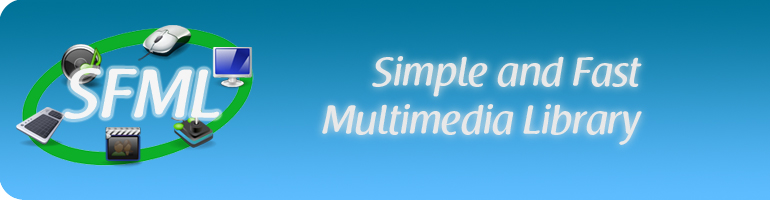
sf::Music Class Reference
Music defines a big sound played using streaming, so usually what we call a music :). More...
#include <Music.hpp>
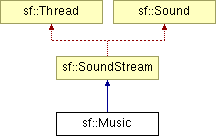
Public Types | |
enum | Status |
Enumeration of the sound states. More... | |
Public Member Functions | |
Music (std::size_t BufferSize=44100) | |
Construct the music with a buffer size. | |
~Music () | |
Destructor. | |
bool | OpenFromFile (const std::string &Filename) |
Open a music file (doesn't play it -- call Play() for that). | |
bool | OpenFromMemory (const char *Data, std::size_t SizeInBytes) |
Open a music file from memory (doesn't play it -- call Play() for that). | |
float | GetDuration () const |
Get the music duration. | |
void | Play () |
Start playing the audio stream. | |
void | Stop () |
Stop playing the audio stream. | |
unsigned int | GetChannelsCount () const |
Return the number of channels (1 = mono, 2 = stereo). | |
unsigned int | GetSampleRate () const |
Get the stream sample rate. | |
Status | GetStatus () const |
Get the status of the stream (stopped, paused, playing). | |
float | GetPlayingOffset () const |
Get the current playing position of the stream. | |
void | SetLoop (bool Loop) |
Set the stream loop state. | |
bool | GetLoop () const |
Tell whether or not the stream is looping. | |
Protected Member Functions | |
void | Initialize (unsigned int ChannelsCount, unsigned int SampleRate) |
Set the audio stream parameters, you must call it before Play(). |
Detailed Description
Music defines a big sound played using streaming, so usually what we call a music :).Definition at line 47 of file Music.hpp.
Member Enumeration Documentation
enum sf::Sound::Status [inherited] |
Constructor & Destructor Documentation
sf::Music::Music | ( | std::size_t | BufferSize = 44100 |
) | [explicit] |
Member Function Documentation
unsigned int sf::SoundStream::GetChannelsCount | ( | ) | const [inherited] |
Return the number of channels (1 = mono, 2 = stereo).
Return the number of channels (1 = mono, 2 = stereo, .
- Returns:
- Number of channels
Definition at line 126 of file SoundStream.cpp.
float sf::Music::GetDuration | ( | ) | const |
bool sf::SoundStream::GetLoop | ( | ) | const [inherited] |
Tell whether or not the stream is looping.
Tell whether or not the music is looping.
- Returns:
- True if the music is looping, false otherwise
Reimplemented from sf::Sound.
Definition at line 180 of file SoundStream.cpp.
float sf::SoundStream::GetPlayingOffset | ( | ) | const [inherited] |
Get the current playing position of the stream.
- Returns:
- Current playing position, expressed in seconds
Current playing position, expressed in seconds
Reimplemented from sf::Sound.
Definition at line 162 of file SoundStream.cpp.
unsigned int sf::SoundStream::GetSampleRate | ( | ) | const [inherited] |
Get the stream sample rate.
Get the sound frequency (sample rate).
- Returns:
- Stream frequency (number of samples per second)
Definition at line 135 of file SoundStream.cpp.
Sound::Status sf::SoundStream::GetStatus | ( | ) | const [inherited] |
Get the status of the stream (stopped, paused, playing).
Get the status of the sound (stopped, paused, playing).
- Returns:
- Current status of the sound
Reimplemented from sf::Sound.
Definition at line 144 of file SoundStream.cpp.
void sf::SoundStream::Initialize | ( | unsigned int | ChannelsCount, | |
unsigned int | SampleRate | |||
) | [protected, inherited] |
Set the audio stream parameters, you must call it before Play().
- Parameters:
-
ChannelsCount : Number of channels SampleRate : Sample rate
Definition at line 64 of file SoundStream.cpp.
bool sf::Music::OpenFromFile | ( | const std::string & | Filename | ) |
bool sf::Music::OpenFromMemory | ( | const char * | Data, | |
std::size_t | SizeInBytes | |||
) |
void sf::SoundStream::Play | ( | ) | [inherited] |
Start playing the audio stream.
Reimplemented from sf::Sound.
Definition at line 85 of file SoundStream.cpp.
void sf::SoundStream::SetLoop | ( | bool | Loop | ) | [inherited] |
Set the stream loop state.
Set the music loop state.
This parameter is disabled by default
- Parameters:
-
Loop : True to play in loop, false to play once
Reimplemented from sf::Sound.
Definition at line 171 of file SoundStream.cpp.
void sf::SoundStream::Stop | ( | ) | [inherited] |
Stop playing the audio stream.
Reimplemented from sf::Sound.
Definition at line 115 of file SoundStream.cpp.
The documentation for this class was generated from the following files:
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::