Sound Lib
C/C++Windowsゲーム用音声再生ライブラリ
|
SoundsManager.h
30 /* Constructor / Destructor ------------------------------------------------------------------------- */
47 /* Getters / Setters -------------------------------------------------------------------------------- */
100 /* Operator Overloads ------------------------------------------------------------------------------- */
108 /* Functions ---------------------------------------------------------------------------------------- */
175 /* Variables ---------------------------------------------------------------------------------------- */
179 /* Constructor / Destructor ------------------------------------------------------------------------- */
182 /* Operator Overloads ------------------------------------------------------------------------------- */
185 /* Functions ---------------------------------------------------------------------------------------- */
uint8_t GetVolume(const T *pKey) const
ボリュームを取得する。
Definition: SoundsManager.cpp:34
bool AddFile(const T *pFilePath, const T *pKey)
音声ファイルを追加する。
Definition: SoundsManager.cpp:109
SoundsManagerTmpl()
コンストラクタ
Definition: SoundsManager.cpp:6
bool Pause(const T *pKey)
再生を一時停止する。
Definition: SoundsManager.cpp:187
bool SetFrequencyRatio(const T *pKey, float ratio)
再生速度とピッチの変化率を設定する。
Definition: SoundsManager.cpp:76
bool Initialize()
XAudio2の初期化処理を行う。
Definition: SoundsManager.cpp:86
bool Start(const T *pKey, bool isLoopPlayback=false)
ファイルの先頭から再生を行う。
Definition: SoundsManager.cpp:147
Definition: CompressedAudio.cpp:13
~SoundsManagerTmpl()
デストラクタ
Definition: SoundsManager.cpp:9
PlayingStatus GetStatus(const T *pKey) const
再生状況を示すステータスを取得する。
Definition: SoundsManager.cpp:25
bool Resume(const T *pKey)
一時停止中の音声を続きから再生する。
Definition: SoundsManager.cpp:197
SoundsManagerTmpl< T > & operator=(SoundsManagerTmpl< T > &&obj)=default
ムーブ代入演算子のオーバーロード
float GetFrequencyRatio(const T *pKey) const
再生速度とピッチの変化率を取得する。
Definition: SoundsManager.cpp:67
bool SetVolume(const T *pKey, uint8_t volume)
ボリュームを設定する。
Definition: SoundsManager.cpp:53
SoundsManagerのコールバック定義用インターフェース
Definition: ISoundsManagerDelegate.h:17
構築:
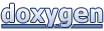