Reorderable List Control for Unity
Adding the drop-down add menu |
The drop-down add menu button is automatically displayed when there is at least one subscriber to the AddMenuClicked event. The presentation of the button varies depending upon whether the regular add button is also shown.
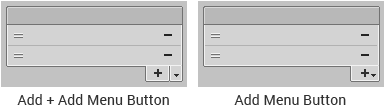
The drop-down add button is a general purpose button with no default behavior which can be used to display a menu or a drop-down window.
In this example a simple menu is constructed and shown upon clicking the add menu button:
using Rotorz.ReorderableList; using System.Collections.Generic; using UnityEditor; using UnityEngine; public class ExampleWindow : EditorWindow { private ReorderableListControl _listControl; private IReorderableListAdaptor _listAdaptor; private List<string> _someList = new List<string>(); private void OnEnable() { // Create list control and pass flag into constructor so that the // regular add button is not displayed. _listControl = new ReorderableListControl(ReorderableListFlags.HideAddButton); // Subscribe to event for when add menu button is clicked which will // also indicate that the add menu button is to be presented. _listControl.AddMenuClicked += OnAddMenuClicked; // Create adaptor for example list. _listAdaptor = new GenericListAdaptor<string>(_someList); } private void OnDisable() { // Unsubscribe from event, good practice. if (_listControl != null) _listControl.AddMenuClicked -= OnAddMenuClicked; } private void OnAddMenuClicked(object sender, AddMenuClickedEventArgs args) { var menu = new GenericMenu(); menu.AddItem(new GUIContent("Tree"), false, OnSelectAddMenuItem, "Tree"); menu.AddItem(new GUIContent("Bush"), false, OnSelectAddMenuItem, "Bush"); menu.AddItem(new GUIContent("Grass"), false, OnSelectAddMenuItem, "Grass"); menu.DropDown(args.ButtonPosition); } private void OnSelectAddMenuItem(object userData) { Debug.Log(userData); } private void OnGUI() { // Draw layout version of reorderable list control. _listControl.Draw(_listAdaptor); } }
#pragma strict import Rotorz.ReorderableList; import System.Collections.Generic; class ExampleWindow extends EditorWindow { var _listControl:ReorderableListControl; var _listAdaptor:IReorderableListAdaptor; var _someList:List.<String> = new List.<String>(); function OnEnable() { // Create list control and pass flag into constructor so that the // regular add button is not displayed. _listControl = new ReorderableListControl(ReorderableListFlags.HideAddButton); // Subscribe to event for when add menu button is clicked which will // also indicate that the add menu button is to be presented. _listControl.AddMenuClicked += OnAddMenuClicked; // Create adaptor for example list. _listAdaptor = new GenericListAdaptor.<String>(_someList); } function OnDisable() { // Unsubscribe from event, good practice. if (_listControl != null) _listControl.AddMenuClicked -= OnAddMenuClicked; } function OnAddMenuClicked(sender:object, args:AddMenuClickedEventArgs) { var menu = new GenericMenu(); menu.AddItem(new GUIContent('Tree'), false, OnSelectAddMenuItem, 'Tree'); menu.AddItem(new GUIContent('Bush'), false, OnSelectAddMenuItem, 'Bush'); menu.AddItem(new GUIContent('Grass'), false, OnSelectAddMenuItem, 'Grass'); menu.DropDown(args.ButtonPosition); } function OnSelectAddMenuItem(userData:object) { Debug.Log(userData); } function OnGUI() { // Draw layout version of reorderable list control. _listControl.Draw(_listAdaptor); } }