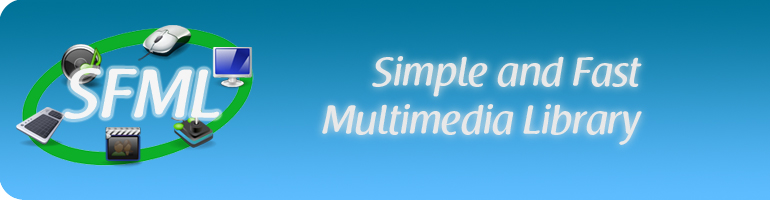
sf::SocketUDP Class Reference
SocketUDP wraps a socket using UDP protocol to send data fastly (but with less safety). More...
#include <SocketUDP.hpp>
Public Member Functions | |
SocketUDP () | |
Default constructor. | |
void | SetBlocking (bool Blocking) |
Change the blocking state of the socket. | |
bool | Bind (unsigned short Port) |
Bind the socket to a specific port. | |
bool | Unbind () |
Unbind the socket from its previous port, if any. | |
Socket::Status | Send (const char *Data, std::size_t Size, const IPAddress &Address, unsigned short Port) |
Send an array of bytes. | |
Socket::Status | Receive (char *Data, std::size_t MaxSize, std::size_t &SizeReceived, IPAddress &Address, unsigned short &Port) |
Receive an array of bytes. | |
Socket::Status | Send (Packet &PacketToSend, const IPAddress &Address, unsigned short Port) |
Send a packet of data. | |
Socket::Status | Receive (Packet &PacketToReceive, IPAddress &Address, unsigned short &Port) |
Receive a packet. | |
bool | Close () |
Close the socket. | |
bool | IsValid () const |
Check if the socket is in a valid state ; this function can be called any time to check if the socket is OK. | |
unsigned short | GetPort () const |
Get the port the socket is currently bound to. | |
bool | operator== (const SocketUDP &Other) const |
Comparison operator ==. | |
bool | operator!= (const SocketUDP &Other) const |
Comparison operator !=. | |
bool | operator< (const SocketUDP &Other) const |
Comparison operator <. | |
Friends | |
class | Selector< SocketUDP > |
Detailed Description
SocketUDP wraps a socket using UDP protocol to send data fastly (but with less safety).Definition at line 45 of file SocketUDP.hpp.
Constructor & Destructor Documentation
sf::SocketUDP::SocketUDP | ( | ) |
Member Function Documentation
bool sf::SocketUDP::Bind | ( | unsigned short | Port | ) |
Bind the socket to a specific port.
- Parameters:
-
Port : Port to bind the socket to
- Returns:
- True if operation has been successful
Definition at line 64 of file SocketUDP.cpp.
bool sf::SocketUDP::Close | ( | ) |
Close the socket.
- Returns:
- True if operation has been successful
Definition at line 316 of file SocketUDP.cpp.
unsigned short sf::SocketUDP::GetPort | ( | ) | const |
Get the port the socket is currently bound to.
- Returns:
- Current port (0 means the socket is not bound)
Definition at line 349 of file SocketUDP.cpp.
bool sf::SocketUDP::IsValid | ( | ) | const |
Check if the socket is in a valid state ; this function can be called any time to check if the socket is OK.
Check if the socket is in a valid state ; this function can be called any time to check if the socket is OK.
- Returns:
- True if the socket is valid
Definition at line 340 of file SocketUDP.cpp.
bool sf::SocketUDP::operator!= | ( | const SocketUDP & | Other | ) | const |
Comparison operator !=.
- Parameters:
-
Other : Socket to compare
- Returns:
- True if *this != Other
Definition at line 367 of file SocketUDP.cpp.
bool sf::SocketUDP::operator< | ( | const SocketUDP & | Other | ) | const |
Comparison operator <.
Provided for compatibility with standard containers, as comparing two sockets doesn't make much sense...
- Parameters:
-
Other : Socket to compare
- Returns:
- True if *this < Other
Definition at line 378 of file SocketUDP.cpp.
bool sf::SocketUDP::operator== | ( | const SocketUDP & | Other | ) | const |
Comparison operator ==.
- Parameters:
-
Other : Socket to compare
- Returns:
- True if *this == Other
Definition at line 358 of file SocketUDP.cpp.
Socket::Status sf::SocketUDP::Receive | ( | Packet & | PacketToReceive, | |
IPAddress & | Address, | |||
unsigned short & | Port | |||
) |
Receive a packet.
This function will block if the socket is blocking
- Parameters:
-
PacketToReceive : Packet to fill with received data Address : Address of the computer which sent the packet Port : Port on which the remote computer sent the data
- Returns:
- Status code
Definition at line 245 of file SocketUDP.cpp.
Socket::Status sf::SocketUDP::Receive | ( | char * | Data, | |
std::size_t | MaxSize, | |||
std::size_t & | SizeReceived, | |||
IPAddress & | Address, | |||
unsigned short & | Port | |||
) |
Receive an array of bytes.
This function will block if the socket is blocking
- Parameters:
-
Data : Pointer to a byte array to fill (make sure it is big enough) MaxSize : Maximum number of bytes to read SizeReceived : Number of bytes received Address : Address of the computer which sent the data Port : Port on which the remote computer sent the data
- Returns:
- Status code
Definition at line 162 of file SocketUDP.cpp.
Socket::Status sf::SocketUDP::Send | ( | Packet & | PacketToSend, | |
const IPAddress & | Address, | |||
unsigned short | Port | |||
) |
Send a packet of data.
- Parameters:
-
PacketToSend : Packet to send Address : Address of the computer to send the packet to Port : Port to send the data to
- Returns:
- Status code
Definition at line 219 of file SocketUDP.cpp.
Socket::Status sf::SocketUDP::Send | ( | const char * | Data, | |
std::size_t | Size, | |||
const IPAddress & | Address, | |||
unsigned short | Port | |||
) |
Send an array of bytes.
- Parameters:
-
Data : Pointer to the bytes to send Size : Number of bytes to send Address : Address of the computer to send the packet to Port : Port to send the data to
- Returns:
- Status code
Definition at line 118 of file SocketUDP.cpp.
void sf::SocketUDP::SetBlocking | ( | bool | Blocking | ) |
Change the blocking state of the socket.
The default behaviour of a socket is blocking
- Parameters:
-
Blocking : Pass true to set the socket as blocking, or false for non-blocking
Definition at line 50 of file SocketUDP.cpp.
bool sf::SocketUDP::Unbind | ( | ) |
Unbind the socket from its previous port, if any.
Unbind the socket to its previous port.
- Returns:
- True if operation has been successful
Definition at line 101 of file SocketUDP.cpp.
The documentation for this class was generated from the following files:
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::