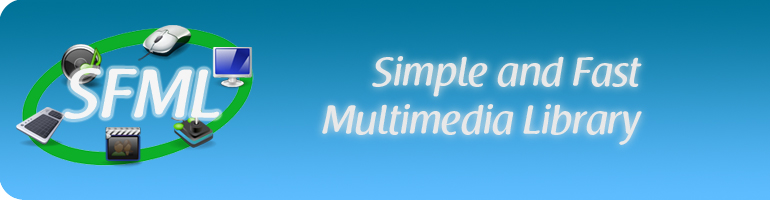
Window.hpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00025 #ifndef SFML_WINDOW_HPP 00026 #define SFML_WINDOW_HPP 00027 00029 // Headers 00031 #include <SFML/Window/Event.hpp> 00032 #include <SFML/Window/Input.hpp> 00033 #include <SFML/Window/VideoMode.hpp> 00034 #include <SFML/Window/WindowHandle.hpp> 00035 #include <SFML/Window/WindowListener.hpp> 00036 #include <SFML/Window/WindowSettings.hpp> 00037 #include <SFML/Window/WindowStyle.hpp> 00038 #include <SFML/System/Clock.hpp> 00039 #include <SFML/System/NonCopyable.hpp> 00040 #include <queue> 00041 #include <string> 00042 00043 00044 namespace sf 00045 { 00046 namespace priv 00047 { 00048 class WindowImpl; 00049 } 00050 00055 class SFML_API Window : public WindowListener, NonCopyable 00056 { 00057 public : 00058 00063 Window(); 00064 00074 Window(VideoMode Mode, const std::string& Title, unsigned long WindowStyle = Style::Resize | Style::Close, const WindowSettings& Params = WindowSettings()); 00075 00083 Window(WindowHandle Handle, const WindowSettings& Params = WindowSettings()); 00084 00089 virtual ~Window(); 00090 00100 void Create(VideoMode Mode, const std::string& Title, unsigned long WindowStyle = Style::Resize | Style::Close, const WindowSettings& Params = WindowSettings()); 00101 00109 void Create(WindowHandle Handle, const WindowSettings& Params = WindowSettings()); 00110 00117 void Close(); 00118 00127 bool IsOpened() const; 00128 00135 unsigned int GetWidth() const; 00136 00143 unsigned int GetHeight() const; 00144 00151 const WindowSettings& GetSettings() const; 00152 00161 bool GetEvent(Event& EventReceived); 00162 00169 void UseVerticalSync(bool Enabled); 00170 00177 void ShowMouseCursor(bool Show); 00178 00186 void SetCursorPosition(unsigned int Left, unsigned int Top); 00187 00196 void SetPosition(int Left, int Top); 00197 00205 void SetSize(unsigned int Width, unsigned int Height); 00206 00213 void Show(bool State); 00214 00222 void EnableKeyRepeat(bool Enabled); 00223 00232 void SetIcon(unsigned int Width, unsigned int Height, const Uint8* Pixels); 00233 00243 bool SetActive(bool Active = true) const; 00244 00249 void Display(); 00250 00257 const Input& GetInput() const; 00258 00265 void SetFramerateLimit(unsigned int Limit); 00266 00273 float GetFrameTime() const; 00274 00282 void SetJoystickThreshold(float Threshold); 00283 00284 private : 00285 00290 virtual void OnCreate(); 00291 00298 virtual void OnEvent(const Event& EventReceived); 00299 00306 void Initialize(priv::WindowImpl* Impl); 00307 00309 // Member data 00311 priv::WindowImpl* myWindow; 00312 std::queue<Event> myEvents; 00313 Input myInput; 00314 Clock myClock; 00315 WindowSettings mySettings; 00316 float myLastFrameTime; 00317 bool myIsExternal; 00318 unsigned int myFramerateLimit; 00319 int mySetCursorPosX; 00320 int mySetCursorPosY; 00321 }; 00322 00323 } // namespace sf 00324 00325 00326 #endif // SFML_WINDOW_HPP
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::