STM32446E_EVAL BSP User Manual
|
This file provides a set of firmware functions to manage LEDs, push-buttons and COM ports available on STM32446E-EVAL evaluation board(MB1045) RevB from STMicroelectronics. More...
#include "stm32446e_eval.h"
Go to the source code of this file.
Data Structures | |
struct | LCD_CONTROLLER_TypeDef |
Defines | |
#define | __STM32446E_EVAL_BSP_VERSION_MAIN (0x01) |
STM32446E EVAL BSP Driver version number V1.1.1. | |
#define | __STM32446E_EVAL_BSP_VERSION_SUB1 (0x01) |
#define | __STM32446E_EVAL_BSP_VERSION_SUB2 (0x01) |
#define | __STM32446E_EVAL_BSP_VERSION_RC (0x00) |
#define | __STM32446E_EVAL_BSP_VERSION |
#define | FMC_BANK1_BASE ((uint32_t)(0x60000000 | 0x00000000)) |
#define | FMC_BANK3_BASE ((uint32_t)(0x60000000 | 0x08000000)) |
#define | FMC_BANK1 ((LCD_CONTROLLER_TypeDef *) FMC_BANK1_BASE) |
Functions | |
static void | I2Cx_MspInit (void) |
Initializes I2C MSP. | |
static void | I2Cx_Init (void) |
Initializes I2C HAL. | |
static HAL_StatusTypeDef | I2Cx_ReadMultiple (uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length) |
Reads multiple data. | |
static HAL_StatusTypeDef | I2Cx_WriteMultiple (uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length) |
Writes a value in a register of the device through BUS in using DMA mode. | |
static HAL_StatusTypeDef | I2Cx_IsDeviceReady (uint16_t DevAddress, uint32_t Trials) |
Checks if target device is ready for communication. | |
static void | I2Cx_Error (uint8_t Addr) |
Manages error callback by re-initializing I2C. | |
static void | FMC_BANK1_WriteData (uint16_t Data) |
Writes register value. | |
static void | FMC_BANK1_WriteReg (uint8_t Reg) |
Writes register address. | |
static uint16_t | FMC_BANK1_ReadData (void) |
Reads register value. | |
static void | FMC_BANK1_Init (void) |
Initializes LCD IO. | |
static void | FMC_BANK1_MspInit (void) |
Initializes FMC_BANK1 MSP. | |
void | LCD_IO_Init (void) |
Initializes LCD low level. | |
void | LCD_IO_WriteData (uint16_t RegValue) |
Writes data on LCD data register. | |
void | LCD_IO_WriteReg (uint8_t Reg) |
Writes register on LCD register. | |
uint16_t | LCD_IO_ReadData (void) |
Reads data from LCD data register. | |
void | AUDIO_IO_Init (void) |
Initializes Audio low level. | |
void | AUDIO_IO_DeInit (void) |
DeInitializes Audio low level. | |
void | AUDIO_IO_Write (uint8_t Addr, uint16_t Reg, uint16_t Value) |
Writes a single data. | |
uint16_t | AUDIO_IO_Read (uint8_t Addr, uint16_t Reg) |
Reads a single data. | |
void | AUDIO_IO_Delay (uint32_t Delay) |
AUDIO Codec delay. | |
void | CAMERA_IO_Init (void) |
Initializes Camera low level. | |
void | CAMERA_Delay (uint32_t Delay) |
Camera delay. | |
void | CAMERA_IO_Write (uint8_t Addr, uint16_t Reg, uint16_t Value) |
Camera writes single data. | |
uint16_t | CAMERA_IO_Read (uint8_t Addr, uint16_t Reg) |
Camera reads single data. | |
void | EEPROM_IO_Init (void) |
Initializes peripherals used by the I2C EEPROM driver. | |
HAL_StatusTypeDef | EEPROM_IO_WriteData (uint16_t DevAddress, uint16_t MemAddress, uint8_t *pBuffer, uint32_t BufferSize) |
Write data to I2C EEPROM driver in using DMA channel. | |
HAL_StatusTypeDef | EEPROM_IO_ReadData (uint16_t DevAddress, uint16_t MemAddress, uint8_t *pBuffer, uint32_t BufferSize) |
Read data from I2C EEPROM driver in using DMA channel. | |
HAL_StatusTypeDef | EEPROM_IO_IsDeviceReady (uint16_t DevAddress, uint32_t Trials) |
Checks if target device is ready for communication. | |
uint32_t | BSP_GetVersion (void) |
This method returns the STM32446E EVAL BSP Driver revision. | |
void | BSP_LED_Init (Led_TypeDef Led) |
Configures LEDs. | |
void | BSP_LED_DeInit (Led_TypeDef Led) |
DeInit LEDs. | |
void | BSP_LED_On (Led_TypeDef Led) |
Turns selected LED On. | |
void | BSP_LED_Off (Led_TypeDef Led) |
Turns selected LED Off. | |
void | BSP_LED_Toggle (Led_TypeDef Led) |
Toggles the selected LED. | |
void | BSP_PB_Init (Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) |
Configures button GPIO and EXTI Line. | |
void | BSP_PB_DeInit (Button_TypeDef Button) |
Push Button DeInit. | |
uint32_t | BSP_PB_GetState (Button_TypeDef Button) |
Returns the selected button state. | |
void | BSP_COM_Init (COM_TypeDef COM, UART_HandleTypeDef *huart) |
Configures COM port. | |
void | BSP_COM_DeInit (COM_TypeDef COM, UART_HandleTypeDef *huart) |
DeInit COM port. | |
Variables | |
const uint32_t | GPIO_PIN [LEDn] |
GPIO_TypeDef * | BUTTON_PORT [BUTTONn] |
const uint16_t | BUTTON_PIN [BUTTONn] |
const uint16_t | BUTTON_IRQn [BUTTONn] |
USART_TypeDef * | COM_USART [COMn] = {EVAL_COM1} |
GPIO_TypeDef * | COM_TX_PORT [COMn] = {EVAL_COM1_TX_GPIO_PORT} |
GPIO_TypeDef * | COM_RX_PORT [COMn] = {EVAL_COM1_RX_GPIO_PORT} |
const uint16_t | COM_TX_PIN [COMn] = {EVAL_COM1_TX_PIN} |
const uint16_t | COM_RX_PIN [COMn] = {EVAL_COM1_RX_PIN} |
const uint16_t | COM_TX_AF [COMn] = {EVAL_COM1_TX_AF} |
const uint16_t | COM_RX_AF [COMn] = {EVAL_COM1_RX_AF} |
static FMPI2C_HandleTypeDef | hEvalI2c |
Detailed Description
This file provides a set of firmware functions to manage LEDs, push-buttons and COM ports available on STM32446E-EVAL evaluation board(MB1045) RevB from STMicroelectronics.
- Attention:
© COPYRIGHT(c) 2015 STMicroelectronics
Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: 1. Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. 2. Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. 3. Neither the name of STMicroelectronics nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
Definition in file stm32446e_eval.c.
Generated on Fri Jan 15 2016 10:06:21 for STM32446E_EVAL BSP User Manual by
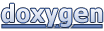