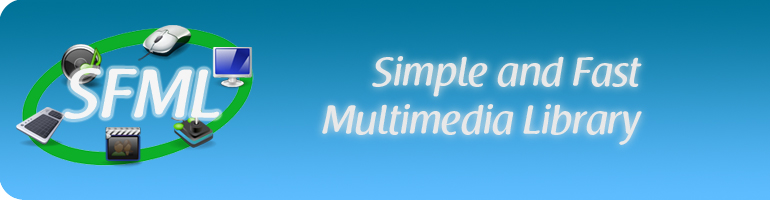
sf::RenderWindow Class Reference
Simple wrapper for sf::Window that allows easy 2D rendering. More...
#include <RenderWindow.hpp>
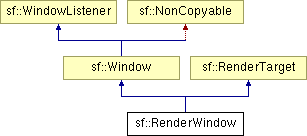
Public Member Functions | |
RenderWindow () | |
Default constructor. | |
RenderWindow (VideoMode Mode, const std::string &Title, unsigned long WindowStyle=Style::Resize|Style::Close, const WindowSettings &Params=WindowSettings()) | |
Construct the window. | |
RenderWindow (WindowHandle Handle, const WindowSettings &Params=WindowSettings()) | |
Construct the window from an existing control. | |
virtual | ~RenderWindow () |
Destructor. | |
virtual unsigned int | GetWidth () const |
Get the width of the rendering region of the window. | |
virtual unsigned int | GetHeight () const |
Get the height of the rendering region of the window. | |
Image | Capture () const |
Save the content of the window to an image. | |
sf::Vector2f | ConvertCoords (unsigned int WindowX, unsigned int WindowY, const View *TargetView=NULL) const |
Convert a point in window coordinates into view coordinates. | |
void | Create (VideoMode Mode, const std::string &Title, unsigned long WindowStyle=Style::Resize|Style::Close, const WindowSettings &Params=WindowSettings()) |
Create (or recreate) the window. | |
void | Create (WindowHandle Handle, const WindowSettings &Params=WindowSettings()) |
Create (or recreate) the window from an existing control. | |
void | Close () |
Close (destroy) the window. | |
bool | IsOpened () const |
Tell whether or not the window is opened (ie. | |
const WindowSettings & | GetSettings () const |
Get the creation settings of the window. | |
bool | GetEvent (Event &EventReceived) |
Get the event on top of events stack, if any, and pop it. | |
void | UseVerticalSync (bool Enabled) |
Enable / disable vertical synchronization. | |
void | ShowMouseCursor (bool Show) |
Show or hide the mouse cursor. | |
void | SetCursorPosition (unsigned int Left, unsigned int Top) |
Change the position of the mouse cursor. | |
void | SetPosition (int Left, int Top) |
Change the position of the window on screen. | |
void | SetSize (unsigned int Width, unsigned int Height) |
Change the size of the rendering region of the window. | |
void | Show (bool State) |
Show or hide the window. | |
void | EnableKeyRepeat (bool Enabled) |
Enable or disable automatic key-repeat. | |
void | SetIcon (unsigned int Width, unsigned int Height, const Uint8 *Pixels) |
Change the window's icon. | |
bool | SetActive (bool Active=true) const |
Activate of deactivate the window as the current target for rendering. | |
void | Display () |
Display the window on screen. | |
const Input & | GetInput () const |
Get the input manager of the window. | |
void | SetFramerateLimit (unsigned int Limit) |
Limit the framerate to a maximum fixed frequency. | |
float | GetFrameTime () const |
Get time elapsed since last frame. | |
void | SetJoystickThreshold (float Threshold) |
Change the joystick threshold, ie. | |
void | Clear (const Color &FillColor=Color(0, 0, 0)) |
Clear the entire target with a single color. | |
virtual void | Draw (const Drawable &Object) |
Draw something into the target. | |
void | SetView (const View &NewView) |
Change the current active view. | |
const View & | GetView () const |
Get the current view. | |
View & | GetDefaultView () |
Get the default view of the window for read / write. | |
void | PreserveOpenGLStates (bool Preserve) |
Tell SFML to preserve external OpenGL states, at the expense of more CPU charge. | |
Protected Member Functions | |
void | Initialize () |
Called by the derived class when it's ready to be initialized. |
Detailed Description
Simple wrapper for sf::Window that allows easy 2D rendering.Definition at line 45 of file RenderWindow.hpp.
Constructor & Destructor Documentation
sf::RenderWindow::RenderWindow | ( | ) |
sf::RenderWindow::RenderWindow | ( | VideoMode | Mode, | |
const std::string & | Title, | |||
unsigned long | WindowStyle = Style::Resize | Style::Close , |
|||
const WindowSettings & | Params = WindowSettings() | |||
) |
Construct the window.
- Parameters:
-
Mode : Video mode to use Title : Title of the window WindowStyle : Window style, see sf::Style (Resize | Close by default) Params : Creation parameters (see default constructor for default values)
Definition at line 49 of file RenderWindow.cpp.
sf::RenderWindow::RenderWindow | ( | WindowHandle | Handle, | |
const WindowSettings & | Params = WindowSettings() | |||
) |
Construct the window from an existing control.
- Parameters:
-
Handle : Platform-specific handle of the control Params : Creation parameters (see default constructor for default values)
Definition at line 58 of file RenderWindow.cpp.
sf::RenderWindow::~RenderWindow | ( | ) | [virtual] |
Member Function Documentation
Image sf::RenderWindow::Capture | ( | ) | const |
Save the content of the window to an image.
- Returns:
- Image instance containing the contents of the screen
Definition at line 107 of file RenderWindow.cpp.
Clear the entire target with a single color.
- Parameters:
-
FillColor : Color to use to clear the render target
Definition at line 60 of file RenderTarget.cpp.
void sf::Window::Close | ( | ) | [inherited] |
Close (destroy) the window.
The sf::Window instance remains valid and you can call Create to recreate the window
The sf::Window instance remains valid and you can call Create to recreate the window
Definition at line 165 of file Window.cpp.
sf::Vector2f sf::RenderWindow::ConvertCoords | ( | unsigned int | WindowX, | |
unsigned int | WindowY, | |||
const View * | TargetView = NULL | |||
) | const |
Convert a point in window coordinates into view coordinates.
- Parameters:
-
WindowX : X coordinate of the point to convert, relative to the window WindowY : Y coordinate of the point to convert, relative to the window TargetView : Target view to convert the point to (NULL by default -- uses the current view)
- Returns:
- Converted point
Definition at line 142 of file RenderWindow.cpp.
void sf::Window::Create | ( | WindowHandle | Handle, | |
const WindowSettings & | Params = WindowSettings() | |||
) | [inherited] |
Create (or recreate) the window from an existing control.
Create the window from an existing control.
- Parameters:
-
Handle : Platform-specific handle of the control Params : Creation parameters (see default constructor for default values)
Definition at line 147 of file Window.cpp.
void sf::Window::Create | ( | VideoMode | Mode, | |
const std::string & | Title, | |||
unsigned long | WindowStyle = Style::Resize | Style::Close , |
|||
const WindowSettings & | Params = WindowSettings() | |||
) | [inherited] |
Create (or recreate) the window.
Create the window.
- Parameters:
-
Mode : Video mode to use Title : Title of the window WindowStyle : Window style, see sf::Style (Resize | Close by default) Params : Creation parameters (see default constructor for default values)
Definition at line 104 of file Window.cpp.
void sf::Window::Display | ( | ) | [inherited] |
void sf::RenderTarget::Draw | ( | const Drawable & | Object | ) | [virtual, inherited] |
Draw something into the target.
Draw something on the window.
- Parameters:
-
Object : Object to draw
Definition at line 76 of file RenderTarget.cpp.
void sf::Window::EnableKeyRepeat | ( | bool | Enabled | ) | [inherited] |
Enable or disable automatic key-repeat.
Automatic key-repeat is enabled by default
- Parameters:
-
Enabled : True to enable, false to disable
Definition at line 317 of file Window.cpp.
View & sf::RenderTarget::GetDefaultView | ( | ) | [inherited] |
Get the default view of the window for read / write.
- Returns:
- Default view
Definition at line 147 of file RenderTarget.cpp.
bool sf::Window::GetEvent | ( | Event & | EventReceived | ) | [inherited] |
Get the event on top of events stack, if any, and pop it.
Get the event on top of events stack, if any.
- Parameters:
-
EventReceived : Event to fill, if any
- Returns:
- True if an event was returned, false if events stack was empty
Definition at line 218 of file Window.cpp.
float sf::Window::GetFrameTime | ( | ) | const [inherited] |
Get time elapsed since last frame.
- Returns:
- Time elapsed, in seconds
Definition at line 394 of file Window.cpp.
unsigned int sf::RenderWindow::GetHeight | ( | ) | const [virtual] |
Get the height of the rendering region of the window.
- Returns:
- Height in pixels
Implements sf::RenderTarget.
Definition at line 98 of file RenderWindow.cpp.
const Input & sf::Window::GetInput | ( | ) | const [inherited] |
Get the input manager of the window.
- Returns:
- Reference to the input
Definition at line 376 of file Window.cpp.
const WindowSettings & sf::Window::GetSettings | ( | ) | const [inherited] |
Get the creation settings of the window.
- Returns:
- Structure containing the creation settings
Definition at line 209 of file Window.cpp.
const View & sf::RenderTarget::GetView | ( | ) | const [inherited] |
Get the current view.
- Returns:
- Current view active in the window
Definition at line 138 of file RenderTarget.cpp.
unsigned int sf::RenderWindow::GetWidth | ( | ) | const [virtual] |
Get the width of the rendering region of the window.
- Returns:
- Width in pixels
Implements sf::RenderTarget.
Definition at line 89 of file RenderWindow.cpp.
void sf::RenderTarget::Initialize | ( | ) | [protected, inherited] |
Called by the derived class when it's ready to be initialized.
Definition at line 170 of file RenderTarget.cpp.
bool sf::Window::IsOpened | ( | ) | const [inherited] |
Tell whether or not the window is opened (ie.
has been created). Note that a hidden window (Show(false)) will still return true
- Returns:
- True if the window is opened
Definition at line 182 of file Window.cpp.
void sf::RenderTarget::PreserveOpenGLStates | ( | bool | Preserve | ) | [inherited] |
Tell SFML to preserve external OpenGL states, at the expense of more CPU charge.
Tell SFML to preserve external OpenGL states, at the expense of more CPU charge.
Use this function if you don't want SFML to mess up your own OpenGL states (if any). Don't enable state preservation if not needed, as it will allow SFML to do internal optimizations and improve performances. This parameter is false by default
- Parameters:
-
Preserve : True to preserve OpenGL states, false to let SFML optimize
Definition at line 161 of file RenderTarget.cpp.
bool sf::Window::SetActive | ( | bool | Active = true |
) | const [inherited] |
Activate of deactivate the window as the current target for rendering.
Activate of deactivate the window as the current target for rendering.
- Parameters:
-
Active : True to activate, false to deactivate (true by default)
- Returns:
- True if operation was successful, false otherwise
Definition at line 338 of file Window.cpp.
void sf::Window::SetCursorPosition | ( | unsigned int | Left, | |
unsigned int | Top | |||
) | [inherited] |
Change the position of the mouse cursor.
- Parameters:
-
Left : Left coordinate of the cursor, relative to the window Top : Top coordinate of the cursor, relative to the window
Definition at line 260 of file Window.cpp.
void sf::Window::SetFramerateLimit | ( | unsigned int | Limit | ) | [inherited] |
Limit the framerate to a maximum fixed frequency.
Set the framerate at a fixed frequency.
- Parameters:
-
Limit : Framerate limit, in frames per seconds (use 0 to disable limit)
Definition at line 385 of file Window.cpp.
void sf::Window::SetIcon | ( | unsigned int | Width, | |
unsigned int | Height, | |||
const Uint8 * | Pixels | |||
) | [inherited] |
Change the window's icon.
- Parameters:
-
Width : Icon's width, in pixels Height : Icon's height, in pixels Pixels : Pointer to the pixels in memory, format must be RGBA 32 bits
Definition at line 327 of file Window.cpp.
void sf::Window::SetJoystickThreshold | ( | float | Threshold | ) | [inherited] |
Change the joystick threshold, ie.
the value below which no move event will be generated
- Parameters:
-
Threshold : New threshold, in range [0, 100]
Definition at line 404 of file Window.cpp.
void sf::Window::SetPosition | ( | int | Left, | |
int | Top | |||
) | [inherited] |
Change the position of the window on screen.
Only works for top-level windows
- Parameters:
-
Left : Left position Top : Top position
Definition at line 276 of file Window.cpp.
void sf::Window::SetSize | ( | unsigned int | Width, | |
unsigned int | Height | |||
) | [inherited] |
Change the size of the rendering region of the window.
- Parameters:
-
Width : New width Height : New height
Definition at line 293 of file Window.cpp.
void sf::RenderTarget::SetView | ( | const View & | NewView | ) | [inherited] |
Change the current active view.
- Parameters:
-
NewView : New view to use (pass GetDefaultView() to set the default view)
Definition at line 129 of file RenderTarget.cpp.
void sf::Window::Show | ( | bool | State | ) | [inherited] |
Show or hide the window.
- Parameters:
-
State : True to show, false to hide
Definition at line 303 of file Window.cpp.
void sf::Window::ShowMouseCursor | ( | bool | Show | ) | [inherited] |
Show or hide the mouse cursor.
- Parameters:
-
Show : True to show, false to hide
Definition at line 250 of file Window.cpp.
void sf::Window::UseVerticalSync | ( | bool | Enabled | ) | [inherited] |
Enable / disable vertical synchronization.
- Parameters:
-
Enabled : True to enable v-sync, false to deactivate
Definition at line 240 of file Window.cpp.
The documentation for this class was generated from the following files:
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::