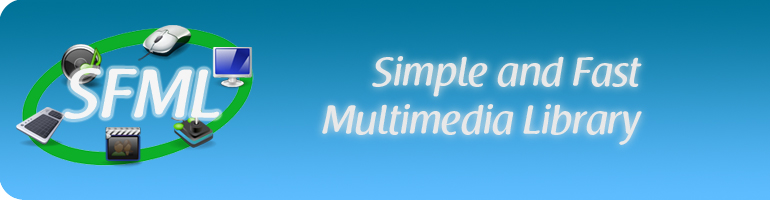
VideoModeSupport.cpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00026 // Headers 00028 #include <SFML/Window/Cocoa/VideoModeSupport.hpp> 00029 #include <ApplicationServices/ApplicationServices.h> 00030 #include <algorithm> 00031 00032 namespace sf 00033 { 00034 namespace priv 00035 { 00039 void VideoModeSupport::GetSupportedVideoModes(std::vector<VideoMode>& Modes) 00040 { 00041 // Ceylo -- using same implementation as in OSXCarbon 00042 00043 // First, clear array to fill 00044 Modes.clear(); 00045 00046 // Enumerate all available video modes for primary display adapter 00047 CFArrayRef DisplayModes = CGDisplayAvailableModes( kCGDirectMainDisplay ); 00048 CFIndex DisplayModeCount = CFArrayGetCount( DisplayModes ); 00049 CFDictionaryRef CurrentMode; 00050 00051 for (int Count = 0; Count < DisplayModeCount; ++Count) 00052 { 00053 CurrentMode = (CFDictionaryRef)CFArrayGetValueAtIndex( DisplayModes, Count ); 00054 00055 VideoMode Mode; 00056 00057 CFNumberGetValue((CFNumberRef)CFDictionaryGetValue(CurrentMode, kCGDisplayWidth), kCFNumberIntType, &(Mode.Width)); 00058 CFNumberGetValue((CFNumberRef)CFDictionaryGetValue(CurrentMode, kCGDisplayHeight), kCFNumberIntType, &(Mode.Height)); 00059 CFNumberGetValue((CFNumberRef)CFDictionaryGetValue(CurrentMode, kCGDisplayBitsPerPixel), kCFNumberIntType, &(Mode.BitsPerPixel)); 00060 00061 // Add it only if it is not already in the array 00062 if (std::find(Modes.begin(), Modes.end(), Mode) == Modes.end()) 00063 Modes.push_back(Mode); 00064 } 00065 } 00066 00067 00071 VideoMode VideoModeSupport::GetDesktopVideoMode() 00072 { 00073 // Ceylo -- using same implementation as in OSXCarbon 00074 00075 CFDictionaryRef CurrentVideoMode = CGDisplayCurrentMode(kCGDirectMainDisplay); 00076 00077 VideoMode DesktopMode; 00078 00079 00080 // Get video mode width 00081 CFNumberGetValue((CFNumberRef)CFDictionaryGetValue(CurrentVideoMode, kCGDisplayWidth), 00082 kCFNumberIntType, 00083 &(DesktopMode.Width)); 00084 00085 // Get video mode height 00086 CFNumberGetValue((CFNumberRef)CFDictionaryGetValue(CurrentVideoMode, kCGDisplayHeight), 00087 kCFNumberIntType, 00088 &(DesktopMode.Height)); 00089 00090 // Get video mode depth 00091 CFNumberGetValue((CFNumberRef)CFDictionaryGetValue(CurrentVideoMode, kCGDisplayBitsPerPixel), 00092 kCFNumberIntType, 00093 &(DesktopMode.BitsPerPixel)); 00094 00095 00096 return DesktopMode; 00097 } 00098 00099 } // namespace priv 00100 00101 } // namespace sf
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::