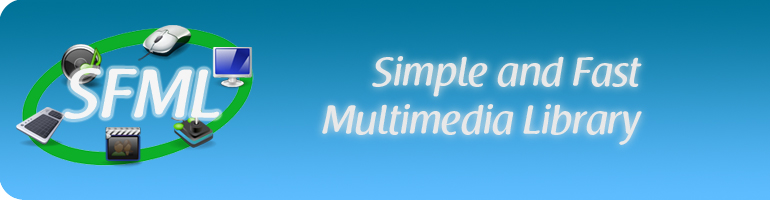
sf::IPAddress Class Reference
IPAddress provides easy manipulation of IP v4 addresses. More...
#include <IPAddress.hpp>
Public Member Functions | |
IPAddress () | |
Default constructor -- constructs an invalid address. | |
IPAddress (const std::string &Address) | |
Construct the address from a string. | |
IPAddress (const char *Address) | |
Construct the address from a C-style string ; Needed for implicit conversions from literal strings to IPAddress to work. | |
IPAddress (Uint8 Byte0, Uint8 Byte1, Uint8 Byte2, Uint8 Byte3) | |
Construct the address from 4 bytes. | |
IPAddress (Uint32 Address) | |
Construct the address from a 32-bits integer. | |
bool | IsValid () const |
Tell if the address is a valid one. | |
std::string | ToString () const |
Get a string representation of the address. | |
Uint32 | ToInteger () const |
Get an integer representation of the address. | |
bool | operator== (const IPAddress &Other) const |
Comparison operator ==. | |
bool | operator!= (const IPAddress &Other) const |
Comparison operator !=. | |
bool | operator< (const IPAddress &Other) const |
Comparison operator <. | |
bool | operator> (const IPAddress &Other) const |
Comparison operator >. | |
bool | operator<= (const IPAddress &Other) const |
Comparison operator <=. | |
bool | operator>= (const IPAddress &Other) const |
Comparison operator >=. | |
Static Public Member Functions | |
static IPAddress | GetLocalAddress () |
Get the computer's local IP address (from the LAN point of view). | |
static IPAddress | GetPublicAddress (float Timeout=0.f) |
Get the computer's public IP address (from the web point of view). | |
Static Public Attributes | |
static const IPAddress | LocalHost |
Local host address (to connect to the same computer). |
Detailed Description
IPAddress provides easy manipulation of IP v4 addresses.Definition at line 42 of file IPAddress.hpp.
Constructor & Destructor Documentation
sf::IPAddress::IPAddress | ( | ) |
Default constructor -- constructs an invalid address.
Default constructor.
Definition at line 45 of file IPAddress.cpp.
sf::IPAddress::IPAddress | ( | const std::string & | Address | ) |
Construct the address from a string.
- Parameters:
-
Address : IP address ("xxx.xxx.xxx.xxx") or network name
Definition at line 55 of file IPAddress.cpp.
sf::IPAddress::IPAddress | ( | const char * | Address | ) |
Construct the address from a C-style string ; Needed for implicit conversions from literal strings to IPAddress to work.
Construct the address from a C-style string ; Needed for implicit conversions from literal strings to IPAddress to work.
- Parameters:
-
Address : IP address ("xxx.xxx.xxx.xxx") or network name
Definition at line 82 of file IPAddress.cpp.
sf::IPAddress::IPAddress | ( | Uint8 | Byte0, | |
Uint8 | Byte1, | |||
Uint8 | Byte2, | |||
Uint8 | Byte3 | |||
) |
Construct the address from 4 bytes.
- Parameters:
-
Byte0 : First byte of the address Byte1 : Second byte of the address Byte2 : Third byte of the address Byte3 : Fourth byte of the address
Definition at line 108 of file IPAddress.cpp.
sf::IPAddress::IPAddress | ( | Uint32 | Address | ) |
Construct the address from a 32-bits integer.
- Parameters:
-
Address : 4 bytes of the address packed into a 32-bits integer
Definition at line 117 of file IPAddress.cpp.
Member Function Documentation
IPAddress sf::IPAddress::GetLocalAddress | ( | ) | [static] |
Get the computer's local IP address (from the LAN point of view).
- Returns:
- Local IP address
Definition at line 156 of file IPAddress.cpp.
IPAddress sf::IPAddress::GetPublicAddress | ( | float | Timeout = 0.f |
) | [static] |
Get the computer's public IP address (from the web point of view).
The only way to get a public address is to ask it to a distant website ; as a consequence, this function may be very slow -- use it as few as possible !
- Parameters:
-
Timeout : Maximum time to wait, in seconds (0 by default : no timeout)
- Returns:
- Public IP address
Definition at line 204 of file IPAddress.cpp.
bool sf::IPAddress::IsValid | ( | ) | const |
Tell if the address is a valid one.
- Returns:
- True if address has a valid syntax
Definition at line 126 of file IPAddress.cpp.
bool sf::IPAddress::operator!= | ( | const IPAddress & | Other | ) | const |
Comparison operator !=.
- Parameters:
-
Other : Address to compare
- Returns:
- True if *this != Other
Definition at line 235 of file IPAddress.cpp.
bool sf::IPAddress::operator< | ( | const IPAddress & | Other | ) | const |
Comparison operator <.
- Parameters:
-
Other : Address to compare
- Returns:
- True if *this < Other
Definition at line 244 of file IPAddress.cpp.
bool sf::IPAddress::operator<= | ( | const IPAddress & | Other | ) | const |
Comparison operator <=.
- Parameters:
-
Other : Address to compare
- Returns:
- True if *this <= Other
Definition at line 262 of file IPAddress.cpp.
bool sf::IPAddress::operator== | ( | const IPAddress & | Other | ) | const |
Comparison operator ==.
- Parameters:
-
Other : Address to compare
- Returns:
- True if *this == Other
Definition at line 226 of file IPAddress.cpp.
bool sf::IPAddress::operator> | ( | const IPAddress & | Other | ) | const |
Comparison operator >.
- Parameters:
-
Other : Address to compare
- Returns:
- True if *this > Other
Definition at line 253 of file IPAddress.cpp.
bool sf::IPAddress::operator>= | ( | const IPAddress & | Other | ) | const |
Comparison operator >=.
- Parameters:
-
Other : Address to compare
- Returns:
- True if *this >= Other
Definition at line 271 of file IPAddress.cpp.
Uint32 sf::IPAddress::ToInteger | ( | ) | const |
Get an integer representation of the address.
- Returns:
- 32-bits integer containing the 4 bytes of the address, in system endianness
Definition at line 147 of file IPAddress.cpp.
std::string sf::IPAddress::ToString | ( | ) | const |
Get a string representation of the address.
- Returns:
- String representation of the IP address ("xxx.xxx.xxx.xxx")
Definition at line 135 of file IPAddress.cpp.
Member Data Documentation
const IPAddress sf::IPAddress::LocalHost [static] |
Local host address (to connect to the same computer).
Static member data.
Definition at line 196 of file IPAddress.hpp.
The documentation for this class was generated from the following files:
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::