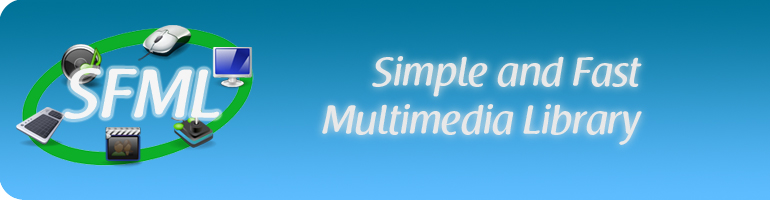
String.hpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00025 #ifndef SFML_STRING_HPP 00026 #define SFML_STRING_HPP 00027 00029 // Headers 00031 #include <SFML/System/Resource.hpp> 00032 #include <SFML/System/Unicode.hpp> 00033 #include <SFML/Graphics/Drawable.hpp> 00034 #include <SFML/Graphics/Font.hpp> 00035 #include <SFML/Graphics/Rect.hpp> 00036 #include <string> 00037 00038 00039 namespace sf 00040 { 00044 class SFML_API String : public Drawable 00045 { 00046 public : 00047 00051 enum Style 00052 { 00053 Regular = 0, 00054 Bold = 1 << 0, 00055 Italic = 1 << 1, 00056 Underlined = 1 << 2 00057 }; 00058 00063 String(); 00064 00073 explicit String(const Unicode::Text& Text, const Font& CharFont = Font::GetDefaultFont(), float Size = 30.f); 00074 00081 void SetText(const Unicode::Text& Text); 00082 00089 void SetFont(const Font& CharFont); 00090 00098 void SetSize(float Size); 00099 00107 void SetStyle(unsigned long TextStyle); 00108 00115 const Unicode::Text& GetText() const; 00116 00123 const Font& GetFont() const; 00124 00131 float GetSize() const; 00132 00139 unsigned long GetStyle() const; 00140 00151 sf::Vector2f GetCharacterPos(std::size_t Index) const; 00152 00159 FloatRect GetRect() const; 00160 00161 protected : 00162 00167 virtual void Render(RenderTarget& Target) const; 00168 00169 private : 00170 00175 void RecomputeRect(); 00176 00178 // Member data 00180 Unicode::Text myText; 00181 ResourcePtr<Font> myFont; 00182 float mySize; 00183 unsigned long myStyle; 00184 FloatRect myBaseRect; 00185 bool myNeedRectUpdate; 00186 }; 00187 00188 } // namespace sf 00189 00190 00191 #endif // SFML_STRING_HPP
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::