STM32446E_EVAL BSP User Manual
|
Functions | |
uint8_t | BSP_AUDIO_OUT_Init (uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq) |
Configures the audio peripherals. | |
uint8_t | BSP_AUDIO_OUT_Play (uint16_t *pBuffer, uint32_t Size) |
Starts playing audio stream from a data buffer for a determined size. | |
void | BSP_AUDIO_OUT_ChangeBuffer (uint16_t *pData, uint16_t Size) |
Sends n-Bytes on the SAI interface. | |
uint8_t | BSP_AUDIO_OUT_Pause (void) |
This function Pauses the audio file stream. | |
uint8_t | BSP_AUDIO_OUT_Resume (void) |
This function Resumes the audio file stream. | |
uint8_t | BSP_AUDIO_OUT_Stop (uint32_t Option) |
Stops audio playing and Power down the Audio Codec. | |
uint8_t | BSP_AUDIO_OUT_SetVolume (uint8_t Volume) |
Controls the current audio volume level. | |
void | BSP_AUDIO_OUT_SetFrequency (uint32_t AudioFreq) |
Updates the audio frequency. | |
void | BSP_AUDIO_OUT_SetAudioFrameSlot (uint32_t AudioFrameSlot) |
Updates the Audio frame slot configuration. | |
uint8_t | BSP_AUDIO_OUT_SetMute (uint32_t Cmd) |
Enables or disables the MUTE mode by software. | |
uint8_t | BSP_AUDIO_OUT_SetOutputMode (uint8_t Output) |
Switch dynamically (while audio file is played) the output target (speaker or headphone). | |
void | BSP_AUDIO_OUT_DeInit (void) |
Deinit the audio peripherals. | |
void | BSP_AUDIO_OUT_TransferComplete_CallBack (void) |
Manages the DMA full Transfer complete event. | |
void | BSP_AUDIO_OUT_HalfTransfer_CallBack (void) |
Manages the DMA Half Transfer complete event. | |
void | BSP_AUDIO_OUT_Error_CallBack (void) |
Manages the DMA FIFO error event. | |
void | BSP_AUDIO_OUT_ClockConfig (SAI_HandleTypeDef *hsai, uint32_t AudioFreq, void *Params) |
Clock Config. | |
void | BSP_AUDIO_OUT_MspInit (SAI_HandleTypeDef *hsai, void *Params) |
Initializes BSP_AUDIO_OUT MSP. | |
void | BSP_AUDIO_OUT_MspDeInit (SAI_HandleTypeDef *hsai, void *Params) |
Deinitializes SAI MSP. |
Function Documentation
void BSP_AUDIO_OUT_ChangeBuffer | ( | uint16_t * | pData, |
uint16_t | Size | ||
) |
Sends n-Bytes on the SAI interface.
- Parameters:
-
pData,: pointer on data address Size,: number of data to be written
Definition at line 281 of file stm32446e_eval_audio.c.
References haudio_out_sai.
void BSP_AUDIO_OUT_ClockConfig | ( | SAI_HandleTypeDef * | hsai, |
uint32_t | AudioFreq, | ||
void * | Params | ||
) |
Clock Config.
- Parameters:
-
hsai,: might be required to set audio peripheral predivider if any. AudioFreq,: Audio frequency used to play the audio stream. Params,: pointer on additional configuration parameters, can be NULL.
- Note:
- This API is called by BSP_AUDIO_OUT_Init() and BSP_AUDIO_OUT_SetFrequency() Being __weak it can be overwritten by the application
Definition at line 647 of file stm32446e_eval_audio.c.
Referenced by BSP_AUDIO_OUT_Init(), and BSP_AUDIO_OUT_SetFrequency().
void BSP_AUDIO_OUT_DeInit | ( | void | ) |
Deinit the audio peripherals.
Definition at line 472 of file stm32446e_eval_audio.c.
References BSP_AUDIO_OUT_MspDeInit(), haudio_out_sai, and SAIx_DeInit().
void BSP_AUDIO_OUT_Error_CallBack | ( | void | ) |
Manages the DMA FIFO error event.
Definition at line 527 of file stm32446e_eval_audio.c.
Referenced by HAL_SAI_ErrorCallback().
void BSP_AUDIO_OUT_HalfTransfer_CallBack | ( | void | ) |
Manages the DMA Half Transfer complete event.
Definition at line 520 of file stm32446e_eval_audio.c.
Referenced by HAL_SAI_TxHalfCpltCallback().
uint8_t BSP_AUDIO_OUT_Init | ( | uint16_t | OutputDevice, |
uint8_t | Volume, | ||
uint32_t | AudioFreq | ||
) |
Configures the audio peripherals.
- Parameters:
-
OutputDevice,: OUTPUT_DEVICE_SPEAKER, OUTPUT_DEVICE_HEADPHONE, or OUTPUT_DEVICE_BOTH. Volume,: Initial volume level (from 0 (Mute) to 100 (Max)) AudioFreq,: Audio frequency used to play the audio stream.
- Note:
- The I2S PLL input clock must be done in the user application.
- Return values:
-
AUDIO_OK if correct communication, else wrong communication
Definition at line 209 of file stm32446e_eval_audio.c.
References audio_drv, AUDIO_ERROR, AUDIO_I2C_ADDRESS, AUDIO_OK, AUDIO_SAIx, BSP_AUDIO_OUT_ClockConfig(), BSP_AUDIO_OUT_MspInit(), haudio_out_sai, SAIx_DeInit(), and SAIx_Init().
void BSP_AUDIO_OUT_MspDeInit | ( | SAI_HandleTypeDef * | hsai, |
void * | Params | ||
) |
Deinitializes SAI MSP.
- Parameters:
-
hsai,: SAI handle Params,: pointer on additional configuration parameters, can be NULL.
Definition at line 606 of file stm32446e_eval_audio.c.
References AUDIO_SAIx, AUDIO_SAIx_CLK_DISABLE, AUDIO_SAIx_DMAx_IRQ, AUDIO_SAIx_FS_PIN, AUDIO_SAIx_MCK_PIN, AUDIO_SAIx_MCLK_SCK_GPIO_PORT, AUDIO_SAIx_SCK_PIN, AUDIO_SAIx_SD_FS_GPIO_PORT, and AUDIO_SAIx_SD_PIN.
Referenced by BSP_AUDIO_OUT_DeInit().
void BSP_AUDIO_OUT_MspInit | ( | SAI_HandleTypeDef * | hsai, |
void * | Params | ||
) |
Initializes BSP_AUDIO_OUT MSP.
- Parameters:
-
hsai,: SAI handle Params,: pointer on additional configuration parameters, can be NULL.
Definition at line 536 of file stm32446e_eval_audio.c.
References AUDIO_OUT_IRQ_PREPRIO, AUDIO_SAIx, AUDIO_SAIx_CLK_ENABLE, AUDIO_SAIx_DMAx_CHANNEL, AUDIO_SAIx_DMAx_CLK_ENABLE, AUDIO_SAIx_DMAx_IRQ, AUDIO_SAIx_DMAx_MEM_DATA_SIZE, AUDIO_SAIx_DMAx_PERIPH_DATA_SIZE, AUDIO_SAIx_DMAx_STREAM, AUDIO_SAIx_FS_PIN, AUDIO_SAIx_MCK_PIN, AUDIO_SAIx_MCLK_SCK_ENABLE, AUDIO_SAIx_MCLK_SCK_GPIO_PORT, AUDIO_SAIx_MCLK_SD_FS_AF, AUDIO_SAIx_SCK_AF, AUDIO_SAIx_SCK_PIN, AUDIO_SAIx_SD_FS_ENABLE, AUDIO_SAIx_SD_FS_GPIO_PORT, and AUDIO_SAIx_SD_PIN.
Referenced by BSP_AUDIO_OUT_Init().
uint8_t BSP_AUDIO_OUT_Pause | ( | void | ) |
This function Pauses the audio file stream.
In case of using DMA, the DMA Pause feature is used. WARNING: When calling BSP_AUDIO_OUT_Pause() function for pause, only BSP_AUDIO_OUT_Resume() function should be called for resume (use of BSP_AUDIO_OUT_Play() function for resume could lead to unexpected behavior).
- Return values:
-
AUDIO_OK if correct communication, else wrong communication
Definition at line 294 of file stm32446e_eval_audio.c.
References audio_drv, AUDIO_ERROR, AUDIO_I2C_ADDRESS, AUDIO_OK, and haudio_out_sai.
uint8_t BSP_AUDIO_OUT_Play | ( | uint16_t * | pBuffer, |
uint32_t | Size | ||
) |
Starts playing audio stream from a data buffer for a determined size.
- Parameters:
-
pBuffer,: Pointer to the buffer Size,: Number of audio data BYTES.
- Return values:
-
AUDIO_OK if correct communication, else wrong communication
Definition at line 260 of file stm32446e_eval_audio.c.
References audio_drv, AUDIO_ERROR, AUDIO_I2C_ADDRESS, AUDIO_OK, AUDIODATA_SIZE, DMA_MAX, and haudio_out_sai.
uint8_t BSP_AUDIO_OUT_Resume | ( | void | ) |
This function Resumes the audio file stream.
WARNING: When calling BSP_AUDIO_OUT_Pause() function for pause, only BSP_AUDIO_OUT_Resume() function should be called for resume (use of BSP_AUDIO_OUT_Play() function for resume could lead to unexpected behavior).
- Return values:
-
AUDIO_OK if correct communication, else wrong communication
Definition at line 318 of file stm32446e_eval_audio.c.
References audio_drv, AUDIO_ERROR, AUDIO_I2C_ADDRESS, AUDIO_OK, and haudio_out_sai.
void BSP_AUDIO_OUT_SetAudioFrameSlot | ( | uint32_t | AudioFrameSlot | ) |
Updates the Audio frame slot configuration.
- Parameters:
-
AudioFrameSlot,: specifies the audio Frame slot This parameter can be any value of STM32446E EVAL Audio Slot TDM mode
- Note:
- This API should be called after the BSP_AUDIO_OUT_Init() to adjust the audio frame slot.
Definition at line 456 of file stm32446e_eval_audio.c.
References haudio_out_sai.
void BSP_AUDIO_OUT_SetFrequency | ( | uint32_t | AudioFreq | ) |
Updates the audio frequency.
- Parameters:
-
AudioFreq,: Audio frequency used to play the audio stream.
- Note:
- This API should be called after the BSP_AUDIO_OUT_Init() to adjust the audio frequency.
Definition at line 433 of file stm32446e_eval_audio.c.
References BSP_AUDIO_OUT_ClockConfig(), and haudio_out_sai.
uint8_t BSP_AUDIO_OUT_SetMute | ( | uint32_t | Cmd | ) |
Enables or disables the MUTE mode by software.
- Parameters:
-
Cmd,: Could be AUDIO_MUTE_ON to mute sound or AUDIO_MUTE_OFF to unmute the codec and restore previous volume level.
- Return values:
-
AUDIO_OK if correct communication, else wrong communication
Definition at line 392 of file stm32446e_eval_audio.c.
References audio_drv, AUDIO_ERROR, AUDIO_I2C_ADDRESS, and AUDIO_OK.
uint8_t BSP_AUDIO_OUT_SetOutputMode | ( | uint8_t | Output | ) |
Switch dynamically (while audio file is played) the output target (speaker or headphone).
- Parameters:
-
Output,: The audio output target: OUTPUT_DEVICE_SPEAKER, OUTPUT_DEVICE_HEADPHONE or OUTPUT_DEVICE_BOTH
- Return values:
-
AUDIO_OK if correct communication, else wrong communication
Definition at line 413 of file stm32446e_eval_audio.c.
References audio_drv, AUDIO_ERROR, AUDIO_I2C_ADDRESS, and AUDIO_OK.
uint8_t BSP_AUDIO_OUT_SetVolume | ( | uint8_t | Volume | ) |
Controls the current audio volume level.
- Parameters:
-
Volume,: Volume level to be set in percentage from 0% to 100% (0 for Mute and 100 for Max volume level).
- Return values:
-
AUDIO_OK if correct communication, else wrong communication
Definition at line 372 of file stm32446e_eval_audio.c.
References audio_drv, AUDIO_ERROR, AUDIO_I2C_ADDRESS, and AUDIO_OK.
uint8_t BSP_AUDIO_OUT_Stop | ( | uint32_t | Option | ) |
Stops audio playing and Power down the Audio Codec.
- Parameters:
-
Option,: could be one of the following parameters - CODEC_PDWN_SW: for software power off (by writing registers). Then no need to reconfigure the Codec after power on.
- CODEC_PDWN_HW: completely shut down the codec (physically). Then need to reconfigure the Codec after power on.
- Return values:
-
AUDIO_OK if correct communication, else wrong communication
Definition at line 344 of file stm32446e_eval_audio.c.
References audio_drv, AUDIO_ERROR, AUDIO_I2C_ADDRESS, AUDIO_OK, and haudio_out_sai.
void BSP_AUDIO_OUT_TransferComplete_CallBack | ( | void | ) |
Manages the DMA full Transfer complete event.
Definition at line 513 of file stm32446e_eval_audio.c.
Referenced by HAL_SAI_TxCpltCallback().
Generated on Fri Jan 15 2016 10:06:22 for STM32446E_EVAL BSP User Manual by
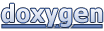