivrSpreadSpectrum
Offset 0x0311 - McIVR RFI Spread Spectrum PCODE MMIO Mailbox: McIVR RFI Spread Spectrum.
Definition: FspsUpd.h:1068
UINT8 PchPciePort8xhDecodePortIndex
Offset 0x0A5D - PCIE Port8xh Decode Port Index The Index of PCIe Port that is selected for Port8xh De...
Definition: FspsUpd.h:3253
UINT8 PchCnviMode
Offset 0x0146 - CNVi Configuration This option allows for automatic detection of Connectivity Solutio...
Definition: FspsUpd.h:481
UINT8 SataTestMode
Offset 0x0A5F - PCH Sata Test Mode Allow entrance to the PCH SATA test modes.
Definition: FspsUpd.h:3265
UINT8 CstateLatencyControl4TimeUnit
Offset 0x0817 - TimeUnit for C-State Latency Control4 Time - 1ns , 1 - 32ns , 2 - 1024ns ...
Definition: FspsUpd.h:2857
UINT32 LogoPtr
Offset 0x0020 - Logo Pointer Points to PEI Display Logo Image.
Definition: FspsUpd.h:91
UINT8 DmiAspm
Offset 0x0201 - DMI ASPM 0=Disable, 1:L0s, 2:L1, 3(Default)=L0sL1 0:Disable, 1:L0s, 2:L1, 3:L0sL1.
Definition: FspsUpd.h:699
UINT8 DisableTurboGt
Offset 0x07BF - Disable Turbo GT 0=Disable: GT frequency is not limited, 1=Enable: Disables Turbo GT ...
Definition: FspsUpd.h:2445
UINT8 SgxSinitDataFromTpm
Offset 0x08BA - SgxSinitDataFromTpm SgxSinitDataFromTpm default values.
Definition: FspsUpd.h:3131
UINT64 ProcessorTraceMemBase
Offset 0x07F3 - Base of memory region allocated for Processor Trace Base address of memory region all...
Definition: FspsUpd.h:2699
UINT8 PchHdaVerbTableEntryNum
Offset 0x008C - PCH HDA Verb Table Entry Number Number of Entries in Verb Table.
Definition: FspsUpd.h:253
UINT8 PchSbAccessUnlock
Offset 0x08C3 - PCH Unlock SideBand access The SideBand PortID mask for certain end point (e...
Definition: FspsUpd.h:3181
UINT8 MaxRingRatioLimit
Offset 0x08A4 - Minimum Ring ratio limit override Maximum Ring ratio limit override.
Definition: FspsUpd.h:3108
UINT8 Eist
Offset 0x0800 - Enable or Disable Intel SpeedStep Technology Enable or Disable Intel SpeedStep Techno...
Definition: FspsUpd.h:2717
UINT8 PchPmDisableNativePowerButton
Offset 0x0686 - PCH Pm Disable Native Power Button Power button native mode disable.
Definition: FspsUpd.h:1731
UINT8 SataRstIrrtOnly
Offset 0x06EA - PCH Sata Rst Irrt Only Allow only IRRT drives to span internal and external ports...
Definition: FspsUpd.h:1893
UINT32 TccOffsetTimeWindowForRatl
Offset 0x0874 - Tcc Offset Time Window for RATL Package PL4 power limit.
Definition: FspsUpd.h:2976
UINT16 PsysPmax
Offset 0x0856 - Platform Power Pmax PCODE MMIO Mailbox: Platform Power Pmax.
Definition: FspsUpd.h:2916
UINT8 PchHdaAudioLinkSsp1
Offset 0x0101 - Enable HD Audio SSP1 Link Enable/disable HD Audio SSP1/I2S link.
Definition: FspsUpd.h:359
UINT8 ProcHotResponse
Offset 0x0806 - Enable or Disable PROCHOT# Response Enable or Disable PROCHOT# Response; 0: Disable; ...
Definition: FspsUpd.h:2755
UINT8 PchPmPmeB0S5Dis
Offset 0x0669 - PCH Pm PME_B0_S5_DIS When cleared (default), wake events from PME_B0_STS are allowed ...
Definition: FspsUpd.h:1582
UINT8 PcieRpImrSelection
Offset 0x066E - PCIE IMR port number Selects PCIE root port number for IMR feature.
Definition: FspsUpd.h:1598
UINT8 SataRstLedLocate
Offset 0x06E9 - PCH Sata Rst Led Locate Indicates that the LED/SGPIO hardware is attached and ping to...
Definition: FspsUpd.h:1887
UINT8 DdiPortEdp
Offset 0x0228 - Enable or disable eDP device 0=Disable, 1(Default)=Enable $EN_DIS.
Definition: FspsUpd.h:766
UINT8 PchEnableDbcObs
Offset 0x067C - USB Overcurrent Override for DbC This option overrides USB Over Current enablement st...
Definition: FspsUpd.h:1687
UINT32 PowerLimit2Power
Offset 0x0868 - Package Short duration turbo mode power limit Package Short duration turbo mode power...
Definition: FspsUpd.h:2958
UINT8 PchIshUart1GpioAssign
Offset 0x0382 - Enable PCH ISH UART1 GPIO pins assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1278
UINT8 C3StateUnDemotion
Offset 0x08A6 - Enable or Disable C3 Cstate UnDemotion Enable or Disable C3 Cstate UnDemotion...
Definition: FspsUpd.h:3120
UINT8 PchIshGp4GpioAssign
Offset 0x038A - Enable PCH ISH GP_4 GPIO pin assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1326
UINT8 PchIshSpiGpioAssign
Offset 0x0380 - Enable PCH ISH SPI GPIO pins assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1266
UINT8 PreWake
Offset 0x0328 - Pre Wake Randomization time PCODE MMIO Mailbox: Acoustic Migitation Range...
Definition: FspsUpd.h:1119
UINT8 EnableItbmDriver
Offset 0x089F - Intel Turbo Boost Max Technology 3.0 Driver Intel Turbo Boost Max Technology 3...
Definition: FspsUpd.h:3077
UINT32 PowerLimit3
Offset 0x086C - Package PL3 power limit Package PL3 power limit.
Definition: FspsUpd.h:2964
UINT8 TetonGlacierCR
Offset 0x0668 - Teton Glacier Cycle Router Specify to which cycle router Teton Glacier is connected...
Definition: FspsUpd.h:1576
UINT8 DisableVrThermalAlert
Offset 0x0807 - Enable or Disable VR Thermal Alert Enable or Disable VR Thermal Alert; 0: Disable; 1:...
Definition: FspsUpd.h:2761
UINT8 CpuWakeUpTimer
Offset 0x08A2 - CpuWakeUpTimer Enable long CPU Wakeup Timer.
Definition: FspsUpd.h:3096
UINT8 DmiTS2TW
Offset 0x071C - Thermal Sensor 2 Target Width Thermal Sensor 2 Target Width.
Definition: FspsUpd.h:2088
UINT8 PcieEnablePort8xhDecode
Offset 0x0A5C - PCIE RP Enable Port8xh Decode This member describes whether PCIE root port Port 8xh D...
Definition: FspsUpd.h:3248
UINT8 PchHdaCodecSxWakeCapability
Offset 0x0091 - PCH HDA Codec Sx Wake Capability Capability to detect wake initiated by a codec in Sx...
Definition: FspsUpd.h:263
UINT8 PchPmSlpSusMinAssert
Offset 0x0678 - PCH Pm Slp Sus Min Assert SLP_SUS Minimum Assertion Width Policy. ...
Definition: FspsUpd.h:1654
UINT8 PchEspiBmeMasterSlaveEnabled
Offset 0x0119 - PCH eSPI Master and Slave BME enabled PCH eSPI Master and Slave BME enabled $EN_DIS...
Definition: FspsUpd.h:432
UINT8 PkgCStateDemotion
Offset 0x080D - Enable or Disable Package Cstate Demotion Enable or Disable Package Cstate Demotion...
Definition: FspsUpd.h:2797
UINT8 PmcDbgMsgEn
Offset 0x011F - PMC Debug Message Enable When Enabled, PMC HW will send debug messages to trace hub; ...
Definition: FspsUpd.h:452
UINT8 GnaEnable
Offset 0x021A - Enable or disable GNA device 0=Disable, 1(Default)=Enable $EN_DIS.
Definition: FspsUpd.h:749
UINT8 C3StateAutoDemotion
Offset 0x08A5 - Enable or Disable C3 Cstate Demotion Enable or Disable C3 Cstate Demotion.
Definition: FspsUpd.h:3114
UINT32 Custom2PowerLimit1
Offset 0x0880 - Short term Power Limit value for custom cTDP level 2 Short term Power Limit value for...
Definition: FspsUpd.h:2994
UINT8 SerialIoDebugUartNumber
Offset 0x0706 - UART Number For Debug Purpose UART number for debug purpose.
Definition: FspsUpd.h:1976
UINT8 PchSbiUnlock
Offset 0x08C2 - PCH Unlock SBI access Deprecated $EN_DIS.
Definition: FspsUpd.h:3174
UINT8 PchPmPwrBtnOverridePeriod
Offset 0x0683 - PCH Pm Pwr Btn Override Period PCH power button override period.
Definition: FspsUpd.h:1715
UINT32 PowerLimit1
Offset 0x0864 - Package Long duration turbo mode power limit Package Long duration turbo mode power l...
Definition: FspsUpd.h:2952
UINT8 PchPmSlpS0Enable
Offset 0x0687 - PCH Pm Slp S0 Enable Indicates whether SLP_S0# is to be asserted when PCH reaches idl...
Definition: FspsUpd.h:1737
UINT8 Enable8254ClockGating
Offset 0x074F - Enable 8254 Static Clock Gating Set 8254CGE=1 is required for SLP_S0 support...
Definition: FspsUpd.h:2213
UINT8 PcieComplianceTestMode
Offset 0x0665 - PCIE Compliance Test Mode Compliance Test Mode shall be enabled when using Compliance...
Definition: FspsUpd.h:1557
UINT8 X2ApicOptOut
Offset 0x021B - State of X2APIC_OPT_OUT bit in the DMAR table 0=Disable/Clear, 1=Enable/Set $EN_DIS...
Definition: FspsUpd.h:755
UINT8 ChapDeviceEnable
Offset 0x07B1 - Enable/Disable Device 7 Enable: Device 7 enabled, Disable (Default): Device 7 disable...
Definition: FspsUpd.h:2368
UINT8 EndOfPostMessage
Offset 0x08BB - End of Post message Test, Send End of Post message.
Definition: FspsUpd.h:3138
UINT8 PchHdaAudioLinkSsp2
Offset 0x0102 - Enable HD Audio SSP2 Link Enable/disable HD Audio SSP2/I2S link.
Definition: FspsUpd.h:365
UINT8 PcieDisableRootPortClockGating
Offset 0x0662 - PCIE Disable RootPort Clock Gating Describes whether the PCI Express Clock Gating for...
Definition: FspsUpd.h:1541
UINT16 PchT1Level
Offset 0x070F - Thermal Throttling Custimized T1Level Value Custimized T1Level value.
Definition: FspsUpd.h:2022
The PCH_DEVICE_INTERRUPT_CONFIG block describes interrupt pin, IRQ and interrupt mode for PCH device...
Definition: FspsUpd.h:74
UINT8 PchPmSlpAMinAssert
Offset 0x0679 - PCH Pm Slp A Min Assert SLP_A Minimum Assertion Width Policy.
Definition: FspsUpd.h:1659
UINT8 DebugInterfaceEnable
Offset 0x07EE - Deprecated DO NOT USE Enable or Disable processor debug features. ...
Definition: FspsUpd.h:2669
UINT8 MctpBroadcastCycle
Offset 0x0A73 - Mctp Broadcast Cycle Test, Determine if MCTP Broadcast is enabled 0: Disable; 1: Enab...
Definition: FspsUpd.h:3288
UINT32 VrPowerDeliveryDesign
Offset 0x0324 - CPU VR Power Delivery Design Used to communicate the power delivery design capability...
Definition: FspsUpd.h:1112
UINT8 FastPkgCRampDisableIa
Offset 0x02A3 - Disable Fast Slew Rate for Deep Package C States for VR IA domain Disable Fast Slew R...
Definition: FspsUpd.h:932
UINT8 SkipPamLock
Offset 0x07B2 - Skip PAM register lock Enable: PAM register will not be locked by RC...
Definition: FspsUpd.h:2375
UINT8 VtdDisable
Offset 0x07BD - Disable VT-d 0=Enable/FALSE(VT-d enabled), 1=Disable/TRUE (VT-d disabled) $EN_DIS...
Definition: FspsUpd.h:2425
UINT8 SataRstOptaneMemory
Offset 0x0750 - PCH Sata Rst Optane Memory Optane Memory $EN_DIS.
Definition: FspsUpd.h:2219
UINT8 PchHdaAudioLinkSndw4
Offset 0x0106 - Enable HD Audio SoundWire#4 Link Enable/disable HD Audio SNDW4 link.
Definition: FspsUpd.h:389
UINT8 FivrSpreadSpectrum
Offset 0x0312 - FIVR RFI Spread Spectrum PCODE MMIO Mailbox: FIVR RFI Spread Spectrum, in 0.1% increments.
Definition: FspsUpd.h:1074
UINT8 PchStartFramePulse
Offset 0x070A - Start Frame Pulse Width Start Frame Pulse Width, 0: PchSfpw4Clk, 1: PchSfpw6Clk...
Definition: FspsUpd.h:2000
UINT8 RenderStandby
Offset 0x07BA - Enable/Disable IGFX RenderStandby Enable(Default): Enable IGFX RenderStandby, Disable: Disable IGFX RenderStandby $EN_DIS.
Definition: FspsUpd.h:2407
UINT8 PcieRpFunctionSwap
Offset 0x0666 - PCIE Rp Function Swap Allows BIOS to use root port function number swapping when root...
Definition: FspsUpd.h:1564
UINT8 PcieEnablePeerMemoryWrite
Offset 0x0663 - PCIE Enable Peer Memory Write This member describes whether Peer Memory Writes are en...
Definition: FspsUpd.h:1547
UINT8 ScsUfsEnabled
Offset 0x0145 - Enable Ufs Controller Enable/disable Ufs 2.0 Controller.
Definition: FspsUpd.h:474
UINT32 Custom3PowerLimit1
Offset 0x0888 - Short term Power Limit value for custom cTDP level 3 Short term Power Limit value for...
Definition: FspsUpd.h:3006
UINT8 SataRstIrrt
Offset 0x06E5 - PCH Sata Rst Irrt Intel Rapid Recovery Technology.
Definition: FspsUpd.h:1863
UINT8 PchIshI2c1GpioAssign
Offset 0x0384 - Enable PCH ISH I2C1 GPIO pins assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1290
UINT8 TccActivationOffset
Offset 0x07D8 - TCC Activation Offset TCC Activation Offset.
Definition: FspsUpd.h:2539
UINT8 Custom3ConfigTdpControl
Offset 0x07E4 - Custom Config Tdp Control Config Tdp Control (0/1/2) value for custom cTDP level 1...
Definition: FspsUpd.h:2608
UINT16 PchTemperatureHotLevel
Offset 0x0732 - Thermal Device Temperature Decides the temperature.
Definition: FspsUpd.h:2188
UINT8 SlowSlewRateForSa
Offset 0x02A6 - Slew Rate configuration for Deep Package C States for VR SA domain Slew Rate configur...
Definition: FspsUpd.h:953
UINT16 CstateLatencyControl0Irtl
Offset 0x0858 - Interrupt Response Time Limit of C-State LatencyContol0 Interrupt Response Time Limit...
Definition: FspsUpd.h:2921
UINT8 DisableProcHotOut
Offset 0x0805 - Enable or Disable PROCHOT# signal being driven externally Enable or Disable PROCHOT# ...
Definition: FspsUpd.h:2749
UINT8 PchPmMeWakeSts
Offset 0x0688 - PCH Pm ME_WAKE_STS Clear the ME_WAKE_STS bit in the Power and Reset Status (PRSTS) re...
Definition: FspsUpd.h:1743
UINT8 MlcStreamerPrefetcher
Offset 0x07EA - Enable or Disable MLC Streamer Prefetcher Enable or Disable MLC Streamer Prefetcher; ...
Definition: FspsUpd.h:2645
UINT8 Enable8254ClockGatingOnS3
Offset 0x0752 - Enable 8254 Static Clock Gating On S3 This is only applicable when Enable8254ClockGat...
Definition: FspsUpd.h:2233
UINT32 PcieRpPtmMask
Offset 0x0108 - PTM for PCIE RP Mask Enable/disable Precision Time Measurement for PCIE Root Ports...
Definition: FspsUpd.h:401
UINT8 ScsEmmcHs400Enabled
Offset 0x0032 - Enable eMMC HS400 Mode Enable eMMC HS400 Mode.
Definition: FspsUpd.h:129
UINT8 ThreeStrikeCounterDisable
Offset 0x0898 - Set Three Strike Counter Disable False (default): Three Strike counter will be increm...
Definition: FspsUpd.h:3031
UINT8 CstateLatencyControl0TimeUnit
Offset 0x0813 - TimeUnit for C-State Latency Control0 TimeUnit for C-State Latency Control0; Valid va...
Definition: FspsUpd.h:2834
UINT8 ScsEmmcEnabled
Offset 0x0031 - Enable eMMC Controller Enable/disable eMMC Controller.
Definition: FspsUpd.h:123
UINT8 PchPmSlpS0VmRuntimeControl
Offset 0x0152 - SLP_S0 VM Dynamic Control SLP_S0 Voltage Margining Runtime Control Policy...
Definition: FspsUpd.h:553
Generated on Wed Aug 22 2018 17:48:55 for CoffeeLake Intel(R) Firmware Support Package (FSP) Integration Guide by
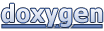