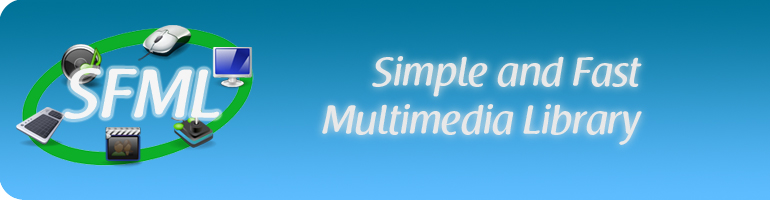
sf::Sound Class Reference
Sound defines the properties of a sound such as position, volume, pitch, etc. More...
#include <Sound.hpp>
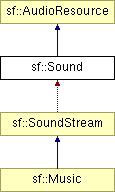
Public Types | |
enum | Status { Stopped, Paused, Playing } |
Enumeration of the sound states. More... | |
Public Member Functions | |
Sound () | |
Default constructor. | |
Sound (const SoundBuffer &Buffer, bool Loop=false, float Pitch=1.f, float Volume=100.f, const Vector3f &Position=Vector3f(0, 0, 0)) | |
Construct the sound from its parameters. | |
Sound (const Sound &Copy) | |
Copy constructor. | |
~Sound () | |
Destructor. | |
void | Play () |
Play the sound. | |
void | Pause () |
Pause the sound. | |
void | Stop () |
Stop the sound. | |
void | SetBuffer (const SoundBuffer &Buffer) |
Set the source buffer. | |
void | SetLoop (bool Loop) |
Set the sound loop state. | |
void | SetPitch (float Pitch) |
Set the sound pitch. | |
void | SetVolume (float Volume) |
Set the sound volume. | |
void | SetPosition (float X, float Y, float Z) |
Set the sound position (take 3 values). | |
void | SetPosition (const Vector3f &Position) |
Set the sound position (take a 3D vector). | |
void | SetRelativeToListener (bool Relative) |
Make the sound's position relative to the listener's position, or absolute. | |
void | SetMinDistance (float MinDistance) |
Set the minimum distance - closer than this distance, the listener will hear the sound at its maximum volume. | |
void | SetAttenuation (float Attenuation) |
Set the attenuation factor - the higher the attenuation, the more the sound will be attenuated with distance from listener. | |
void | SetPlayingOffset (float TimeOffset) |
Set the current playing position of the sound. | |
const SoundBuffer * | GetBuffer () const |
Get the source buffer. | |
bool | GetLoop () const |
Tell whether or not the sound is looping. | |
float | GetPitch () const |
Get the pitch. | |
float | GetVolume () const |
Get the volume. | |
Vector3f | GetPosition () const |
Get the sound position. | |
bool | IsRelativeToListener () const |
Tell if the sound's position is relative to the listener's position, or if it's absolute. | |
float | GetMinDistance () const |
Get the minimum distance. | |
float | GetAttenuation () const |
Get the attenuation factor. | |
Status | GetStatus () const |
Get the status of the sound (stopped, paused, playing). | |
float | GetPlayingOffset () const |
Get the current playing position of the sound. | |
Sound & | operator= (const Sound &Other) |
Assignment operator. | |
void | ResetBuffer () |
Reset the internal buffer. | |
Friends | |
class | SoundStream |
Detailed Description
Sound defines the properties of a sound such as position, volume, pitch, etc.Definition at line 45 of file Sound.hpp.
Member Enumeration Documentation
enum sf::Sound::Status |
Constructor & Destructor Documentation
sf::Sound::Sound | ( | const SoundBuffer & | Buffer, | |
bool | Loop = false , |
|||
float | Pitch = 1.f , |
|||
float | Volume = 100.f , |
|||
const Vector3f & | Position = Vector3f(0, 0, 0) | |||
) | [explicit] |
sf::Sound::Sound | ( | const Sound & | Copy | ) |
Member Function Documentation
float sf::Sound::GetAttenuation | ( | ) | const |
const SoundBuffer * sf::Sound::GetBuffer | ( | ) | const |
bool sf::Sound::GetLoop | ( | ) | const |
Tell whether or not the sound is looping.
- Returns:
- True if the sound is looping, false otherwise
Reimplemented in sf::SoundStream.
float sf::Sound::GetMinDistance | ( | ) | const |
float sf::Sound::GetPitch | ( | ) | const |
float sf::Sound::GetPlayingOffset | ( | ) | const |
Get the current playing position of the sound.
- Returns:
- Current playing position, expressed in seconds
Reimplemented in sf::SoundStream.
Vector3f sf::Sound::GetPosition | ( | ) | const |
Sound::Status sf::Sound::GetStatus | ( | ) | const |
Get the status of the sound (stopped, paused, playing).
- Returns:
- Current status of the sound
Reimplemented in sf::SoundStream.
float sf::Sound::GetVolume | ( | ) | const |
bool sf::Sound::IsRelativeToListener | ( | ) | const |
void sf::Sound::Play | ( | ) |
void sf::Sound::ResetBuffer | ( | ) |
void sf::Sound::SetAttenuation | ( | float | Attenuation | ) |
Set the attenuation factor - the higher the attenuation, the more the sound will be attenuated with distance from listener.
Set the attenuation factor - the higher the attenuation, the more the sound will be attenuated with distance from listener.
The default attenuation factor 1.0
- Parameters:
-
Attenuation : New attenuation factor for the sound
void sf::Sound::SetBuffer | ( | const SoundBuffer & | Buffer | ) |
void sf::Sound::SetLoop | ( | bool | Loop | ) |
Set the sound loop state.
This parameter is disabled by default
- Parameters:
-
Loop : True to play in loop, false to play once
Reimplemented in sf::SoundStream.
void sf::Sound::SetMinDistance | ( | float | MinDistance | ) |
Set the minimum distance - closer than this distance, the listener will hear the sound at its maximum volume.
Set the minimum distance - closer than this distance, the listener will hear the sound at its maximum volume.
The default minimum distance is 1.0
- Parameters:
-
MinDistance : New minimum distance for the sound
void sf::Sound::SetPitch | ( | float | Pitch | ) |
void sf::Sound::SetPlayingOffset | ( | float | TimeOffset | ) |
void sf::Sound::SetPosition | ( | const Vector3f & | Position | ) |
void sf::Sound::SetPosition | ( | float | X, | |
float | Y, | |||
float | Z | |||
) |
void sf::Sound::SetRelativeToListener | ( | bool | Relative | ) |
Make the sound's position relative to the listener's position, or absolute.
Make the sound's position relative to the listener's position, or absolute.
The default value is false (absolute)
- Parameters:
-
Relative : True to set the position relative, false to set it absolute
void sf::Sound::SetVolume | ( | float | Volume | ) |
void sf::Sound::Stop | ( | ) |
The documentation for this class was generated from the following files:
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::