PxVehicleEngineData Class Reference
[Vehicle]
#include <PxVehicleComponents.h>
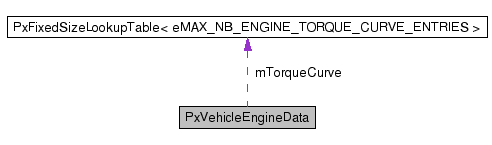
Public Types | |
enum | { eMAX_NB_ENGINE_TORQUE_CURVE_ENTRIES = 8 } |
Public Member Functions | |
PxVehicleEngineData () | |
PX_FORCE_INLINE PxReal | getRecipMOI () const |
Return value of mRecipMOI(=1.0f/mMOI) that is automatically set by PxVehicleDriveSimData::setEngineData. | |
PX_FORCE_INLINE PxReal | getRecipMaxOmega () const |
Return value of mRecipMaxOmega( = 1.0f / mMaxOmega ) that is automatically set by PxVehicleDriveSimData::setEngineData. | |
PxVehicleEngineData (const PxEMPTY) | |
Public Attributes | |
PxFixedSizeLookupTable < eMAX_NB_ENGINE_TORQUE_CURVE_ENTRIES > | mTorqueCurve |
Graph of normalized torque (torque/mPeakTorque) against normalized engine speed ( engineRotationSpeed / mMaxOmega ). | |
PxReal | mMOI |
Moment of inertia of the engine around the axis of rotation. | |
PxReal | mPeakTorque |
Maximum torque available to apply to the engine when the accelerator pedal is at maximum. | |
PxReal | mMaxOmega |
Maximum rotation speed of the engine. | |
PxReal | mDampingRateFullThrottle |
Damping rate of engine when full throttle is applied. | |
PxReal | mDampingRateZeroThrottleClutchEngaged |
Damping rate of engine when full throttle is applied. | |
PxReal | mDampingRateZeroThrottleClutchDisengaged |
Damping rate of engine when full throttle is applied. | |
Private Member Functions | |
bool | isValid () const |
Private Attributes | |
PxReal | mRecipMOI |
Reciprocal of the engine moment of inertia. | |
PxReal | mRecipMaxOmega |
Reciprocal of the maximum rotation speed of the engine. | |
Friends | |
class | PxVehicleDriveSimData |
Member Enumeration Documentation
Constructor & Destructor Documentation
PxVehicleEngineData::PxVehicleEngineData | ( | ) | [inline] |
PxVehicleEngineData::PxVehicleEngineData | ( | const | PxEMPTY | ) | [inline] |
Member Function Documentation
PX_FORCE_INLINE PxReal PxVehicleEngineData::getRecipMaxOmega | ( | ) | const [inline] |
Return value of mRecipMaxOmega( = 1.0f / mMaxOmega ) that is automatically set by PxVehicleDriveSimData::setEngineData.
PX_FORCE_INLINE PxReal PxVehicleEngineData::getRecipMOI | ( | ) | const [inline] |
Return value of mRecipMOI(=1.0f/mMOI) that is automatically set by PxVehicleDriveSimData::setEngineData.
bool PxVehicleEngineData::isValid | ( | ) | const [private] |
Friends And Related Function Documentation
friend class PxVehicleDriveSimData [friend] |
Member Data Documentation
Damping rate of engine when full throttle is applied.
- Note:
- If the clutch is engaged (any gear except neutral) then the damping rate applied at run-time is an interpolation between mDampingRateZeroThrottleClutchEngaged and mDampingRateFullThrottle: mDampingRateZeroThrottleClutchEngaged + (mDampingRateFullThrottle-mDampingRateZeroThrottleClutchEngaged)*acceleratorPedal;
If the clutch is disengaged (in neutral gear) the damping rate applied at run-time is an interpolation between mDampingRateZeroThrottleClutchDisengaged and mDampingRateFullThrottle: mDampingRateZeroThrottleClutchDisengaged + (mDampingRateFullThrottle-mDampingRateZeroThrottleClutchDisengaged)*acceleratorPedal;
Specified in kilograms metres-squared per second (kg m^2 s^-1).
Damping rate of engine when full throttle is applied.
- Note:
- If the clutch is engaged (any gear except neutral) then the damping rate applied at run-time is an interpolation between mDampingRateZeroThrottleClutchEngaged and mDampingRateFullThrottle: mDampingRateZeroThrottleClutchEngaged + (mDampingRateFullThrottle-mDampingRateZeroThrottleClutchEngaged)*acceleratorPedal;
If the clutch is disengaged (in neutral gear) the damping rate applied at run-time is an interpolation between mDampingRateZeroThrottleClutchDisengaged and mDampingRateFullThrottle: mDampingRateZeroThrottleClutchDisengaged + (mDampingRateFullThrottle-mDampingRateZeroThrottleClutchDisengaged)*acceleratorPedal;
Specified in kilograms metres-squared per second (kg m^2 s^-1).
Damping rate of engine when full throttle is applied.
- Note:
- If the clutch is engaged (any gear except neutral) then the damping rate applied at run-time is an interpolation between mDampingRateZeroThrottleClutchEngaged and mDampingRateFullThrottle: mDampingRateZeroThrottleClutchEngaged + (mDampingRateFullThrottle-mDampingRateZeroThrottleClutchEngaged)*acceleratorPedal;
If the clutch is disengaged (in neutral gear) the damping rate applied at run-time is an interpolation between mDampingRateZeroThrottleClutchDisengaged and mDampingRateFullThrottle: mDampingRateZeroThrottleClutchDisengaged + (mDampingRateFullThrottle-mDampingRateZeroThrottleClutchDisengaged)*acceleratorPedal;
Specified in kilograms metres-squared per second (kg m^2 s^-1).
Maximum rotation speed of the engine.
- Note:
- Specified in radians per second (s^-1).
PxReal PxVehicleEngineData::mMOI |
Moment of inertia of the engine around the axis of rotation.
- Note:
- Specified in kilograms metres-squared (kg m^2)
Maximum torque available to apply to the engine when the accelerator pedal is at maximum.
- Note:
- The torque available is the value of the accelerator pedal (in range [0, 1]) multiplied by the normalized torque as computed from mTorqueCurve multiplied by mPeakTorque.
Specified in kilograms metres-squared per second-squared (kg m^2 s^-2).
PxReal PxVehicleEngineData::mRecipMaxOmega [private] |
Reciprocal of the maximum rotation speed of the engine.
- Note:
- Not necessary to set this value because it is set by PxVehicleDriveSimData::setEngineData
PxReal PxVehicleEngineData::mRecipMOI [private] |
Reciprocal of the engine moment of inertia.
- Note:
- Not necessary to set this value because it is set by PxVehicleDriveSimData::setEngineData
PxFixedSizeLookupTable<eMAX_NB_ENGINE_TORQUE_CURVE_ENTRIES> PxVehicleEngineData::mTorqueCurve |
Graph of normalized torque (torque/mPeakTorque) against normalized engine speed ( engineRotationSpeed / mMaxOmega ).
- Note:
- The normalized engine speed is the x-axis of the graph, while the normalized torque is the y-axis of the graph.
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com