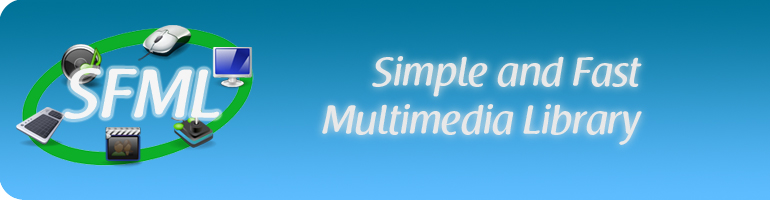
sf::String Class Reference
String defines a graphical 2D text, that can be drawn on screen. More...
#include <String.hpp>

Public Types | |
enum | Style { Regular = 0, Bold = 1 << 0, Italic = 1 << 1, Underlined = 1 << 2 } |
Enumerate the string drawing styles. More... | |
Public Member Functions | |
String () | |
Default constructor. | |
String (const Unicode::Text &Text, const Font &CharFont=Font::GetDefaultFont(), float Size=30.f) | |
Construct the string from any kind of text. | |
void | SetText (const Unicode::Text &Text) |
Set the text (from any kind of string). | |
void | SetFont (const Font &CharFont) |
Set the font of the string. | |
void | SetSize (float Size) |
Set the size of the string The default size is 30. | |
void | SetStyle (unsigned long TextStyle) |
Set the style of the text The default style is Regular. | |
const Unicode::Text & | GetText () const |
Get the text (the returned text can be converted implicitely to any kind of string). | |
const Font & | GetFont () const |
Get the font used by the string. | |
float | GetSize () const |
Get the size of the characters. | |
unsigned long | GetStyle () const |
Get the style of the text. | |
sf::Vector2f | GetCharacterPos (std::size_t Index) const |
Return the visual position of the Index-th character of the string, in coordinates relative to the string (note : translation, center, rotation and scale are not applied). | |
FloatRect | GetRect () const |
Get the string rectangle on screen. | |
void | SetPosition (float X, float Y) |
Set the position of the object (take 2 values). | |
void | SetPosition (const Vector2f &Position) |
Set the position of the object (take a 2D vector). | |
void | SetX (float X) |
Set the X position of the object. | |
void | SetY (float Y) |
Set the Y position of the object. | |
void | SetScale (float ScaleX, float ScaleY) |
Set the scale of the object (take 2 values). | |
void | SetScale (const Vector2f &Scale) |
Set the scale of the object (take a 2D vector). | |
void | SetScaleX (float FactorX) |
Set the X scale factor of the object. | |
void | SetScaleY (float FactorY) |
Set the Y scale factor of the object. | |
void | SetCenter (float CenterX, float CenterY) |
Set the center of the object, in coordinates relative to the top-left of the object (take 2 values). | |
void | SetCenter (const Vector2f &Center) |
Set the center of the object, in coordinates relative to the top-left of the object (take a 2D vector). | |
void | SetRotation (float Rotation) |
Set the orientation of the object. | |
void | SetColor (const Color &Col) |
Set the color of the object. | |
void | SetBlendMode (Blend::Mode Mode) |
Set the blending mode for the object. | |
const Vector2f & | GetPosition () const |
Get the position of the object. | |
const Vector2f & | GetScale () const |
Get the current scale of the object. | |
const Vector2f & | GetCenter () const |
Get the center of the object. | |
float | GetRotation () const |
Get the orientation of the object. | |
const Color & | GetColor () const |
Get the color of the object. | |
Blend::Mode | GetBlendMode () const |
Get the current blending mode. | |
void | Move (float OffsetX, float OffsetY) |
Move the object of a given offset (take 2 values). | |
void | Move (const Vector2f &Offset) |
Move the object of a given offset (take a 2D vector). | |
void | Scale (float FactorX, float FactorY) |
Scale the object (take 2 values). | |
void | Scale (const Vector2f &Factor) |
Scale the object (take a 2D vector). | |
void | Rotate (float Angle) |
Rotate the object. | |
sf::Vector2f | TransformToLocal (const sf::Vector2f &Point) const |
Transform a point from global coordinates into local coordinates (ie it applies the inverse of object's center, translation, rotation and scale to the point). | |
sf::Vector2f | TransformToGlobal (const sf::Vector2f &Point) const |
Transform a point from local coordinates into global coordinates (ie it applies the object's center, translation, rotation and scale to the point). | |
Protected Member Functions | |
virtual void | Render (RenderTarget &Target) const |
/see Drawable::Render | |
const Matrix3 & | GetMatrix () const |
Get the transform matrix of the drawable. | |
const Matrix3 & | GetInverseMatrix () const |
Get the inverse transform matrix of the drawable. |
Detailed Description
String defines a graphical 2D text, that can be drawn on screen.Definition at line 44 of file String.hpp.
Member Enumeration Documentation
enum sf::String::Style |
Enumerate the string drawing styles.
- Enumerator:
-
Regular Regular characters, no style. Bold Characters are bold. Italic Characters are in italic. Underlined Characters are underlined.
Definition at line 51 of file String.hpp.
Constructor & Destructor Documentation
sf::String::String | ( | ) |
sf::String::String | ( | const Unicode::Text & | Text, | |
const Font & | CharFont = Font::GetDefaultFont() , |
|||
float | Size = 30.f | |||
) | [explicit] |
Construct the string from any kind of text.
- Parameters:
-
Text : Text assigned to the string Font : Font used to draw the string (SFML built-in font by default) Size : Characters size (30 by default)
Definition at line 52 of file String.cpp.
Member Function Documentation
Blend::Mode sf::Drawable::GetBlendMode | ( | ) | const [inherited] |
Get the current blending mode.
- Returns:
- Current blending mode
Definition at line 258 of file Drawable.cpp.
const Vector2f & sf::Drawable::GetCenter | ( | ) | const [inherited] |
Get the center of the object.
- Returns:
- Current position of the center
Definition at line 231 of file Drawable.cpp.
sf::Vector2f sf::String::GetCharacterPos | ( | std::size_t | Index | ) | const |
Return the visual position of the Index-th character of the string, in coordinates relative to the string (note : translation, center, rotation and scale are not applied).
Return the visual position of the Index-th character of the string, in coordinates relative to the string (note : translation, center, rotation and scale are not applied).
- Parameters:
-
Index : Index of the character
- Returns:
- Position of the Index-th character (end of string if Index is out of range)
Definition at line 153 of file String.cpp.
const Color & sf::Drawable::GetColor | ( | ) | const [inherited] |
const Font & sf::String::GetFont | ( | ) | const |
const Matrix3 & sf::Drawable::GetInverseMatrix | ( | ) | const [protected, inherited] |
Get the inverse transform matrix of the drawable.
- Returns:
- Inverse transform matrix
Definition at line 350 of file Drawable.cpp.
const Matrix3 & sf::Drawable::GetMatrix | ( | ) | const [protected, inherited] |
Get the transform matrix of the drawable.
- Returns:
- Transform matrix
Definition at line 334 of file Drawable.cpp.
const Vector2f & sf::Drawable::GetPosition | ( | ) | const [inherited] |
Get the position of the object.
- Returns:
- Current position
Definition at line 213 of file Drawable.cpp.
FloatRect sf::String::GetRect | ( | ) | const |
Get the string rectangle on screen.
- Returns:
- Rectangle contaning the string in screen coordinates
Definition at line 195 of file String.cpp.
float sf::Drawable::GetRotation | ( | ) | const [inherited] |
Get the orientation of the object.
Rotation is always in the range [0, 360]
- Returns:
- Current rotation, in degrees
Definition at line 240 of file Drawable.cpp.
const Vector2f & sf::Drawable::GetScale | ( | ) | const [inherited] |
Get the current scale of the object.
- Returns:
- Current scale factor (always positive)
Definition at line 222 of file Drawable.cpp.
float sf::String::GetSize | ( | ) | const |
Get the size of the characters.
- Returns:
- Size of the characters
Definition at line 133 of file String.cpp.
unsigned long sf::String::GetStyle | ( | ) | const |
Get the style of the text.
- Returns:
- Current string style (combination of Style enum values)
Definition at line 142 of file String.cpp.
const Unicode::Text & sf::String::GetText | ( | ) | const |
Get the text (the returned text can be converted implicitely to any kind of string).
- Returns:
- String's text
Definition at line 115 of file String.cpp.
void sf::Drawable::Move | ( | const Vector2f & | Offset | ) | [inherited] |
Move the object of a given offset (take a 2D vector).
- Parameters:
-
Offset : Amount of units to move the object of
Definition at line 278 of file Drawable.cpp.
void sf::Drawable::Move | ( | float | OffsetX, | |
float | OffsetY | |||
) | [inherited] |
Move the object of a given offset (take 2 values).
- Parameters:
-
OffsetX : X offset OffsetY : Y offset
Definition at line 268 of file Drawable.cpp.
void sf::String::Render | ( | RenderTarget & | Target | ) | const [protected, virtual] |
/see Drawable::Render
/see sfDrawable::Render
Implements sf::Drawable.
Definition at line 213 of file String.cpp.
void sf::Drawable::Rotate | ( | float | Angle | ) | [inherited] |
Rotate the object.
- Parameters:
-
Angle : Angle of rotation, in degrees
Definition at line 306 of file Drawable.cpp.
void sf::Drawable::Scale | ( | const Vector2f & | Factor | ) | [inherited] |
Scale the object (take a 2D vector).
- Parameters:
-
Factor : Scaling factors (both values must be strictly positive)
Definition at line 297 of file Drawable.cpp.
void sf::Drawable::Scale | ( | float | FactorX, | |
float | FactorY | |||
) | [inherited] |
Scale the object (take 2 values).
- Parameters:
-
FactorX : Scaling factor on X (must be strictly positive) FactorY : Scaling factor on Y (must be strictly positive)
Definition at line 287 of file Drawable.cpp.
void sf::Drawable::SetBlendMode | ( | Blend::Mode | Mode | ) | [inherited] |
Set the blending mode for the object.
The default blend mode is Blend::Alpha
- Parameters:
-
Mode : New blending mode
Definition at line 204 of file Drawable.cpp.
void sf::Drawable::SetCenter | ( | const Vector2f & | Center | ) | [inherited] |
Set the center of the object, in coordinates relative to the top-left of the object (take a 2D vector).
Set the center of the object, in coordinates relative to the top-left of the object (take a 2D vector).
The default center is (0, 0)
- Parameters:
-
Center : New center
Definition at line 171 of file Drawable.cpp.
void sf::Drawable::SetCenter | ( | float | CenterX, | |
float | CenterY | |||
) | [inherited] |
Set the center of the object, in coordinates relative to the top-left of the object (take 2 values).
Set the center of the object, in coordinates relative to the top-left of the object (take 2 values).
The default center is (0, 0)
- Parameters:
-
CenterX : X coordinate of the center CenterY : Y coordinate of the center
Definition at line 157 of file Drawable.cpp.
void sf::Drawable::SetColor | ( | const Color & | Col | ) | [inherited] |
Set the color of the object.
The default color is white
- Parameters:
-
Col : New color
Definition at line 194 of file Drawable.cpp.
void sf::String::SetFont | ( | const Font & | CharFont | ) |
void sf::Drawable::SetPosition | ( | const Vector2f & | Position | ) | [inherited] |
Set the position of the object (take a 2D vector).
- Parameters:
-
Position : New position
Definition at line 75 of file Drawable.cpp.
void sf::Drawable::SetPosition | ( | float | X, | |
float | Y | |||
) | [inherited] |
Set the position of the object (take 2 values).
- Parameters:
-
X : New X coordinate Y : New Y coordinate
Definition at line 65 of file Drawable.cpp.
void sf::Drawable::SetRotation | ( | float | Rotation | ) | [inherited] |
Set the orientation of the object.
- Parameters:
-
Rotation : Angle of rotation, in degrees
Definition at line 180 of file Drawable.cpp.
void sf::Drawable::SetScale | ( | const Vector2f & | Scale | ) | [inherited] |
Set the scale of the object (take a 2D vector).
- Parameters:
-
Scale : New scale (both values must be strictly positive)
Definition at line 117 of file Drawable.cpp.
void sf::Drawable::SetScale | ( | float | ScaleX, | |
float | ScaleY | |||
) | [inherited] |
Set the scale of the object (take 2 values).
- Parameters:
-
ScaleX : New horizontal scale (must be strictly positive) ScaleY : New vertical scale (must be strictly positive)
Definition at line 107 of file Drawable.cpp.
void sf::Drawable::SetScaleX | ( | float | FactorX | ) | [inherited] |
Set the X scale factor of the object.
- Parameters:
-
X : New X scale factor
Definition at line 127 of file Drawable.cpp.
void sf::Drawable::SetScaleY | ( | float | FactorY | ) | [inherited] |
Set the Y scale factor of the object.
- Parameters:
-
Y : New Y scale factor
Definition at line 141 of file Drawable.cpp.
void sf::String::SetSize | ( | float | Size | ) |
Set the size of the string The default size is 30.
Set the size of the string.
- Parameters:
-
Size : New size, in pixels
Definition at line 88 of file String.cpp.
void sf::String::SetStyle | ( | unsigned long | TextStyle | ) |
Set the style of the text The default style is Regular.
Set the style of the text The default style is Regular.
- Parameters:
-
TextStyle : New text style, (combination of Style enum values)
Definition at line 102 of file String.cpp.
void sf::String::SetText | ( | const Unicode::Text & | Text | ) |
Set the text (from any kind of string).
- Parameters:
-
Text : New text
Definition at line 65 of file String.cpp.
void sf::Drawable::SetX | ( | float | X | ) | [inherited] |
Set the X position of the object.
- Parameters:
-
X : New X coordinate
Definition at line 85 of file Drawable.cpp.
void sf::Drawable::SetY | ( | float | Y | ) | [inherited] |
Set the Y position of the object.
- Parameters:
-
Y : New Y coordinate
Definition at line 96 of file Drawable.cpp.
sf::Vector2f sf::Drawable::TransformToGlobal | ( | const sf::Vector2f & | Point | ) | const [inherited] |
Transform a point from local coordinates into global coordinates (ie it applies the object's center, translation, rotation and scale to the point).
Transform a point from local coordinates into global coordinates (ie it applies the object's center, translation, rotation and scale to the point).
- Parameters:
-
Point : Point to transform
- Returns:
- Transformed point
Definition at line 325 of file Drawable.cpp.
sf::Vector2f sf::Drawable::TransformToLocal | ( | const sf::Vector2f & | Point | ) | const [inherited] |
Transform a point from global coordinates into local coordinates (ie it applies the inverse of object's center, translation, rotation and scale to the point).
Transform a point from global coordinates into local coordinates (ie it applies the inverse of object's center, translation, rotation and scale to the point).
- Parameters:
-
Point : Point to transform
- Returns:
- Transformed point
Definition at line 316 of file Drawable.cpp.
The documentation for this class was generated from the following files:
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::