PxVehicleDrive4WRawInputData Class Reference
[Vehicle]
Used to produce smooth vehicle driving control values from analog and digital inputs.
More...
#include <PxVehicleUtilControl.h>
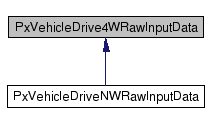
Public Member Functions | |
PxVehicleDrive4WRawInputData () | |
virtual | ~PxVehicleDrive4WRawInputData () |
void | setDigitalAccel (const bool accelKeyPressed) |
Record if the accel button has been pressed on keyboard. | |
void | setDigitalBrake (const bool brakeKeyPressed) |
Record if the brake button has been pressed on keyboard. | |
void | setDigitalHandbrake (const bool handbrakeKeyPressed) |
Record if the handbrake button has been pressed on keyboard. | |
void | setDigitalSteerLeft (const bool steerLeftKeyPressed) |
Record if the left steer button has been pressed on keyboard. | |
void | setDigitalSteerRight (const bool steerRightKeyPressed) |
Record if the right steer button has been pressed on keyboard. | |
bool | getDigitalAccel () const |
Return if the accel button has been pressed on keyboard. | |
bool | getDigitalBrake () const |
Return if the brake button has been pressed on keyboard. | |
bool | getDigitalHandbrake () const |
Return if the handbrake button has been pressed on keyboard. | |
bool | getDigitalSteerLeft () const |
Return if the left steer button has been pressed on keyboard. | |
bool | getDigitalSteerRight () const |
Return if the right steer button has been pressed on keyboard. | |
void | setAnalogAccel (const PxReal accel) |
Set the analog accel value from the gamepad. | |
void | setAnalogBrake (const PxReal brake) |
Set the analog brake value from the gamepad. | |
void | setAnalogHandbrake (const PxReal handbrake) |
Set the analog handbrake value from the gamepad. | |
void | setAnalogSteer (const PxReal steer) |
Set the analog steer value from the gamepad. | |
PxReal | getAnalogAccel () const |
Return the analog accel value from the gamepad. | |
PxReal | getAnalogBrake () const |
Return the analog brake value from the gamepad. | |
PxReal | getAnalogHandbrake () const |
Return the analog handbrake value from the gamepad. | |
PxReal | getAnalogSteer () const |
Return the analog steer value from the gamepad. | |
void | setGearUp (const bool gearUpKeyPressed) |
Record if the gearup button has been pressed on keyboard or gamepad. | |
void | setGearDown (const bool gearDownKeyPressed) |
Record if the geardown button has been pressed on keyboard or gamepad. | |
bool | getGearUp () const |
Return if the gearup button has been pressed on keyboard or gamepad. | |
bool | getGearDown () const |
Record if the geardown button has been pressed on keyboard or gamepad. | |
Private Attributes | |
bool | mRawDigitalInputs [PxVehicleDrive4WControl::eMAX_NB_DRIVE4W_ANALOG_INPUTS] |
PxReal | mRawAnalogInputs [PxVehicleDrive4WControl::eMAX_NB_DRIVE4W_ANALOG_INPUTS] |
bool | mGearUp |
bool | mGearDown |
Detailed Description
Used to produce smooth vehicle driving control values from analog and digital inputs.
- See also:
- PxVehicleDrive4WSmoothDigitalRawInputsAndSetAnalogInputs, PxVehicleDrive4WSmoothAnalogRawInputsAndSetAnalogInputs
Constructor & Destructor Documentation
PxVehicleDrive4WRawInputData::PxVehicleDrive4WRawInputData | ( | ) | [inline] |
virtual PxVehicleDrive4WRawInputData::~PxVehicleDrive4WRawInputData | ( | ) | [inline, virtual] |
Member Function Documentation
PxReal PxVehicleDrive4WRawInputData::getAnalogAccel | ( | ) | const [inline] |
Return the analog accel value from the gamepad.
- Returns:
- The analog accel value.
References PxVehicleDrive4WControl::eANALOG_INPUT_ACCEL.
PxReal PxVehicleDrive4WRawInputData::getAnalogBrake | ( | ) | const [inline] |
Return the analog brake value from the gamepad.
- Returns:
- The analog brake value.
References PxVehicleDrive4WControl::eANALOG_INPUT_BRAKE.
PxReal PxVehicleDrive4WRawInputData::getAnalogHandbrake | ( | ) | const [inline] |
Return the analog handbrake value from the gamepad.
- Returns:
- The analog handbrake value.
References PxVehicleDrive4WControl::eANALOG_INPUT_HANDBRAKE.
PxReal PxVehicleDrive4WRawInputData::getAnalogSteer | ( | ) | const [inline] |
Return the analog steer value from the gamepad.
References PxVehicleDrive4WControl::eANALOG_INPUT_STEER_RIGHT.
bool PxVehicleDrive4WRawInputData::getDigitalAccel | ( | ) | const [inline] |
Return if the accel button has been pressed on keyboard.
- Returns:
- True if the accel button has been pressed, false otherwise.
References PxVehicleDrive4WControl::eANALOG_INPUT_ACCEL.
bool PxVehicleDrive4WRawInputData::getDigitalBrake | ( | ) | const [inline] |
Return if the brake button has been pressed on keyboard.
- Returns:
- True if the brake button has been pressed, false otherwise.
References PxVehicleDrive4WControl::eANALOG_INPUT_BRAKE.
bool PxVehicleDrive4WRawInputData::getDigitalHandbrake | ( | ) | const [inline] |
Return if the handbrake button has been pressed on keyboard.
- Returns:
- True if the handbrake button has been pressed, false otherwise.
References PxVehicleDrive4WControl::eANALOG_INPUT_HANDBRAKE.
bool PxVehicleDrive4WRawInputData::getDigitalSteerLeft | ( | ) | const [inline] |
Return if the left steer button has been pressed on keyboard.
- Returns:
- True if the steer-left button has been pressed, false otherwise.
References PxVehicleDrive4WControl::eANALOG_INPUT_STEER_LEFT.
bool PxVehicleDrive4WRawInputData::getDigitalSteerRight | ( | ) | const [inline] |
Return if the right steer button has been pressed on keyboard.
- Returns:
- True if the steer-right button has been pressed, false otherwise.
References PxVehicleDrive4WControl::eANALOG_INPUT_STEER_RIGHT.
bool PxVehicleDrive4WRawInputData::getGearDown | ( | ) | const [inline] |
Record if the geardown button has been pressed on keyboard or gamepad.
- Returns:
- The value of the gear-down button.
bool PxVehicleDrive4WRawInputData::getGearUp | ( | ) | const [inline] |
Return if the gearup button has been pressed on keyboard or gamepad.
- Returns:
- The value of the gear-up button.
void PxVehicleDrive4WRawInputData::setAnalogAccel | ( | const PxReal | accel | ) | [inline] |
Set the analog accel value from the gamepad.
- Parameters:
-
[in] accel is the analog accelerator pedal value in range(0,1) where 1 represents the pedal fully pressed and 0 represents the pedal in its rest state.
References PxVehicleDrive4WControl::eANALOG_INPUT_ACCEL.
void PxVehicleDrive4WRawInputData::setAnalogBrake | ( | const PxReal | brake | ) | [inline] |
Set the analog brake value from the gamepad.
- Parameters:
-
[in] brake is the analog brake pedal value in range(0,1) where 1 represents the pedal fully pressed and 0 represents the pedal in its rest state.
References PxVehicleDrive4WControl::eANALOG_INPUT_BRAKE.
void PxVehicleDrive4WRawInputData::setAnalogHandbrake | ( | const PxReal | handbrake | ) | [inline] |
Set the analog handbrake value from the gamepad.
- Parameters:
-
[in] handbrake is the analog handbrake value in range(0,1) where 1 represents the handbrake fully engaged and 0 represents the handbrake in its rest state.
References PxVehicleDrive4WControl::eANALOG_INPUT_HANDBRAKE.
void PxVehicleDrive4WRawInputData::setAnalogSteer | ( | const PxReal | steer | ) | [inline] |
Set the analog steer value from the gamepad.
- Parameters:
-
[in] steer is the analog steer value in range(-1,1) where -1 represents the steering wheel at left lock and +1 represents the steering wheel at right lock.
References PxVehicleDrive4WControl::eANALOG_INPUT_STEER_RIGHT.
void PxVehicleDrive4WRawInputData::setDigitalAccel | ( | const bool | accelKeyPressed | ) | [inline] |
Record if the accel button has been pressed on keyboard.
- Parameters:
-
[in] accelKeyPressed is true if the accelerator key has been pressed and false otherwise.
References PxVehicleDrive4WControl::eANALOG_INPUT_ACCEL.
void PxVehicleDrive4WRawInputData::setDigitalBrake | ( | const bool | brakeKeyPressed | ) | [inline] |
Record if the brake button has been pressed on keyboard.
- Parameters:
-
[in] brakeKeyPressed is true if the brake key has been pressed and false otherwise.
References PxVehicleDrive4WControl::eANALOG_INPUT_BRAKE.
void PxVehicleDrive4WRawInputData::setDigitalHandbrake | ( | const bool | handbrakeKeyPressed | ) | [inline] |
Record if the handbrake button has been pressed on keyboard.
- Parameters:
-
[in] handbrakeKeyPressed is true if the handbrake key has been pressed and false otherwise.
References PxVehicleDrive4WControl::eANALOG_INPUT_HANDBRAKE.
void PxVehicleDrive4WRawInputData::setDigitalSteerLeft | ( | const bool | steerLeftKeyPressed | ) | [inline] |
Record if the left steer button has been pressed on keyboard.
- Parameters:
-
[in] steerLeftKeyPressed is true if the steer-left key has been pressed and false otherwise.
References PxVehicleDrive4WControl::eANALOG_INPUT_STEER_LEFT.
void PxVehicleDrive4WRawInputData::setDigitalSteerRight | ( | const bool | steerRightKeyPressed | ) | [inline] |
Record if the right steer button has been pressed on keyboard.
- Parameters:
-
[in] steerRightKeyPressed is true if the steer-right key has been pressed and false otherwise.
References PxVehicleDrive4WControl::eANALOG_INPUT_STEER_RIGHT.
void PxVehicleDrive4WRawInputData::setGearDown | ( | const bool | gearDownKeyPressed | ) | [inline] |
Record if the geardown button has been pressed on keyboard or gamepad.
- Parameters:
-
[in] gearDownKeyPressed is true if the gear-down button has been pressed, false otherwise.
void PxVehicleDrive4WRawInputData::setGearUp | ( | const bool | gearUpKeyPressed | ) | [inline] |
Record if the gearup button has been pressed on keyboard or gamepad.
- Parameters:
-
[in] gearUpKeyPressed is true if the gear-up button has been pressed, false otherwise.
Member Data Documentation
bool PxVehicleDrive4WRawInputData::mGearDown [private] |
bool PxVehicleDrive4WRawInputData::mGearUp [private] |
PxReal PxVehicleDrive4WRawInputData::mRawAnalogInputs[PxVehicleDrive4WControl::eMAX_NB_DRIVE4W_ANALOG_INPUTS] [private] |
bool PxVehicleDrive4WRawInputData::mRawDigitalInputs[PxVehicleDrive4WControl::eMAX_NB_DRIVE4W_ANALOG_INPUTS] [private] |
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com