STM32446E_EVAL BSP User Manual
|
stm32446e_eval_io.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32446e_eval_io.c 00004 * @author MCD Application Team 00005 * @version V1.1.1 00006 * @date 13-January-2016 00007 * @brief This file provides a set of functions needed to manage the IO pins 00008 * on STM32446E-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the IO module of the STM32446E-EVAL evaluation 00044 board. 00045 - The MFXSTM32L152 IO expander device component driver must be included with this 00046 driver in order to run the IO functionalities commanded by the IO expander (MFX) 00047 device mounted on the evaluation board. 00048 00049 2. Driver description: 00050 --------------------- 00051 + Initialization steps: 00052 o Initialize the IO module using the BSP_IO_Init() function. This 00053 function includes the MSP layer hardware resources initialization and the 00054 communication layer configuration to start the IO functionalities use. 00055 00056 + IO functionalities use 00057 o The IO pin mode is configured when calling the function BSP_IO_ConfigPin(), you 00058 must specify the desired IO mode by choosing the "IO_ModeTypedef" parameter 00059 predefined value. 00060 o If an IO pin is used in interrupt mode, the function BSP_IO_ITGetStatus() is 00061 needed to get the interrupt status. To clear the IT pending bits, you should 00062 call the function BSP_IO_ITClear() with specifying the IO pending bit to clear. 00063 o The IT is handled using the corresponding external interrupt IRQ handler, 00064 the user IT callback treatment is implemented on the same external interrupt 00065 callback. 00066 o The IRQ_OUT pin (common for all functionalities: TS, JOY, SD, etc) can be 00067 configured using the function BSP_IO_ConfigIrqOutPin() 00068 o To get/set an IO pin combination state you can use the functions 00069 BSP_IO_ReadPin()/BSP_IO_WritePin() or the function BSP_IO_TogglePin() to toggle the pin 00070 state. 00071 00072 ------------------------------------------------------------------------------*/ 00073 00074 /* Includes ------------------------------------------------------------------*/ 00075 #include "stm32446e_eval_io.h" 00076 00077 /** @addtogroup BSP 00078 * @{ 00079 */ 00080 00081 /** @addtogroup STM32446E_EVAL 00082 * @{ 00083 */ 00084 00085 /** @defgroup STM32446E_EVAL_IO STM32446E EVAL IO 00086 * @{ 00087 */ 00088 00089 /** @defgroup STM32446E_EVAL_IO_Private_Types_Definitions STM32446E EVAL IO Private Types Definitions 00090 * @{ 00091 */ 00092 /** 00093 * @} 00094 */ 00095 00096 /** @defgroup STM32446E_EVAL_IO_Private_Defines STM32446E EVAL IO Private Defines 00097 * @{ 00098 */ 00099 /** 00100 * @} 00101 */ 00102 00103 /** @defgroup STM32446E_EVAL_IO_Private_Macros STM32446E EVAL IO Private Macros 00104 * @{ 00105 */ 00106 /** 00107 * @} 00108 */ 00109 00110 /** @defgroup STM32446E_EVAL_IO_Private_Variables STM32446E EVAL IO Private Variables 00111 * @{ 00112 */ 00113 static IO_DrvTypeDef *IoDrv = NULL; 00114 static uint8_t mfxstm32l152Identifier; 00115 00116 /** 00117 * @} 00118 */ 00119 00120 /** @defgroup STM32446E_EVAL_IO_Private_Function_Prototypes STM32446E EVAL IO Private Function Prototypes 00121 * @{ 00122 */ 00123 /** 00124 * @} 00125 */ 00126 00127 /** @defgroup STM32446E_EVAL_IO_Private_Functions STM32446E EVAL IO Private Functions 00128 * @{ 00129 */ 00130 00131 /** 00132 * @brief Initializes and configures the IO functionalities and configures all 00133 * necessary hardware resources (MFX, ...). 00134 * @note BSP_IO_Init() is using HAL_Delay() function to ensure that MFXSTM32L152 00135 * IO Expander is correctly reset. HAL_Delay() function provides accurate 00136 * delay (in milliseconds) based on variable incremented in SysTick ISR. 00137 * This implies that if BSP_IO_Init() is called from a peripheral ISR process, 00138 * then the SysTick interrupt must have higher priority (numerically lower) 00139 * than the peripheral interrupt. Otherwise the caller ISR process will be blocked. 00140 * @retval IO_OK if all initializations are OK. Other value if error. 00141 */ 00142 uint8_t BSP_IO_Init(void) 00143 { 00144 uint8_t ret = IO_OK; 00145 00146 if ( IoDrv == NULL) 00147 { 00148 /* Read ID and verify the IO expander is ready */ 00149 mfxstm32l152Identifier=0; 00150 mfxstm32l152Identifier = mfxstm32l152_io_drv.ReadID(IO_I2C_ADDRESS); 00151 if((mfxstm32l152Identifier == MFXSTM32L152_ID_1) || (mfxstm32l152Identifier == MFXSTM32L152_ID_2)) 00152 { 00153 /* Initialize the IO driver structure */ 00154 IoDrv = &mfxstm32l152_io_drv; 00155 } 00156 else 00157 { 00158 ret = IO_ERROR; 00159 } 00160 00161 if(ret == IO_OK) 00162 { 00163 IoDrv->Init(IO_I2C_ADDRESS); 00164 IoDrv->Start(IO_I2C_ADDRESS, IO_PIN_ALL); 00165 } 00166 } 00167 00168 return ret; 00169 } 00170 00171 /** 00172 * @brief DeInit allows Mfx Initialization to be executed again 00173 * @note BSP_IO_Init() has no effect if the IoDrv is already initialized 00174 * BSP_IO_DeInit() allows to erase the pointer such to allow init to be effective 00175 * @retval IO_OK 00176 */ 00177 uint8_t BSP_IO_DeInit(void) 00178 { 00179 IoDrv = NULL; 00180 return IO_OK; 00181 } 00182 00183 /** 00184 * @brief Gets the selected pins IT status. 00185 * @param IoPin: Selected pins to check the status. 00186 * This parameter can be any combination of the IO pins. 00187 * @retval IO_OK if read status OK. Other value if error. 00188 */ 00189 uint32_t BSP_IO_ITGetStatus(uint32_t IoPin) 00190 { 00191 /* Return the IO Pin IT status */ 00192 return (IoDrv->ITStatus(IO_I2C_ADDRESS, IoPin)); 00193 } 00194 00195 /** 00196 * @brief Clears all the IO IT pending bits. 00197 */ 00198 void BSP_IO_ITClear(void) 00199 { 00200 /* Clear all IO IT pending bits */ 00201 IoDrv->ClearIT(IO_I2C_ADDRESS, MFXSTM32L152_GPIO_PINS_ALL); 00202 } 00203 00204 /** 00205 * @brief Configures the IO pin(s) according to IO mode structure value. 00206 * @param IoPin: IO pin(s) to be configured. 00207 * This parameter can be one of the following values: 00208 * @arg MFXSTM32L152_GPIO_PIN_x: where x can be from 0 to 23. 00209 * @param IoMode: IO pin mode to configure 00210 * This parameter can be one of the following values: 00211 * @arg IO_MODE_INPUT 00212 * @arg IO_MODE_OUTPUT 00213 * @arg IO_MODE_IT_RISING_EDGE 00214 * @arg IO_MODE_IT_FALLING_EDGE 00215 * @arg IO_MODE_IT_LOW_LEVEL 00216 * @arg IO_MODE_IT_HIGH_LEVEL 00217 * @arg IO_MODE_ANALOG 00218 * @arg IO_MODE_OFF 00219 * @arg IO_MODE_INPUT_PU, 00220 * @arg IO_MODE_INPUT_PD, 00221 * @arg IO_MODE_OUTPUT_OD, 00222 * @arg IO_MODE_OUTPUT_OD_PU, 00223 * @arg IO_MODE_OUTPUT_OD_PD, 00224 * @arg IO_MODE_OUTPUT_PP, 00225 * @arg IO_MODE_OUTPUT_PP_PU, 00226 * @arg IO_MODE_OUTPUT_PP_PD, 00227 * @arg IO_MODE_IT_RISING_EDGE_PU 00228 * @arg IO_MODE_IT_FALLING_EDGE_PU 00229 * @arg IO_MODE_IT_LOW_LEVEL_PU 00230 * @arg IO_MODE_IT_HIGH_LEVEL_PU 00231 * @arg IO_MODE_IT_RISING_EDGE_PD 00232 * @arg IO_MODE_IT_FALLING_EDGE_PD 00233 * @arg IO_MODE_IT_LOW_LEVEL_PD 00234 * @arg IO_MODE_IT_HIGH_LEVEL_PD 00235 * @retval IO_OK if all initializations are OK. Other value if error. 00236 */ 00237 uint8_t BSP_IO_ConfigPin(uint32_t IoPin, IO_ModeTypedef IoMode) 00238 { 00239 /* Configure the selected IO pin(s) mode */ 00240 IoDrv->Config(IO_I2C_ADDRESS, IoPin, IoMode); 00241 00242 return IO_OK; 00243 } 00244 00245 /** 00246 * @brief Sets the IRQ_OUT pin polarity and type 00247 * @param IoIrqOutPinPolarity: High/Low 00248 * @param IoIrqOutPinType: OpenDrain/PushPull 00249 * @retval OK 00250 */ 00251 uint8_t BSP_IO_ConfigIrqOutPin(uint8_t IoIrqOutPinPolarity, uint8_t IoIrqOutPinType) 00252 { 00253 if((mfxstm32l152Identifier == MFXSTM32L152_ID_1) || (mfxstm32l152Identifier == MFXSTM32L152_ID_2)) 00254 { 00255 /* Initialize the IO driver structure */ 00256 mfxstm32l152_SetIrqOutPinPolarity(IO_I2C_ADDRESS, IoIrqOutPinPolarity); 00257 mfxstm32l152_SetIrqOutPinType(IO_I2C_ADDRESS, IoIrqOutPinType); 00258 } 00259 00260 return IO_OK; 00261 } 00262 00263 /** 00264 * @brief Sets the selected pins state. 00265 * @param IoPin: Selected pins to write. 00266 * This parameter can be any combination of the IO pins. 00267 * @param PinState: New pins state to write 00268 */ 00269 void BSP_IO_WritePin(uint32_t IoPin, BSP_IO_PinStateTypeDef PinState) 00270 { 00271 /* Set the Pin state */ 00272 IoDrv->WritePin(IO_I2C_ADDRESS, IoPin, PinState); 00273 } 00274 00275 /** 00276 * @brief Gets the selected pins current state. 00277 * @param IoPin: Selected pins to read. 00278 * This parameter can be any combination of the IO pins. 00279 * @retval The current pins state 00280 */ 00281 uint32_t BSP_IO_ReadPin(uint32_t IoPin) 00282 { 00283 return(IoDrv->ReadPin(IO_I2C_ADDRESS, IoPin)); 00284 } 00285 00286 /** 00287 * @brief Toggles the selected pins state. 00288 * @param IoPin: Selected pins to toggle. 00289 * This parameter can be any combination of the IO pins. 00290 * @note This function is only used to toggle one pin in the same time 00291 */ 00292 void BSP_IO_TogglePin(uint32_t IoPin) 00293 { 00294 /* Toggle the current pin state */ 00295 if(IoDrv->ReadPin(IO_I2C_ADDRESS, IoPin) != 0) /* Set */ 00296 { 00297 IoDrv->WritePin(IO_I2C_ADDRESS, IoPin, 0); /* Reset */ 00298 } 00299 else 00300 { 00301 IoDrv->WritePin(IO_I2C_ADDRESS, IoPin, 1); /* Set */ 00302 } 00303 } 00304 00305 /** 00306 * @} 00307 */ 00308 00309 /** 00310 * @} 00311 */ 00312 00313 /** 00314 * @} 00315 */ 00316 00317 /** 00318 * @} 00319 */ 00320 00321 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jan 15 2016 10:06:21 for STM32446E_EVAL BSP User Manual by
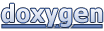