STM32L4R9I_EVAL BSP User Manual
|
stm32l4r9i_eval_lcd.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4r9i_eval_lcd.h 00004 * @author MCD Application Team 00005 * @brief This file contains alias for compatibility with DSI and TFT LCD. 00006 ****************************************************************************** 00007 * @attention 00008 * 00009 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00010 * 00011 * Redistribution and use in source and binary forms, with or without modification, 00012 * are permitted provided that the following conditions are met: 00013 * 1. Redistributions of source code must retain the above copyright notice, 00014 * this list of conditions and the following disclaimer. 00015 * 2. Redistributions in binary form must reproduce the above copyright notice, 00016 * this list of conditions and the following disclaimer in the documentation 00017 * and/or other materials provided with the distribution. 00018 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00019 * may be used to endorse or promote products derived from this software 00020 * without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00023 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00024 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00025 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00026 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00027 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00028 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00029 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00030 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00031 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00032 * 00033 ****************************************************************************** 00034 */ 00035 00036 /* Define to prevent recursive inclusion -------------------------------------*/ 00037 #ifndef __STM32L4R9I_EVAL_LCD_H 00038 #define __STM32L4R9I_EVAL_LCD_H 00039 00040 #ifdef __cplusplus 00041 extern "C" { 00042 #endif 00043 00044 #include "../../../Utilities/Fonts/fonts.h" 00045 00046 /** @addtogroup BSP 00047 * @{ 00048 */ 00049 00050 /** @addtogroup STM32L4R9I_EVAL 00051 * @{ 00052 */ 00053 00054 /** @addtogroup STM32L4R9I_EVAL_LCD STM32L4R9I_EVAL LCD 00055 * @{ 00056 */ 00057 00058 /** @defgroup STM32L4R9I_EVAL_LCD_Exported_Constants Exported Constants 00059 * @{ 00060 */ 00061 00062 /* LTDC layer configuration structure */ 00063 #define LCD_LayerCfgTypeDef LTDC_LayerCfgTypeDef 00064 00065 /* Maximum number of LTDC layers */ 00066 #define LTDC_MAX_LAYER_NUMBER ((uint32_t) 2) 00067 00068 /* LTDC Background layer index */ 00069 #define LTDC_ACTIVE_LAYER_BACKGROUND LTDC_LAYER_1 00070 00071 /* LTDC Foreground layer index : Not used on STM32L4R9I_EVAL, only one layer used */ 00072 #define LTDC_ACTIVE_LAYER_FOREGROUND LTDC_LAYER_2 00073 00074 /* Number of LTDC layers */ 00075 #define LTDC_NB_OF_LAYERS ((uint32_t) 2) 00076 00077 /* LTDC Default used layer index */ 00078 #define LTDC_DEFAULT_ACTIVE_LAYER LTDC_ACTIVE_LAYER_BACKGROUND 00079 00080 /* LCD status */ 00081 #define LCD_OK 0x00 00082 #define LCD_ERROR 0x01 00083 #define LCD_TIMEOUT 0x02 00084 00085 /* LCD color definitions values in ARGB8888 format */ 00086 /* Blue value in ARGB8888 format */ 00087 #define LCD_COLOR_BLUE ((uint32_t) 0xFF0000FF) 00088 /* Green value in ARGB8888 format */ 00089 #define LCD_COLOR_GREEN ((uint32_t) 0xFF00FF00) 00090 /* Red value in ARGB8888 format */ 00091 #define LCD_COLOR_RED ((uint32_t) 0xFFFF0000) 00092 /* Cyan value in ARGB8888 format */ 00093 #define LCD_COLOR_CYAN ((uint32_t) 0xFF00FFFF) 00094 /* Magenta value in ARGB8888 format */ 00095 #define LCD_COLOR_MAGENTA ((uint32_t) 0xFFFF00FF) 00096 /* Yellow value in ARGB8888 format */ 00097 #define LCD_COLOR_YELLOW ((uint32_t) 0xFFFFFF00) 00098 /* Light Blue value in ARGB8888 format */ 00099 #define LCD_COLOR_LIGHTBLUE ((uint32_t) 0xFF8080FF) 00100 /* Light Green value in ARGB8888 format */ 00101 #define LCD_COLOR_LIGHTGREEN ((uint32_t) 0xFF80FF80) 00102 /* Light Red value in ARGB8888 format */ 00103 #define LCD_COLOR_LIGHTRED ((uint32_t) 0xFFFF8080) 00104 /* Light Cyan value in ARGB8888 format */ 00105 #define LCD_COLOR_LIGHTCYAN ((uint32_t) 0xFF80FFFF) 00106 /* Light Magenta value in ARGB8888 format */ 00107 #define LCD_COLOR_LIGHTMAGENTA ((uint32_t) 0xFFFF80FF) 00108 /* Light Yellow value in ARGB8888 format */ 00109 #define LCD_COLOR_LIGHTYELLOW ((uint32_t) 0xFFFFFF80) 00110 /* Dark Blue value in ARGB8888 format */ 00111 #define LCD_COLOR_DARKBLUE ((uint32_t) 0xFF000080) 00112 /* Light Dark Green value in ARGB8888 format */ 00113 #define LCD_COLOR_DARKGREEN ((uint32_t) 0xFF008000) 00114 /* Light Dark Red value in ARGB8888 format */ 00115 #define LCD_COLOR_DARKRED ((uint32_t) 0xFF800000) 00116 /* Dark Cyan value in ARGB8888 format */ 00117 #define LCD_COLOR_DARKCYAN ((uint32_t) 0xFF008080) 00118 /* Dark Magenta value in ARGB8888 format */ 00119 #define LCD_COLOR_DARKMAGENTA ((uint32_t) 0xFF800080) 00120 /* Dark Yellow value in ARGB8888 format */ 00121 #define LCD_COLOR_DARKYELLOW ((uint32_t) 0xFF808000) 00122 /* White value in ARGB8888 format */ 00123 #define LCD_COLOR_WHITE ((uint32_t) 0xFFFFFFFF) 00124 /* Light Gray value in ARGB8888 format */ 00125 #define LCD_COLOR_LIGHTGRAY ((uint32_t) 0xFFD3D3D3) 00126 /* Gray value in ARGB8888 format */ 00127 #define LCD_COLOR_GRAY ((uint32_t) 0xFF808080) 00128 /* Dark Gray value in ARGB8888 format */ 00129 #define LCD_COLOR_DARKGRAY ((uint32_t) 0xFF404040) 00130 /* Black value in ARGB8888 format */ 00131 #define LCD_COLOR_BLACK ((uint32_t) 0xFF000000) 00132 /* Brown value in ARGB8888 format */ 00133 #define LCD_COLOR_BROWN ((uint32_t) 0xFFA52A2A) 00134 /* Orange value in ARGB8888 format */ 00135 #define LCD_COLOR_ORANGE ((uint32_t) 0xFFFFA500) 00136 /* Transparent value in ARGB8888 format */ 00137 #define LCD_COLOR_TRANSPARENT ((uint32_t) 0xFF000000) 00138 00139 /* LCD default font */ 00140 #define LCD_DEFAULT_FONT Font24 00141 00142 /** 00143 * @} 00144 */ 00145 00146 /** @defgroup STM32L4R9I_EVAL_LCD_Exported_Types Exported Types 00147 * @{ 00148 */ 00149 00150 /* LCD Drawing main properties */ 00151 typedef struct 00152 { 00153 uint32_t TextColor; /*!< Specifies the color of text */ 00154 uint32_t BackColor; /*!< Specifies the background color below the text */ 00155 sFONT *pFont; /*!< Specifies the font used for the text */ 00156 } LCD_DrawPropTypeDef; 00157 00158 /* LCD Drawing point (pixel) geometric definition */ 00159 typedef struct 00160 { 00161 int16_t X; /*!< geometric X position of drawing */ 00162 int16_t Y; /*!< geometric Y position of drawing */ 00163 } Point; 00164 00165 /* Pointer on LCD Drawing point (pixel) geometric definition */ 00166 typedef Point * pPoint; 00167 00168 /* LCD drawing Line alignment mode definitions */ 00169 typedef enum 00170 { 00171 CENTER_MODE = 0x01, /*!< Center mode */ 00172 RIGHT_MODE = 0x02, /*!< Right mode */ 00173 LEFT_MODE = 0x03 /*!< Left mode */ 00174 } Text_AlignModeTypdef; 00175 00176 /* LCD_OrientationTypeDef : Possible values of Display Orientation */ 00177 typedef enum 00178 { 00179 LCD_ORIENTATION_PORTRAIT = 0x00, /*!< Portrait orientation choice of LCD screen */ 00180 LCD_ORIENTATION_LANDSCAPE = 0x01, /*!< Landscape orientation choice of LCD screen */ 00181 LCD_ORIENTATION_INVALID = 0x02 /*!< Invalid orientation choice of LCD screen */ 00182 } LCD_OrientationTypeDef; 00183 00184 /** 00185 * @} 00186 */ 00187 00188 /** @addtogroup STM32L4R9I_EVAL_DSI_LCD_Exported_Functions 00189 * @{ 00190 */ 00191 00192 #if defined(USE_GVO_390x390) 00193 00194 #include "stm32l4r9i_eval_dsi_lcd.h" 00195 00196 #define BSP_LCD_DMA2D_IRQHandler BSP_DSI_LCD_DMA2D_IRQHandler 00197 #define BSP_LCD_DSI_IRQHandler BSP_DSI_LCD_DSI_IRQHandler 00198 #define BSP_LCD_LTDC_IRQHandler BSP_DSI_LCD_LTDC_IRQHandler 00199 #define BSP_LCD_LTDC_ER_IRQHandler BSP_DSI_LCD_LTDC_ER_IRQHandler 00200 00201 #define BSP_LCD_Init BSP_DSI_LCD_Init 00202 #define BSP_LCD_DeInit BSP_DSI_LCD_DeInit 00203 00204 #define BSP_LCD_MspDeInit BSP_DSI_LCD_MspDeInit 00205 #define BSP_LCD_MspInit BSP_DSI_LCD_MspInit 00206 00207 #define BSP_LCD_GetXSize BSP_DSI_LCD_GetXSize 00208 #define BSP_LCD_GetYSize BSP_DSI_LCD_GetYSize 00209 00210 #define BSP_LCD_SetTransparency BSP_DSI_LCD_SetTransparency 00211 #define BSP_LCD_SetColorKeying BSP_DSI_LCD_SetColorKeying 00212 #define BSP_LCD_ResetColorKeying BSP_DSI_LCD_ResetColorKeying 00213 00214 #define BSP_LCD_SelectLayer BSP_DSI_LCD_SelectLayer 00215 #define BSP_LCD_SetLayerVisible BSP_DSI_LCD_SetLayerVisible 00216 00217 #define BSP_LCD_SetTextColor BSP_DSI_LCD_SetTextColor 00218 #define BSP_LCD_GetTextColor BSP_DSI_LCD_GetTextColor 00219 #define BSP_LCD_SetBackColor BSP_DSI_LCD_SetBackColor 00220 #define BSP_LCD_GetBackColor BSP_DSI_LCD_GetBackColor 00221 #define BSP_LCD_SetFont BSP_DSI_LCD_SetFont 00222 #define BSP_LCD_GetFont BSP_DSI_LCD_GetFont 00223 00224 #define BSP_LCD_ReadPixel BSP_DSI_LCD_ReadPixel 00225 #define BSP_LCD_DrawPixel BSP_DSI_LCD_DrawPixel 00226 #define BSP_LCD_Clear BSP_DSI_LCD_Clear 00227 #define BSP_LCD_ClearStringLine BSP_DSI_LCD_ClearStringLine 00228 #define BSP_LCD_DisplayStringAtLine BSP_DSI_LCD_DisplayStringAtLine 00229 #define BSP_LCD_DisplayStringAt BSP_DSI_LCD_DisplayStringAt 00230 #define BSP_LCD_DisplayChar BSP_DSI_LCD_DisplayChar 00231 00232 #define BSP_LCD_DrawHLine BSP_DSI_LCD_DrawHLine 00233 #define BSP_LCD_DrawVLine BSP_DSI_LCD_DrawVLine 00234 #define BSP_LCD_DrawLine BSP_DSI_LCD_DrawLine 00235 #define BSP_LCD_DrawRect BSP_DSI_LCD_DrawRect 00236 #define BSP_LCD_DrawCircle BSP_DSI_LCD_DrawCircle 00237 #define BSP_LCD_DrawPolygon BSP_DSI_LCD_DrawPolygon 00238 #define BSP_LCD_DrawEllipse BSP_DSI_LCD_DrawEllipse 00239 #define BSP_LCD_DrawBitmap BSP_DSI_LCD_DrawBitmap 00240 00241 #define BSP_LCD_FillRect BSP_DSI_LCD_FillRect 00242 #define BSP_LCD_FillCircle BSP_DSI_LCD_FillCircle 00243 #define BSP_LCD_FillPolygon BSP_DSI_LCD_FillPolygon 00244 #define BSP_LCD_FillEllipse BSP_DSI_LCD_FillEllipse 00245 00246 #define BSP_LCD_DisplayOff BSP_DSI_LCD_DisplayOff 00247 #define BSP_LCD_DisplayOn BSP_DSI_LCD_DisplayOn 00248 00249 #define BSP_LCD_Refresh BSP_DSI_LCD_Refresh 00250 #define BSP_LCD_IsFrameBufferAvailable BSP_DSI_LCD_IsFrameBufferAvailable 00251 00252 #define BSP_LCD_SetBrightness BSP_DSI_LCD_SetBrightness 00253 00254 #endif /* USE_GVO_390x390 */ 00255 00256 #if defined(USE_ROCKTECH_480x272) 00257 00258 #include "stm32l4r9i_eval_rgb_lcd.h" 00259 00260 #define BSP_LCD_DMA2D_IRQHandler BSP_RGB_LCD_DMA2D_IRQHandler 00261 #define BSP_LCD_LTDC_IRQHandler BSP_RGB_LCD_LTDC_IRQHandler 00262 #define BSP_LCD_LTDC_ER_IRQHandler BSP_RGB_LCD_LTDC_ER_IRQHandler 00263 00264 #define BSP_LCD_Init BSP_RGB_LCD_Init 00265 #define BSP_LCD_DeInit BSP_RGB_LCD_DeInit 00266 00267 #define BSP_LCD_MspDeInit BSP_RGB_LCD_MspDeInit 00268 #define BSP_LCD_MspInit BSP_RGB_LCD_MspInit 00269 00270 #define BSP_LCD_GetXSize BSP_RGB_LCD_GetXSize 00271 #define BSP_LCD_GetYSize BSP_RGB_LCD_GetYSize 00272 00273 #define BSP_LCD_SetTransparency BSP_RGB_LCD_SetTransparency 00274 #define BSP_LCD_SetColorKeying BSP_RGB_LCD_SetColorKeying 00275 #define BSP_LCD_ResetColorKeying BSP_RGB_LCD_ResetColorKeying 00276 00277 #define BSP_LCD_LayerDefaultInit BSP_RGB_LCD_LayerRgb565Init 00278 #define BSP_LCD_SelectLayer BSP_RGB_LCD_SelectLayer 00279 #define BSP_LCD_SetLayerVisible BSP_RGB_LCD_SetLayerVisible 00280 00281 #define BSP_LCD_SetTextColor BSP_RGB_LCD_SetTextColor 00282 #define BSP_LCD_GetTextColor BSP_RGB_LCD_GetTextColor 00283 #define BSP_LCD_SetBackColor BSP_RGB_LCD_SetBackColor 00284 #define BSP_LCD_GetBackColor BSP_RGB_LCD_GetBackColor 00285 #define BSP_LCD_SetFont BSP_RGB_LCD_SetFont 00286 #define BSP_LCD_GetFont BSP_RGB_LCD_GetFont 00287 00288 #define BSP_LCD_ReadPixel BSP_RGB_LCD_ReadPixel 00289 #define BSP_LCD_DrawPixel BSP_RGB_LCD_DrawPixel 00290 #define BSP_LCD_Clear BSP_RGB_LCD_Clear 00291 #define BSP_LCD_ClearStringLine BSP_RGB_LCD_ClearStringLine 00292 #define BSP_LCD_DisplayStringAtLine BSP_RGB_LCD_DisplayStringAtLine 00293 #define BSP_LCD_DisplayStringAt BSP_RGB_LCD_DisplayStringAt 00294 #define BSP_LCD_DisplayChar BSP_RGB_LCD_DisplayChar 00295 00296 #define BSP_LCD_DrawHLine BSP_RGB_LCD_DrawHLine 00297 #define BSP_LCD_DrawVLine BSP_RGB_LCD_DrawVLine 00298 #define BSP_LCD_DrawLine BSP_RGB_LCD_DrawLine 00299 #define BSP_LCD_DrawRect BSP_RGB_LCD_DrawRect 00300 #define BSP_LCD_DrawCircle BSP_RGB_LCD_DrawCircle 00301 #define BSP_LCD_DrawPolygon BSP_RGB_LCD_DrawPolygon 00302 #define BSP_LCD_DrawEllipse BSP_RGB_LCD_DrawEllipse 00303 #define BSP_LCD_DrawBitmap BSP_RGB_LCD_DrawBitmap 00304 00305 #define BSP_LCD_FillRect BSP_RGB_LCD_FillRect 00306 #define BSP_LCD_FillCircle BSP_RGB_LCD_FillCircle 00307 #define BSP_LCD_FillPolygon BSP_RGB_LCD_FillPolygon 00308 #define BSP_LCD_FillEllipse BSP_RGB_LCD_FillEllipse 00309 00310 #define BSP_LCD_DisplayOff BSP_RGB_LCD_DisplayOff 00311 #define BSP_LCD_DisplayOn BSP_RGB_LCD_DisplayOn 00312 00313 #endif /* USE_ROCKTECH_480x272 */ 00314 00315 /** 00316 * @} 00317 */ 00318 00319 /** 00320 * @} 00321 */ 00322 00323 /** 00324 * @} 00325 */ 00326 00327 /** 00328 * @} 00329 */ 00330 00331 #ifdef __cplusplus 00332 } 00333 #endif 00334 00335 #endif /* __STM32L4R9I_EVAL_LCD_H */ 00336 00337 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Oct 12 2017 10:53:59 for STM32L4R9I_EVAL BSP User Manual by
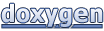