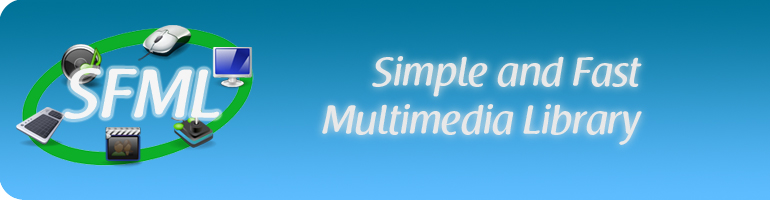
Sound.hpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00025 #ifndef SFML_SOUND_HPP 00026 #define SFML_SOUND_HPP 00027 00029 // Headers 00031 #include <SFML/System/Resource.hpp> 00032 #include <SFML/System/Vector3.hpp> 00033 #include <SFML/Audio/AudioResource.hpp> 00034 #include <cstdlib> 00035 00036 00037 namespace sf 00038 { 00039 class SoundBuffer; 00040 00045 class SFML_API Sound : public AudioResource 00046 { 00047 public : 00048 00052 enum Status 00053 { 00054 Stopped, 00055 Paused, 00056 Playing 00057 }; 00058 00063 Sound(); 00064 00075 explicit Sound(const SoundBuffer& Buffer, bool Loop = false, float Pitch = 1.f, float Volume = 100.f, const Vector3f& Position = Vector3f(0, 0, 0)); 00076 00083 Sound(const Sound& Copy); 00084 00089 ~Sound(); 00090 00095 void Play(); 00096 00101 void Pause(); 00102 00107 void Stop(); 00108 00115 void SetBuffer(const SoundBuffer& Buffer); 00116 00124 void SetLoop(bool Loop); 00125 00133 void SetPitch(float Pitch); 00134 00142 void SetVolume(float Volume); 00143 00151 void SetPosition(float X, float Y, float Z); 00152 00160 void SetPosition(const Vector3f& Position); 00161 00170 void SetRelativeToListener(bool Relative); 00171 00180 void SetMinDistance(float MinDistance); 00181 00190 void SetAttenuation(float Attenuation); 00191 00198 void SetPlayingOffset(float TimeOffset); 00199 00206 const SoundBuffer* GetBuffer() const; 00207 00214 bool GetLoop() const; 00215 00222 float GetPitch() const; 00223 00230 float GetVolume() const; 00231 00238 Vector3f GetPosition() const; 00239 00247 bool IsRelativeToListener() const; 00248 00255 float GetMinDistance() const; 00256 00263 float GetAttenuation() const; 00264 00271 Status GetStatus() const; 00272 00279 float GetPlayingOffset() const; 00280 00289 Sound& operator =(const Sound& Other); 00290 00298 void ResetBuffer(); 00299 00300 private : 00301 00302 friend class SoundStream; 00303 00305 // Member data 00307 unsigned int mySource; 00308 ResourcePtr<SoundBuffer> myBuffer; 00309 }; 00310 00311 } // namespace sf 00312 00313 00314 #endif // SFML_SOUND_HPP
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::