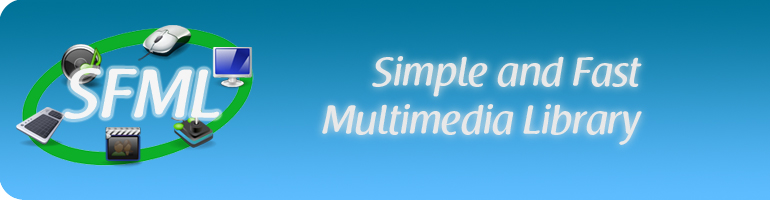
sf::Window Class Reference
Window is a rendering window ; it can create a new window or connect to an existing one. More...
#include <Window.hpp>
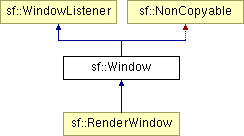
Public Member Functions | |
Window () | |
Default constructor. | |
Window (VideoMode Mode, const std::string &Title, unsigned long WindowStyle=Style::Resize|Style::Close, const WindowSettings &Params=WindowSettings()) | |
Construct a new window. | |
Window (WindowHandle Handle, const WindowSettings &Params=WindowSettings()) | |
Construct the window from an existing control. | |
virtual | ~Window () |
Destructor. | |
void | Create (VideoMode Mode, const std::string &Title, unsigned long WindowStyle=Style::Resize|Style::Close, const WindowSettings &Params=WindowSettings()) |
Create (or recreate) the window. | |
void | Create (WindowHandle Handle, const WindowSettings &Params=WindowSettings()) |
Create (or recreate) the window from an existing control. | |
void | Close () |
Close (destroy) the window. | |
bool | IsOpened () const |
Tell whether or not the window is opened (ie. | |
unsigned int | GetWidth () const |
Get the width of the rendering region of the window. | |
unsigned int | GetHeight () const |
Get the height of the rendering region of the window. | |
const WindowSettings & | GetSettings () const |
Get the creation settings of the window. | |
bool | GetEvent (Event &EventReceived) |
Get the event on top of events stack, if any, and pop it. | |
void | UseVerticalSync (bool Enabled) |
Enable / disable vertical synchronization. | |
void | ShowMouseCursor (bool Show) |
Show or hide the mouse cursor. | |
void | SetCursorPosition (unsigned int Left, unsigned int Top) |
Change the position of the mouse cursor. | |
void | SetPosition (int Left, int Top) |
Change the position of the window on screen. | |
void | SetSize (unsigned int Width, unsigned int Height) |
Change the size of the rendering region of the window. | |
void | Show (bool State) |
Show or hide the window. | |
void | EnableKeyRepeat (bool Enabled) |
Enable or disable automatic key-repeat. | |
void | SetIcon (unsigned int Width, unsigned int Height, const Uint8 *Pixels) |
Change the window's icon. | |
bool | SetActive (bool Active=true) const |
Activate of deactivate the window as the current target for rendering. | |
void | Display () |
Display the window on screen. | |
const Input & | GetInput () const |
Get the input manager of the window. | |
void | SetFramerateLimit (unsigned int Limit) |
Limit the framerate to a maximum fixed frequency. | |
float | GetFrameTime () const |
Get time elapsed since last frame. | |
void | SetJoystickThreshold (float Threshold) |
Change the joystick threshold, ie. |
Detailed Description
Window is a rendering window ; it can create a new window or connect to an existing one.Definition at line 55 of file Window/Window.hpp.
Constructor & Destructor Documentation
sf::Window::Window | ( | ) |
sf::Window::Window | ( | VideoMode | Mode, | |
const std::string & | Title, | |||
unsigned long | WindowStyle = Style::Resize | Style::Close , |
|||
const WindowSettings & | Params = WindowSettings() | |||
) |
Construct a new window.
- Parameters:
-
Mode : Video mode to use Title : Title of the window WindowStyle : Window style, see sf::Style (Resize | Close by default) Params : Creation parameters (see default constructor for default values)
Definition at line 64 of file Window.cpp.
sf::Window::Window | ( | WindowHandle | Handle, | |
const WindowSettings & | Params = WindowSettings() | |||
) |
Construct the window from an existing control.
- Parameters:
-
Handle : Platform-specific handle of the control Params : Creation parameters (see default constructor for default values)
Definition at line 79 of file Window.cpp.
sf::Window::~Window | ( | ) | [virtual] |
Member Function Documentation
void sf::Window::Close | ( | ) |
Close (destroy) the window.
The sf::Window instance remains valid and you can call Create to recreate the window
The sf::Window instance remains valid and you can call Create to recreate the window
Definition at line 165 of file Window.cpp.
void sf::Window::Create | ( | WindowHandle | Handle, | |
const WindowSettings & | Params = WindowSettings() | |||
) |
Create (or recreate) the window from an existing control.
Create the window from an existing control.
- Parameters:
-
Handle : Platform-specific handle of the control Params : Creation parameters (see default constructor for default values)
Definition at line 147 of file Window.cpp.
void sf::Window::Create | ( | VideoMode | Mode, | |
const std::string & | Title, | |||
unsigned long | WindowStyle = Style::Resize | Style::Close , |
|||
const WindowSettings & | Params = WindowSettings() | |||
) |
Create (or recreate) the window.
Create the window.
- Parameters:
-
Mode : Video mode to use Title : Title of the window WindowStyle : Window style, see sf::Style (Resize | Close by default) Params : Creation parameters (see default constructor for default values)
Definition at line 104 of file Window.cpp.
void sf::Window::Display | ( | ) |
void sf::Window::EnableKeyRepeat | ( | bool | Enabled | ) |
Enable or disable automatic key-repeat.
Automatic key-repeat is enabled by default
- Parameters:
-
Enabled : True to enable, false to disable
Definition at line 317 of file Window.cpp.
bool sf::Window::GetEvent | ( | Event & | EventReceived | ) |
Get the event on top of events stack, if any, and pop it.
Get the event on top of events stack, if any.
- Parameters:
-
EventReceived : Event to fill, if any
- Returns:
- True if an event was returned, false if events stack was empty
Definition at line 218 of file Window.cpp.
float sf::Window::GetFrameTime | ( | ) | const |
Get time elapsed since last frame.
- Returns:
- Time elapsed, in seconds
Definition at line 394 of file Window.cpp.
unsigned int sf::Window::GetHeight | ( | ) | const |
Get the height of the rendering region of the window.
- Returns:
- Height in pixels
Reimplemented in sf::RenderWindow.
Definition at line 200 of file Window.cpp.
const Input & sf::Window::GetInput | ( | ) | const |
Get the input manager of the window.
- Returns:
- Reference to the input
Definition at line 376 of file Window.cpp.
const WindowSettings & sf::Window::GetSettings | ( | ) | const |
Get the creation settings of the window.
- Returns:
- Structure containing the creation settings
Definition at line 209 of file Window.cpp.
unsigned int sf::Window::GetWidth | ( | ) | const |
Get the width of the rendering region of the window.
- Returns:
- Width in pixels
Reimplemented in sf::RenderWindow.
Definition at line 191 of file Window.cpp.
bool sf::Window::IsOpened | ( | ) | const |
Tell whether or not the window is opened (ie.
has been created). Note that a hidden window (Show(false)) will still return true
- Returns:
- True if the window is opened
Definition at line 182 of file Window.cpp.
bool sf::Window::SetActive | ( | bool | Active = true |
) | const |
Activate of deactivate the window as the current target for rendering.
Activate of deactivate the window as the current target for rendering.
- Parameters:
-
Active : True to activate, false to deactivate (true by default)
- Returns:
- True if operation was successful, false otherwise
Definition at line 338 of file Window.cpp.
void sf::Window::SetCursorPosition | ( | unsigned int | Left, | |
unsigned int | Top | |||
) |
Change the position of the mouse cursor.
- Parameters:
-
Left : Left coordinate of the cursor, relative to the window Top : Top coordinate of the cursor, relative to the window
Definition at line 260 of file Window.cpp.
void sf::Window::SetFramerateLimit | ( | unsigned int | Limit | ) |
Limit the framerate to a maximum fixed frequency.
Set the framerate at a fixed frequency.
- Parameters:
-
Limit : Framerate limit, in frames per seconds (use 0 to disable limit)
Definition at line 385 of file Window.cpp.
void sf::Window::SetIcon | ( | unsigned int | Width, | |
unsigned int | Height, | |||
const Uint8 * | Pixels | |||
) |
Change the window's icon.
- Parameters:
-
Width : Icon's width, in pixels Height : Icon's height, in pixels Pixels : Pointer to the pixels in memory, format must be RGBA 32 bits
Definition at line 327 of file Window.cpp.
void sf::Window::SetJoystickThreshold | ( | float | Threshold | ) |
Change the joystick threshold, ie.
the value below which no move event will be generated
- Parameters:
-
Threshold : New threshold, in range [0, 100]
Definition at line 404 of file Window.cpp.
void sf::Window::SetPosition | ( | int | Left, | |
int | Top | |||
) |
Change the position of the window on screen.
Only works for top-level windows
- Parameters:
-
Left : Left position Top : Top position
Definition at line 276 of file Window.cpp.
void sf::Window::SetSize | ( | unsigned int | Width, | |
unsigned int | Height | |||
) |
Change the size of the rendering region of the window.
- Parameters:
-
Width : New width Height : New height
Definition at line 293 of file Window.cpp.
void sf::Window::Show | ( | bool | State | ) |
Show or hide the window.
- Parameters:
-
State : True to show, false to hide
Definition at line 303 of file Window.cpp.
void sf::Window::ShowMouseCursor | ( | bool | Show | ) |
Show or hide the mouse cursor.
- Parameters:
-
Show : True to show, false to hide
Definition at line 250 of file Window.cpp.
void sf::Window::UseVerticalSync | ( | bool | Enabled | ) |
Enable / disable vertical synchronization.
- Parameters:
-
Enabled : True to enable v-sync, false to deactivate
Definition at line 240 of file Window.cpp.
The documentation for this class was generated from the following files:
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::