STM32446E_EVAL BSP User Manual
|
Functions | |
uint8_t | BSP_LCD_Init (void) |
Initializes the LCD. | |
uint8_t | BSP_LCD_DeInit (void) |
DeInitializes the LCD. | |
uint32_t | BSP_LCD_GetXSize (void) |
Gets the LCD X size. | |
uint32_t | BSP_LCD_GetYSize (void) |
Gets the LCD Y size. | |
uint16_t | BSP_LCD_GetTextColor (void) |
Gets the LCD text color. | |
uint16_t | BSP_LCD_GetBackColor (void) |
Gets the LCD background color. | |
void | BSP_LCD_SetTextColor (uint16_t Color) |
Sets the LCD text color. | |
void | BSP_LCD_SetBackColor (uint16_t Color) |
Sets the LCD background color. | |
void | BSP_LCD_SetFont (sFONT *fonts) |
Sets the LCD text font. | |
sFONT * | BSP_LCD_GetFont (void) |
Gets the LCD text font. | |
void | BSP_LCD_Clear (uint16_t Color) |
Clears the hole LCD. | |
void | BSP_LCD_ClearStringLine (uint16_t Line) |
Clears the selected line. | |
void | BSP_LCD_DisplayChar (uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) |
Displays one character. | |
void | BSP_LCD_DisplayStringAt (uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Line_ModeTypdef Mode) |
Displays characters on the LCD. | |
void | BSP_LCD_DisplayStringAtLine (uint16_t Line, uint8_t *ptr) |
Displays a character on the LCD. | |
uint16_t | BSP_LCD_ReadPixel (uint16_t Xpos, uint16_t Ypos) |
Reads an LCD pixel. | |
void | BSP_LCD_DrawPixel (uint16_t Xpos, uint16_t Ypos, uint16_t RGB_Code) |
Draws a pixel on LCD. | |
void | BSP_LCD_DrawHLine (uint16_t Xpos, uint16_t Ypos, uint16_t Length) |
Draws an horizontal line. | |
void | BSP_LCD_DrawVLine (uint16_t Xpos, uint16_t Ypos, uint16_t Length) |
Draws a vertical line. | |
void | BSP_LCD_DrawLine (uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) |
Draws an uni-line (between two points). | |
void | BSP_LCD_DrawRect (uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) |
Draws a rectangle. | |
void | BSP_LCD_DrawCircle (uint16_t Xpos, uint16_t Ypos, uint16_t Radius) |
Draws a circle. | |
void | BSP_LCD_DrawPolygon (pPoint Points, uint16_t PointCount) |
Draws an poly-line (between many points). | |
void | BSP_LCD_DrawEllipse (int Xpos, int Ypos, int XRadius, int YRadius) |
Draws an ellipse on LCD. | |
void | BSP_LCD_DrawBitmap (uint16_t Xpos, uint16_t Ypos, uint8_t *pbmp) |
Draws a bitmap picture (16 bpp). | |
void | BSP_LCD_DrawRGBImage (uint16_t Xpos, uint16_t Ypos, uint16_t Xsize, uint16_t Ysize, uint8_t *pdata) |
Draws RGB Image (16 bpp). | |
void | BSP_LCD_FillRect (uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) |
Draws a full rectangle. | |
void | BSP_LCD_FillCircle (uint16_t Xpos, uint16_t Ypos, uint16_t Radius) |
Draws a full circle. | |
void | BSP_LCD_FillPolygon (pPoint Points, uint16_t PointCount) |
Draws a full poly-line (between many points). | |
void | BSP_LCD_FillEllipse (int Xpos, int Ypos, int XRadius, int YRadius) |
Draws a full ellipse. | |
void | BSP_LCD_DisplayOn (void) |
Enables the display. | |
void | BSP_LCD_DisplayOff (void) |
Disables the display. | |
static void | DrawChar (uint16_t Xpos, uint16_t Ypos, const uint8_t *c) |
Draws a character on LCD. | |
static void | SetDisplayWindow (uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) |
Sets display window. | |
static void | FillTriangle (uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3) |
Fills a triangle (between 3 points). |
Function Documentation
void BSP_LCD_Clear | ( | uint16_t | Color | ) |
Clears the hole LCD.
- Parameters:
-
Color,: Color of the background
Definition at line 247 of file stm32446e_eval_lcd.c.
References BSP_LCD_DrawHLine(), BSP_LCD_GetXSize(), BSP_LCD_GetYSize(), BSP_LCD_SetTextColor(), and LCD_DrawPropTypeDef::TextColor.
void BSP_LCD_ClearStringLine | ( | uint16_t | Line | ) |
Clears the selected line.
- Parameters:
-
Line,: Line to be cleared This parameter can be one of the following values: - 0..9: if the Current fonts is Font16x24
- 0..19: if the Current fonts is Font12x12 or Font8x12
- 0..29: if the Current fonts is Font8x8
Definition at line 272 of file stm32446e_eval_lcd.c.
References LCD_DrawPropTypeDef::BackColor, BSP_LCD_FillRect(), BSP_LCD_GetXSize(), BSP_LCD_SetTextColor(), LCD_DrawPropTypeDef::pFont, and LCD_DrawPropTypeDef::TextColor.
uint8_t BSP_LCD_DeInit | ( | void | ) |
DeInitializes the LCD.
- Return values:
-
LCD state
Definition at line 165 of file stm32446e_eval_lcd.c.
References LCD_OK.
void BSP_LCD_DisplayChar | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint8_t | Ascii | ||
) |
Displays one character.
- Parameters:
-
Xpos,: Start column address Ypos,: Line where to display the character shape. Ascii,: Character ascii code This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E
Definition at line 292 of file stm32446e_eval_lcd.c.
References DrawChar(), and LCD_DrawPropTypeDef::pFont.
Referenced by BSP_LCD_DisplayStringAt().
void BSP_LCD_DisplayOff | ( | void | ) |
void BSP_LCD_DisplayOn | ( | void | ) |
void BSP_LCD_DisplayStringAt | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint8_t * | Text, | ||
Line_ModeTypdef | Mode | ||
) |
Displays characters on the LCD.
- Parameters:
-
Xpos,: X position (in pixel) Ypos,: Y position (in pixel) Text,: Pointer to string to display on LCD Mode,: Display mode This parameter can be one of the following values: - CENTER_MODE
- RIGHT_MODE
- LEFT_MODE
Definition at line 309 of file stm32446e_eval_lcd.c.
References BSP_LCD_DisplayChar(), BSP_LCD_GetXSize(), CENTER_MODE, LEFT_MODE, LCD_DrawPropTypeDef::pFont, and RIGHT_MODE.
Referenced by BSP_LCD_DisplayStringAtLine().
void BSP_LCD_DisplayStringAtLine | ( | uint16_t | Line, |
uint8_t * | ptr | ||
) |
Displays a character on the LCD.
- Parameters:
-
Line,: Line where to display the character shape This parameter can be one of the following values: - 0..9: if the Current fonts is Font16x24
- 0..19: if the Current fonts is Font12x12 or Font8x12
- 0..29: if the Current fonts is Font8x8
ptr,: Pointer to string to display on LCD
Definition at line 373 of file stm32446e_eval_lcd.c.
References BSP_LCD_DisplayStringAt(), and LEFT_MODE.
void BSP_LCD_DrawBitmap | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint8_t * | pbmp | ||
) |
Draws a bitmap picture (16 bpp).
- Parameters:
-
Xpos,: Bmp X position in the LCD Ypos,: Bmp Y position in the LCD pbmp,: Pointer to Bmp picture address.
Definition at line 662 of file stm32446e_eval_lcd.c.
References BSP_LCD_GetXSize(), BSP_LCD_GetYSize(), LcdDrv, and SetDisplayWindow().
void BSP_LCD_DrawCircle | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Radius | ||
) |
Draws a circle.
- Parameters:
-
Xpos,: X position Ypos,: Y position Radius,: Circle radius
Definition at line 554 of file stm32446e_eval_lcd.c.
References BSP_LCD_DrawPixel(), BSP_LCD_SetFont(), LCD_DEFAULT_FONT, and LCD_DrawPropTypeDef::TextColor.
Referenced by BSP_LCD_FillCircle().
void BSP_LCD_DrawEllipse | ( | int | Xpos, |
int | Ypos, | ||
int | XRadius, | ||
int | YRadius | ||
) |
Draws an ellipse on LCD.
- Parameters:
-
Xpos,: X position Ypos,: Y position XRadius,: Ellipse X radius YRadius,: Ellipse Y radius
Definition at line 630 of file stm32446e_eval_lcd.c.
References BSP_LCD_DrawPixel(), and LCD_DrawPropTypeDef::TextColor.
void BSP_LCD_DrawHLine | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Length | ||
) |
Draws an horizontal line.
- Parameters:
-
Xpos,: X position Ypos,: Y position Length,: Line length
Definition at line 416 of file stm32446e_eval_lcd.c.
References BSP_LCD_DrawPixel(), LcdDrv, and LCD_DrawPropTypeDef::TextColor.
Referenced by BSP_LCD_Clear(), BSP_LCD_DrawRect(), BSP_LCD_FillCircle(), BSP_LCD_FillEllipse(), and BSP_LCD_FillRect().
void BSP_LCD_DrawLine | ( | uint16_t | x1, |
uint16_t | y1, | ||
uint16_t | x2, | ||
uint16_t | y2 | ||
) |
Draws an uni-line (between two points).
- Parameters:
-
x1,: Point 1 X position y1,: Point 1 Y position x2,: Point 2 X position y2,: Point 2 Y position
Definition at line 463 of file stm32446e_eval_lcd.c.
References ABS, BSP_LCD_DrawPixel(), and LCD_DrawPropTypeDef::TextColor.
Referenced by BSP_LCD_DrawPolygon(), and FillTriangle().
void BSP_LCD_DrawPixel | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | RGB_Code | ||
) |
Draws a pixel on LCD.
- Parameters:
-
Xpos,: X position Ypos,: Y position RGB_Code,: Pixel color in RGB mode (5-6-5)
Definition at line 402 of file stm32446e_eval_lcd.c.
References LcdDrv.
Referenced by BSP_LCD_DrawCircle(), BSP_LCD_DrawEllipse(), BSP_LCD_DrawHLine(), BSP_LCD_DrawLine(), BSP_LCD_DrawVLine(), and DrawChar().
void BSP_LCD_DrawPolygon | ( | pPoint | Points, |
uint16_t | PointCount | ||
) |
Draws an poly-line (between many points).
- Parameters:
-
Points,: Pointer to the points array PointCount,: Number of points
Definition at line 603 of file stm32446e_eval_lcd.c.
References BSP_LCD_DrawLine(), Point::X, and Point::Y.
void BSP_LCD_DrawRect | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Width, | ||
uint16_t | Height | ||
) |
Draws a rectangle.
- Parameters:
-
Xpos,: X position Ypos,: Y position Width,: Rectangle width Height,: Rectangle height
Definition at line 537 of file stm32446e_eval_lcd.c.
References BSP_LCD_DrawHLine(), and BSP_LCD_DrawVLine().
void BSP_LCD_DrawRGBImage | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Xsize, | ||
uint16_t | Ysize, | ||
uint8_t * | pdata | ||
) |
Draws RGB Image (16 bpp).
- Parameters:
-
Xpos,: X position in the LCD Ypos,: Y position in the LCD Xsize,: X size in the LCD Ysize,: Y size in the LCD pdata,: Pointer to the RGB Image address.
Definition at line 693 of file stm32446e_eval_lcd.c.
References BSP_LCD_GetXSize(), BSP_LCD_GetYSize(), LcdDrv, and SetDisplayWindow().
void BSP_LCD_DrawVLine | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Length | ||
) |
Draws a vertical line.
- Parameters:
-
Xpos,: X position Ypos,: Y position Length,: Line length
Definition at line 439 of file stm32446e_eval_lcd.c.
References BSP_LCD_DrawPixel(), LcdDrv, and LCD_DrawPropTypeDef::TextColor.
Referenced by BSP_LCD_DrawRect().
void BSP_LCD_FillCircle | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Radius | ||
) |
Draws a full circle.
- Parameters:
-
Xpos,: X position Ypos,: Y position Radius,: Circle radius
Definition at line 728 of file stm32446e_eval_lcd.c.
References BSP_LCD_DrawCircle(), BSP_LCD_DrawHLine(), BSP_LCD_SetTextColor(), and LCD_DrawPropTypeDef::TextColor.
void BSP_LCD_FillEllipse | ( | int | Xpos, |
int | Ypos, | ||
int | XRadius, | ||
int | YRadius | ||
) |
Draws a full ellipse.
- Parameters:
-
Xpos,: X position Ypos,: Y position XRadius,: Ellipse X radius YRadius,: Ellipse Y radius
Definition at line 842 of file stm32446e_eval_lcd.c.
References BSP_LCD_DrawHLine().
void BSP_LCD_FillPolygon | ( | pPoint | Points, |
uint16_t | PointCount | ||
) |
Draws a full poly-line (between many points).
- Parameters:
-
Points,: Pointer to the points array PointCount,: Number of points
Definition at line 775 of file stm32446e_eval_lcd.c.
References FillTriangle(), POLY_X, POLY_Y, Point::X, and Point::Y.
void BSP_LCD_FillRect | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Width, | ||
uint16_t | Height | ||
) |
Draws a full rectangle.
- Parameters:
-
Xpos,: X position Ypos,: Y position Width,: Rectangle width Height,: Rectangle height
Definition at line 712 of file stm32446e_eval_lcd.c.
References BSP_LCD_DrawHLine(), BSP_LCD_SetTextColor(), and LCD_DrawPropTypeDef::TextColor.
Referenced by BSP_LCD_ClearStringLine().
uint16_t BSP_LCD_GetBackColor | ( | void | ) |
Gets the LCD background color.
- Return values:
-
Used background color
Definition at line 202 of file stm32446e_eval_lcd.c.
References LCD_DrawPropTypeDef::BackColor.
sFONT* BSP_LCD_GetFont | ( | void | ) |
Gets the LCD text font.
- Return values:
-
Used font
Definition at line 238 of file stm32446e_eval_lcd.c.
References LCD_DrawPropTypeDef::pFont.
uint16_t BSP_LCD_GetTextColor | ( | void | ) |
Gets the LCD text color.
- Return values:
-
Used text color.
Definition at line 193 of file stm32446e_eval_lcd.c.
References LCD_DrawPropTypeDef::TextColor.
uint32_t BSP_LCD_GetXSize | ( | void | ) |
Gets the LCD X size.
- Return values:
-
Used LCD X size
Definition at line 175 of file stm32446e_eval_lcd.c.
References LcdDrv.
Referenced by BSP_LCD_Clear(), BSP_LCD_ClearStringLine(), BSP_LCD_DisplayStringAt(), BSP_LCD_DrawBitmap(), and BSP_LCD_DrawRGBImage().
uint32_t BSP_LCD_GetYSize | ( | void | ) |
Gets the LCD Y size.
- Return values:
-
Used LCD Y size
Definition at line 184 of file stm32446e_eval_lcd.c.
References LcdDrv.
Referenced by BSP_LCD_Clear(), BSP_LCD_DrawBitmap(), and BSP_LCD_DrawRGBImage().
uint8_t BSP_LCD_Init | ( | void | ) |
Initializes the LCD.
- Return values:
-
LCD state
Definition at line 136 of file stm32446e_eval_lcd.c.
References LCD_DrawPropTypeDef::BackColor, BSP_LCD_SetFont(), LCD_DEFAULT_FONT, LCD_ERROR, LCD_OK, LcdDrv, LCD_DrawPropTypeDef::pFont, and LCD_DrawPropTypeDef::TextColor.
uint16_t BSP_LCD_ReadPixel | ( | uint16_t | Xpos, |
uint16_t | Ypos | ||
) |
Reads an LCD pixel.
- Parameters:
-
Xpos,: X position Ypos,: Y position
- Return values:
-
RGB pixel color
Definition at line 384 of file stm32446e_eval_lcd.c.
References LcdDrv.
void BSP_LCD_SetBackColor | ( | uint16_t | Color | ) |
Sets the LCD background color.
- Parameters:
-
Color,: Background color code RGB(5-6-5)
Definition at line 220 of file stm32446e_eval_lcd.c.
References LCD_DrawPropTypeDef::BackColor.
void BSP_LCD_SetFont | ( | sFONT * | fonts | ) |
Sets the LCD text font.
- Parameters:
-
fonts,: Font to be used
Definition at line 229 of file stm32446e_eval_lcd.c.
References LCD_DrawPropTypeDef::pFont.
Referenced by BSP_LCD_DrawCircle(), and BSP_LCD_Init().
void BSP_LCD_SetTextColor | ( | uint16_t | Color | ) |
Sets the LCD text color.
- Parameters:
-
Color,: Text color code RGB(5-6-5)
Definition at line 211 of file stm32446e_eval_lcd.c.
References LCD_DrawPropTypeDef::TextColor.
Referenced by BSP_LCD_Clear(), BSP_LCD_ClearStringLine(), BSP_LCD_FillCircle(), and BSP_LCD_FillRect().
static void DrawChar | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
const uint8_t * | c | ||
) | [static] |
Draws a character on LCD.
- Parameters:
-
Xpos,: Line where to display the character shape Ypos,: Start column address c,: Pointer to the character data
Definition at line 894 of file stm32446e_eval_lcd.c.
References LCD_DrawPropTypeDef::BackColor, BSP_LCD_DrawPixel(), LCD_DrawPropTypeDef::pFont, and LCD_DrawPropTypeDef::TextColor.
Referenced by BSP_LCD_DisplayChar().
static void FillTriangle | ( | uint16_t | x1, |
uint16_t | x2, | ||
uint16_t | x3, | ||
uint16_t | y1, | ||
uint16_t | y2, | ||
uint16_t | y3 | ||
) | [static] |
Fills a triangle (between 3 points).
- Parameters:
-
x1,: Point 1 X position y1,: Point 1 Y position x2,: Point 2 X position y2,: Point 2 Y position x3,: Point 3 X position y3,: Point 3 Y position
Definition at line 966 of file stm32446e_eval_lcd.c.
References ABS, and BSP_LCD_DrawLine().
Referenced by BSP_LCD_FillPolygon().
static void SetDisplayWindow | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Width, | ||
uint16_t | Height | ||
) | [static] |
Sets display window.
- Parameters:
-
Xpos,: LCD X position Ypos,: LCD Y position Width,: LCD window width Height,: LCD window height
Definition at line 949 of file stm32446e_eval_lcd.c.
References LcdDrv.
Referenced by BSP_LCD_DrawBitmap(), and BSP_LCD_DrawRGBImage().
Generated on Fri Jan 15 2016 10:06:22 for STM32446E_EVAL BSP User Manual by
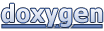