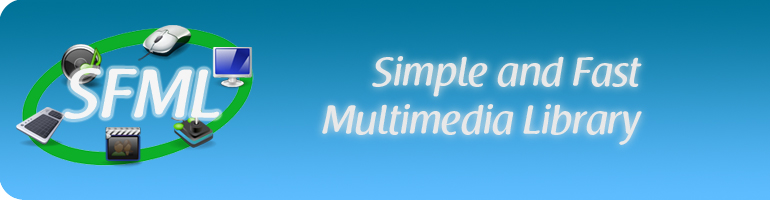
Vector2.inl
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00025 00029 template <typename T> 00030 Vector2<T>::Vector2() : 00031 x(0), 00032 y(0) 00033 { 00034 00035 } 00036 00037 00041 template <typename T> 00042 Vector2<T>::Vector2(T X, T Y) : 00043 x(X), 00044 y(Y) 00045 { 00046 00047 } 00048 00049 00053 template <typename T> 00054 Vector2<T> operator -(const Vector2<T>& V) 00055 { 00056 return Vector2<T>(-V.x, -V.y); 00057 } 00058 00059 00063 template <typename T> 00064 Vector2<T>& operator +=(Vector2<T>& V1, const Vector2<T>& V2) 00065 { 00066 V1.x += V2.x; 00067 V1.y += V2.y; 00068 00069 return V1; 00070 } 00071 00072 00076 template <typename T> 00077 Vector2<T>& operator -=(Vector2<T>& V1, const Vector2<T>& V2) 00078 { 00079 V1.x -= V2.x; 00080 V1.y -= V2.y; 00081 00082 return V1; 00083 } 00084 00085 00089 template <typename T> 00090 Vector2<T> operator +(const Vector2<T>& V1, const Vector2<T>& V2) 00091 { 00092 return Vector2<T>(V1.x + V2.x, V1.y + V2.y); 00093 } 00094 00095 00099 template <typename T> 00100 Vector2<T> operator -(const Vector2<T>& V1, const Vector2<T>& V2) 00101 { 00102 return Vector2<T>(V1.x - V2.x, V1.y - V2.y); 00103 } 00104 00105 00109 template <typename T> 00110 Vector2<T> operator *(const Vector2<T>& V, T X) 00111 { 00112 return Vector2<T>(V.x * X, V.y * X); 00113 } 00114 00115 00119 template <typename T> 00120 Vector2<T> operator *(T X, const Vector2<T>& V) 00121 { 00122 return Vector2<T>(V.x * X, V.y * X); 00123 } 00124 00125 00129 template <typename T> 00130 Vector2<T>& operator *=(Vector2<T>& V, T X) 00131 { 00132 V.x *= X; 00133 V.y *= X; 00134 00135 return V; 00136 } 00137 00138 00142 template <typename T> 00143 Vector2<T> operator /(const Vector2<T>& V, T X) 00144 { 00145 return Vector2<T>(V.x / X, V.y / X); 00146 } 00147 00148 00152 template <typename T> 00153 Vector2<T>& operator /=(Vector2<T>& V, T X) 00154 { 00155 V.x /= X; 00156 V.y /= X; 00157 00158 return V; 00159 } 00160 00161 00165 template <typename T> 00166 bool operator ==(const Vector2<T>& V1, const Vector2<T>& V2) 00167 { 00168 return (V1.x == V2.x) && (V1.y == V2.y); 00169 } 00170 00171 00175 template <typename T> 00176 bool operator !=(const Vector2<T>& V1, const Vector2<T>& V2) 00177 { 00178 return (V1.x != V2.x) || (V1.y != V2.y); 00179 }
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::