STM32446E_EVAL BSP User Manual
|
stm32446e_eval_io.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32446e_eval_io.h 00004 * @author MCD Application Team 00005 * @version V1.1.1 00006 * @date 13-January-2016 00007 * @brief This file contains the common defines and functions prototypes for 00008 * the stm32446e_eval_io.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32446E_EVAL_IO_H 00041 #define __STM32446E_EVAL_IO_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "stm32446e_eval.h" 00049 /* Include IO component driver */ 00050 #include "../Components/mfxstm32l152/mfxstm32l152.h" 00051 00052 /** @addtogroup BSP 00053 * @{ 00054 */ 00055 00056 /** @addtogroup STM32446E_EVAL 00057 * @{ 00058 */ 00059 00060 /** @defgroup STM32446E_EVAL_IO STM32446E EVAL IO 00061 * @{ 00062 */ 00063 00064 /** @defgroup STM32446E_EVAL_IO_Exported_Types STM32446E EVAL IO Exported Types 00065 * @{ 00066 */ 00067 00068 typedef enum 00069 { 00070 BSP_IO_PIN_RESET = 0, 00071 BSP_IO_PIN_SET = 1 00072 }BSP_IO_PinStateTypeDef; 00073 00074 typedef enum 00075 { 00076 IO_OK = 0, 00077 IO_ERROR = 1, 00078 IO_TIMEOUT = 2 00079 }IO_StatusTypeDef; 00080 00081 /** 00082 * @} 00083 */ 00084 00085 /** @defgroup STM32446E_EVAL_IO_Exported_Constants STM32446E EVAL IO Exported Constants 00086 * @{ 00087 */ 00088 #define IO_PIN_0 ((uint32_t)0x0001) 00089 #define IO_PIN_1 ((uint32_t)0x0002) 00090 #define IO_PIN_2 ((uint32_t)0x0004) 00091 #define IO_PIN_3 ((uint32_t)0x0008) 00092 #define IO_PIN_4 ((uint32_t)0x0010) 00093 #define IO_PIN_5 ((uint32_t)0x0020) 00094 #define IO_PIN_6 ((uint32_t)0x0040) 00095 #define IO_PIN_7 ((uint32_t)0x0080) 00096 #define IO_PIN_8 ((uint32_t)0x0100) 00097 #define IO_PIN_9 ((uint32_t)0x0200) 00098 #define IO_PIN_10 ((uint32_t)0x0400) 00099 #define IO_PIN_11 ((uint32_t)0x0800) 00100 #define IO_PIN_12 ((uint32_t)0x1000) 00101 #define IO_PIN_13 ((uint32_t)0x2000) 00102 #define IO_PIN_14 ((uint32_t)0x4000) 00103 #define IO_PIN_15 ((uint32_t)0x8000) 00104 #define IO_PIN_16 ((uint32_t)0x010000) 00105 #define IO_PIN_17 ((uint32_t)0x020000) 00106 #define IO_PIN_18 ((uint32_t)0x040000) 00107 #define IO_PIN_19 ((uint32_t)0x080000) 00108 #define IO_PIN_20 ((uint32_t)0x100000) 00109 #define IO_PIN_21 ((uint32_t)0x200000) 00110 #define IO_PIN_22 ((uint32_t)0x400000) 00111 #define IO_PIN_23 ((uint32_t)0x800000) 00112 #define IO_PIN_ALL ((uint32_t)0xFFFFFF) 00113 /** 00114 * @} 00115 */ 00116 00117 /** @defgroup STM32446E_EVAL_IO_Exported_Macro STM32446E EVAL IO Exported Macro 00118 * @{ 00119 */ 00120 /** 00121 * @} 00122 */ 00123 00124 /** @defgroup STM32446E_EVAL_IO_Exported_Functions STM32446E EVAL IO Exported Functions 00125 * @{ 00126 */ 00127 uint8_t BSP_IO_Init(void); 00128 uint8_t BSP_IO_DeInit(void); 00129 uint8_t BSP_IO_ConfigIrqOutPin(uint8_t IoIrqOutPinPolarity, uint8_t IoIrqOutPinType); 00130 uint32_t BSP_IO_ITGetStatus(uint32_t IoPin); 00131 void BSP_IO_ITClear(void); 00132 uint8_t BSP_IO_ConfigPin(uint32_t IoPin, IO_ModeTypedef IoMode); 00133 void BSP_IO_WritePin(uint32_t IoPin, BSP_IO_PinStateTypeDef PinState); 00134 uint32_t BSP_IO_ReadPin(uint32_t IoPin); 00135 void BSP_IO_TogglePin(uint32_t IoPin); 00136 00137 /** 00138 * @} 00139 */ 00140 00141 /** 00142 * @} 00143 */ 00144 00145 /** 00146 * @} 00147 */ 00148 00149 /** 00150 * @} 00151 */ 00152 00153 #ifdef __cplusplus 00154 } 00155 #endif 00156 00157 #endif /* __STM32446E_EVAL_IO_H */ 00158 00159 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jan 15 2016 10:06:21 for STM32446E_EVAL BSP User Manual by
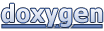