Sound Lib
C/C++Windowsゲーム用音声再生ライブラリ
|
サウンド統括クラス [詳解]
#include <SoundsManager.h>
公開メンバ関数 | |
SoundsManagerTmpl () | |
コンストラクタ [詳解] | |
SoundsManagerTmpl (SoundsManagerTmpl< T > &&obj)=default | |
ムーブコンストラクタ [詳解] | |
~SoundsManagerTmpl () | |
デストラクタ [詳解] | |
PlayingStatus | GetStatus (const T *pKey) const |
再生状況を示すステータスを取得する。 [詳解] | |
uint8_t | GetVolume (const T *pKey) const |
ボリュームを取得する。 [詳解] | |
bool | SetVolume (const T *pKey, uint8_t volume) |
ボリュームを設定する。 [詳解] | |
float | GetFrequencyRatio (const T *pKey) const |
再生速度とピッチの変化率を取得する。 [詳解] | |
bool | SetFrequencyRatio (const T *pKey, float ratio) |
再生速度とピッチの変化率を設定する。 [詳解] | |
SoundsManagerTmpl< T > & | operator= (SoundsManagerTmpl< T > &&obj)=default |
ムーブ代入演算子のオーバーロード [詳解] | |
bool | Initialize () |
XAudio2の初期化処理を行う。 [詳解] | |
bool | AddFile (const T *pFilePath, const T *pKey) |
音声ファイルを追加する。 [詳解] | |
bool | Start (const T *pKey, bool isLoopPlayback=false) |
ファイルの先頭から再生を行う。 [詳解] | |
bool | Start (const T *pKey, ISoundsManagerDelegate< T > *pDelegate) |
ファイルの先頭から再生を行う。 [詳解] | |
bool | Start (const T *pKey, void(*onPlayedToEndCallback)(const T *pKey)) |
ファイルの先頭から再生を行う。 [詳解] | |
bool | Stop (const T *pKey) |
再生を停止する。 [詳解] | |
bool | Pause (const T *pKey) |
再生を一時停止する。 [詳解] | |
bool | Resume (const T *pKey) |
一時停止中の音声を続きから再生する。 [詳解] | |
詳解
template<typename T>
class SoundLib::SoundsManagerTmpl< T >
サウンド統括クラス
構築子と解体子
◆ SoundsManagerTmpl() [1/2]
template<typename T >
SoundLib::SoundsManagerTmpl< T >::SoundsManagerTmpl | ( | ) |
コンストラクタ
◆ SoundsManagerTmpl() [2/2]
template<typename T >
|
default |
ムーブコンストラクタ
- 引数
-
obj ムーブ対象オブジェクト
◆ ~SoundsManagerTmpl()
template<typename T >
SoundLib::SoundsManagerTmpl< T >::~SoundsManagerTmpl | ( | ) |
デストラクタ
関数詳解
◆ AddFile()
template<typename T >
bool SoundLib::SoundsManagerTmpl< T >::AddFile | ( | const T * | pFilePath, |
const T * | pKey | ||
) |
音声ファイルを追加する。
- 引数
-
pFilePath ファイルパス pKey 音声ファイルを識別するキー
- 戻り値
- 成否
◆ GetFrequencyRatio()
template<typename T >
float SoundLib::SoundsManagerTmpl< T >::GetFrequencyRatio | ( | const T * | pKey | ) | const |
再生速度とピッチの変化率を取得する。
- 引数
-
pKey 音声ファイルを識別するキー
- 戻り値
- 音源からの変化率
1.0の場合、音源から変化なし。 2.0の場合、再生速度2倍で1オクターブ高音。 0.5の場合、再生速度1/2で1オクターブ低音。
- 参照
- IXAudio2SourceVoice::GetFrequencyRatio()
◆ GetStatus()
template<typename T >
PlayingStatus SoundLib::SoundsManagerTmpl< T >::GetStatus | ( | const T * | pKey | ) | const |
再生状況を示すステータスを取得する。
- 引数
-
pKey 音声ファイルを識別するキー
- 戻り値
- 再生ステータス
◆ GetVolume()
template<typename T >
uint8_t SoundLib::SoundsManagerTmpl< T >::GetVolume | ( | const T * | pKey | ) | const |
ボリュームを取得する。
- 引数
-
pKey 音声ファイルを識別するキー
- 戻り値
- ボリューム(0:無音 100:音源ボリューム)
◆ Initialize()
template<typename T >
bool SoundLib::SoundsManagerTmpl< T >::Initialize | ( | ) |
XAudio2の初期化処理を行う。
- 戻り値
- 成否
ライブラリ使用時はまず当メソッドを呼び出して下さい。
◆ operator=()
template<typename T >
|
default |
ムーブ代入演算子のオーバーロード
- 引数
-
obj ムーブ対象オブジェクト
- 戻り値
- ムーブ後のオブジェクト
◆ Pause()
template<typename T >
bool SoundLib::SoundsManagerTmpl< T >::Pause | ( | const T * | pKey | ) |
◆ Resume()
template<typename T >
bool SoundLib::SoundsManagerTmpl< T >::Resume | ( | const T * | pKey | ) |
◆ SetFrequencyRatio()
template<typename T >
bool SoundLib::SoundsManagerTmpl< T >::SetFrequencyRatio | ( | const T * | pKey, |
float | ratio | ||
) |
再生速度とピッチの変化率を設定する。
- 引数
-
pKey 音声ファイルを識別するキー ratio 音源からの変化率
- 戻り値
- 成否
1.0の場合、音源から変化なし。 2.0の場合、再生速度2倍で1オクターブ高音。 0.5の場合、再生速度1/2で1オクターブ低音。
設定可能最大値はAudioHandler::MAX_FREQENCY_RATIO。
- 参照
- IXAudio2SourceVoice::SetFrequencyRatio(float, UINT32)
◆ SetVolume()
template<typename T >
bool SoundLib::SoundsManagerTmpl< T >::SetVolume | ( | const T * | pKey, |
uint8_t | volume | ||
) |
ボリュームを設定する。
- 引数
-
pKey 音声ファイルを識別するキー volume ボリューム(0:無音 100:音源ボリューム)
- 戻り値
- 成否
◆ Start() [1/3]
template<typename T >
bool SoundLib::SoundsManagerTmpl< T >::Start | ( | const T * | pKey, |
bool | isLoopPlayback = false |
||
) |
ファイルの先頭から再生を行う。
- 引数
-
pKey 音声ファイルを識別するキー isLoopPlayback ループ再生を行うかどうか
- 戻り値
- 成否
◆ Start() [2/3]
template<typename T >
bool SoundLib::SoundsManagerTmpl< T >::Start | ( | const T * | pKey, |
ISoundsManagerDelegate< T > * | pDelegate | ||
) |
ファイルの先頭から再生を行う。
- 引数
-
pKey 音声ファイルを識別するキー pDelegate 最後まで再生完了後に呼び出すコールバック関数を定義したオブジェクト
- 戻り値
- 成否
C++から利用する場合用
◆ Start() [3/3]
template<typename T >
bool SoundLib::SoundsManagerTmpl< T >::Start | ( | const T * | pKey, |
void(*)(const T *pKey) | onPlayedToEndCallback | ||
) |
ファイルの先頭から再生を行う。
- 引数
-
pKey 音声ファイルを識別するキー onPlayedToEndCallback 最後まで再生完了後に呼び出すコールバック関数
- 戻り値
- 成否
C言語から利用する場合用
◆ Stop()
template<typename T >
bool SoundLib::SoundsManagerTmpl< T >::Stop | ( | const T * | pKey | ) |
このクラス詳解は次のファイルから抽出されました:
- SoundLib/SoundsManager.h
- SoundLib/SoundsManager.cpp
構築:
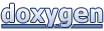