STM32446E_EVAL BSP User Manual
|
Functions | |
uint8_t | BSP_SD_Init (void) |
Initializes the SD card device. | |
uint8_t | BSP_SD_DeInit (void) |
DeInitializes the SD card device. | |
uint8_t | BSP_SD_ITConfig (void) |
Configures Interrupt mode for SD detection pin. | |
uint8_t | BSP_SD_IsDetected (void) |
Detects if SD card is correctly plugged in the memory slot or not. | |
uint8_t | BSP_SD_ReadBlocks (uint32_t *pData, uint64_t ReadAddr, uint32_t BlockSize, uint32_t NumOfBlocks) |
Reads block(s) from a specified address in an SD card, in polling mode. | |
uint8_t | BSP_SD_WriteBlocks (uint32_t *pData, uint64_t WriteAddr, uint32_t BlockSize, uint32_t NumOfBlocks) |
Writes block(s) to a specified address in an SD card, in polling mode. | |
uint8_t | BSP_SD_ReadBlocks_DMA (uint32_t *pData, uint64_t ReadAddr, uint32_t BlockSize, uint32_t NumOfBlocks) |
Reads block(s) from a specified address in an SD card, in DMA mode. | |
uint8_t | BSP_SD_WriteBlocks_DMA (uint32_t *pData, uint64_t WriteAddr, uint32_t BlockSize, uint32_t NumOfBlocks) |
Writes block(s) to a specified address in an SD card, in DMA mode. | |
uint8_t | BSP_SD_Erase (uint64_t StartAddr, uint64_t EndAddr) |
Erases the specified memory area of the given SD card. | |
__weak void | BSP_SD_MspInit (SD_HandleTypeDef *hsd, void *Params) |
Initializes the SD MSP. | |
__weak void | BSP_SD_MspDeInit (SD_HandleTypeDef *hsd, void *Params) |
DeInitializes the SD MSP. | |
void | BSP_SD_IRQHandler (void) |
Handles SD card interrupt request. | |
void | BSP_SD_DMA_Tx_IRQHandler (void) |
Handles SD DMA Tx transfer interrupt request. | |
void | BSP_SD_DMA_Rx_IRQHandler (void) |
Handles SD DMA Rx transfer interrupt request. | |
HAL_SD_TransferStateTypedef | BSP_SD_GetStatus (void) |
Gets the current SD card data status. | |
void | BSP_SD_GetCardInfo (HAL_SD_CardInfoTypedef *CardInfo) |
Get SD information about specific SD card. |
Function Documentation
uint8_t BSP_SD_DeInit | ( | void | ) |
DeInitializes the SD card device.
- Return values:
-
SD status
Definition at line 199 of file stm32446e_eval_sd.c.
References BSP_IO_ConfigPin(), BSP_SD_MspDeInit(), MSD_ERROR, MSD_OK, uSdHandle, and UseExtiModeDetection.
void BSP_SD_DMA_Rx_IRQHandler | ( | void | ) |
Handles SD DMA Rx transfer interrupt request.
Definition at line 556 of file stm32446e_eval_sd.c.
References uSdHandle.
void BSP_SD_DMA_Tx_IRQHandler | ( | void | ) |
Handles SD DMA Tx transfer interrupt request.
Definition at line 548 of file stm32446e_eval_sd.c.
References uSdHandle.
uint8_t BSP_SD_Erase | ( | uint64_t | StartAddr, |
uint64_t | EndAddr | ||
) |
Erases the specified memory area of the given SD card.
- Parameters:
-
StartAddr,: Start byte address EndAddr,: End byte address
- Return values:
-
SD status
Definition at line 381 of file stm32446e_eval_sd.c.
void BSP_SD_GetCardInfo | ( | HAL_SD_CardInfoTypedef * | CardInfo | ) |
Get SD information about specific SD card.
- Parameters:
-
CardInfo,: Pointer to HAL_SD_CardInfoTypedef structure
Definition at line 578 of file stm32446e_eval_sd.c.
References uSdHandle.
HAL_SD_TransferStateTypedef BSP_SD_GetStatus | ( | void | ) |
Gets the current SD card data status.
- Return values:
-
Data transfer state. This value can be one of the following values: - SD_TRANSFER_OK: No data transfer is acting
- SD_TRANSFER_BUSY: Data transfer is acting
- SD_TRANSFER_ERROR: Data transfer error
Definition at line 569 of file stm32446e_eval_sd.c.
References uSdHandle.
uint8_t BSP_SD_Init | ( | void | ) |
Initializes the SD card device.
- Return values:
-
SD status
Definition at line 145 of file stm32446e_eval_sd.c.
References BSP_IO_ConfigPin(), BSP_IO_Init(), BSP_SD_IsDetected(), BSP_SD_MspInit(), MSD_ERROR, MSD_ERROR_SD_NOT_PRESENT, MSD_OK, SD_PRESENT, uSdCardInfo, and uSdHandle.
void BSP_SD_IRQHandler | ( | void | ) |
Handles SD card interrupt request.
Definition at line 540 of file stm32446e_eval_sd.c.
References uSdHandle.
uint8_t BSP_SD_IsDetected | ( | void | ) |
Detects if SD card is correctly plugged in the memory slot or not.
- Return values:
-
Returns if SD is detected or not
Definition at line 240 of file stm32446e_eval_sd.c.
References BSP_IO_ConfigPin(), BSP_IO_ReadPin(), SD_NOT_PRESENT, SD_PRESENT, and UseExtiModeDetection.
Referenced by BSP_SD_Init(), and BSP_SD_ITConfig().
uint8_t BSP_SD_ITConfig | ( | void | ) |
Configures Interrupt mode for SD detection pin.
- Return values:
-
Returns 0
Definition at line 226 of file stm32446e_eval_sd.c.
References BSP_SD_IsDetected(), and UseExtiModeDetection.
__weak void BSP_SD_MspDeInit | ( | SD_HandleTypeDef * | hsd, |
void * | Params | ||
) |
DeInitializes the SD MSP.
- Parameters:
-
hsd,: SD handle Params,: pointer on additional configuration parameters, can be NULL.
Definition at line 507 of file stm32446e_eval_sd.c.
References SD_DMAx_Rx_IRQn, SD_DMAx_Rx_STREAM, SD_DMAx_Tx_IRQn, and SD_DMAx_Tx_STREAM.
Referenced by BSP_SD_DeInit().
__weak void BSP_SD_MspInit | ( | SD_HandleTypeDef * | hsd, |
void * | Params | ||
) |
Initializes the SD MSP.
- Parameters:
-
hsd,: SD handle Params,: pointer on additional configuration parameters, can be NULL.
Definition at line 398 of file stm32446e_eval_sd.c.
References __DMAx_TxRx_CLK_ENABLE, BSP_IO_ConfigPin(), BSP_IO_PIN_RESET, BSP_IO_WritePin(), SD_DMAx_Rx_CHANNEL, SD_DMAx_Rx_IRQn, SD_DMAx_Rx_STREAM, SD_DMAx_Tx_CHANNEL, SD_DMAx_Tx_IRQn, and SD_DMAx_Tx_STREAM.
Referenced by BSP_SD_Init().
uint8_t BSP_SD_ReadBlocks | ( | uint32_t * | pData, |
uint64_t | ReadAddr, | ||
uint32_t | BlockSize, | ||
uint32_t | NumOfBlocks | ||
) |
Reads block(s) from a specified address in an SD card, in polling mode.
- Parameters:
-
pData,: Pointer to the buffer that will contain the data to transmit ReadAddr,: Address from where data is to be read BlockSize,: SD card data block size, that should be 512 NumOfBlocks,: Number of SD blocks to read
- Return values:
-
SD status
Definition at line 275 of file stm32446e_eval_sd.c.
uint8_t BSP_SD_ReadBlocks_DMA | ( | uint32_t * | pData, |
uint64_t | ReadAddr, | ||
uint32_t | BlockSize, | ||
uint32_t | NumOfBlocks | ||
) |
Reads block(s) from a specified address in an SD card, in DMA mode.
- Parameters:
-
pData,: Pointer to the buffer that will contain the data to transmit ReadAddr,: Address from where data is to be read BlockSize,: SD card data block size, that should be 512 NumOfBlocks,: Number of SD blocks to read
- Return values:
-
SD status
Definition at line 315 of file stm32446e_eval_sd.c.
References MSD_ERROR, MSD_OK, SD_DATATIMEOUT, and uSdHandle.
uint8_t BSP_SD_WriteBlocks | ( | uint32_t * | pData, |
uint64_t | WriteAddr, | ||
uint32_t | BlockSize, | ||
uint32_t | NumOfBlocks | ||
) |
Writes block(s) to a specified address in an SD card, in polling mode.
- Parameters:
-
pData,: Pointer to the buffer that will contain the data to transmit WriteAddr,: Address from where data is to be written BlockSize,: SD card data block size, that should be 512 NumOfBlocks,: Number of SD blocks to write
- Return values:
-
SD status
Definition at line 295 of file stm32446e_eval_sd.c.
uint8_t BSP_SD_WriteBlocks_DMA | ( | uint32_t * | pData, |
uint64_t | WriteAddr, | ||
uint32_t | BlockSize, | ||
uint32_t | NumOfBlocks | ||
) |
Writes block(s) to a specified address in an SD card, in DMA mode.
- Parameters:
-
pData,: Pointer to the buffer that will contain the data to transmit WriteAddr,: Address from where data is to be written BlockSize,: SD card data block size, that should be 512 NumOfBlocks,: Number of SD blocks to write
- Return values:
-
SD status
Definition at line 349 of file stm32446e_eval_sd.c.
References MSD_ERROR, MSD_OK, SD_DATATIMEOUT, and uSdHandle.
Generated on Fri Jan 15 2016 10:06:22 for STM32446E_EVAL BSP User Manual by
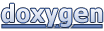