STM32L4R9I_EVAL BSP User Manual
|
Functions | |
uint8_t | BSP_DSI_LCD_DeInit (void) |
De-initialize the DSI LCD. | |
uint32_t | BSP_DSI_LCD_GetXSize (void) |
Gets the LCD X size. | |
uint32_t | BSP_DSI_LCD_GetYSize (void) |
Gets the LCD Y size. | |
uint8_t | BSP_DSI_LCD_SelectLayer (uint32_t LayerIndex) |
Selects the LCD Layer. | |
uint8_t | BSP_DSI_LCD_SetLayerVisible (uint32_t LayerIndex, FunctionalState State) |
Sets an LCD Layer visible. | |
uint8_t | BSP_DSI_LCD_SetTransparency (uint32_t LayerIndex, uint8_t Transparency) |
Configures the transparency. | |
uint8_t | BSP_DSI_LCD_SetColorKeying (uint32_t LayerIndex, uint32_t RGBValue) |
Configures and sets the color keying. | |
uint8_t | BSP_DSI_LCD_ResetColorKeying (uint32_t LayerIndex) |
Disables the color keying. | |
void | BSP_DSI_LCD_SetTextColor (uint32_t Color) |
Sets the LCD text color. | |
uint32_t | BSP_DSI_LCD_GetTextColor (void) |
Gets the LCD text color. | |
void | BSP_DSI_LCD_SetBackColor (uint32_t Color) |
Sets the LCD background color. | |
uint32_t | BSP_DSI_LCD_GetBackColor (void) |
Gets the LCD background color. | |
void | BSP_DSI_LCD_SetFont (sFONT *fonts) |
Sets the LCD text font. | |
sFONT * | BSP_DSI_LCD_GetFont (void) |
Gets the LCD text font. | |
uint32_t | BSP_DSI_LCD_ReadPixel (uint16_t Xpos, uint16_t Ypos) |
Reads an LCD pixel. | |
void | BSP_DSI_LCD_Clear (uint32_t Color) |
Clears the whole currently active layer of LTDC. | |
void | BSP_DSI_LCD_ClearStringLine (uint32_t Line) |
Clears the selected line in currently active layer. | |
void | BSP_DSI_LCD_DisplayChar (uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) |
Displays one character in currently active layer. | |
void | BSP_DSI_LCD_DisplayStringAt (uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Text_AlignModeTypdef Mode) |
Displays characters in currently active layer. | |
void | BSP_DSI_LCD_DisplayStringAtLine (uint16_t Line, uint8_t *ptr) |
Displays string on specified line of the LCD. | |
void | BSP_DSI_LCD_DrawHLine (uint16_t Xpos, uint16_t Ypos, uint16_t Length) |
Draws an horizontal line in currently active layer. | |
void | BSP_DSI_LCD_DrawVLine (uint16_t Xpos, uint16_t Ypos, uint16_t Length) |
Draws a vertical line in currently active layer. | |
void | BSP_DSI_LCD_DrawLine (uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) |
Draws an uni-line (between two points) in currently active layer. | |
void | BSP_DSI_LCD_DrawRect (uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) |
Draws a rectangle in currently active layer. | |
void | BSP_DSI_LCD_DrawCircle (uint16_t Xpos, uint16_t Ypos, uint16_t Radius) |
Draws a circle in currently active layer. | |
void | BSP_DSI_LCD_DrawPolygon (pPoint Points, uint16_t PointCount) |
Draws an poly-line (between many points) in currently active layer. | |
void | BSP_DSI_LCD_DrawEllipse (int Xpos, int Ypos, int XRadius, int YRadius) |
Draws an ellipse on LCD in currently active layer. | |
void | BSP_DSI_LCD_DrawBitmap (uint32_t Xpos, uint32_t Ypos, uint8_t *pbmp) |
Draws a bitmap picture loaded in the internal Flash (32 bpp) in currently active layer. | |
void | BSP_DSI_LCD_FillRect (uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) |
Draws a full rectangle in currently active layer. | |
void | BSP_DSI_LCD_FillCircle (uint16_t Xpos, uint16_t Ypos, uint16_t Radius) |
Draws a full circle in currently active layer. | |
void | BSP_DSI_LCD_FillPolygon (pPoint Points, uint16_t PointCount) |
Draws a full poly-line (between many points) in currently active layer. | |
void | BSP_DSI_LCD_FillEllipse (int Xpos, int Ypos, int XRadius, int YRadius) |
Draws a full ellipse in currently active layer. | |
void | BSP_DSI_LCD_DrawPixel (uint16_t Xpos, uint16_t Ypos, uint32_t RGB_Code) |
Draws a pixel on LCD. | |
void | BSP_DSI_LCD_DisplayOn (void) |
Switch back on the display if was switched off by previous call of BSP_DSI_LCD_DisplayOff(). | |
void | BSP_DSI_LCD_DisplayOff (void) |
Switch Off the display. | |
void | BSP_DSI_LCD_Refresh (void) |
Refresh the display. | |
uint8_t | BSP_DSI_LCD_IsFrameBufferAvailable (void) |
Check if frame buffer is available. | |
void | BSP_DSI_LCD_SetBrightness (uint8_t BrightnessValue) |
Set the brightness value. | |
__weak void | BSP_DSI_LCD_DMA2D_IRQHandler (void) |
Handles DMA2D interrupt request. | |
__weak void | BSP_DSI_LCD_DSI_IRQHandler (void) |
Handles DSI interrupt request. | |
__weak void | BSP_DSI_LCD_LTDC_IRQHandler (void) |
Handles LTDC interrupt request. | |
__weak void | BSP_DSI_LCD_LTDC_ER_IRQHandler (void) |
This function handles LTDC Error interrupt Handler. | |
__weak void | BSP_DSI_LCD_MspDeInit (void) |
De-Initializes the BSP LCD Msp. | |
__weak void | BSP_DSI_LCD_MspInit (void) |
Initialize the BSP LCD Msp. | |
uint8_t | BSP_DSI_LCD_Init (void) |
Function Documentation
void BSP_DSI_LCD_Clear | ( | uint32_t | Color | ) |
Clears the whole currently active layer of LTDC.
- Parameters:
-
Color,: Color of the background (in ARGB8888 format)
Definition at line 857 of file stm32l4r9i_eval_dsi_lcd.c.
References ActiveLayer, and LL_FillBuffer().
void BSP_DSI_LCD_ClearStringLine | ( | uint32_t | Line | ) |
Clears the selected line in currently active layer.
- Parameters:
-
Line,: Line to be cleared
Definition at line 868 of file stm32l4r9i_eval_dsi_lcd.c.
References ActiveLayer, LCD_DrawPropTypeDef::BackColor, BSP_DSI_LCD_FillRect(), BSP_DSI_LCD_SetTextColor(), LCD_DrawPropTypeDef::pFont, and LCD_DrawPropTypeDef::TextColor.
uint8_t BSP_DSI_LCD_DeInit | ( | void | ) |
De-initialize the DSI LCD.
- Return values:
-
LCD state
Definition at line 581 of file stm32l4r9i_eval_dsi_lcd.c.
References BSP_DSI_LCD_MspDeInit(), bsp_lcd_initialized, LCD_ERROR, and LCD_OK.
void BSP_DSI_LCD_DisplayChar | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint8_t | Ascii | ||
) |
Displays one character in currently active layer.
- Parameters:
-
Xpos,: Start column address Ypos,: Line where to display the character shape. Ascii,: Character ascii code This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E
Definition at line 887 of file stm32l4r9i_eval_dsi_lcd.c.
References ActiveLayer, DrawChar(), and LCD_DrawPropTypeDef::pFont.
Referenced by BSP_DSI_LCD_DisplayStringAt().
void BSP_DSI_LCD_DisplayOff | ( | void | ) |
Switch Off the display.
Definition at line 1455 of file stm32l4r9i_eval_dsi_lcd.c.
void BSP_DSI_LCD_DisplayOn | ( | void | ) |
Switch back on the display if was switched off by previous call of BSP_DSI_LCD_DisplayOff().
Definition at line 1442 of file stm32l4r9i_eval_dsi_lcd.c.
void BSP_DSI_LCD_DisplayStringAt | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint8_t * | Text, | ||
Text_AlignModeTypdef | Mode | ||
) |
Displays characters in currently active layer.
- Parameters:
-
Xpos,: X position (in pixel) Ypos,: Y position (in pixel) Text,: Pointer to string to display on LCD Mode,: Display mode This parameter can be one of the following values: - CENTER_MODE
- RIGHT_MODE
- LEFT_MODE
Definition at line 903 of file stm32l4r9i_eval_dsi_lcd.c.
References ActiveLayer, BSP_DSI_LCD_DisplayChar(), CENTER_MODE, LEFT_MODE, LCD_DrawPropTypeDef::pFont, and RIGHT_MODE.
Referenced by BSP_DSI_LCD_DisplayStringAtLine().
void BSP_DSI_LCD_DisplayStringAtLine | ( | uint16_t | Line, |
uint8_t * | ptr | ||
) |
Displays string on specified line of the LCD.
- Parameters:
-
Line,: Line where to display the character shape ptr,: Pointer to string to display on LCD
Definition at line 965 of file stm32l4r9i_eval_dsi_lcd.c.
References BSP_DSI_LCD_DisplayStringAt(), BSP_DSI_LCD_GetFont(), and CENTER_MODE.
void BSP_DSI_LCD_DMA2D_IRQHandler | ( | void | ) |
Handles DMA2D interrupt request.
- Note:
- Application can surcharge if needed this function implementation.
Definition at line 1510 of file stm32l4r9i_eval_dsi_lcd.c.
References hdma2d_eval.
void BSP_DSI_LCD_DrawBitmap | ( | uint32_t | Xpos, |
uint32_t | Ypos, | ||
uint8_t * | pbmp | ||
) |
Draws a bitmap picture loaded in the internal Flash (32 bpp) in currently active layer.
- Parameters:
-
Xpos,: Bmp X position in the LCD Ypos,: Bmp Y position in the LCD pbmp,: Pointer to Bmp picture address in the internal Flash
Definition at line 1207 of file stm32l4r9i_eval_dsi_lcd.c.
References ActiveLayer, and LL_ConvertLineToARGB8888().
void BSP_DSI_LCD_DrawCircle | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Radius | ||
) |
Draws a circle in currently active layer.
- Parameters:
-
Xpos,: X position Ypos,: Y position Radius,: Circle radius
Definition at line 1102 of file stm32l4r9i_eval_dsi_lcd.c.
References ActiveLayer, and BSP_DSI_LCD_DrawPixel().
Referenced by BSP_DSI_LCD_FillCircle().
void BSP_DSI_LCD_DrawEllipse | ( | int | Xpos, |
int | Ypos, | ||
int | XRadius, | ||
int | YRadius | ||
) |
Draws an ellipse on LCD in currently active layer.
- Parameters:
-
Xpos,: X position Ypos,: Y position XRadius,: Ellipse X radius YRadius,: Ellipse Y radius
Definition at line 1175 of file stm32l4r9i_eval_dsi_lcd.c.
References ActiveLayer, and BSP_DSI_LCD_DrawPixel().
void BSP_DSI_LCD_DrawHLine | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Length | ||
) |
Draws an horizontal line in currently active layer.
- Parameters:
-
Xpos,: X position Ypos,: Y position Length,: Line length
Definition at line 976 of file stm32l4r9i_eval_dsi_lcd.c.
References ActiveLayer, and LL_FillBuffer().
Referenced by BSP_DSI_LCD_DrawRect(), BSP_DSI_LCD_FillCircle(), and BSP_DSI_LCD_FillEllipse().
void BSP_DSI_LCD_DrawLine | ( | uint16_t | x1, |
uint16_t | y1, | ||
uint16_t | x2, | ||
uint16_t | y2 | ||
) |
Draws an uni-line (between two points) in currently active layer.
- Parameters:
-
x1,: Point 1 X position y1,: Point 1 Y position x2,: Point 2 X position y2,: Point 2 Y position
Definition at line 1011 of file stm32l4r9i_eval_dsi_lcd.c.
References ABS, ActiveLayer, and BSP_DSI_LCD_DrawPixel().
Referenced by BSP_DSI_LCD_DrawPolygon(), and FillTriangle().
void BSP_DSI_LCD_DrawPixel | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint32_t | RGB_Code | ||
) |
Draws a pixel on LCD.
- Parameters:
-
Xpos,: X position Ypos,: Y position RGB_Code,: Pixel color in ARGB mode (8-8-8-8)
Definition at line 1433 of file stm32l4r9i_eval_dsi_lcd.c.
References ActiveLayer.
Referenced by BSP_DSI_LCD_DrawCircle(), BSP_DSI_LCD_DrawEllipse(), BSP_DSI_LCD_DrawLine(), and DrawChar().
void BSP_DSI_LCD_DrawPolygon | ( | pPoint | Points, |
uint16_t | PointCount | ||
) |
Draws an poly-line (between many points) in currently active layer.
- Parameters:
-
Points,: Pointer to the points array PointCount,: Number of points
Definition at line 1148 of file stm32l4r9i_eval_dsi_lcd.c.
References BSP_DSI_LCD_DrawLine(), Point::X, and Point::Y.
void BSP_DSI_LCD_DrawRect | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Width, | ||
uint16_t | Height | ||
) |
Draws a rectangle in currently active layer.
- Parameters:
-
Xpos,: X position Ypos,: Y position Width,: Rectangle width Height,: Rectangle height
Definition at line 1085 of file stm32l4r9i_eval_dsi_lcd.c.
References BSP_DSI_LCD_DrawHLine(), and BSP_DSI_LCD_DrawVLine().
void BSP_DSI_LCD_DrawVLine | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Length | ||
) |
Draws a vertical line in currently active layer.
- Parameters:
-
Xpos,: X position Ypos,: Y position Length,: Line length
Definition at line 993 of file stm32l4r9i_eval_dsi_lcd.c.
References ActiveLayer, and LL_FillBuffer().
Referenced by BSP_DSI_LCD_DrawRect().
void BSP_DSI_LCD_DSI_IRQHandler | ( | void | ) |
Handles DSI interrupt request.
- Note:
- Application can surcharge if needed this function implementation.
Definition at line 1519 of file stm32l4r9i_eval_dsi_lcd.c.
void BSP_DSI_LCD_FillCircle | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Radius | ||
) |
Draws a full circle in currently active layer.
- Parameters:
-
Xpos,: X position Ypos,: Y position Radius,: Circle radius
Definition at line 1287 of file stm32l4r9i_eval_dsi_lcd.c.
References ActiveLayer, BSP_DSI_LCD_DrawCircle(), BSP_DSI_LCD_DrawHLine(), and BSP_DSI_LCD_SetTextColor().
void BSP_DSI_LCD_FillEllipse | ( | int | Xpos, |
int | Ypos, | ||
int | XRadius, | ||
int | YRadius | ||
) |
Draws a full ellipse in currently active layer.
- Parameters:
-
Xpos,: X position Ypos,: Y position XRadius,: Ellipse X radius YRadius,: Ellipse Y radius
Definition at line 1401 of file stm32l4r9i_eval_dsi_lcd.c.
References BSP_DSI_LCD_DrawHLine().
void BSP_DSI_LCD_FillPolygon | ( | pPoint | Points, |
uint16_t | PointCount | ||
) |
Draws a full poly-line (between many points) in currently active layer.
- Parameters:
-
Points,: Pointer to the points array PointCount,: Number of points
Definition at line 1334 of file stm32l4r9i_eval_dsi_lcd.c.
References FillTriangle(), POLY_X, POLY_Y, Point::X, and Point::Y.
void BSP_DSI_LCD_FillRect | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Width, | ||
uint16_t | Height | ||
) |
Draws a full rectangle in currently active layer.
- Parameters:
-
Xpos,: X position Ypos,: Y position Width,: Rectangle width Height,: Rectangle height
Definition at line 1267 of file stm32l4r9i_eval_dsi_lcd.c.
References ActiveLayer, BSP_DSI_LCD_SetTextColor(), and LL_FillBuffer().
Referenced by BSP_DSI_LCD_ClearStringLine().
uint32_t BSP_DSI_LCD_GetBackColor | ( | void | ) |
Gets the LCD background color.
- Return values:
-
Used background color
Definition at line 812 of file stm32l4r9i_eval_dsi_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::BackColor.
sFONT * BSP_DSI_LCD_GetFont | ( | void | ) |
Gets the LCD text font.
- Return values:
-
Used layer font
Definition at line 830 of file stm32l4r9i_eval_dsi_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::pFont.
Referenced by BSP_DSI_LCD_DisplayStringAtLine().
uint32_t BSP_DSI_LCD_GetTextColor | ( | void | ) |
Gets the LCD text color.
- Return values:
-
Used text color.
Definition at line 794 of file stm32l4r9i_eval_dsi_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::TextColor.
uint32_t BSP_DSI_LCD_GetXSize | ( | void | ) |
Gets the LCD X size.
- Return values:
-
Used LCD X size
Definition at line 644 of file stm32l4r9i_eval_dsi_lcd.c.
uint32_t BSP_DSI_LCD_GetYSize | ( | void | ) |
Gets the LCD Y size.
- Return values:
-
Used LCD Y size
Definition at line 653 of file stm32l4r9i_eval_dsi_lcd.c.
uint8_t BSP_DSI_LCD_Init | ( | void | ) |
uint8_t BSP_DSI_LCD_IsFrameBufferAvailable | ( | void | ) |
Check if frame buffer is available.
- Return values:
-
LCD_OK if frame buffer is available else LCD_ERROR (frame buffer busy)
Definition at line 1481 of file stm32l4r9i_eval_dsi_lcd.c.
void BSP_DSI_LCD_LTDC_ER_IRQHandler | ( | void | ) |
This function handles LTDC Error interrupt Handler.
- Note:
- Application can surcharge if needed this function implementation.
Definition at line 1539 of file stm32l4r9i_eval_dsi_lcd.c.
void BSP_DSI_LCD_LTDC_IRQHandler | ( | void | ) |
Handles LTDC interrupt request.
- Note:
- Application can surcharge if needed this function implementation.
Definition at line 1529 of file stm32l4r9i_eval_dsi_lcd.c.
void BSP_DSI_LCD_MspDeInit | ( | void | ) |
De-Initializes the BSP LCD Msp.
- Note:
- Application can surcharge if needed this function implementation.
Definition at line 1548 of file stm32l4r9i_eval_dsi_lcd.c.
References bsp_lcd_hse_to_disable.
Referenced by BSP_DSI_LCD_DeInit().
void BSP_DSI_LCD_MspInit | ( | void | ) |
Initialize the BSP LCD Msp.
- Note:
- Application can surcharge if needed this function implementation.
Definition at line 1591 of file stm32l4r9i_eval_dsi_lcd.c.
References bsp_lcd_hse_to_disable.
uint32_t BSP_DSI_LCD_ReadPixel | ( | uint16_t | Xpos, |
uint16_t | Ypos | ||
) |
Reads an LCD pixel.
- Parameters:
-
Xpos,: X position Ypos,: Y position
- Return values:
-
ARGB8888 pixel color
Definition at line 841 of file stm32l4r9i_eval_dsi_lcd.c.
References ActiveLayer.
void BSP_DSI_LCD_Refresh | ( | void | ) |
Refresh the display.
Definition at line 1468 of file stm32l4r9i_eval_dsi_lcd.c.
uint8_t BSP_DSI_LCD_ResetColorKeying | ( | uint32_t | LayerIndex | ) |
Disables the color keying.
- Parameters:
-
LayerIndex,: Layer foreground (1) or background (0)
- Note:
- : Only backgroung layer can be used.
- Return values:
-
LCD state
Definition at line 765 of file stm32l4r9i_eval_dsi_lcd.c.
References LCD_ERROR, LCD_OK, and LTDC_ACTIVE_LAYER_BACKGROUND.
uint8_t BSP_DSI_LCD_SelectLayer | ( | uint32_t | LayerIndex | ) |
Selects the LCD Layer.
- Parameters:
-
LayerIndex,: Layer foreground (1) or background (0)
- Note:
- : Only backgroung layer can be used.
- Return values:
-
LCD state
Definition at line 664 of file stm32l4r9i_eval_dsi_lcd.c.
References ActiveLayer, LCD_ERROR, LCD_OK, and LTDC_ACTIVE_LAYER_BACKGROUND.
void BSP_DSI_LCD_SetBackColor | ( | uint32_t | Color | ) |
Sets the LCD background color.
- Parameters:
-
Color,: Layer background color code ARGB8888
Definition at line 803 of file stm32l4r9i_eval_dsi_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::BackColor.
void BSP_DSI_LCD_SetBrightness | ( | uint8_t | BrightnessValue | ) |
Set the brightness value.
- Parameters:
-
BrightnessValue,: [0% Min (black), 100% Max]
Definition at line 1494 of file stm32l4r9i_eval_dsi_lcd.c.
uint8_t BSP_DSI_LCD_SetColorKeying | ( | uint32_t | LayerIndex, |
uint32_t | RGBValue | ||
) |
Configures and sets the color keying.
- Parameters:
-
LayerIndex,: Layer foreground (1) or background (0) RGBValue,: Color reference
- Note:
- : Only backgroung layer can be used.
- Return values:
-
LCD state
Definition at line 742 of file stm32l4r9i_eval_dsi_lcd.c.
References LCD_ERROR, LCD_OK, and LTDC_ACTIVE_LAYER_BACKGROUND.
void BSP_DSI_LCD_SetFont | ( | sFONT * | fonts | ) |
Sets the LCD text font.
- Parameters:
-
fonts,: Layer font to be used
Definition at line 821 of file stm32l4r9i_eval_dsi_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::pFont.
uint8_t BSP_DSI_LCD_SetLayerVisible | ( | uint32_t | LayerIndex, |
FunctionalState | State | ||
) |
Sets an LCD Layer visible.
- Parameters:
-
LayerIndex,: Visible Layer State,: New state of the specified layer This parameter can be one of the following values: - ENABLE
- DISABLE
- Note:
- : Only backgroung layer can be used.
- Return values:
-
LCD state
Definition at line 689 of file stm32l4r9i_eval_dsi_lcd.c.
References LCD_ERROR, LCD_OK, and LTDC_ACTIVE_LAYER_BACKGROUND.
void BSP_DSI_LCD_SetTextColor | ( | uint32_t | Color | ) |
Sets the LCD text color.
- Parameters:
-
Color,: Text color code ARGB8888
Definition at line 785 of file stm32l4r9i_eval_dsi_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::TextColor.
Referenced by BSP_DSI_LCD_ClearStringLine(), BSP_DSI_LCD_FillCircle(), and BSP_DSI_LCD_FillRect().
uint8_t BSP_DSI_LCD_SetTransparency | ( | uint32_t | LayerIndex, |
uint8_t | Transparency | ||
) |
Configures the transparency.
- Parameters:
-
LayerIndex,: Layer foreground or background. Transparency,: Transparency This parameter must be a number between Min_Data = 0x00 and Max_Data = 0xFF
- Note:
- : Only backgroung layer can be used.
- Return values:
-
LCD state
Definition at line 720 of file stm32l4r9i_eval_dsi_lcd.c.
References LCD_ERROR, LCD_OK, and LTDC_ACTIVE_LAYER_BACKGROUND.
Generated on Thu Oct 12 2017 10:54:00 for STM32L4R9I_EVAL BSP User Manual by
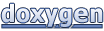