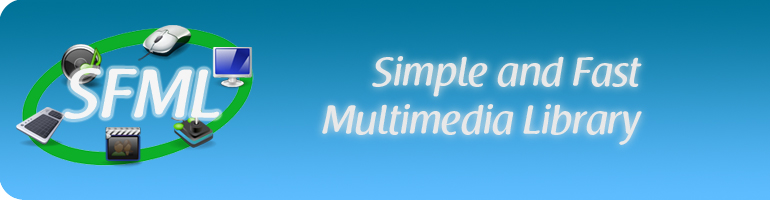
Window.cpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00026 // Headers 00028 #include <SFML/Window/Window.hpp> 00029 #include <SFML/Window/Context.hpp> 00030 #include <SFML/Window/WindowImpl.hpp> 00031 #include <SFML/System/Sleep.hpp> 00032 #include <iostream> 00033 00034 00036 // Private data 00038 namespace 00039 { 00040 const sf::Window* FullscreenWindow = NULL; 00041 } 00042 00043 00044 namespace sf 00045 { 00049 Window::Window() : 00050 myWindow (NULL), 00051 myLastFrameTime (0.f), 00052 myIsExternal (false), 00053 myFramerateLimit(0), 00054 mySetCursorPosX (0xFFFF), 00055 mySetCursorPosY (0xFFFF) 00056 { 00057 00058 } 00059 00060 00064 Window::Window(VideoMode Mode, const std::string& Title, unsigned long WindowStyle, const WindowSettings& Params) : 00065 myWindow (NULL), 00066 myLastFrameTime (0.f), 00067 myIsExternal (false), 00068 myFramerateLimit(0), 00069 mySetCursorPosX (0xFFFF), 00070 mySetCursorPosY (0xFFFF) 00071 { 00072 Create(Mode, Title, WindowStyle, Params); 00073 } 00074 00075 00079 Window::Window(WindowHandle Handle, const WindowSettings& Params) : 00080 myWindow (NULL), 00081 myLastFrameTime (0.f), 00082 myIsExternal (true), 00083 myFramerateLimit(0), 00084 mySetCursorPosX (0xFFFF), 00085 mySetCursorPosY (0xFFFF) 00086 { 00087 Create(Handle, Params); 00088 } 00089 00090 00094 Window::~Window() 00095 { 00096 // Close the window 00097 Close(); 00098 } 00099 00100 00104 void Window::Create(VideoMode Mode, const std::string& Title, unsigned long WindowStyle, const WindowSettings& Params) 00105 { 00106 // Destroy the previous window implementation 00107 Close(); 00108 00109 // Fullscreen style requires some tests 00110 if (WindowStyle & Style::Fullscreen) 00111 { 00112 // Make sure there's not already a fullscreen window (only one is allowed) 00113 if (FullscreenWindow) 00114 { 00115 std::cerr << "Creating two fullscreen windows is not allowed, switching to windowed mode" << std::endl; 00116 WindowStyle &= ~Style::Fullscreen; 00117 } 00118 else 00119 { 00120 // Make sure the chosen video mode is compatible 00121 if (!Mode.IsValid()) 00122 { 00123 std::cerr << "The requested video mode is not available, switching to a valid mode" << std::endl; 00124 Mode = VideoMode::GetMode(0); 00125 } 00126 00127 // Update the fullscreen window 00128 FullscreenWindow = this; 00129 } 00130 } 00131 00132 // Check validity of style 00133 if ((WindowStyle & Style::Close) || (WindowStyle & Style::Resize)) 00134 WindowStyle |= Style::Titlebar; 00135 00136 // Activate the global context 00137 Context::GetGlobal().SetActive(true); 00138 00139 mySettings = Params; 00140 Initialize(priv::WindowImpl::New(Mode, Title, WindowStyle, mySettings)); 00141 } 00142 00143 00147 void Window::Create(WindowHandle Handle, const WindowSettings& Params) 00148 { 00149 // Destroy the previous window implementation 00150 Close(); 00151 00152 // Activate the global context 00153 Context::GetGlobal().SetActive(true); 00154 00155 mySettings = Params; 00156 Initialize(priv::WindowImpl::New(Handle, mySettings)); 00157 } 00158 00159 00165 void Window::Close() 00166 { 00167 // Delete the window implementation 00168 delete myWindow; 00169 myWindow = NULL; 00170 00171 // Update the fullscreen window 00172 if (this == FullscreenWindow) 00173 FullscreenWindow = NULL; 00174 } 00175 00176 00182 bool Window::IsOpened() const 00183 { 00184 return myWindow != NULL; 00185 } 00186 00187 00191 unsigned int Window::GetWidth() const 00192 { 00193 return myWindow ? myWindow->GetWidth() : 0; 00194 } 00195 00196 00200 unsigned int Window::GetHeight() const 00201 { 00202 return myWindow ? myWindow->GetHeight() : 0; 00203 } 00204 00205 00209 const WindowSettings& Window::GetSettings() const 00210 { 00211 return mySettings; 00212 } 00213 00214 00218 bool Window::GetEvent(Event& EventReceived) 00219 { 00220 // Let the window implementation process incoming events if the events queue is empty 00221 if (myWindow && myEvents.empty()) 00222 myWindow->DoEvents(); 00223 00224 // Pop first event of queue, if not empty 00225 if (!myEvents.empty()) 00226 { 00227 EventReceived = myEvents.front(); 00228 myEvents.pop(); 00229 00230 return true; 00231 } 00232 00233 return false; 00234 } 00235 00236 00240 void Window::UseVerticalSync(bool Enabled) 00241 { 00242 if (SetActive()) 00243 myWindow->UseVerticalSync(Enabled); 00244 } 00245 00246 00250 void Window::ShowMouseCursor(bool Show) 00251 { 00252 if (myWindow) 00253 myWindow->ShowMouseCursor(Show); 00254 } 00255 00256 00260 void Window::SetCursorPosition(unsigned int Left, unsigned int Top) 00261 { 00262 if (myWindow) 00263 { 00264 // Keep coordinates for later checking (to reject the generated MouseMoved event) 00265 mySetCursorPosX = Left; 00266 mySetCursorPosY = Top; 00267 00268 myWindow->SetCursorPosition(Left, Top); 00269 } 00270 } 00271 00272 00276 void Window::SetPosition(int Left, int Top) 00277 { 00278 if (!myIsExternal) 00279 { 00280 if (myWindow) 00281 myWindow->SetPosition(Left, Top); 00282 } 00283 else 00284 { 00285 std::cerr << "Warning : trying to change the position of an external SFML window, which is not allowed" << std::endl; 00286 } 00287 } 00288 00289 00293 void Window::SetSize(unsigned int Width, unsigned int Height) 00294 { 00295 if (myWindow) 00296 myWindow->SetSize(Width, Height); 00297 } 00298 00299 00303 void Window::Show(bool State) 00304 { 00305 if (!myIsExternal) 00306 { 00307 if (myWindow) 00308 myWindow->Show(State); 00309 } 00310 } 00311 00312 00317 void Window::EnableKeyRepeat(bool Enabled) 00318 { 00319 if (myWindow) 00320 myWindow->EnableKeyRepeat(Enabled); 00321 } 00322 00323 00327 void Window::SetIcon(unsigned int Width, unsigned int Height, const Uint8* Pixels) 00328 { 00329 if (myWindow) 00330 myWindow->SetIcon(Width, Height, Pixels); 00331 } 00332 00333 00338 bool Window::SetActive(bool Active) const 00339 { 00340 if (myWindow) 00341 { 00342 myWindow->SetActive(Active); 00343 return true; 00344 } 00345 00346 return false; 00347 } 00348 00349 00353 void Window::Display() 00354 { 00355 // Limit the framerate if needed 00356 if (myFramerateLimit > 0) 00357 { 00358 float RemainingTime = 1.f / myFramerateLimit - myClock.GetElapsedTime(); 00359 if (RemainingTime > 0) 00360 Sleep(RemainingTime); 00361 } 00362 00363 // Measure the time elapsed since last frame 00364 myLastFrameTime = myClock.GetElapsedTime(); 00365 myClock.Reset(); 00366 00367 // Display the backbuffer on screen 00368 if (SetActive()) 00369 myWindow->Display(); 00370 } 00371 00372 00376 const Input& Window::GetInput() const 00377 { 00378 return myInput; 00379 } 00380 00381 00385 void Window::SetFramerateLimit(unsigned int Limit) 00386 { 00387 myFramerateLimit = Limit; 00388 } 00389 00390 00394 float Window::GetFrameTime() const 00395 { 00396 return myLastFrameTime; 00397 } 00398 00399 00404 void Window::SetJoystickThreshold(float Threshold) 00405 { 00406 if (myWindow) 00407 myWindow->SetJoystickThreshold(Threshold); 00408 } 00409 00410 00414 void Window::OnCreate() 00415 { 00416 // Nothing by default 00417 } 00418 00419 00423 void Window::OnEvent(const Event& EventReceived) 00424 { 00425 // Discard MouseMove events generated by SetCursorPosition 00426 if ((EventReceived.Type == Event::MouseMoved) && 00427 (EventReceived.MouseMove.X == mySetCursorPosX) && 00428 (EventReceived.MouseMove.Y == mySetCursorPosY)) 00429 { 00430 mySetCursorPosX = 0xFFFF; 00431 mySetCursorPosY = 0xFFFF; 00432 return; 00433 } 00434 00435 myEvents.push(EventReceived); 00436 } 00437 00438 00442 void Window::Initialize(priv::WindowImpl* Window) 00443 { 00444 // Assign and initialize the new window 00445 myWindow = Window; 00446 myWindow->Initialize(); 00447 00448 // Clear the event queue 00449 while (!myEvents.empty()) 00450 myEvents.pop(); 00451 00452 // Listen to events from the new window 00453 myWindow->AddListener(this); 00454 myWindow->AddListener(&myInput); 00455 00456 // Setup default behaviours (to get a consistent behaviour across different implementations) 00457 Show(true); 00458 UseVerticalSync(false); 00459 ShowMouseCursor(true); 00460 EnableKeyRepeat(true); 00461 00462 // Reset frame time 00463 myClock.Reset(); 00464 myLastFrameTime = 0.f; 00465 00466 // Activate the window 00467 SetActive(true); 00468 00469 // Notify the derived class 00470 OnCreate(); 00471 } 00472 00473 } // namespace sf
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::