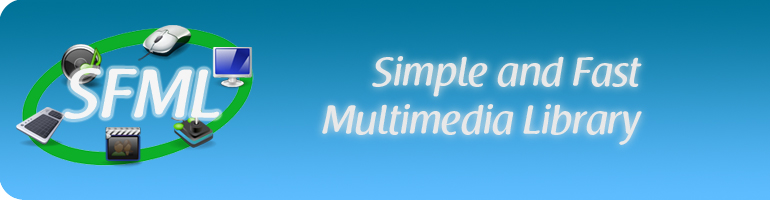
Drawable.cpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00026 // Headers 00028 #include <SFML/Graphics/Drawable.hpp> 00029 #include <SFML/Graphics/GraphicsContext.hpp> 00030 #include <SFML/Window/Window.hpp> 00031 #include <math.h> 00032 00033 00034 namespace sf 00035 { 00039 Drawable::Drawable(const Vector2f& Position, const Vector2f& Scale, float Rotation, const Color& Col) : 00040 myPosition (Position), 00041 myScale (Scale), 00042 myCenter (0, 0), 00043 myRotation (Rotation), 00044 myColor (Col), 00045 myBlendMode (Blend::Alpha), 00046 myNeedUpdate (true), 00047 myInvNeedUpdate(true) 00048 { 00049 00050 } 00051 00052 00056 Drawable::~Drawable() 00057 { 00058 // Nothing to do 00059 } 00060 00061 00065 void Drawable::SetPosition(float X, float Y) 00066 { 00067 SetX(X); 00068 SetY(Y); 00069 } 00070 00071 00075 void Drawable::SetPosition(const Vector2f& Position) 00076 { 00077 SetX(Position.x); 00078 SetY(Position.y); 00079 } 00080 00081 00085 void Drawable::SetX(float X) 00086 { 00087 myPosition.x = X; 00088 myNeedUpdate = true; 00089 myInvNeedUpdate = true; 00090 } 00091 00092 00096 void Drawable::SetY(float Y) 00097 { 00098 myPosition.y = Y; 00099 myNeedUpdate = true; 00100 myInvNeedUpdate = true; 00101 } 00102 00103 00107 void Drawable::SetScale(float ScaleX, float ScaleY) 00108 { 00109 SetScaleX(ScaleX); 00110 SetScaleY(ScaleY); 00111 } 00112 00113 00117 void Drawable::SetScale(const Vector2f& Scale) 00118 { 00119 SetScaleX(Scale.x); 00120 SetScaleY(Scale.y); 00121 } 00122 00123 00127 void Drawable::SetScaleX(float FactorX) 00128 { 00129 if (FactorX > 0) 00130 { 00131 myScale.x = FactorX; 00132 myNeedUpdate = true; 00133 myInvNeedUpdate = true; 00134 } 00135 } 00136 00137 00141 void Drawable::SetScaleY(float FactorY) 00142 { 00143 if (FactorY > 0) 00144 { 00145 myScale.y = FactorY; 00146 myNeedUpdate = true; 00147 myInvNeedUpdate = true; 00148 } 00149 } 00150 00151 00157 void Drawable::SetCenter(float CenterX, float CenterY) 00158 { 00159 myCenter.x = CenterX; 00160 myCenter.y = CenterY; 00161 myNeedUpdate = true; 00162 myInvNeedUpdate = true; 00163 } 00164 00165 00171 void Drawable::SetCenter(const Vector2f& Center) 00172 { 00173 SetCenter(Center.x, Center.y); 00174 } 00175 00176 00180 void Drawable::SetRotation(float Rotation) 00181 { 00182 myRotation = static_cast<float>(fmod(Rotation, 360)); 00183 if (myRotation < 0) 00184 myRotation += 360.f; 00185 myNeedUpdate = true; 00186 myInvNeedUpdate = true; 00187 } 00188 00189 00194 void Drawable::SetColor(const Color& Col) 00195 { 00196 myColor = Col; 00197 } 00198 00199 00204 void Drawable::SetBlendMode(Blend::Mode Mode) 00205 { 00206 myBlendMode = Mode; 00207 } 00208 00209 00213 const Vector2f& Drawable::GetPosition() const 00214 { 00215 return myPosition; 00216 } 00217 00218 00222 const Vector2f& Drawable::GetScale() const 00223 { 00224 return myScale; 00225 } 00226 00227 00231 const Vector2f& Drawable::GetCenter() const 00232 { 00233 return myCenter; 00234 } 00235 00236 00240 float Drawable::GetRotation() const 00241 { 00242 return myRotation; 00243 } 00244 00245 00249 const Color& Drawable::GetColor() const 00250 { 00251 return myColor; 00252 } 00253 00254 00258 Blend::Mode Drawable::GetBlendMode() const 00259 { 00260 return myBlendMode; 00261 } 00262 00263 00268 void Drawable::Move(float OffsetX, float OffsetY) 00269 { 00270 SetX(myPosition.x + OffsetX); 00271 SetY(myPosition.y + OffsetY); 00272 } 00273 00274 00278 void Drawable::Move(const Vector2f& Offset) 00279 { 00280 Move(Offset.x, Offset.y); 00281 } 00282 00283 00287 void Drawable::Scale(float FactorX, float FactorY) 00288 { 00289 SetScaleX(myScale.x * FactorX); 00290 SetScaleY(myScale.y * FactorY); 00291 } 00292 00293 00297 void Drawable::Scale(const Vector2f& Factor) 00298 { 00299 Scale(Factor.x, Factor.y); 00300 } 00301 00302 00306 void Drawable::Rotate(float Angle) 00307 { 00308 SetRotation(myRotation + Angle); 00309 } 00310 00311 00316 sf::Vector2f Drawable::TransformToLocal(const sf::Vector2f& Point) const 00317 { 00318 return GetInverseMatrix().Transform(Point); 00319 } 00320 00325 sf::Vector2f Drawable::TransformToGlobal(const sf::Vector2f& Point) const 00326 { 00327 return GetMatrix().Transform(Point); 00328 } 00329 00330 00334 const Matrix3& Drawable::GetMatrix() const 00335 { 00336 // First recompute it if needed 00337 if (myNeedUpdate) 00338 { 00339 myMatrix.SetFromTransformations(myCenter, myPosition, myRotation, myScale); 00340 myNeedUpdate = false; 00341 } 00342 00343 return myMatrix; 00344 } 00345 00346 00350 const Matrix3& Drawable::GetInverseMatrix() const 00351 { 00352 // First recompute it if needed 00353 if (myInvNeedUpdate) 00354 { 00355 myInvMatrix = GetMatrix().GetInverse(); 00356 myInvNeedUpdate = false; 00357 } 00358 00359 return myInvMatrix; 00360 } 00361 00362 00366 void Drawable::Draw(RenderTarget& Target) const 00367 { 00368 // Save the current modelview matrix and set the new one 00369 GLCheck(glMatrixMode(GL_MODELVIEW)); 00370 GLCheck(glPushMatrix()); 00371 GLCheck(glMultMatrixf(GetMatrix().Get4x4Elements())); 00372 00373 // Setup alpha-blending 00374 if (myBlendMode == Blend::None) 00375 { 00376 GLCheck(glDisable(GL_BLEND)); 00377 } 00378 else 00379 { 00380 GLCheck(glEnable(GL_BLEND)); 00381 00382 switch (myBlendMode) 00383 { 00384 case Blend::Alpha : GLCheck(glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA)); break; 00385 case Blend::Add : GLCheck(glBlendFunc(GL_SRC_ALPHA, GL_ONE)); break; 00386 case Blend::Multiply : GLCheck(glBlendFunc(GL_DST_COLOR, GL_ZERO)); break; 00387 default : break; 00388 } 00389 } 00390 00391 // Set color 00392 GLCheck(glColor4f(myColor.r / 255.f, myColor.g / 255.f, myColor.b / 255.f, myColor.a / 255.f)); 00393 00394 // Let the derived class render the object geometry 00395 Render(Target); 00396 00397 // Restore the previous modelview matrix 00398 GLCheck(glMatrixMode(GL_MODELVIEW)); 00399 GLCheck(glPopMatrix()); 00400 } 00401 00402 } // namespace sf
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::