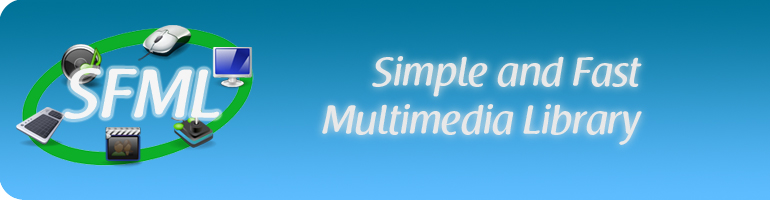
Music.cpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00026 // Headers 00028 #include <SFML/Audio/Music.hpp> 00029 #include <SFML/Audio/OpenAL.hpp> 00030 #include <SFML/Audio/SoundFile.hpp> 00031 #include <fstream> 00032 #include <iostream> 00033 00034 00035 namespace sf 00036 { 00040 Music::Music(std::size_t BufferSize) : 00041 myFile (NULL), 00042 myDuration(0.f), 00043 mySamples (BufferSize) 00044 { 00045 00046 } 00047 00048 00052 Music::~Music() 00053 { 00054 // We must stop before destroying the file :) 00055 Stop(); 00056 00057 delete myFile; 00058 } 00059 00060 00064 bool Music::OpenFromFile(const std::string& Filename) 00065 { 00066 // First stop the music if it was already running 00067 Stop(); 00068 00069 // Create the sound file implementation, and open it in read mode 00070 delete myFile; 00071 myFile = priv::SoundFile::CreateRead(Filename); 00072 if (!myFile) 00073 { 00074 std::cerr << "Failed to open \"" << Filename << "\" for reading" << std::endl; 00075 return false; 00076 } 00077 00078 // Compute the duration 00079 myDuration = static_cast<float>(myFile->GetSamplesCount()) / myFile->GetSampleRate() / myFile->GetChannelsCount(); 00080 00081 // Initialize the stream 00082 Initialize(myFile->GetChannelsCount(), myFile->GetSampleRate()); 00083 00084 return true; 00085 } 00086 00087 00091 bool Music::OpenFromMemory(const char* Data, std::size_t SizeInBytes) 00092 { 00093 // First stop the music if it was already running 00094 Stop(); 00095 00096 // Create the sound file implementation, and open it in read mode 00097 delete myFile; 00098 myFile = priv::SoundFile::CreateRead(Data, SizeInBytes); 00099 if (!myFile) 00100 { 00101 std::cerr << "Failed to open music from memory for reading" << std::endl; 00102 return false; 00103 } 00104 00105 // Compute the duration 00106 myDuration = static_cast<float>(myFile->GetSamplesCount()) / myFile->GetSampleRate(); 00107 00108 // Initialize the stream 00109 Initialize(myFile->GetChannelsCount(), myFile->GetSampleRate()); 00110 00111 return true; 00112 } 00113 00114 00118 bool Music::OnStart() 00119 { 00120 return myFile && myFile->Restart(); 00121 } 00122 00123 00127 bool Music::OnGetData(SoundStream::Chunk& Data) 00128 { 00129 if (myFile) 00130 { 00131 // Fill the chunk parameters 00132 Data.Samples = &mySamples[0]; 00133 Data.NbSamples = myFile->Read(&mySamples[0], mySamples.size()); 00134 00135 // Check if we have reached the end of the audio file 00136 return Data.NbSamples == mySamples.size(); 00137 } 00138 else 00139 { 00140 return false; 00141 } 00142 } 00143 00144 00148 float Music::GetDuration() const 00149 { 00150 return myDuration; 00151 } 00152 00153 } // namespace sf
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::