STM32L4R9I_EVAL BSP User Manual
|
stm32l4r9i_eval_dsi_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4r9i_eval_dsi_lcd.c 00004 * @author MCD Application Team 00005 * @brief This file includes the driver for DSI Liquid Crystal Display (LCD) 00006 * module mounted on STM32L4R9I_EVAL evaluation board. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* File Info: ------------------------------------------------------------------ 00038 User NOTES 00039 1. How To use this driver: 00040 -------------------------- 00041 - This driver is used to drive directly in command mode a LCD TFT using the 00042 DSI interface. 00043 The following IPs are implied : DSI Host IP block working 00044 in conjunction to the LTDC controller. 00045 - This driver is linked by construction to LCD. 00046 00047 2. Driver description: 00048 ---------------------- 00049 + Initialization steps: 00050 o Initialize the LCD using the BSP_DSI_LCD_Init() function. 00051 Please note that LCD display will be turned on at the end of this function. 00052 o De-inietialize the LCD using BSP_DSI_LCD_DeInit() function. 00053 00054 + Options 00055 o Modify in the fly the display properties using the following functions: 00056 - BSP_DSI_LCD_SetTransparency(). 00057 - BSP_DSI_LCD_SetColorKeying(). 00058 - BSP_DSI_LCD_ResetColorKeying(). 00059 - BSP_DSI_LCD_SetFont(). 00060 - BSP_DSI_LCD_SetBackColor(). 00061 - BSP_DSI_LCD_SetTextColor(). 00062 - BSP_DSI_LCD_SetBrightness(). 00063 o You can set display on/off using following functions: 00064 - BSP_DSI_LCD_DisplayOn(). 00065 - BSP_DSI_LCD_DisplayOff(). 00066 00067 + Display on LCD 00068 o First, check that frame buffer is available using BSP_DSI_LCD_IsFrameBufferAvailable(). 00069 o When frame buffer is available, modify it using following functions: 00070 - BSP_DSI_LCD_Clear(). 00071 - BSP_DSI_LCD_ClearStringLine(). 00072 - BSP_DSI_LCD_DisplayChar(). 00073 - BSP_DSI_LCD_DisplayStringAt(). 00074 - BSP_DSI_LCD_DisplayStringAtLine(). 00075 - BSP_DSI_LCD_DrawBitmap(). 00076 - BSP_DSI_LCD_DrawCircle(). 00077 - BSP_DSI_LCD_DrawEllipse(). 00078 - .... 00079 o Call BSP_DSI_LCD_Refresh() to refresh LCD display. 00080 00081 ------------------------------------------------------------------------------*/ 00082 00083 /* Includes ------------------------------------------------------------------*/ 00084 #include "stm32l4r9i_eval_lcd.h" 00085 #include "stm32l4r9i_eval_dsi_lcd.h" 00086 #include "stm32l4r9i_eval_gfxmmu_lut.h" 00087 #include "stm32l4r9i_eval_io.h" 00088 #include "../../../Utilities/Fonts/fonts.h" 00089 #include "../../../Utilities/Fonts/font24.c" 00090 #include "../../../Utilities/Fonts/font20.c" 00091 #include "../../../Utilities/Fonts/font16.c" 00092 #include "../../../Utilities/Fonts/font12.c" 00093 #include "../../../Utilities/Fonts/font8.c" 00094 00095 /** @addtogroup BSP 00096 * @{ 00097 */ 00098 00099 /** @addtogroup STM32L4R9I_EVAL 00100 * @{ 00101 */ 00102 00103 /** @defgroup STM32L4R9I_EVAL_LCD STM32L4R9I_EVAL LCD 00104 * @{ 00105 */ 00106 00107 /** @defgroup STM32L4R9I_EVAL_DSI_LCD STM32L4R9I_EVAL DSI LCD 00108 * @{ 00109 */ 00110 00111 /** @defgroup STM32L4R9I_EVAL_DSI_LCD_Private_Macros Private Macros 00112 * @{ 00113 */ 00114 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00115 00116 #define POLY_X(Z) ((int32_t)((Points + (Z))->X)) 00117 #define POLY_Y(Z) ((int32_t)((Points + (Z))->Y)) 00118 /** 00119 * @} 00120 */ 00121 00122 /** @defgroup STM32L4R9I_EVAL_DSI_LCD_Exported_Variables Exported Variables 00123 * @{ 00124 */ 00125 /* DMA2D handle */ 00126 DMA2D_HandleTypeDef hdma2d_eval; 00127 00128 /** 00129 * @} 00130 */ 00131 00132 /** @defgroup STM32L4R9I_EVAL_DSI_LCD_Private_Variables Private Variables 00133 * @{ 00134 */ 00135 /* LCD initialization status */ 00136 static uint32_t bsp_lcd_initialized = 0; 00137 /* Flag to indicate if HSE has to be disabled during de-initialization */ 00138 static uint32_t bsp_lcd_hse_to_disable = 0; 00139 /* Default Active LTDC Layer in which drawing is made is LTDC Layer Background */ 00140 static uint32_t ActiveLayer = LTDC_ACTIVE_LAYER_BACKGROUND; 00141 /* Current Drawing Layer properties variable */ 00142 static LCD_DrawPropTypeDef DrawProp[LTDC_MAX_LAYER_NUMBER]; 00143 00144 /* Physical frame buffer for active layer */ 00145 /* 390*390 pixels with 32bpp - 20% */ 00146 #if defined ( __ICCARM__ ) /* IAR Compiler */ 00147 #pragma data_alignment = 16 00148 uint32_t PhysFrameBuffer[121680]; 00149 #elif defined (__GNUC__) /* GNU Compiler */ 00150 uint32_t PhysFrameBuffer[121680] __attribute__ ((aligned (16))); 00151 #else /* ARM Compiler */ 00152 __align(16) uint32_t PhysFrameBuffer[121680]; 00153 #endif 00154 00155 /* Global variable used to know if frame buffer is available (1) or not because refresh is on going (0) */ 00156 __IO uint32_t FrameBufferAvailable = 1; 00157 /* LCD size */ 00158 uint32_t lcd_x_size = 390; 00159 uint32_t lcd_y_size = 390; 00160 /* GFXMMU, LTDC and DSI handles */ 00161 GFXMMU_HandleTypeDef hgfxmmu_eval; 00162 LTDC_HandleTypeDef hltdc_eval; 00163 DSI_HandleTypeDef hdsi_eval; 00164 /** 00165 * @} 00166 */ 00167 00168 /** @defgroup STM32L4R9I_EVAL_DSI_LCD_Private_FunctionPrototypes Private FunctionPrototypes 00169 * @{ 00170 */ 00171 static void LCD_Reset(void); 00172 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c); 00173 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3); 00174 static void LL_FillBuffer(uint32_t LayerIndex, void *pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex); 00175 static void LL_ConvertLineToARGB8888(void * pSrc, void *pDst, uint32_t xSize, uint32_t ColorMode); 00176 /** 00177 * @} 00178 */ 00179 00180 /** @defgroup STM32L4R9I_EVAL_DSI_LCD_Exported_Functions Exported Functions 00181 * @{ 00182 */ 00183 00184 /** 00185 * @brief Initialize the DSI LCD. 00186 * The initialization is done as below: 00187 * - GFXMMU initialization 00188 * - DSI PLL initialization 00189 * - DSI initialization 00190 * - LTDC initialization 00191 * - RM67162 LCD Display IC Driver initialization 00192 * @retval LCD state 00193 */ 00194 uint8_t BSP_DSI_LCD_Init(void) 00195 { 00196 if(bsp_lcd_initialized == 0) 00197 { 00198 LTDC_LayerCfgTypeDef LayerCfg; 00199 DSI_PLLInitTypeDef dsiPllInit; 00200 DSI_PHY_TimerTypeDef PhyTimings; 00201 DSI_HOST_TimeoutTypeDef HostTimeouts; 00202 DSI_LPCmdTypeDef LPCmd; 00203 DSI_CmdCfgTypeDef CmdCfg; 00204 00205 /* Toggle Hardware Reset of the DSI LCD using its XRES signal (active low) */ 00206 LCD_Reset(); 00207 00208 /* Call first MSP Initialize 00209 * This will set IP blocks LTDC, DSI and DMA2D 00210 * - out of reset 00211 * - clocked 00212 * - NVIC IRQ related to IP blocks enabled 00213 */ 00214 BSP_DSI_LCD_MspInit(); 00215 00216 /************************/ 00217 /* GFXMMU CONFIGURATION */ 00218 /************************/ 00219 hgfxmmu_eval.Instance = GFXMMU; 00220 __HAL_GFXMMU_RESET_HANDLE_STATE(&hgfxmmu_eval); 00221 hgfxmmu_eval.Init.BlocksPerLine = GFXMMU_192BLOCKS; 00222 hgfxmmu_eval.Init.DefaultValue = 0xFFFFFFFF; 00223 hgfxmmu_eval.Init.Buffers.Buf0Address = (uint32_t) PhysFrameBuffer; 00224 hgfxmmu_eval.Init.Buffers.Buf1Address = 0; /* NU */ 00225 hgfxmmu_eval.Init.Buffers.Buf2Address = 0; /* NU */ 00226 hgfxmmu_eval.Init.Buffers.Buf3Address = 0; /* NU */ 00227 hgfxmmu_eval.Init.Interrupts.Activation = DISABLE; 00228 hgfxmmu_eval.Init.Interrupts.UsedInterrupts = GFXMMU_AHB_MASTER_ERROR_IT; /* NU */ 00229 if(HAL_OK != HAL_GFXMMU_Init(&hgfxmmu_eval)) 00230 { 00231 return(LCD_ERROR); 00232 } 00233 00234 /* Initialize LUT */ 00235 if(HAL_OK != HAL_GFXMMU_ConfigLut(&hgfxmmu_eval, 0, 390, (uint32_t) gfxmmu_lut_config_argb8888)) 00236 { 00237 return(LCD_ERROR); 00238 } 00239 /* Disable non visible lines : from line 390 to 1023 */ 00240 if(HAL_OK != HAL_GFXMMU_DisableLutLines(&hgfxmmu_eval, 390, 634)) 00241 { 00242 return(LCD_ERROR); 00243 } 00244 00245 /**********************/ 00246 /* LTDC CONFIGURATION */ 00247 /**********************/ 00248 00249 /* LTDC initialization */ 00250 hltdc_eval.Instance = LTDC; 00251 __HAL_LTDC_RESET_HANDLE_STATE(&hltdc_eval); 00252 hltdc_eval.Init.HSPolarity = LTDC_HSPOLARITY_AL; 00253 hltdc_eval.Init.VSPolarity = LTDC_VSPOLARITY_AL; 00254 hltdc_eval.Init.DEPolarity = LTDC_DEPOLARITY_AL; 00255 hltdc_eval.Init.PCPolarity = LTDC_PCPOLARITY_IPC; 00256 hltdc_eval.Init.HorizontalSync = 0; /* HSYNC width - 1 */ 00257 hltdc_eval.Init.VerticalSync = 0; /* VSYNC width - 1 */ 00258 hltdc_eval.Init.AccumulatedHBP = 1; /* HSYNC width + HBP - 1 */ 00259 hltdc_eval.Init.AccumulatedVBP = 1; /* VSYNC width + VBP - 1 */ 00260 hltdc_eval.Init.AccumulatedActiveW = 391; /* HSYNC width + HBP + Active width - 1 */ 00261 hltdc_eval.Init.AccumulatedActiveH = 391; /* VSYNC width + VBP + Active height - 1 */ 00262 hltdc_eval.Init.TotalWidth = 392; /* HSYNC width + HBP + Active width + HFP - 1 */ 00263 hltdc_eval.Init.TotalHeigh = 392; /* VSYNC width + VBP + Active height + VFP - 1 */ 00264 hltdc_eval.Init.Backcolor.Red = 255; 00265 hltdc_eval.Init.Backcolor.Green = 255; 00266 hltdc_eval.Init.Backcolor.Blue = 0; 00267 hltdc_eval.Init.Backcolor.Reserved = 0xFF; 00268 if(HAL_LTDC_Init(&hltdc_eval) != HAL_OK) 00269 { 00270 return(LCD_ERROR); 00271 } 00272 00273 /* LTDC layers configuration */ 00274 LayerCfg.WindowX0 = 0; 00275 LayerCfg.WindowX1 = 390; 00276 LayerCfg.WindowY0 = 0; 00277 LayerCfg.WindowY1 = 390; 00278 LayerCfg.PixelFormat = LTDC_PIXEL_FORMAT_ARGB8888; 00279 LayerCfg.Alpha = 0xFF; /* NU default value */ 00280 LayerCfg.Alpha0 = 0; /* NU default value */ 00281 LayerCfg.BlendingFactor1 = LTDC_BLENDING_FACTOR1_PAxCA; /* NU default value */ 00282 LayerCfg.BlendingFactor2 = LTDC_BLENDING_FACTOR2_PAxCA; /* NU default value */ 00283 LayerCfg.FBStartAdress = GFXMMU_VIRTUAL_BUFFER0_BASE; 00284 LayerCfg.ImageWidth = 768; /* virtual frame buffer contains 768 pixels per line for 32bpp */ 00285 LayerCfg.ImageHeight = 390; 00286 LayerCfg.Backcolor.Red = 0; /* NU default value */ 00287 LayerCfg.Backcolor.Green = 0; /* NU default value */ 00288 LayerCfg.Backcolor.Blue = 0; /* NU default value */ 00289 LayerCfg.Backcolor.Reserved = 0xFF; 00290 if(HAL_LTDC_ConfigLayer(&hltdc_eval, &LayerCfg, 0) != HAL_OK) 00291 { 00292 return(LCD_ERROR); 00293 } 00294 00295 DrawProp[0].BackColor = LCD_COLOR_WHITE; 00296 DrawProp[0].pFont = &Font24; 00297 DrawProp[0].TextColor = LCD_COLOR_BLACK; 00298 00299 /*********************/ 00300 /* DSI CONFIGURATION */ 00301 /*********************/ 00302 00303 /* DSI initialization */ 00304 hdsi_eval.Instance = DSI; 00305 __HAL_DSI_RESET_HANDLE_STATE(&hdsi_eval); 00306 hdsi_eval.Init.AutomaticClockLaneControl = DSI_AUTO_CLK_LANE_CTRL_DISABLE; 00307 /* We have 1 data lane at 500Mbps => lane byte clock at 500/8 = 62,5 MHZ */ 00308 /* We want TX escape clock at arround 20MHz and under 20MHz so clock division is set to 4 */ 00309 hdsi_eval.Init.TXEscapeCkdiv = 4; 00310 hdsi_eval.Init.NumberOfLanes = DSI_ONE_DATA_LANE; 00311 /* We have HSE value at 25MHz or 16MHz and we want data lane at 500Mbps */ 00312 dsiPllInit.PLLNDIV = (HSE_VALUE == 25000000) ? 100 : 125; 00313 dsiPllInit.PLLIDF = (HSE_VALUE == 25000000) ? DSI_PLL_IN_DIV5 : DSI_PLL_IN_DIV4; 00314 dsiPllInit.PLLODF = DSI_PLL_OUT_DIV1; 00315 if(HAL_DSI_Init(&hdsi_eval, &dsiPllInit) != HAL_OK) 00316 { 00317 return(LCD_ERROR); 00318 } 00319 00320 PhyTimings.ClockLaneHS2LPTime = 33; /* Tclk-post + Tclk-trail + Ths-exit = [(60ns + 52xUI) + (60ns) + (300ns)]/16ns */ 00321 PhyTimings.ClockLaneLP2HSTime = 30; /* Tlpx + (Tclk-prepare + Tclk-zero) + Tclk-pre = [150ns + 300ns + 8xUI]/16ns */ 00322 PhyTimings.DataLaneHS2LPTime = 11; /* Ths-trail + Ths-exit = [(60ns + 4xUI) + 100ns]/16ns */ 00323 PhyTimings.DataLaneLP2HSTime = 21; /* Tlpx + (Ths-prepare + Ths-zero) + Ths-sync = [150ns + (145ns + 10xUI) + 8xUI]/16ns */ 00324 PhyTimings.DataLaneMaxReadTime = 0; 00325 PhyTimings.StopWaitTime = 7; 00326 if(HAL_DSI_ConfigPhyTimer(&hdsi_eval, &PhyTimings) != HAL_OK) 00327 { 00328 return(LCD_ERROR); 00329 } 00330 00331 HostTimeouts.TimeoutCkdiv = 1; 00332 HostTimeouts.HighSpeedTransmissionTimeout = 0; 00333 HostTimeouts.LowPowerReceptionTimeout = 0; 00334 HostTimeouts.HighSpeedReadTimeout = 0; 00335 HostTimeouts.LowPowerReadTimeout = 0; 00336 HostTimeouts.HighSpeedWriteTimeout = 0; 00337 HostTimeouts.HighSpeedWritePrespMode = 0; 00338 HostTimeouts.LowPowerWriteTimeout = 0; 00339 HostTimeouts.BTATimeout = 0; 00340 if(HAL_DSI_ConfigHostTimeouts(&hdsi_eval, &HostTimeouts) != HAL_OK) 00341 { 00342 return(LCD_ERROR); 00343 } 00344 00345 LPCmd.LPGenShortWriteNoP = DSI_LP_GSW0P_ENABLE; 00346 LPCmd.LPGenShortWriteOneP = DSI_LP_GSW1P_ENABLE; 00347 LPCmd.LPGenShortWriteTwoP = DSI_LP_GSW2P_ENABLE; 00348 LPCmd.LPGenShortReadNoP = DSI_LP_GSR0P_ENABLE; 00349 LPCmd.LPGenShortReadOneP = DSI_LP_GSR1P_ENABLE; 00350 LPCmd.LPGenShortReadTwoP = DSI_LP_GSR2P_ENABLE; 00351 LPCmd.LPGenLongWrite = DSI_LP_GLW_DISABLE; 00352 LPCmd.LPDcsShortWriteNoP = DSI_LP_DSW0P_ENABLE; 00353 LPCmd.LPDcsShortWriteOneP = DSI_LP_DSW1P_ENABLE; 00354 LPCmd.LPDcsShortReadNoP = DSI_LP_DSR0P_ENABLE; 00355 LPCmd.LPDcsLongWrite = DSI_LP_DLW_DISABLE; 00356 LPCmd.LPMaxReadPacket = DSI_LP_MRDP_DISABLE; 00357 LPCmd.AcknowledgeRequest = DSI_ACKNOWLEDGE_DISABLE; 00358 if(HAL_DSI_ConfigCommand(&hdsi_eval, &LPCmd) != HAL_OK) 00359 { 00360 return(LCD_ERROR); 00361 } 00362 00363 CmdCfg.VirtualChannelID = 0; 00364 CmdCfg.ColorCoding = DSI_RGB888; 00365 CmdCfg.CommandSize = 390; 00366 CmdCfg.TearingEffectSource = DSI_TE_DSILINK; 00367 CmdCfg.TearingEffectPolarity = DSI_TE_FALLING_EDGE; 00368 CmdCfg.HSPolarity = DSI_HSYNC_ACTIVE_LOW; 00369 CmdCfg.VSPolarity = DSI_VSYNC_ACTIVE_LOW; 00370 CmdCfg.DEPolarity = DSI_DATA_ENABLE_ACTIVE_HIGH; 00371 CmdCfg.VSyncPol = DSI_VSYNC_FALLING; 00372 CmdCfg.AutomaticRefresh = DSI_AR_ENABLE; 00373 CmdCfg.TEAcknowledgeRequest = DSI_TE_ACKNOWLEDGE_ENABLE; 00374 if(HAL_DSI_ConfigAdaptedCommandMode(&hdsi_eval, &CmdCfg) != HAL_OK) 00375 { 00376 return(LCD_ERROR); 00377 } 00378 00379 /* Disable the Tearing Effect interrupt activated by default on previous function */ 00380 __HAL_DSI_DISABLE_IT(&hdsi_eval, DSI_IT_TE); 00381 00382 if(HAL_DSI_ConfigFlowControl(&hdsi_eval, DSI_FLOW_CONTROL_BTA) != HAL_OK) 00383 { 00384 return(LCD_ERROR); 00385 } 00386 00387 /* Enable DSI */ 00388 __HAL_DSI_ENABLE(&hdsi_eval); 00389 00390 /*************************/ 00391 /* LCD POWER ON SEQUENCE */ 00392 /*************************/ 00393 /* Step 1 */ 00394 /* Go to command 2 */ 00395 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0xFE, 0x01); 00396 /* IC Frame rate control, set power, sw mapping, mux swithc timing command */ 00397 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x06, 0x62); 00398 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x0E, 0x80); 00399 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x0F, 0x80); 00400 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x10, 0x71); 00401 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x13, 0x81); 00402 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x14, 0x81); 00403 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x15, 0x82); 00404 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x16, 0x82); 00405 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x18, 0x88); 00406 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x19, 0x55); 00407 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x1A, 0x10); 00408 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x1C, 0x99); 00409 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x1D, 0x03); 00410 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x1E, 0x03); 00411 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x1F, 0x03); 00412 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x20, 0x03); 00413 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x25, 0x03); 00414 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x26, 0x8D); 00415 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x2A, 0x03); 00416 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x2B, 0x8D); 00417 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x36, 0x00); 00418 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x37, 0x10); 00419 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x3A, 0x00); 00420 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x3B, 0x00); 00421 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x3D, 0x20); 00422 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x3F, 0x3A); 00423 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x40, 0x30); 00424 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x41, 0x1A); 00425 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x42, 0x33); 00426 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x43, 0x22); 00427 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x44, 0x11); 00428 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x45, 0x66); 00429 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x46, 0x55); 00430 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x47, 0x44); 00431 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x4C, 0x33); 00432 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x4D, 0x22); 00433 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x4E, 0x11); 00434 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x4F, 0x66); 00435 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x50, 0x55); 00436 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x51, 0x44); 00437 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x57, 0x33); 00438 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x6B, 0x1B); 00439 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x70, 0x55); 00440 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x74, 0x0C); 00441 00442 /* Go to command 3 */ 00443 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0xFE, 0x02); 00444 /* Set the VGMP/VGSP coltage control */ 00445 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x9B, 0x40); 00446 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x9C, 0x00); 00447 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x9D, 0x20); 00448 00449 /* Go to command 4 */ 00450 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0xFE, 0x03); 00451 /* Set the VGMP/VGSP coltage control */ 00452 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x9B, 0x40); 00453 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x9C, 0x00); 00454 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x9D, 0x20); 00455 00456 00457 /* Go to command 5 */ 00458 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0xFE, 0x04); 00459 /* VSR command */ 00460 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x5D, 0x10); 00461 /* VSR1 timing set */ 00462 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x00, 0x8D); 00463 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x01, 0x00); 00464 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x02, 0x01); 00465 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x03, 0x01); 00466 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x04, 0x10); 00467 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x05, 0x01); 00468 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x06, 0xA7); 00469 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x07, 0x20); 00470 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x08, 0x00); 00471 /* VSR2 timing set */ 00472 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x09, 0xC2); 00473 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x0A, 0x00); 00474 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x0B, 0x02); 00475 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x0C, 0x01); 00476 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x0D, 0x40); 00477 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x0E, 0x06); 00478 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x0F, 0x01); 00479 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x10, 0xA7); 00480 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x11, 0x00); 00481 /* VSR3 timing set */ 00482 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x12, 0xC2); 00483 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x13, 0x00); 00484 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x14, 0x02); 00485 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x15, 0x01); 00486 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x16, 0x40); 00487 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x17, 0x07); 00488 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x18, 0x01); 00489 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x19, 0xA7); 00490 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x1A, 0x00); 00491 /* VSR4 timing set */ 00492 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x1B, 0x82); 00493 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x1C, 0x00); 00494 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x1D, 0xFF); 00495 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x1E, 0x05); 00496 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x1F, 0x60); 00497 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x20, 0x02); 00498 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x21, 0x01); 00499 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x22, 0x7C); 00500 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x23, 0x00); 00501 /* VSR5 timing set */ 00502 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x24, 0xC2); 00503 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x25, 0x00); 00504 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x26, 0x04); 00505 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x27, 0x02); 00506 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x28, 0x70); 00507 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x29, 0x05); 00508 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x2A, 0x74); 00509 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x2B, 0x8D); 00510 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x2D, 0x00); 00511 /* VSR6 timing set */ 00512 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x2F, 0xC2); 00513 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x30, 0x00); 00514 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x31, 0x04); 00515 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x32, 0x02); 00516 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x33, 0x70); 00517 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x34, 0x07); 00518 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x35, 0x74); 00519 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x36, 0x8D); 00520 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x37, 0x00); 00521 /* VSR marping command */ 00522 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x5E, 0x20); 00523 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x5F, 0x31); 00524 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x60, 0x54); 00525 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x61, 0x76); 00526 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x62, 0x98); 00527 00528 /* Go to command 6 */ 00529 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0xFE, 0x05); 00530 /* Set the ELVSS voltage */ 00531 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x05, 0x17); 00532 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x2A, 0x04); 00533 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0x91, 0x00); 00534 00535 /* Go back in standard commands */ 00536 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0xFE, 0x00); 00537 00538 /* Set tear off */ 00539 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, DSI_SET_TEAR_OFF, 0x0); 00540 00541 /* Set DSI mode to internal timing added vs ORIGINAL for Command mode */ 00542 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, 0xC2, 0x0); 00543 00544 /* Set memory address MODIFIED vs ORIGINAL */ 00545 uint8_t InitParam1[4]= {0x00, 0x04, 0x01, 0x89}; // MODIF OFe: adjusted w/ real image 00546 HAL_DSI_LongWrite(&hdsi_eval, 0, DSI_DCS_LONG_PKT_WRITE, 4, DSI_SET_COLUMN_ADDRESS, InitParam1); 00547 uint8_t InitParam2[4]= {0x00, 0x00, 0x01, 0x85}; 00548 HAL_DSI_LongWrite(&hdsi_eval, 0, DSI_DCS_LONG_PKT_WRITE, 4, DSI_SET_PAGE_ADDRESS, InitParam2); 00549 00550 /* Sleep out */ 00551 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P0, DSI_EXIT_SLEEP_MODE, 0x0); 00552 00553 HAL_Delay(120); 00554 00555 /* Set display on */ 00556 if(HAL_DSI_ShortWrite(&hdsi_eval, 00557 0, 00558 DSI_DCS_SHORT_PKT_WRITE_P0, 00559 DSI_SET_DISPLAY_ON, 00560 0x0) != HAL_OK) 00561 { 00562 return(LCD_ERROR); 00563 } 00564 00565 /* Enable DSI Wrapper */ 00566 __HAL_DSI_WRAPPER_ENABLE(&hdsi_eval); 00567 00568 /* Initialize the font */ 00569 BSP_DSI_LCD_SetFont(&LCD_DEFAULT_FONT); 00570 00571 bsp_lcd_initialized = 1; 00572 } 00573 00574 return(LCD_OK); 00575 } 00576 00577 /** 00578 * @brief De-initialize the DSI LCD. 00579 * @retval LCD state 00580 */ 00581 uint8_t BSP_DSI_LCD_DeInit(void) 00582 { 00583 if(bsp_lcd_initialized == 1) 00584 { 00585 /* Disable DSI wrapper */ 00586 __HAL_DSI_WRAPPER_DISABLE(&hdsi_eval); 00587 00588 /* Set display off */ 00589 if(HAL_DSI_ShortWrite(&hdsi_eval, 00590 0, 00591 DSI_DCS_SHORT_PKT_WRITE_P0, 00592 DSI_SET_DISPLAY_OFF, 00593 0x0) != HAL_OK) 00594 { 00595 return(LCD_ERROR); 00596 } 00597 00598 /* Wait before entering in sleep mode */ 00599 HAL_Delay(2000); 00600 00601 /* Put LCD in sleep mode */ 00602 if(HAL_DSI_ShortWrite(&hdsi_eval, 00603 0, 00604 DSI_DCS_SHORT_PKT_WRITE_P0, 00605 DSI_ENTER_SLEEP_MODE, 00606 0x0) != HAL_OK) 00607 { 00608 return(LCD_ERROR); 00609 } 00610 00611 HAL_Delay(120); 00612 00613 /* De-initialize DSI */ 00614 if(HAL_DSI_DeInit(&hdsi_eval) != HAL_OK) 00615 { 00616 return(LCD_ERROR); 00617 } 00618 00619 /* De-initialize LTDC */ 00620 if(HAL_LTDC_DeInit(&hltdc_eval) != HAL_OK) 00621 { 00622 return(LCD_ERROR); 00623 } 00624 00625 /* De-initialize GFXMMU */ 00626 if(HAL_GFXMMU_DeInit(&hgfxmmu_eval) != HAL_OK) 00627 { 00628 return(LCD_ERROR); 00629 } 00630 00631 /* Call MSP de-initialize function */ 00632 BSP_DSI_LCD_MspDeInit(); 00633 00634 bsp_lcd_initialized = 0; 00635 } 00636 00637 return(LCD_OK); 00638 } 00639 00640 /** 00641 * @brief Gets the LCD X size. 00642 * @retval Used LCD X size 00643 */ 00644 uint32_t BSP_DSI_LCD_GetXSize(void) 00645 { 00646 return (lcd_x_size); 00647 } 00648 00649 /** 00650 * @brief Gets the LCD Y size. 00651 * @retval Used LCD Y size 00652 */ 00653 uint32_t BSP_DSI_LCD_GetYSize(void) 00654 { 00655 return (lcd_y_size); 00656 } 00657 00658 /** 00659 * @brief Selects the LCD Layer. 00660 * @param LayerIndex: Layer foreground (1) or background (0) 00661 * @note : Only backgroung layer can be used. 00662 * @retval LCD state 00663 */ 00664 uint8_t BSP_DSI_LCD_SelectLayer(uint32_t LayerIndex) 00665 { 00666 uint8_t status = LCD_OK; 00667 00668 if(LayerIndex == LTDC_ACTIVE_LAYER_BACKGROUND) 00669 { 00670 ActiveLayer = LayerIndex; 00671 } 00672 else 00673 { 00674 status = LCD_ERROR; 00675 } 00676 return(status); 00677 } 00678 00679 /** 00680 * @brief Sets an LCD Layer visible 00681 * @param LayerIndex: Visible Layer 00682 * @param State: New state of the specified layer 00683 * This parameter can be one of the following values: 00684 * @arg ENABLE 00685 * @arg DISABLE 00686 * @note : Only backgroung layer can be used. 00687 * @retval LCD state 00688 */ 00689 uint8_t BSP_DSI_LCD_SetLayerVisible(uint32_t LayerIndex, FunctionalState State) 00690 { 00691 uint8_t status = LCD_OK; 00692 00693 if(LayerIndex == LTDC_ACTIVE_LAYER_BACKGROUND) 00694 { 00695 if(State == ENABLE) 00696 { 00697 __HAL_LTDC_LAYER_ENABLE(&(hltdc_eval), LayerIndex); 00698 } 00699 else 00700 { 00701 __HAL_LTDC_LAYER_DISABLE(&(hltdc_eval), LayerIndex); 00702 } 00703 __HAL_LTDC_RELOAD_IMMEDIATE_CONFIG(&(hltdc_eval)); 00704 } 00705 else 00706 { 00707 status = LCD_ERROR; 00708 } 00709 return(status); 00710 } 00711 00712 /** 00713 * @brief Configures the transparency. 00714 * @param LayerIndex: Layer foreground or background. 00715 * @param Transparency: Transparency 00716 * This parameter must be a number between Min_Data = 0x00 and Max_Data = 0xFF 00717 * @note : Only backgroung layer can be used. 00718 * @retval LCD state 00719 */ 00720 uint8_t BSP_DSI_LCD_SetTransparency(uint32_t LayerIndex, uint8_t Transparency) 00721 { 00722 uint8_t status = LCD_OK; 00723 00724 if(LayerIndex == LTDC_ACTIVE_LAYER_BACKGROUND) 00725 { 00726 HAL_LTDC_SetAlpha(&(hltdc_eval), Transparency, LayerIndex); 00727 } 00728 else 00729 { 00730 status = LCD_ERROR; 00731 } 00732 return(status); 00733 } 00734 00735 /** 00736 * @brief Configures and sets the color keying. 00737 * @param LayerIndex: Layer foreground (1) or background (0) 00738 * @param RGBValue: Color reference 00739 * @note : Only backgroung layer can be used. 00740 * @retval LCD state 00741 */ 00742 uint8_t BSP_DSI_LCD_SetColorKeying(uint32_t LayerIndex, uint32_t RGBValue) 00743 { 00744 uint8_t status = LCD_OK; 00745 00746 if(LayerIndex == LTDC_ACTIVE_LAYER_BACKGROUND) 00747 { 00748 /* Configure and Enable the color Keying for LCD Layer */ 00749 HAL_LTDC_ConfigColorKeying(&(hltdc_eval), RGBValue, LayerIndex); 00750 HAL_LTDC_EnableColorKeying(&(hltdc_eval), LayerIndex); 00751 } 00752 else 00753 { 00754 status = LCD_ERROR; 00755 } 00756 return(status); 00757 } 00758 00759 /** 00760 * @brief Disables the color keying. 00761 * @param LayerIndex: Layer foreground (1) or background (0) 00762 * @note : Only backgroung layer can be used. 00763 * @retval LCD state 00764 */ 00765 uint8_t BSP_DSI_LCD_ResetColorKeying(uint32_t LayerIndex) 00766 { 00767 uint8_t status = LCD_OK; 00768 00769 if(LayerIndex == LTDC_ACTIVE_LAYER_BACKGROUND) 00770 { 00771 /* Disable the color Keying for LCD Layer */ 00772 HAL_LTDC_DisableColorKeying(&(hltdc_eval), LayerIndex); 00773 } 00774 else 00775 { 00776 status = LCD_ERROR; 00777 } 00778 return(status); 00779 } 00780 00781 /** 00782 * @brief Sets the LCD text color. 00783 * @param Color: Text color code ARGB8888 00784 */ 00785 void BSP_DSI_LCD_SetTextColor(uint32_t Color) 00786 { 00787 DrawProp[ActiveLayer].TextColor = Color; 00788 } 00789 00790 /** 00791 * @brief Gets the LCD text color. 00792 * @retval Used text color. 00793 */ 00794 uint32_t BSP_DSI_LCD_GetTextColor(void) 00795 { 00796 return DrawProp[ActiveLayer].TextColor; 00797 } 00798 00799 /** 00800 * @brief Sets the LCD background color. 00801 * @param Color: Layer background color code ARGB8888 00802 */ 00803 void BSP_DSI_LCD_SetBackColor(uint32_t Color) 00804 { 00805 DrawProp[ActiveLayer].BackColor = Color; 00806 } 00807 00808 /** 00809 * @brief Gets the LCD background color. 00810 * @retval Used background color 00811 */ 00812 uint32_t BSP_DSI_LCD_GetBackColor(void) 00813 { 00814 return DrawProp[ActiveLayer].BackColor; 00815 } 00816 00817 /** 00818 * @brief Sets the LCD text font. 00819 * @param fonts: Layer font to be used 00820 */ 00821 void BSP_DSI_LCD_SetFont(sFONT *fonts) 00822 { 00823 DrawProp[ActiveLayer].pFont = fonts; 00824 } 00825 00826 /** 00827 * @brief Gets the LCD text font. 00828 * @retval Used layer font 00829 */ 00830 sFONT *BSP_DSI_LCD_GetFont(void) 00831 { 00832 return DrawProp[ActiveLayer].pFont; 00833 } 00834 00835 /** 00836 * @brief Reads an LCD pixel. 00837 * @param Xpos: X position 00838 * @param Ypos: Y position 00839 * @retval ARGB8888 pixel color 00840 */ 00841 uint32_t BSP_DSI_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00842 { 00843 uint32_t ret = 0; 00844 00845 /* Read value of corresponding pixel */ 00846 /* We have 768 pixels per line and 4 bytes per pixel so 3072 bytes per line */ 00847 ret = *(__IO uint32_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (4*(Ypos*768 + Xpos))); 00848 00849 /* Return pixel value */ 00850 return ret; 00851 } 00852 00853 /** 00854 * @brief Clears the whole currently active layer of LTDC. 00855 * @param Color: Color of the background (in ARGB8888 format) 00856 */ 00857 void BSP_DSI_LCD_Clear(uint32_t Color) 00858 { 00859 /* Clear the LCD */ 00860 /* Offset line is 768 - 390 = 378 */ 00861 LL_FillBuffer(ActiveLayer, (uint32_t *)(hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress), 390, 390, 378, Color); 00862 } 00863 00864 /** 00865 * @brief Clears the selected line in currently active layer. 00866 * @param Line: Line to be cleared 00867 */ 00868 void BSP_DSI_LCD_ClearStringLine(uint32_t Line) 00869 { 00870 uint32_t color_backup = DrawProp[ActiveLayer].TextColor; 00871 DrawProp[ActiveLayer].TextColor = DrawProp[ActiveLayer].BackColor; 00872 00873 /* Draw rectangle with background color */ 00874 BSP_DSI_LCD_FillRect(0, (Line * DrawProp[ActiveLayer].pFont->Height), 390, DrawProp[ActiveLayer].pFont->Height); 00875 00876 DrawProp[ActiveLayer].TextColor = color_backup; 00877 BSP_DSI_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 00878 } 00879 00880 /** 00881 * @brief Displays one character in currently active layer. 00882 * @param Xpos: Start column address 00883 * @param Ypos: Line where to display the character shape. 00884 * @param Ascii: Character ascii code 00885 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00886 */ 00887 void BSP_DSI_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00888 { 00889 DrawChar(Xpos, Ypos, &DrawProp[ActiveLayer].pFont->table[(Ascii-' ') * DrawProp[ActiveLayer].pFont->Height * ((DrawProp[ActiveLayer].pFont->Width + 7) / 8)]); 00890 } 00891 00892 /** 00893 * @brief Displays characters in currently active layer. 00894 * @param Xpos: X position (in pixel) 00895 * @param Ypos: Y position (in pixel) 00896 * @param Text: Pointer to string to display on LCD 00897 * @param Mode: Display mode 00898 * This parameter can be one of the following values: 00899 * @arg CENTER_MODE 00900 * @arg RIGHT_MODE 00901 * @arg LEFT_MODE 00902 */ 00903 void BSP_DSI_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Text_AlignModeTypdef Mode) 00904 { 00905 uint16_t refcolumn = 1, i = 0; 00906 uint32_t size = 0, xsize = 0; 00907 uint8_t *ptr = Text; 00908 00909 /* Get the text size */ 00910 while (*ptr++) size ++ ; 00911 00912 /* Characters number per line */ 00913 xsize = (390/DrawProp[ActiveLayer].pFont->Width); 00914 00915 switch (Mode) 00916 { 00917 case CENTER_MODE: 00918 { 00919 refcolumn = Xpos + ((xsize - size)* DrawProp[ActiveLayer].pFont->Width) / 2; 00920 break; 00921 } 00922 case LEFT_MODE: 00923 { 00924 refcolumn = Xpos; 00925 break; 00926 } 00927 case RIGHT_MODE: 00928 { 00929 refcolumn = - Xpos + ((xsize - size)*DrawProp[ActiveLayer].pFont->Width); 00930 break; 00931 } 00932 default: 00933 { 00934 refcolumn = Xpos; 00935 break; 00936 } 00937 } 00938 00939 /* Check that the Start column is located in the screen */ 00940 if ((refcolumn < 1) || (refcolumn >= 0x8000)) 00941 { 00942 refcolumn = 1; 00943 } 00944 00945 /* Send the string character by character on LCD */ 00946 while ((*Text != 0) & (((390 - (i*DrawProp[ActiveLayer].pFont->Width)) & 0xFFFF) >= DrawProp[ActiveLayer].pFont->Width)) 00947 { 00948 /* Display one character on LCD */ 00949 BSP_DSI_LCD_DisplayChar(refcolumn, Ypos, *Text); 00950 /* Decrement the column position by 16 */ 00951 refcolumn += DrawProp[ActiveLayer].pFont->Width; 00952 00953 /* Point on the next character */ 00954 Text++; 00955 i++; 00956 } 00957 00958 } 00959 00960 /** 00961 * @brief Displays string on specified line of the LCD. 00962 * @param Line: Line where to display the character shape 00963 * @param ptr: Pointer to string to display on LCD 00964 */ 00965 void BSP_DSI_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr) 00966 { 00967 BSP_DSI_LCD_DisplayStringAt(0, Line * (((sFONT *)BSP_DSI_LCD_GetFont())->Height), ptr, CENTER_MODE); 00968 } 00969 00970 /** 00971 * @brief Draws an horizontal line in currently active layer. 00972 * @param Xpos: X position 00973 * @param Ypos: Y position 00974 * @param Length: Line length 00975 */ 00976 void BSP_DSI_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00977 { 00978 uint32_t Xaddress = 0; 00979 00980 /* Get the line address */ 00981 Xaddress = (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress) + 4*(768*Ypos + Xpos); 00982 00983 /* Write line */ 00984 LL_FillBuffer(ActiveLayer, (uint32_t *)Xaddress, Length, 1, (768 - Length), DrawProp[ActiveLayer].TextColor); 00985 } 00986 00987 /** 00988 * @brief Draws a vertical line in currently active layer. 00989 * @param Xpos: X position 00990 * @param Ypos: Y position 00991 * @param Length: Line length 00992 */ 00993 void BSP_DSI_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00994 { 00995 uint32_t Xaddress = 0; 00996 00997 /* Get the line address */ 00998 Xaddress = (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress) + 4*(768*Ypos + Xpos); 00999 01000 /* Write line */ 01001 LL_FillBuffer(ActiveLayer, (uint32_t *)Xaddress, 1, Length, (768 - 1), DrawProp[ActiveLayer].TextColor); 01002 } 01003 01004 /** 01005 * @brief Draws an uni-line (between two points) in currently active layer. 01006 * @param x1: Point 1 X position 01007 * @param y1: Point 1 Y position 01008 * @param x2: Point 2 X position 01009 * @param y2: Point 2 Y position 01010 */ 01011 void BSP_DSI_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) 01012 { 01013 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 01014 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 01015 curpixel = 0; 01016 01017 deltax = ABS(x2 - x1); /* The difference between the x's */ 01018 deltay = ABS(y2 - y1); /* The difference between the y's */ 01019 x = x1; /* Start x off at the first pixel */ 01020 y = y1; /* Start y off at the first pixel */ 01021 01022 if (x2 >= x1) /* The x-values are increasing */ 01023 { 01024 xinc1 = 1; 01025 xinc2 = 1; 01026 } 01027 else /* The x-values are decreasing */ 01028 { 01029 xinc1 = -1; 01030 xinc2 = -1; 01031 } 01032 01033 if (y2 >= y1) /* The y-values are increasing */ 01034 { 01035 yinc1 = 1; 01036 yinc2 = 1; 01037 } 01038 else /* The y-values are decreasing */ 01039 { 01040 yinc1 = -1; 01041 yinc2 = -1; 01042 } 01043 01044 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 01045 { 01046 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 01047 yinc2 = 0; /* Don't change the y for every iteration */ 01048 den = deltax; 01049 num = deltax / 2; 01050 numadd = deltay; 01051 numpixels = deltax; /* There are more x-values than y-values */ 01052 } 01053 else /* There is at least one y-value for every x-value */ 01054 { 01055 xinc2 = 0; /* Don't change the x for every iteration */ 01056 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 01057 den = deltay; 01058 num = deltay / 2; 01059 numadd = deltax; 01060 numpixels = deltay; /* There are more y-values than x-values */ 01061 } 01062 01063 for (curpixel = 0; curpixel <= numpixels; curpixel++) 01064 { 01065 BSP_DSI_LCD_DrawPixel(x, y, DrawProp[ActiveLayer].TextColor); /* Draw the current pixel */ 01066 num += numadd; /* Increase the numerator by the top of the fraction */ 01067 if (num >= den) /* Check if numerator >= denominator */ 01068 { 01069 num -= den; /* Calculate the new numerator value */ 01070 x += xinc1; /* Change the x as appropriate */ 01071 y += yinc1; /* Change the y as appropriate */ 01072 } 01073 x += xinc2; /* Change the x as appropriate */ 01074 y += yinc2; /* Change the y as appropriate */ 01075 } 01076 } 01077 01078 /** 01079 * @brief Draws a rectangle in currently active layer. 01080 * @param Xpos: X position 01081 * @param Ypos: Y position 01082 * @param Width: Rectangle width 01083 * @param Height: Rectangle height 01084 */ 01085 void BSP_DSI_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 01086 { 01087 /* Draw horizontal lines */ 01088 BSP_DSI_LCD_DrawHLine(Xpos, Ypos, Width); 01089 BSP_DSI_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 01090 01091 /* Draw vertical lines */ 01092 BSP_DSI_LCD_DrawVLine(Xpos, Ypos, Height); 01093 BSP_DSI_LCD_DrawVLine((Xpos + Width), Ypos, Height); 01094 } 01095 01096 /** 01097 * @brief Draws a circle in currently active layer. 01098 * @param Xpos: X position 01099 * @param Ypos: Y position 01100 * @param Radius: Circle radius 01101 */ 01102 void BSP_DSI_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 01103 { 01104 int32_t D; /* Decision Variable */ 01105 uint32_t CurX; /* Current X Value */ 01106 uint32_t CurY; /* Current Y Value */ 01107 01108 D = 3 - (Radius << 1); 01109 CurX = 0; 01110 CurY = Radius; 01111 01112 while (CurX <= CurY) 01113 { 01114 BSP_DSI_LCD_DrawPixel((Xpos + CurX), (Ypos - CurY), DrawProp[ActiveLayer].TextColor); 01115 01116 BSP_DSI_LCD_DrawPixel((Xpos - CurX), (Ypos - CurY), DrawProp[ActiveLayer].TextColor); 01117 01118 BSP_DSI_LCD_DrawPixel((Xpos + CurY), (Ypos - CurX), DrawProp[ActiveLayer].TextColor); 01119 01120 BSP_DSI_LCD_DrawPixel((Xpos - CurY), (Ypos - CurX), DrawProp[ActiveLayer].TextColor); 01121 01122 BSP_DSI_LCD_DrawPixel((Xpos + CurX), (Ypos + CurY), DrawProp[ActiveLayer].TextColor); 01123 01124 BSP_DSI_LCD_DrawPixel((Xpos - CurX), (Ypos + CurY), DrawProp[ActiveLayer].TextColor); 01125 01126 BSP_DSI_LCD_DrawPixel((Xpos + CurY), (Ypos + CurX), DrawProp[ActiveLayer].TextColor); 01127 01128 BSP_DSI_LCD_DrawPixel((Xpos - CurY), (Ypos + CurX), DrawProp[ActiveLayer].TextColor); 01129 01130 if (D < 0) 01131 { 01132 D += (CurX << 2) + 6; 01133 } 01134 else 01135 { 01136 D += ((CurX - CurY) << 2) + 10; 01137 CurY--; 01138 } 01139 CurX++; 01140 } 01141 } 01142 01143 /** 01144 * @brief Draws an poly-line (between many points) in currently active layer. 01145 * @param Points: Pointer to the points array 01146 * @param PointCount: Number of points 01147 */ 01148 void BSP_DSI_LCD_DrawPolygon(pPoint Points, uint16_t PointCount) 01149 { 01150 int16_t X = 0, Y = 0; 01151 01152 if(PointCount < 2) 01153 { 01154 return; 01155 } 01156 01157 BSP_DSI_LCD_DrawLine(Points->X, Points->Y, (Points+PointCount-1)->X, (Points+PointCount-1)->Y); 01158 01159 while(--PointCount) 01160 { 01161 X = Points->X; 01162 Y = Points->Y; 01163 Points++; 01164 BSP_DSI_LCD_DrawLine(X, Y, Points->X, Points->Y); 01165 } 01166 } 01167 01168 /** 01169 * @brief Draws an ellipse on LCD in currently active layer. 01170 * @param Xpos: X position 01171 * @param Ypos: Y position 01172 * @param XRadius: Ellipse X radius 01173 * @param YRadius: Ellipse Y radius 01174 */ 01175 void BSP_DSI_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 01176 { 01177 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 01178 float K = 0, rad1 = 0, rad2 = 0; 01179 01180 rad1 = XRadius; 01181 rad2 = YRadius; 01182 01183 K = (float)(rad2/rad1); 01184 01185 do { 01186 BSP_DSI_LCD_DrawPixel((Xpos-(uint16_t)(x/K)), (Ypos+y), DrawProp[ActiveLayer].TextColor); 01187 BSP_DSI_LCD_DrawPixel((Xpos+(uint16_t)(x/K)), (Ypos+y), DrawProp[ActiveLayer].TextColor); 01188 BSP_DSI_LCD_DrawPixel((Xpos+(uint16_t)(x/K)), (Ypos-y), DrawProp[ActiveLayer].TextColor); 01189 BSP_DSI_LCD_DrawPixel((Xpos-(uint16_t)(x/K)), (Ypos-y), DrawProp[ActiveLayer].TextColor); 01190 01191 e2 = err; 01192 if (e2 <= x) { 01193 err += ++x*2+1; 01194 if (-y == x && e2 <= y) e2 = 0; 01195 } 01196 if (e2 > y) err += ++y*2+1; 01197 } 01198 while (y <= 0); 01199 } 01200 01201 /** 01202 * @brief Draws a bitmap picture loaded in the internal Flash (32 bpp) in currently active layer. 01203 * @param Xpos: Bmp X position in the LCD 01204 * @param Ypos: Bmp Y position in the LCD 01205 * @param pbmp: Pointer to Bmp picture address in the internal Flash 01206 */ 01207 void BSP_DSI_LCD_DrawBitmap(uint32_t Xpos, uint32_t Ypos, uint8_t *pbmp) 01208 { 01209 uint32_t index = 0, width = 0, height = 0, bit_pixel = 0; 01210 uint32_t Address; 01211 uint32_t InputColorMode = 0; 01212 01213 /* Get bitmap data address offset */ 01214 index = *(__IO uint16_t *) (pbmp + 10); 01215 index |= (*(__IO uint16_t *) (pbmp + 12)) << 16; 01216 01217 /* Read bitmap width */ 01218 width = *(uint16_t *) (pbmp + 18); 01219 width |= (*(uint16_t *) (pbmp + 20)) << 16; 01220 01221 /* Read bitmap height */ 01222 height = *(uint16_t *) (pbmp + 22); 01223 height |= (*(uint16_t *) (pbmp + 24)) << 16; 01224 01225 /* Read bit/pixel */ 01226 bit_pixel = *(uint16_t *) (pbmp + 28); 01227 01228 /* Set the address */ 01229 Address = hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (((768*Ypos) + Xpos)*(4)); 01230 01231 /* Get the layer pixel format */ 01232 if ((bit_pixel/8) == 4) 01233 { 01234 InputColorMode = DMA2D_INPUT_ARGB8888; 01235 } 01236 else if ((bit_pixel/8) == 2) 01237 { 01238 InputColorMode = DMA2D_INPUT_RGB565; 01239 } 01240 else 01241 { 01242 InputColorMode = DMA2D_INPUT_RGB888; 01243 } 01244 01245 /* Bypass the bitmap header */ 01246 pbmp += (index + (width * (height - 1) * (bit_pixel/8))); 01247 01248 /* Convert picture to ARGB8888 pixel format */ 01249 for(index=0; index < height; index++) 01250 { 01251 /* Pixel format conversion */ 01252 LL_ConvertLineToARGB8888((uint32_t *)pbmp, (uint32_t *)Address, width, InputColorMode); 01253 01254 /* Increment the source and destination buffers */ 01255 Address+= (768*4); 01256 pbmp -= width*(bit_pixel/8); 01257 } 01258 } 01259 01260 /** 01261 * @brief Draws a full rectangle in currently active layer. 01262 * @param Xpos: X position 01263 * @param Ypos: Y position 01264 * @param Width: Rectangle width 01265 * @param Height: Rectangle height 01266 */ 01267 void BSP_DSI_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 01268 { 01269 uint32_t Xaddress = 0; 01270 01271 /* Set the text color */ 01272 BSP_DSI_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 01273 01274 /* Get the rectangle start address */ 01275 Xaddress = (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress) + 4*(768*Ypos + Xpos); 01276 01277 /* Fill the rectangle */ 01278 LL_FillBuffer(ActiveLayer, (uint32_t *)Xaddress, Width, Height, (768 - Width), DrawProp[ActiveLayer].TextColor); 01279 } 01280 01281 /** 01282 * @brief Draws a full circle in currently active layer. 01283 * @param Xpos: X position 01284 * @param Ypos: Y position 01285 * @param Radius: Circle radius 01286 */ 01287 void BSP_DSI_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 01288 { 01289 int32_t D; /* Decision Variable */ 01290 uint32_t CurX; /* Current X Value */ 01291 uint32_t CurY; /* Current Y Value */ 01292 01293 D = 3 - (Radius << 1); 01294 01295 CurX = 0; 01296 CurY = Radius; 01297 01298 BSP_DSI_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 01299 01300 while (CurX <= CurY) 01301 { 01302 if(CurY > 0) 01303 { 01304 BSP_DSI_LCD_DrawHLine(Xpos - CurY, Ypos + CurX, 2*CurY); 01305 BSP_DSI_LCD_DrawHLine(Xpos - CurY, Ypos - CurX, 2*CurY); 01306 } 01307 01308 if(CurX > 0) 01309 { 01310 BSP_DSI_LCD_DrawHLine(Xpos - CurX, Ypos - CurY, 2*CurX); 01311 BSP_DSI_LCD_DrawHLine(Xpos - CurX, Ypos + CurY, 2*CurX); 01312 } 01313 if (D < 0) 01314 { 01315 D += (CurX << 2) + 6; 01316 } 01317 else 01318 { 01319 D += ((CurX - CurY) << 2) + 10; 01320 CurY--; 01321 } 01322 CurX++; 01323 } 01324 01325 BSP_DSI_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 01326 BSP_DSI_LCD_DrawCircle(Xpos, Ypos, Radius); 01327 } 01328 01329 /** 01330 * @brief Draws a full poly-line (between many points) in currently active layer. 01331 * @param Points: Pointer to the points array 01332 * @param PointCount: Number of points 01333 */ 01334 void BSP_DSI_LCD_FillPolygon(pPoint Points, uint16_t PointCount) 01335 { 01336 int16_t X = 0, Y = 0, X2 = 0, Y2 = 0, X_center = 0, Y_center = 0, X_first = 0, Y_first = 0, pixelX = 0, pixelY = 0, counter = 0; 01337 uint16_t IMAGE_LEFT = 0, IMAGE_RIGHT = 0, IMAGE_TOP = 0, IMAGE_BOTTOM = 0; 01338 01339 IMAGE_LEFT = IMAGE_RIGHT = Points->X; 01340 IMAGE_TOP= IMAGE_BOTTOM = Points->Y; 01341 01342 for(counter = 1; counter < PointCount; counter++) 01343 { 01344 pixelX = POLY_X(counter); 01345 if(pixelX < IMAGE_LEFT) 01346 { 01347 IMAGE_LEFT = pixelX; 01348 } 01349 if(pixelX > IMAGE_RIGHT) 01350 { 01351 IMAGE_RIGHT = pixelX; 01352 } 01353 01354 pixelY = POLY_Y(counter); 01355 if(pixelY < IMAGE_TOP) 01356 { 01357 IMAGE_TOP = pixelY; 01358 } 01359 if(pixelY > IMAGE_BOTTOM) 01360 { 01361 IMAGE_BOTTOM = pixelY; 01362 } 01363 } 01364 01365 if(PointCount < 2) 01366 { 01367 return; 01368 } 01369 01370 X_center = (IMAGE_LEFT + IMAGE_RIGHT)/2; 01371 Y_center = (IMAGE_BOTTOM + IMAGE_TOP)/2; 01372 01373 X_first = Points->X; 01374 Y_first = Points->Y; 01375 01376 while(--PointCount) 01377 { 01378 X = Points->X; 01379 Y = Points->Y; 01380 Points++; 01381 X2 = Points->X; 01382 Y2 = Points->Y; 01383 01384 FillTriangle(X, X2, X_center, Y, Y2, Y_center); 01385 FillTriangle(X, X_center, X2, Y, Y_center, Y2); 01386 FillTriangle(X_center, X2, X, Y_center, Y2, Y); 01387 } 01388 01389 FillTriangle(X_first, X2, X_center, Y_first, Y2, Y_center); 01390 FillTriangle(X_first, X_center, X2, Y_first, Y_center, Y2); 01391 FillTriangle(X_center, X2, X_first, Y_center, Y2, Y_first); 01392 } 01393 01394 /** 01395 * @brief Draws a full ellipse in currently active layer. 01396 * @param Xpos: X position 01397 * @param Ypos: Y position 01398 * @param XRadius: Ellipse X radius 01399 * @param YRadius: Ellipse Y radius 01400 */ 01401 void BSP_DSI_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 01402 { 01403 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 01404 float K = 0, rad1 = 0, rad2 = 0; 01405 01406 rad1 = XRadius; 01407 rad2 = YRadius; 01408 01409 K = (float)(rad2/rad1); 01410 01411 do 01412 { 01413 BSP_DSI_LCD_DrawHLine((Xpos-(uint16_t)(x/K)), (Ypos+y), (2*(uint16_t)(x/K) + 1)); 01414 BSP_DSI_LCD_DrawHLine((Xpos-(uint16_t)(x/K)), (Ypos-y), (2*(uint16_t)(x/K) + 1)); 01415 01416 e2 = err; 01417 if (e2 <= x) 01418 { 01419 err += ++x*2+1; 01420 if (-y == x && e2 <= y) e2 = 0; 01421 } 01422 if (e2 > y) err += ++y*2+1; 01423 } 01424 while (y <= 0); 01425 } 01426 01427 /** 01428 * @brief Draws a pixel on LCD. 01429 * @param Xpos: X position 01430 * @param Ypos: Y position 01431 * @param RGB_Code: Pixel color in ARGB mode (8-8-8-8) 01432 */ 01433 void BSP_DSI_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint32_t RGB_Code) 01434 { 01435 /* Write pixel */ 01436 *(__IO uint32_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (4*(Ypos*768 + Xpos))) = RGB_Code; 01437 } 01438 01439 /** 01440 * @brief Switch back on the display if was switched off by previous call of BSP_DSI_LCD_DisplayOff(). 01441 */ 01442 void BSP_DSI_LCD_DisplayOn(void) 01443 { 01444 /* Send Display on DCS command to display */ 01445 HAL_DSI_ShortWrite(&hdsi_eval, 01446 0, 01447 DSI_DCS_SHORT_PKT_WRITE_P0, 01448 DSI_SET_DISPLAY_ON, 01449 0x0); 01450 } 01451 01452 /** 01453 * @brief Switch Off the display. 01454 */ 01455 void BSP_DSI_LCD_DisplayOff(void) 01456 { 01457 /* Send Display off DCS Command to display */ 01458 HAL_DSI_ShortWrite(&hdsi_eval, 01459 0, 01460 DSI_DCS_SHORT_PKT_WRITE_P0, 01461 DSI_SET_DISPLAY_OFF, 01462 0x0); 01463 } 01464 01465 /** 01466 * @brief Refresh the display. 01467 */ 01468 void BSP_DSI_LCD_Refresh(void) 01469 { 01470 /* Set frame buffer busy */ 01471 FrameBufferAvailable = 0; 01472 01473 /* Set tear on */ 01474 HAL_DSI_ShortWrite(&hdsi_eval, 0, DSI_DCS_SHORT_PKT_WRITE_P1, DSI_SET_TEAR_ON, 0x0); 01475 } 01476 01477 /** 01478 * @brief Check if frame buffer is available. 01479 * @retval LCD_OK if frame buffer is available else LCD_ERROR (frame buffer busy) 01480 */ 01481 uint8_t BSP_DSI_LCD_IsFrameBufferAvailable(void) 01482 { 01483 uint8_t status; 01484 /* Check frame buffer status */ 01485 status = (FrameBufferAvailable == 1) ? LCD_OK : LCD_ERROR; 01486 01487 return(status); 01488 } 01489 01490 /** 01491 * @brief Set the brightness value 01492 * @param BrightnessValue: [0% Min (black), 100% Max] 01493 */ 01494 void BSP_DSI_LCD_SetBrightness(uint8_t BrightnessValue) 01495 { 01496 /* Send Display on DCS command to display */ 01497 HAL_DSI_ShortWrite(&hdsi_eval, 01498 0, 01499 DSI_DCS_SHORT_PKT_WRITE_P1, 01500 0x51, (uint16_t)(BrightnessValue * 255)/100); 01501 } 01502 01503 /******************************************************************************* 01504 LTDC, DMA2D and DSI BSP Routines 01505 *******************************************************************************/ 01506 /** 01507 * @brief Handles DMA2D interrupt request. 01508 * @note Application can surcharge if needed this function implementation. 01509 */ 01510 __weak void BSP_DSI_LCD_DMA2D_IRQHandler(void) 01511 { 01512 HAL_DMA2D_IRQHandler(&hdma2d_eval); 01513 } 01514 01515 /** 01516 * @brief Handles DSI interrupt request. 01517 * @note Application can surcharge if needed this function implementation. 01518 */ 01519 __weak void BSP_DSI_LCD_DSI_IRQHandler(void) 01520 { 01521 HAL_DSI_IRQHandler(&(hdsi_eval)); 01522 } 01523 01524 01525 /** 01526 * @brief Handles LTDC interrupt request. 01527 * @note Application can surcharge if needed this function implementation. 01528 */ 01529 __weak void BSP_DSI_LCD_LTDC_IRQHandler(void) 01530 { 01531 HAL_LTDC_IRQHandler(&(hltdc_eval)); 01532 } 01533 01534 /** 01535 * @brief This function handles LTDC Error interrupt Handler. 01536 * @note Application can surcharge if needed this function implementation. 01537 */ 01538 01539 __weak void BSP_DSI_LCD_LTDC_ER_IRQHandler(void) 01540 { 01541 HAL_LTDC_IRQHandler(&(hltdc_eval)); 01542 } 01543 01544 /** 01545 * @brief De-Initializes the BSP LCD Msp. 01546 * @note Application can surcharge if needed this function implementation. 01547 */ 01548 __weak void BSP_DSI_LCD_MspDeInit(void) 01549 { 01550 /* Disable IRQ of LTDC IP */ 01551 HAL_NVIC_DisableIRQ(LTDC_IRQn); 01552 HAL_NVIC_DisableIRQ(LTDC_ER_IRQn); 01553 01554 /* Disable IRQ of DMA2D IP */ 01555 HAL_NVIC_DisableIRQ(DMA2D_IRQn); 01556 01557 /* Disable IRQ of DSI IP */ 01558 HAL_NVIC_DisableIRQ(DSI_IRQn); 01559 01560 /* Disable HSE used for DSI PLL */ 01561 if(bsp_lcd_hse_to_disable == 1) 01562 { 01563 RCC_OscInitTypeDef RCC_OscInitStruct; 01564 RCC_OscInitStruct.OscillatorType = RCC_OSCILLATORTYPE_HSE; 01565 RCC_OscInitStruct.HSEState = RCC_HSE_OFF; 01566 RCC_OscInitStruct.PLL.PLLState = RCC_PLL_NONE; 01567 if (HAL_RCC_OscConfig(&RCC_OscInitStruct) != HAL_OK) 01568 { 01569 while(1); 01570 } 01571 bsp_lcd_hse_to_disable = 0; 01572 } 01573 01574 /* Force and let in reset state GFXMMU, LTDC, DMA2D and DSI Host + Wrapper IPs */ 01575 __HAL_RCC_GFXMMU_FORCE_RESET(); 01576 __HAL_RCC_LTDC_FORCE_RESET(); 01577 __HAL_RCC_DMA2D_FORCE_RESET(); 01578 __HAL_RCC_DSI_FORCE_RESET(); 01579 01580 /* Disable the GFXMMU, LTDC, DMA2D and DSI Host and Wrapper clocks */ 01581 __HAL_RCC_GFXMMU_CLK_DISABLE(); 01582 __HAL_RCC_LTDC_CLK_DISABLE(); 01583 __HAL_RCC_DMA2D_CLK_DISABLE(); 01584 __HAL_RCC_DSI_CLK_DISABLE(); 01585 } 01586 01587 /** 01588 * @brief Initialize the BSP LCD Msp. 01589 * @note Application can surcharge if needed this function implementation. 01590 */ 01591 __weak void BSP_DSI_LCD_MspInit(void) 01592 { 01593 /* Enable the GFXMMU clock */ 01594 __HAL_RCC_GFXMMU_CLK_ENABLE(); 01595 01596 /* Reset of GFXMMU IP */ 01597 __HAL_RCC_GFXMMU_FORCE_RESET(); 01598 __HAL_RCC_GFXMMU_RELEASE_RESET(); 01599 01600 /* Enable the LTDC clock */ 01601 __HAL_RCC_LTDC_CLK_ENABLE(); 01602 01603 /* Reset of LTDC IP */ 01604 __HAL_RCC_LTDC_FORCE_RESET(); 01605 __HAL_RCC_LTDC_RELEASE_RESET(); 01606 01607 /* Enable the DMA2D clock */ 01608 __HAL_RCC_DMA2D_CLK_ENABLE(); 01609 01610 /* Reset of DMA2D IP */ 01611 __HAL_RCC_DMA2D_FORCE_RESET(); 01612 __HAL_RCC_DMA2D_RELEASE_RESET(); 01613 01614 /* Enable DSI Host and wrapper clocks */ 01615 __HAL_RCC_DSI_CLK_ENABLE(); 01616 01617 /* Reset the DSI Host and wrapper */ 01618 __HAL_RCC_DSI_FORCE_RESET(); 01619 __HAL_RCC_DSI_RELEASE_RESET(); 01620 01621 /* Configure the clock for the LTDC */ 01622 /* We want DSI PHI at 500MHz */ 01623 /* We have only one line => 500Mbps */ 01624 /* With 24bits per pixel, equivalent PCLK is 500/24 = 20.8MHz */ 01625 /* We will set PCLK at 15MHz */ 01626 /* Following values are OK with MSI = 4MHz */ 01627 /* (4*60)/(1*4*4) = 15MHz */ 01628 RCC_PeriphCLKInitTypeDef PeriphClkInit; 01629 PeriphClkInit.PeriphClockSelection = RCC_PERIPHCLK_LTDC; 01630 PeriphClkInit.PLLSAI2.PLLSAI2Source = RCC_PLLSOURCE_MSI; 01631 PeriphClkInit.PLLSAI2.PLLSAI2M = 1; 01632 PeriphClkInit.PLLSAI2.PLLSAI2N = 60; 01633 PeriphClkInit.PLLSAI2.PLLSAI2R = RCC_PLLR_DIV4; 01634 PeriphClkInit.LtdcClockSelection = RCC_LTDCCLKSOURCE_PLLSAI2_DIV4; 01635 PeriphClkInit.PLLSAI2.PLLSAI2ClockOut = RCC_PLLSAI2_LTDCCLK; 01636 if(HAL_RCCEx_PeriphCLKConfig(&PeriphClkInit) != HAL_OK) 01637 { 01638 while(1); 01639 } 01640 01641 /* Enable HSE used for DSI PLL */ 01642 RCC_OscInitTypeDef RCC_OscInitStruct; 01643 HAL_RCC_GetOscConfig(&RCC_OscInitStruct); 01644 if(RCC_OscInitStruct.HSEState == RCC_HSE_OFF) 01645 { 01646 RCC_OscInitStruct.OscillatorType = RCC_OSCILLATORTYPE_HSE; 01647 RCC_OscInitStruct.HSEState = RCC_HSE_ON; 01648 RCC_OscInitStruct.PLL.PLLState = RCC_PLL_NONE; 01649 if (HAL_RCC_OscConfig(&RCC_OscInitStruct) != HAL_OK) 01650 { 01651 while(1); 01652 } 01653 bsp_lcd_hse_to_disable = 1; 01654 } 01655 01656 /* NVIC configuration for LTDC interrupts that are now enabled */ 01657 HAL_NVIC_SetPriority(LTDC_IRQn, 3, 0); 01658 HAL_NVIC_EnableIRQ(LTDC_IRQn); 01659 HAL_NVIC_SetPriority(LTDC_ER_IRQn, 3, 0); 01660 HAL_NVIC_EnableIRQ(LTDC_ER_IRQn); 01661 01662 /* NVIC configuration for DMA2D interrupt that is now enabled */ 01663 HAL_NVIC_SetPriority(DMA2D_IRQn, 3, 0); 01664 HAL_NVIC_EnableIRQ(DMA2D_IRQn); 01665 01666 /* NVIC configuration for DSI interrupt that is now enabled */ 01667 HAL_NVIC_SetPriority(DSI_IRQn, 3, 0); 01668 HAL_NVIC_EnableIRQ(DSI_IRQn); 01669 } 01670 01671 /** 01672 * @} 01673 */ 01674 01675 /** @defgroup STM32L4R9I_EVAL_DSI_LCD_Private_Functions Private Functions 01676 * @{ 01677 */ 01678 01679 /** 01680 * @brief End of Refresh DSI callback. 01681 * @param hdsi: pointer to a DSI_HandleTypeDef structure that contains 01682 * the configuration information for the DSI. 01683 * @retval None 01684 */ 01685 void HAL_DSI_EndOfRefreshCallback(DSI_HandleTypeDef *hdsi) 01686 { 01687 /* Clear pending tearing effect flag */ 01688 __HAL_DSI_CLEAR_FLAG(hdsi, DSI_FLAG_TE); 01689 01690 /* Set frame buffer available */ 01691 FrameBufferAvailable = 1; 01692 } 01693 01694 /** 01695 * @brief LCD Reset 01696 * Hw reset the LCD DSI activating its XRES signal (active low for some time) 01697 * and desactivating it later. 01698 */ 01699 static void LCD_Reset(void) 01700 { 01701 /* Reset the LCD by activation of XRES (active low) */ 01702 BSP_IO_Init(); 01703 01704 /* Configure the GPIO connected to XRES signal */ 01705 BSP_IO_ConfigPin(IO_PIN_9, IO_MODE_OUTPUT); 01706 01707 /* Activate XRES (active low) */ 01708 BSP_IO_WritePin(IO_PIN_9, GPIO_PIN_RESET); 01709 01710 /* Wait at least 1 ms (reset low pulse width) */ 01711 HAL_Delay(2); 01712 01713 /* Desactivate XRES */ 01714 BSP_IO_WritePin(IO_PIN_9, GPIO_PIN_SET); 01715 01716 /* Wait reset complete time (maximum time is 5ms when LCD in sleep mode and 120ms when LCD is not in sleep mode) */ 01717 HAL_Delay(120); 01718 } 01719 01720 /** 01721 * @brief Draws a character on LCD. 01722 * @param Xpos: Line where to display the character shape 01723 * @param Ypos: Start column address 01724 * @param c: Pointer to the character data 01725 */ 01726 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c) 01727 { 01728 uint32_t i = 0, j = 0; 01729 uint16_t height, width; 01730 uint8_t offset; 01731 uint8_t *pchar; 01732 uint32_t line; 01733 01734 height = DrawProp[ActiveLayer].pFont->Height; 01735 width = DrawProp[ActiveLayer].pFont->Width; 01736 01737 offset = 8 *((width + 7)/8) - width ; 01738 01739 for(i = 0; i < height; i++) 01740 { 01741 pchar = ((uint8_t *)c + (width + 7)/8 * i); 01742 01743 switch(((width + 7)/8)) 01744 { 01745 01746 case 1: 01747 line = pchar[0]; 01748 break; 01749 01750 case 2: 01751 line = (pchar[0]<< 8) | pchar[1]; 01752 break; 01753 01754 case 3: 01755 default: 01756 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 01757 break; 01758 } 01759 01760 for (j = 0; j < width; j++) 01761 { 01762 if(line & (1 << (width- j + offset- 1))) 01763 { 01764 BSP_DSI_LCD_DrawPixel((Xpos + j), Ypos, DrawProp[ActiveLayer].TextColor); 01765 } 01766 else 01767 { 01768 BSP_DSI_LCD_DrawPixel((Xpos + j), Ypos, DrawProp[ActiveLayer].BackColor); 01769 } 01770 } 01771 Ypos++; 01772 } 01773 } 01774 01775 /** 01776 * @brief Fills a triangle (between 3 points). 01777 * @param x1: Point 1 X position 01778 * @param y1: Point 1 Y position 01779 * @param x2: Point 2 X position 01780 * @param y2: Point 2 Y position 01781 * @param x3: Point 3 X position 01782 * @param y3: Point 3 Y position 01783 */ 01784 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3) 01785 { 01786 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 01787 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 01788 curpixel = 0; 01789 01790 deltax = ABS(x2 - x1); /* The difference between the x's */ 01791 deltay = ABS(y2 - y1); /* The difference between the y's */ 01792 x = x1; /* Start x off at the first pixel */ 01793 y = y1; /* Start y off at the first pixel */ 01794 01795 if (x2 >= x1) /* The x-values are increasing */ 01796 { 01797 xinc1 = 1; 01798 xinc2 = 1; 01799 } 01800 else /* The x-values are decreasing */ 01801 { 01802 xinc1 = -1; 01803 xinc2 = -1; 01804 } 01805 01806 if (y2 >= y1) /* The y-values are increasing */ 01807 { 01808 yinc1 = 1; 01809 yinc2 = 1; 01810 } 01811 else /* The y-values are decreasing */ 01812 { 01813 yinc1 = -1; 01814 yinc2 = -1; 01815 } 01816 01817 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 01818 { 01819 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 01820 yinc2 = 0; /* Don't change the y for every iteration */ 01821 den = deltax; 01822 num = deltax / 2; 01823 numadd = deltay; 01824 numpixels = deltax; /* There are more x-values than y-values */ 01825 } 01826 else /* There is at least one y-value for every x-value */ 01827 { 01828 xinc2 = 0; /* Don't change the x for every iteration */ 01829 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 01830 den = deltay; 01831 num = deltay / 2; 01832 numadd = deltax; 01833 numpixels = deltay; /* There are more y-values than x-values */ 01834 } 01835 01836 for (curpixel = 0; curpixel <= numpixels; curpixel++) 01837 { 01838 BSP_DSI_LCD_DrawLine(x, y, x3, y3); 01839 01840 num += numadd; /* Increase the numerator by the top of the fraction */ 01841 if (num >= den) /* Check if numerator >= denominator */ 01842 { 01843 num -= den; /* Calculate the new numerator value */ 01844 x += xinc1; /* Change the x as appropriate */ 01845 y += yinc1; /* Change the y as appropriate */ 01846 } 01847 x += xinc2; /* Change the x as appropriate */ 01848 y += yinc2; /* Change the y as appropriate */ 01849 } 01850 } 01851 01852 /** 01853 * @brief Fills a buffer. 01854 * @param LayerIndex: Layer index 01855 * @param pDst: Pointer to destination buffer 01856 * @param xSize: Buffer width 01857 * @param ySize: Buffer height 01858 * @param OffLine: Offset 01859 * @param ColorIndex: Color index 01860 */ 01861 static void LL_FillBuffer(uint32_t LayerIndex, void *pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex) 01862 { 01863 /* Register to memory mode with ARGB8888 as color Mode */ 01864 hdma2d_eval.Init.Mode = DMA2D_R2M; 01865 hdma2d_eval.Init.ColorMode = DMA2D_OUTPUT_ARGB8888; 01866 hdma2d_eval.Init.OutputOffset = OffLine; 01867 hdma2d_eval.Init.AlphaInverted = DMA2D_REGULAR_ALPHA; 01868 hdma2d_eval.Init.RedBlueSwap = DMA2D_RB_REGULAR; 01869 01870 hdma2d_eval.Instance = DMA2D; 01871 01872 /* DMA2D Initialization */ 01873 if(HAL_DMA2D_Init(&hdma2d_eval) == HAL_OK) 01874 { 01875 if(HAL_DMA2D_ConfigLayer(&hdma2d_eval, LayerIndex) == HAL_OK) 01876 { 01877 if (HAL_DMA2D_Start(&hdma2d_eval, ColorIndex, (uint32_t)pDst, xSize, ySize) == HAL_OK) 01878 { 01879 /* Polling For DMA transfer */ 01880 HAL_DMA2D_PollForTransfer(&hdma2d_eval, 10); 01881 } 01882 } 01883 } 01884 } 01885 01886 /** 01887 * @brief Converts a line to an ARGB8888 pixel format. 01888 * @param pSrc: Pointer to source buffer 01889 * @param pDst: Output color 01890 * @param xSize: Buffer width 01891 * @param ColorMode: Input color mode 01892 */ 01893 static void LL_ConvertLineToARGB8888(void *pSrc, void *pDst, uint32_t xSize, uint32_t ColorMode) 01894 { 01895 /* Configure the DMA2D Mode, Color Mode and output offset */ 01896 hdma2d_eval.Init.Mode = DMA2D_M2M_PFC; 01897 hdma2d_eval.Init.ColorMode = DMA2D_OUTPUT_ARGB8888; 01898 hdma2d_eval.Init.OutputOffset = 0; 01899 hdma2d_eval.Init.LineOffsetMode = DMA2D_LOM_PIXELS; 01900 hdma2d_eval.Init.BytesSwap = DMA2D_BYTES_REGULAR; 01901 hdma2d_eval.Init.AlphaInverted = DMA2D_REGULAR_ALPHA; 01902 hdma2d_eval.Init.RedBlueSwap = DMA2D_RB_REGULAR; 01903 01904 /* Foreground Configuration */ 01905 hdma2d_eval.LayerCfg[1].AlphaMode = DMA2D_NO_MODIF_ALPHA; 01906 hdma2d_eval.LayerCfg[1].InputAlpha = 0xFF; 01907 hdma2d_eval.LayerCfg[1].InputColorMode = ColorMode; 01908 hdma2d_eval.LayerCfg[1].InputOffset = 0; 01909 hdma2d_eval.LayerCfg[1].AlphaInverted = DMA2D_REGULAR_ALPHA; 01910 hdma2d_eval.LayerCfg[1].RedBlueSwap = DMA2D_RB_REGULAR; 01911 01912 hdma2d_eval.Instance = DMA2D; 01913 01914 /* DMA2D Initialization */ 01915 if(HAL_DMA2D_Init(&hdma2d_eval) == HAL_OK) 01916 { 01917 if(HAL_DMA2D_ConfigLayer(&hdma2d_eval, 1) == HAL_OK) 01918 { 01919 if (HAL_DMA2D_Start(&hdma2d_eval, (uint32_t)pSrc, (uint32_t)pDst, xSize, 1) == HAL_OK) 01920 { 01921 /* Polling For DMA transfer */ 01922 HAL_DMA2D_PollForTransfer(&hdma2d_eval, 10); 01923 } 01924 } 01925 } 01926 } 01927 01928 /** 01929 * @} 01930 */ 01931 01932 /** 01933 * @} 01934 */ 01935 01936 /** 01937 * @} 01938 */ 01939 01940 /** 01941 * @} 01942 */ 01943 01944 /** 01945 * @} 01946 */ 01947 01948 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Oct 12 2017 10:53:59 for STM32L4R9I_EVAL BSP User Manual by
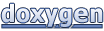