PxVehicleDrivableSurfaceToTireFrictionPairs Class Reference
[Vehicle]
Friction for each combination of driving surface type and tire type.
More...
#include <PxVehicleTireFriction.h>
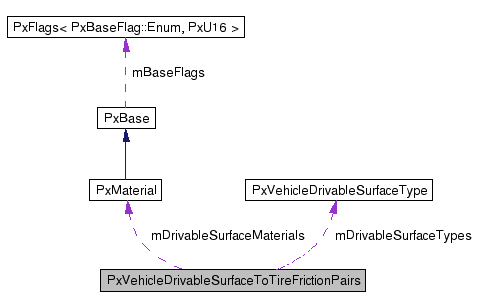
Public Types | |
enum | { eMAX_NB_SURFACE_TYPES = 256 } |
Public Member Functions | |
void | setup (const PxU32 nbTireTypes, const PxU32 nbSurfaceTypes, const PxMaterial **drivableSurfaceMaterials, const PxVehicleDrivableSurfaceType *drivableSurfaceTypes) |
Set up a PxVehicleDrivableSurfaceToTireFrictionPairs instance for combinations of nbTireTypes tire types and nbSurfaceTypes surface types. | |
void | release () |
Deallocate a PxVehicleDrivableSurfaceToTireFrictionPairs instance. | |
void | setTypePairFriction (const PxU32 surfaceType, const PxU32 tireType, const PxReal value) |
Set the friction for a specified pair of tire type and drivable surface type. | |
PxReal | getTypePairFriction (const PxU32 surfaceType, const PxU32 tireType) const |
Return the friction for a specified combination of surface type and tire type. | |
PxU32 | getMaxNbSurfaceTypes () const |
Return the maximum number of surface types. | |
PxU32 | getMaxNbTireTypes () const |
Return the maximum number of tire types. | |
Static Public Member Functions | |
static PxVehicleDrivableSurfaceToTireFrictionPairs * | allocate (const PxU32 maxNbTireTypes, const PxU32 maxNbSurfaceTypes) |
Allocate the memory for a PxVehicleDrivableSurfaceToTireFrictionPairs instance that can hold data for combinations of tire type and surface type with up to maxNbTireTypes types of tire and maxNbSurfaceTypes types of surface. | |
Private Member Functions | |
PxVehicleDrivableSurfaceToTireFrictionPairs () | |
~PxVehicleDrivableSurfaceToTireFrictionPairs () | |
Private Attributes | |
PxReal * | mPairs |
Ptr to base address of a 2d PxReal array with dimensions [mNbSurfaceTypes][mNbTireTypes]. | |
const PxMaterial ** | mDrivableSurfaceMaterials |
Ptr to 1d array of material ptrs that is of length mNbSurfaceTypes. | |
PxVehicleDrivableSurfaceType * | mDrivableSurfaceTypes |
Ptr to 1d array of PxVehicleDrivableSurfaceType that is of length mNbSurfaceTypes. | |
PxU32 | mNbSurfaceTypes |
Number of different driving surface types. | |
PxU32 | mMaxNbSurfaceTypes |
Maximum number of different driving surface types. | |
PxU32 | mNbTireTypes |
Number of different tire types. | |
PxU32 | mMaxNbTireTypes |
Maximum number of different tire types. | |
PxU32 | mPad [1] |
Friends | |
class | VehicleSurfaceTypeHashTable |
Detailed Description
Friction for each combination of driving surface type and tire type.
Member Enumeration Documentation
Constructor & Destructor Documentation
PxVehicleDrivableSurfaceToTireFrictionPairs::PxVehicleDrivableSurfaceToTireFrictionPairs | ( | ) | [inline, private] |
PxVehicleDrivableSurfaceToTireFrictionPairs::~PxVehicleDrivableSurfaceToTireFrictionPairs | ( | ) | [inline, private] |
Member Function Documentation
static PxVehicleDrivableSurfaceToTireFrictionPairs* PxVehicleDrivableSurfaceToTireFrictionPairs::allocate | ( | const PxU32 | maxNbTireTypes, | |
const PxU32 | maxNbSurfaceTypes | |||
) | [static] |
Allocate the memory for a PxVehicleDrivableSurfaceToTireFrictionPairs instance that can hold data for combinations of tire type and surface type with up to maxNbTireTypes types of tire and maxNbSurfaceTypes types of surface.
- Parameters:
-
[in] maxNbTireTypes is the maximum number of allowed tire types. [in] maxNbSurfaceTypes is the maximum number of allowed surface types. Must be less than or equal to eMAX_NB_SURFACE_TYPES
- Returns:
- a PxVehicleDrivableSurfaceToTireFrictionPairs instance that can be reused later with new type and friction data.
- See also:
- setup
PxU32 PxVehicleDrivableSurfaceToTireFrictionPairs::getMaxNbSurfaceTypes | ( | ) | const [inline] |
Return the maximum number of surface types.
- Returns:
- The maximum number of surface types
- See also:
- allocate
PxU32 PxVehicleDrivableSurfaceToTireFrictionPairs::getMaxNbTireTypes | ( | ) | const [inline] |
PxReal PxVehicleDrivableSurfaceToTireFrictionPairs::getTypePairFriction | ( | const PxU32 | surfaceType, | |
const PxU32 | tireType | |||
) | const |
Return the friction for a specified combination of surface type and tire type.
- Returns:
- The friction for a specified combination of surface type and tire type.
- Note:
- The final friction value used by the tire model is the value returned by getTypePairFriction multiplied by the value computed from PxVehicleTireData::mFrictionVsSlipGraph
void PxVehicleDrivableSurfaceToTireFrictionPairs::release | ( | ) |
Deallocate a PxVehicleDrivableSurfaceToTireFrictionPairs instance.
void PxVehicleDrivableSurfaceToTireFrictionPairs::setTypePairFriction | ( | const PxU32 | surfaceType, | |
const PxU32 | tireType, | |||
const PxReal | value | |||
) |
Set the friction for a specified pair of tire type and drivable surface type.
- Parameters:
-
[in] surfaceType describes the surface type [in] tireType describes the tire type. [in] value describes the friction coefficient for the combination of surface type and tire type.
void PxVehicleDrivableSurfaceToTireFrictionPairs::setup | ( | const PxU32 | nbTireTypes, | |
const PxU32 | nbSurfaceTypes, | |||
const PxMaterial ** | drivableSurfaceMaterials, | |||
const PxVehicleDrivableSurfaceType * | drivableSurfaceTypes | |||
) |
Set up a PxVehicleDrivableSurfaceToTireFrictionPairs instance for combinations of nbTireTypes tire types and nbSurfaceTypes surface types.
- Parameters:
-
[in] nbTireTypes is the number of different types of tire. This value must be less than or equal to maxNbTireTypes specified in allocate(). [in] nbSurfaceTypes is the number of different types of surface. This value must be less than or equal to maxNbSurfaceTypes specified in allocate(). [in] drivableSurfaceMaterials is an array of PxMaterial pointers of length nbSurfaceTypes. [in] drivableSurfaceTypes is an array of PxVehicleDrivableSurfaceType instances of length nbSurfaceTypes.
- Note:
- If the pointer to the PxMaterial that touches the tire is found in drivableSurfaceMaterials[x] then the surface type is drivableSurfaceTypes[x].mType and the friction is the value that is set with setTypePairFriction(drivableSurfaceTypes[x].mType, PxVehicleTireData::mType, frictionValue).
A friction value of 1.0 will be assigned as default to each combination of tire and surface type. To override this use setTypePairFriction.
Friends And Related Function Documentation
friend class VehicleSurfaceTypeHashTable [friend] |
Member Data Documentation
Ptr to 1d array of material ptrs that is of length mNbSurfaceTypes.
- Note:
- If the PxMaterial that touches the tire corresponds to mDrivableSurfaceMaterials[x] then the drivable surface type is mDrivableSurfaceTypes[x].mType and the friction for that contact is mPairs[mDrivableSurfaceTypes[x].mType][y], assuming a tire type y.
If the PxMaterial that touches the tire is not found in mDrivableSurfaceMaterials then the friction is mPairs[0][y], assuming a tire type y.
PxVehicleDrivableSurfaceType* PxVehicleDrivableSurfaceToTireFrictionPairs::mDrivableSurfaceTypes [private] |
Ptr to 1d array of PxVehicleDrivableSurfaceType that is of length mNbSurfaceTypes.
- Note:
- If the PxMaterial that touches the tire is found in mDrivableSurfaceMaterials[x] then the drivable surface type is mDrivableSurfaceTypes[x].mType and the friction for that contact is mPairs[mDrivableSurfaceTypes[x].mType][y], assuming a tire type y.
If the PxMaterial that touches the tire is not found in mDrivableSurfaceMaterials then the friction is mPairs[0][y], assuming a tire type y.
Maximum number of different driving surface types.
- Note:
- mMaxNbSurfaceTypes must be less than or equal to eMAX_NB_SURFACE_TYPES.
Number of different driving surface types.
- Note:
- mDrivableSurfaceMaterials and mDrivableSurfaceTypes are both 1d arrays of length mMaxNbSurfaceTypes.
mNbSurfaceTypes must be less than or equal to mMaxNbSurfaceTypes.
PxU32 PxVehicleDrivableSurfaceToTireFrictionPairs::mPad[1] [private] |
PxReal* PxVehicleDrivableSurfaceToTireFrictionPairs::mPairs [private] |
Ptr to base address of a 2d PxReal array with dimensions [mNbSurfaceTypes][mNbTireTypes].
- Note:
- Each element of the array describes the maximum friction provided by a surface type-tire type combination. eg the friction corresponding to a combination of surface type x and tire type y is mPairs[x][y]
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com