STM32446E_EVAL BSP User Manual
|
stm32446e_eval_sd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32446e_eval_sd.c 00004 * @author MCD Application Team 00005 * @version V1.1.1 00006 * @date 13-January-2016 00007 * @brief This file includes the uSD card driver mounted on STM32446E-EVAL 00008 * evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the micro SD external card mounted on STM32446E-EVAL 00044 evaluation board. 00045 - This driver does not need a specific component driver for the micro SD device 00046 to be included with. 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps: 00051 o Initialize the micro SD card using the BSP_SD_Init() function. This 00052 function includes the MSP layer hardware resources initialization and the 00053 SDIO interface configuration to interface with the external micro SD. It 00054 also includes the micro SD initialization sequence. 00055 o To check the SD card presence you can use the function BSP_SD_IsDetected() which 00056 returns the detection status 00057 o If SD presence detection interrupt mode is desired, you must configure the 00058 SD detection interrupt mode by calling the function BSP_SD_ITConfig(). The interrupt 00059 is generated as an external interrupt whenever the micro SD card is 00060 plugged/unplugged in/from the evaluation board. The SD detection interrupt 00061 is handled by calling the function BSP_SD_DetectIT() which is called in the IRQ 00062 handler file, the user callback is implemented in the function BSP_SD_DetectCallback(). 00063 o The function BSP_SD_GetCardInfo() is used to get the micro SD card information 00064 which is stored in the structure "HAL_SD_CardInfoTypedef". 00065 00066 + Micro SD card operations 00067 o The micro SD card can be accessed with read/write block(s) operations once 00068 it is ready for access. The access can be performed whether using the polling 00069 mode by calling the functions BSP_SD_ReadBlocks()/BSP_SD_WriteBlocks(), or by DMA 00070 transfer using the functions BSP_SD_ReadBlocks_DMA()/BSP_SD_WriteBlocks_DMA() 00071 o The DMA transfer complete is used with interrupt mode. Once the SD transfer 00072 is complete, the SD interrupt is handled using the function BSP_SD_IRQHandler(), 00073 the DMA Tx/Rx transfer complete are handled using the functions 00074 BSP_SD_DMA_Tx_IRQHandler()/BSP_SD_DMA_Rx_IRQHandler(). The corresponding user callbacks 00075 are implemented by the user at application level. 00076 o The SD erase block(s) is performed using the function BSP_SD_Erase() with specifying 00077 the number of blocks to erase. 00078 o The SD runtime status is returned when calling the function BSP_SD_GetStatus(). 00079 00080 ------------------------------------------------------------------------------*/ 00081 00082 /* Includes ------------------------------------------------------------------*/ 00083 #include "stm32446e_eval_sd.h" 00084 00085 /** @addtogroup BSP 00086 * @{ 00087 */ 00088 00089 /** @addtogroup STM32446E_EVAL 00090 * @{ 00091 */ 00092 00093 /** @defgroup STM32446E_EVAL_SD STM32446E EVAL SD 00094 * @{ 00095 */ 00096 00097 00098 /** @defgroup STM32446E_EVAL_SD_Private_TypesDefinitions STM32446E EVAL SD Private TypesDefinitions 00099 * @{ 00100 */ 00101 /** 00102 * @} 00103 */ 00104 00105 /** @defgroup STM32446E_EVAL_SD_Private_Defines STM32446E EVAL SD Private Defines 00106 * @{ 00107 */ 00108 /** 00109 * @} 00110 */ 00111 00112 /** @defgroup STM32446E_EVAL_SD_Private_Macros STM32446E EVAL SD Private Macros 00113 * @{ 00114 */ 00115 /** 00116 * @} 00117 */ 00118 00119 /** @defgroup STM32446E_EVAL_SD_Private_Variables STM32446E EVAL SD Private Variables 00120 * @{ 00121 */ 00122 static SD_HandleTypeDef uSdHandle; 00123 static SD_CardInfo uSdCardInfo; 00124 static uint8_t UseExtiModeDetection = 0; 00125 00126 /** 00127 * @} 00128 */ 00129 00130 /** @defgroup STM32446E_EVAL_SD_Private_FunctionPrototypes STM32446E EVAL SD Private FunctionPrototypes 00131 * @{ 00132 */ 00133 /** 00134 * @} 00135 */ 00136 00137 /** @defgroup STM32446E_EVAL_SD_Private_Functions STM32446E EVAL SD Private Functions 00138 * @{ 00139 */ 00140 00141 /** 00142 * @brief Initializes the SD card device. 00143 * @retval SD status 00144 */ 00145 uint8_t BSP_SD_Init(void) 00146 { 00147 uint8_t sd_state = MSD_OK; 00148 00149 /* uSD device interface configuration */ 00150 uSdHandle.Instance = SDIO; 00151 00152 uSdHandle.Init.ClockEdge = SDIO_CLOCK_EDGE_RISING; 00153 uSdHandle.Init.ClockBypass = SDIO_CLOCK_BYPASS_DISABLE; 00154 uSdHandle.Init.ClockPowerSave = SDIO_CLOCK_POWER_SAVE_DISABLE; 00155 uSdHandle.Init.BusWide = SDIO_BUS_WIDE_1B; 00156 uSdHandle.Init.HardwareFlowControl = SDIO_HARDWARE_FLOW_CONTROL_ENABLE; 00157 uSdHandle.Init.ClockDiv = SDIO_TRANSFER_CLK_DIV; 00158 00159 /* Initialize IO functionalities (MFX) used by SD detect pin */ 00160 BSP_IO_Init(); 00161 00162 /* Check if the SD card is plugged in the slot */ 00163 BSP_IO_ConfigPin(SD_DETECT_PIN, IO_MODE_INPUT); 00164 if(BSP_SD_IsDetected() != SD_PRESENT) 00165 { 00166 return MSD_ERROR_SD_NOT_PRESENT; 00167 } 00168 00169 /* Msp SD initialization */ 00170 BSP_SD_MspInit(&uSdHandle, NULL); 00171 00172 /* HAL SD initialization */ 00173 if(HAL_SD_Init(&uSdHandle, &uSdCardInfo) != SD_OK) 00174 { 00175 sd_state = MSD_ERROR; 00176 } 00177 00178 /* Configure SD Bus width */ 00179 if(sd_state == MSD_OK) 00180 { 00181 /* Enable wide operation */ 00182 if(HAL_SD_WideBusOperation_Config(&uSdHandle, SDIO_BUS_WIDE_4B) != SD_OK) 00183 { 00184 sd_state = MSD_ERROR; 00185 } 00186 else 00187 { 00188 sd_state = MSD_OK; 00189 } 00190 } 00191 00192 return sd_state; 00193 } 00194 00195 /** 00196 * @brief DeInitializes the SD card device. 00197 * @retval SD status 00198 */ 00199 uint8_t BSP_SD_DeInit(void) 00200 { 00201 uint8_t sd_state = MSD_OK; 00202 00203 uSdHandle.Instance = SDIO; 00204 00205 /* Set back Mfx pin to INPUT mode in case it was in exti */ 00206 UseExtiModeDetection = 0; 00207 BSP_IO_ConfigPin(SD_DETECT_PIN, IO_MODE_INPUT); 00208 00209 /* HAL SD deinitialization */ 00210 if(HAL_SD_DeInit(&uSdHandle) != HAL_OK) 00211 { 00212 sd_state = MSD_ERROR; 00213 } 00214 00215 /* Msp SD deinitialization */ 00216 uSdHandle.Instance = SDIO; 00217 BSP_SD_MspDeInit(&uSdHandle, NULL); 00218 00219 return sd_state; 00220 } 00221 00222 /** 00223 * @brief Configures Interrupt mode for SD detection pin. 00224 * @retval Returns 0 00225 */ 00226 uint8_t BSP_SD_ITConfig(void) 00227 { 00228 /* Configure Interrupt mode for SD detection pin */ 00229 /* Note: disabling exti mode can be done calling SD_DeInit() */ 00230 UseExtiModeDetection = 1; 00231 BSP_SD_IsDetected(); 00232 00233 return 0; 00234 } 00235 00236 /** 00237 * @brief Detects if SD card is correctly plugged in the memory slot or not. 00238 * @retval Returns if SD is detected or not 00239 */ 00240 uint8_t BSP_SD_IsDetected(void) 00241 { 00242 __IO uint8_t status = SD_PRESENT; 00243 00244 /* Check SD card detect pin */ 00245 if((BSP_IO_ReadPin(SD_DETECT_PIN)&SD_DETECT_PIN) != SD_DETECT_PIN) 00246 { 00247 if (UseExtiModeDetection) 00248 { 00249 BSP_IO_ConfigPin(SD_DETECT_PIN, IO_MODE_IT_RISING_EDGE); 00250 } 00251 00252 } 00253 else 00254 { 00255 status = SD_NOT_PRESENT; 00256 if (UseExtiModeDetection) 00257 { 00258 BSP_IO_ConfigPin(SD_DETECT_PIN, IO_MODE_IT_FALLING_EDGE); 00259 } 00260 } 00261 00262 return status; 00263 } 00264 00265 00266 00267 /** 00268 * @brief Reads block(s) from a specified address in an SD card, in polling mode. 00269 * @param pData: Pointer to the buffer that will contain the data to transmit 00270 * @param ReadAddr: Address from where data is to be read 00271 * @param BlockSize: SD card data block size, that should be 512 00272 * @param NumOfBlocks: Number of SD blocks to read 00273 * @retval SD status 00274 */ 00275 uint8_t BSP_SD_ReadBlocks(uint32_t *pData, uint64_t ReadAddr, uint32_t BlockSize, uint32_t NumOfBlocks) 00276 { 00277 if(HAL_SD_ReadBlocks(&uSdHandle, pData, ReadAddr, BlockSize, NumOfBlocks) != SD_OK) 00278 { 00279 return MSD_ERROR; 00280 } 00281 else 00282 { 00283 return MSD_OK; 00284 } 00285 } 00286 00287 /** 00288 * @brief Writes block(s) to a specified address in an SD card, in polling mode. 00289 * @param pData: Pointer to the buffer that will contain the data to transmit 00290 * @param WriteAddr: Address from where data is to be written 00291 * @param BlockSize: SD card data block size, that should be 512 00292 * @param NumOfBlocks: Number of SD blocks to write 00293 * @retval SD status 00294 */ 00295 uint8_t BSP_SD_WriteBlocks(uint32_t *pData, uint64_t WriteAddr, uint32_t BlockSize, uint32_t NumOfBlocks) 00296 { 00297 if(HAL_SD_WriteBlocks(&uSdHandle, pData, WriteAddr, BlockSize, NumOfBlocks) != SD_OK) 00298 { 00299 return MSD_ERROR; 00300 } 00301 else 00302 { 00303 return MSD_OK; 00304 } 00305 } 00306 00307 /** 00308 * @brief Reads block(s) from a specified address in an SD card, in DMA mode. 00309 * @param pData: Pointer to the buffer that will contain the data to transmit 00310 * @param ReadAddr: Address from where data is to be read 00311 * @param BlockSize: SD card data block size, that should be 512 00312 * @param NumOfBlocks: Number of SD blocks to read 00313 * @retval SD status 00314 */ 00315 uint8_t BSP_SD_ReadBlocks_DMA(uint32_t *pData, uint64_t ReadAddr, uint32_t BlockSize, uint32_t NumOfBlocks) 00316 { 00317 uint8_t sd_state = MSD_OK; 00318 00319 /* Read block(s) in DMA transfer mode */ 00320 if(HAL_SD_ReadBlocks_DMA(&uSdHandle, pData, ReadAddr, BlockSize, NumOfBlocks) != SD_OK) 00321 { 00322 sd_state = MSD_ERROR; 00323 } 00324 00325 /* Wait until transfer is complete */ 00326 if(sd_state == MSD_OK) 00327 { 00328 if(HAL_SD_CheckReadOperation(&uSdHandle, (uint32_t)SD_DATATIMEOUT) != SD_OK) 00329 { 00330 sd_state = MSD_ERROR; 00331 } 00332 else 00333 { 00334 sd_state = MSD_OK; 00335 } 00336 } 00337 00338 return sd_state; 00339 } 00340 00341 /** 00342 * @brief Writes block(s) to a specified address in an SD card, in DMA mode. 00343 * @param pData: Pointer to the buffer that will contain the data to transmit 00344 * @param WriteAddr: Address from where data is to be written 00345 * @param BlockSize: SD card data block size, that should be 512 00346 * @param NumOfBlocks: Number of SD blocks to write 00347 * @retval SD status 00348 */ 00349 uint8_t BSP_SD_WriteBlocks_DMA(uint32_t *pData, uint64_t WriteAddr, uint32_t BlockSize, uint32_t NumOfBlocks) 00350 { 00351 uint8_t sd_state = MSD_OK; 00352 00353 /* Write block(s) in DMA transfer mode */ 00354 if(HAL_SD_WriteBlocks_DMA(&uSdHandle, pData, WriteAddr, BlockSize, NumOfBlocks) != SD_OK) 00355 { 00356 sd_state = MSD_ERROR; 00357 } 00358 00359 /* Wait until transfer is complete */ 00360 if(sd_state == MSD_OK) 00361 { 00362 if(HAL_SD_CheckWriteOperation(&uSdHandle, (uint32_t)SD_DATATIMEOUT) != SD_OK) 00363 { 00364 sd_state = MSD_ERROR; 00365 } 00366 else 00367 { 00368 sd_state = MSD_OK; 00369 } 00370 } 00371 00372 return sd_state; 00373 } 00374 00375 /** 00376 * @brief Erases the specified memory area of the given SD card. 00377 * @param StartAddr: Start byte address 00378 * @param EndAddr: End byte address 00379 * @retval SD status 00380 */ 00381 uint8_t BSP_SD_Erase(uint64_t StartAddr, uint64_t EndAddr) 00382 { 00383 if(HAL_SD_Erase(&uSdHandle, StartAddr, EndAddr) != SD_OK) 00384 { 00385 return MSD_ERROR; 00386 } 00387 else 00388 { 00389 return MSD_OK; 00390 } 00391 } 00392 00393 /** 00394 * @brief Initializes the SD MSP. 00395 * @param hsd: SD handle 00396 * @param Params: pointer on additional configuration parameters, can be NULL. 00397 */ 00398 __weak void BSP_SD_MspInit(SD_HandleTypeDef *hsd, void *Params) 00399 { 00400 static DMA_HandleTypeDef dma_rx_handle; 00401 static DMA_HandleTypeDef dma_tx_handle; 00402 GPIO_InitTypeDef gpio_init_structure; 00403 00404 /* SD pins are in conflict with Camera pins therefore Camera is power down */ 00405 /* __weak function can be modified by the application */ 00406 BSP_IO_ConfigPin(RSTI_PIN, IO_MODE_OUTPUT); 00407 BSP_IO_ConfigPin(XSDN_PIN, IO_MODE_OUTPUT); 00408 /* De-assert the camera STANDBY pin (active high) */ 00409 BSP_IO_WritePin(XSDN_PIN, BSP_IO_PIN_RESET); 00410 /* Assert the camera RSTI pin (active low) */ 00411 BSP_IO_WritePin(RSTI_PIN, BSP_IO_PIN_RESET); 00412 HAL_Delay(100); 00413 00414 /* Enable SDIO clock */ 00415 __HAL_RCC_SDIO_CLK_ENABLE(); 00416 00417 /* Enable DMA2 clocks */ 00418 __DMAx_TxRx_CLK_ENABLE(); 00419 00420 /* Enable GPIOs clock */ 00421 __HAL_RCC_GPIOC_CLK_ENABLE(); 00422 __HAL_RCC_GPIOD_CLK_ENABLE(); 00423 00424 /* Common GPIO configuration */ 00425 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00426 gpio_init_structure.Pull = GPIO_PULLUP; 00427 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00428 gpio_init_structure.Alternate = GPIO_AF12_SDIO; 00429 00430 /* GPIOC configuration */ 00431 gpio_init_structure.Pin = GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12; 00432 00433 HAL_GPIO_Init(GPIOC, &gpio_init_structure); 00434 00435 /* GPIOD configuration */ 00436 gpio_init_structure.Pin = GPIO_PIN_2; 00437 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00438 00439 /* NVIC configuration for SDIO interrupts */ 00440 HAL_NVIC_SetPriority(SDIO_IRQn, 5, 0); 00441 HAL_NVIC_EnableIRQ(SDIO_IRQn); 00442 00443 /* Configure DMA Rx parameters */ 00444 dma_rx_handle.Init.Channel = SD_DMAx_Rx_CHANNEL; 00445 dma_rx_handle.Init.Direction = DMA_PERIPH_TO_MEMORY; 00446 dma_rx_handle.Init.PeriphInc = DMA_PINC_DISABLE; 00447 dma_rx_handle.Init.MemInc = DMA_MINC_ENABLE; 00448 dma_rx_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00449 dma_rx_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00450 dma_rx_handle.Init.Mode = DMA_PFCTRL; 00451 dma_rx_handle.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00452 dma_rx_handle.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00453 dma_rx_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00454 dma_rx_handle.Init.MemBurst = DMA_MBURST_INC4; 00455 dma_rx_handle.Init.PeriphBurst = DMA_PBURST_INC4; 00456 00457 dma_rx_handle.Instance = SD_DMAx_Rx_STREAM; 00458 00459 /* Associate the DMA handle */ 00460 __HAL_LINKDMA(hsd, hdmarx, dma_rx_handle); 00461 00462 /* Deinitialize the stream for new transfer */ 00463 HAL_DMA_DeInit(&dma_rx_handle); 00464 00465 /* Configure the DMA stream */ 00466 HAL_DMA_Init(&dma_rx_handle); 00467 00468 /* Configure DMA Tx parameters */ 00469 dma_tx_handle.Init.Channel = SD_DMAx_Tx_CHANNEL; 00470 dma_tx_handle.Init.Direction = DMA_MEMORY_TO_PERIPH; 00471 dma_tx_handle.Init.PeriphInc = DMA_PINC_DISABLE; 00472 dma_tx_handle.Init.MemInc = DMA_MINC_ENABLE; 00473 dma_tx_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00474 dma_tx_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00475 dma_tx_handle.Init.Mode = DMA_PFCTRL; 00476 dma_tx_handle.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00477 dma_tx_handle.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00478 dma_tx_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00479 dma_tx_handle.Init.MemBurst = DMA_MBURST_INC4; 00480 dma_tx_handle.Init.PeriphBurst = DMA_PBURST_INC4; 00481 00482 dma_tx_handle.Instance = SD_DMAx_Tx_STREAM; 00483 00484 /* Associate the DMA handle */ 00485 __HAL_LINKDMA(hsd, hdmatx, dma_tx_handle); 00486 00487 /* Deinitialize the stream for new transfer */ 00488 HAL_DMA_DeInit(&dma_tx_handle); 00489 00490 /* Configure the DMA stream */ 00491 HAL_DMA_Init(&dma_tx_handle); 00492 00493 /* NVIC configuration for DMA transfer complete interrupt */ 00494 HAL_NVIC_SetPriority(SD_DMAx_Rx_IRQn, 6, 0); 00495 HAL_NVIC_EnableIRQ(SD_DMAx_Rx_IRQn); 00496 00497 /* NVIC configuration for DMA transfer complete interrupt */ 00498 HAL_NVIC_SetPriority(SD_DMAx_Tx_IRQn, 6, 0); 00499 HAL_NVIC_EnableIRQ(SD_DMAx_Tx_IRQn); 00500 } 00501 00502 /** 00503 * @brief DeInitializes the SD MSP. 00504 * @param hsd: SD handle 00505 * @param Params: pointer on additional configuration parameters, can be NULL. 00506 */ 00507 __weak void BSP_SD_MspDeInit(SD_HandleTypeDef *hsd, void *Params) 00508 { 00509 static DMA_HandleTypeDef dma_rx_handle; 00510 static DMA_HandleTypeDef dma_tx_handle; 00511 00512 /* Disable NVIC for DMA transfer complete interrupts */ 00513 HAL_NVIC_DisableIRQ(SD_DMAx_Rx_IRQn); 00514 HAL_NVIC_DisableIRQ(SD_DMAx_Tx_IRQn); 00515 00516 /* Deinitialize the stream for new transfer */ 00517 dma_rx_handle.Instance = SD_DMAx_Rx_STREAM; 00518 HAL_DMA_DeInit(&dma_rx_handle); 00519 00520 /* Deinitialize the stream for new transfer */ 00521 dma_tx_handle.Instance = SD_DMAx_Tx_STREAM; 00522 HAL_DMA_DeInit(&dma_tx_handle); 00523 00524 /* Disable NVIC for SDIO interrupts */ 00525 HAL_NVIC_DisableIRQ(SDIO_IRQn); 00526 00527 /* DeInit GPIO pins can be done in the application 00528 (by surcharging this __weak function) */ 00529 00530 /* Disable SDIO clock */ 00531 __HAL_RCC_SDIO_CLK_DISABLE(); 00532 00533 /* GPIO pins clock and DMA clocks can be shut down in the application 00534 by surcharging this __weak function */ 00535 } 00536 00537 /** 00538 * @brief Handles SD card interrupt request. 00539 */ 00540 void BSP_SD_IRQHandler(void) 00541 { 00542 HAL_SD_IRQHandler(&uSdHandle); 00543 } 00544 00545 /** 00546 * @brief Handles SD DMA Tx transfer interrupt request. 00547 */ 00548 void BSP_SD_DMA_Tx_IRQHandler(void) 00549 { 00550 HAL_DMA_IRQHandler(uSdHandle.hdmatx); 00551 } 00552 00553 /** 00554 * @brief Handles SD DMA Rx transfer interrupt request. 00555 */ 00556 void BSP_SD_DMA_Rx_IRQHandler(void) 00557 { 00558 HAL_DMA_IRQHandler(uSdHandle.hdmarx); 00559 } 00560 00561 /** 00562 * @brief Gets the current SD card data status. 00563 * @retval Data transfer state. 00564 * This value can be one of the following values: 00565 * @arg SD_TRANSFER_OK: No data transfer is acting 00566 * @arg SD_TRANSFER_BUSY: Data transfer is acting 00567 * @arg SD_TRANSFER_ERROR: Data transfer error 00568 */ 00569 HAL_SD_TransferStateTypedef BSP_SD_GetStatus(void) 00570 { 00571 return(HAL_SD_GetStatus(&uSdHandle)); 00572 } 00573 00574 /** 00575 * @brief Get SD information about specific SD card. 00576 * @param CardInfo: Pointer to HAL_SD_CardInfoTypedef structure 00577 */ 00578 void BSP_SD_GetCardInfo(HAL_SD_CardInfoTypedef *CardInfo) 00579 { 00580 /* Get SD card Information */ 00581 HAL_SD_Get_CardInfo(&uSdHandle, CardInfo); 00582 } 00583 00584 /** 00585 * @} 00586 */ 00587 00588 /** 00589 * @} 00590 */ 00591 00592 /** 00593 * @} 00594 */ 00595 00596 /** 00597 * @} 00598 */ 00599 00600 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jan 15 2016 10:06:21 for STM32446E_EVAL BSP User Manual by
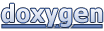