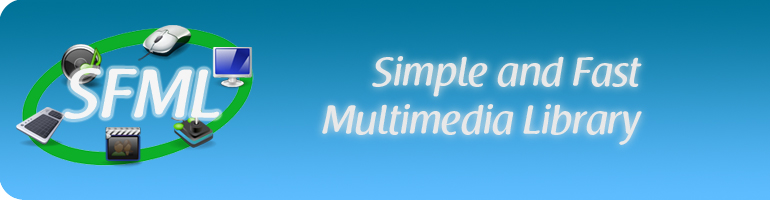
Vector3.inl
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00025 00029 template <typename T> 00030 Vector3<T>::Vector3() : 00031 x(0), 00032 y(0), 00033 z(0) 00034 { 00035 00036 } 00037 00038 00042 template <typename T> 00043 Vector3<T>::Vector3(T X, T Y, T Z) : 00044 x(X), 00045 y(Y), 00046 z(Z) 00047 { 00048 00049 } 00050 00051 00055 template <typename T> 00056 Vector3<T> operator -(const Vector3<T>& V) 00057 { 00058 return Vector3<T>(-V.x, -V.y, -V.z); 00059 } 00060 00061 00065 template <typename T> 00066 Vector3<T>& operator +=(Vector3<T>& V1, const Vector3<T>& V2) 00067 { 00068 V1.x += V2.x; 00069 V1.y += V2.y; 00070 V1.z += V2.z; 00071 00072 return V1; 00073 } 00074 00075 00079 template <typename T> 00080 Vector3<T>& operator -=(Vector3<T>& V1, const Vector3<T>& V2) 00081 { 00082 V1.x -= V2.x; 00083 V1.y -= V2.y; 00084 V1.z -= V2.z; 00085 00086 return V1; 00087 } 00088 00089 00093 template <typename T> 00094 Vector3<T> operator +(const Vector3<T>& V1, const Vector3<T>& V2) 00095 { 00096 return Vector3<T>(V1.x + V2.x, V1.y + V2.y, V1.z + V2.z); 00097 } 00098 00099 00103 template <typename T> 00104 Vector3<T> operator -(const Vector3<T>& V1, const Vector3<T>& V2) 00105 { 00106 return Vector3<T>(V1.x - V2.x, V1.y - V2.y, V1.z - V2.z); 00107 } 00108 00109 00113 template <typename T> 00114 Vector3<T> operator *(const Vector3<T>& V, T X) 00115 { 00116 return Vector3<T>(V.x * X, V.y * X, V.z * X); 00117 } 00118 00119 00123 template <typename T> 00124 Vector3<T> operator *(T X, const Vector3<T>& V) 00125 { 00126 return Vector3<T>(V.x * X, V.y * X, V.z * X); 00127 } 00128 00129 00133 template <typename T> 00134 Vector3<T>& operator *=(Vector3<T>& V, T X) 00135 { 00136 V.x *= X; 00137 V.y *= X; 00138 V.z *= X; 00139 00140 return V; 00141 } 00142 00143 00147 template <typename T> 00148 Vector3<T> operator /(const Vector3<T>& V, T X) 00149 { 00150 return Vector3<T>(V.x / X, V.y / X, V.z / X); 00151 } 00152 00153 00157 template <typename T> 00158 Vector3<T>& operator /=(Vector3<T>& V, T X) 00159 { 00160 V.x /= X; 00161 V.y /= X; 00162 V.z /= X; 00163 00164 return V; 00165 } 00166 00167 00171 template <typename T> 00172 bool operator ==(const Vector3<T>& V1, const Vector3<T>& V2) 00173 { 00174 return (V1.x == V2.x) && (V1.y == V2.y) && (V1.z == V2.z); 00175 } 00176 00177 00181 template <typename T> 00182 bool operator !=(const Vector3<T>& V1, const Vector3<T>& V2) 00183 { 00184 return (V1.x != V2.x) || (V1.y != V2.y) || (V1.z != V2.z); 00185 }
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::