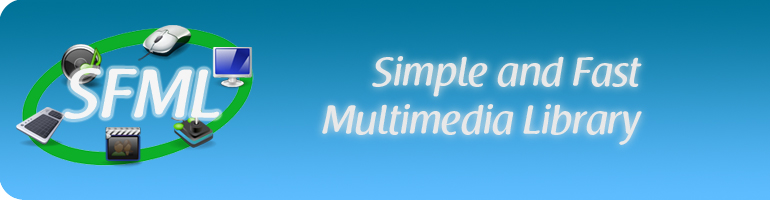
View.cpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00026 // Headers 00028 #include <SFML/Graphics/View.hpp> 00029 #include <algorithm> 00030 00031 00032 namespace sf 00033 { 00037 View::View(const FloatRect& ViewRect) 00038 { 00039 SetFromRect(ViewRect); 00040 } 00041 00042 00046 View::View(const sf::Vector2f& Center, const sf::Vector2f& HalfSize) : 00047 myCenter (Center), 00048 myHalfSize (HalfSize), 00049 myNeedUpdate(true) 00050 { 00051 00052 } 00053 00054 00058 void View::SetCenter(float X, float Y) 00059 { 00060 myCenter.x = X; 00061 myCenter.y = Y; 00062 myNeedUpdate = true; 00063 } 00064 00065 00069 void View::SetCenter(const sf::Vector2f& Center) 00070 { 00071 SetCenter(Center.x, Center.y); 00072 } 00073 00074 00078 void View::SetHalfSize(float HalfWidth, float HalfHeight) 00079 { 00080 myHalfSize.x = HalfWidth; 00081 myHalfSize.y = HalfHeight; 00082 myNeedUpdate = true; 00083 } 00084 00085 00089 void View::SetHalfSize(const sf::Vector2f& HalfSize) 00090 { 00091 SetHalfSize(HalfSize.x, HalfSize.y); 00092 } 00093 00094 00098 void View::SetFromRect(const FloatRect& ViewRect) 00099 { 00100 SetCenter( (ViewRect.Right + ViewRect.Left) / 2, (ViewRect.Bottom + ViewRect.Top) / 2); 00101 SetHalfSize((ViewRect.Right - ViewRect.Left) / 2, (ViewRect.Bottom - ViewRect.Top) / 2); 00102 } 00103 00104 00108 const sf::Vector2f& View::GetCenter() const 00109 { 00110 return myCenter; 00111 } 00112 00113 00117 const sf::Vector2f& View::GetHalfSize() const 00118 { 00119 return myHalfSize; 00120 } 00121 00122 00126 const sf::FloatRect& View::GetRect() const 00127 { 00128 // Recompute it if needed 00129 if (myNeedUpdate) 00130 const_cast<View*>(this)->RecomputeMatrix(); 00131 00132 return myRect; 00133 } 00134 00135 00139 void View::Move(float OffsetX, float OffsetY) 00140 { 00141 myCenter.x += OffsetX; 00142 myCenter.y += OffsetY; 00143 myNeedUpdate = true; 00144 } 00145 00146 00150 void View::Move(const sf::Vector2f& Offset) 00151 { 00152 Move(Offset.x, Offset.y); 00153 } 00154 00155 00159 void View::Zoom(float Factor) 00160 { 00161 if (Factor != 0) 00162 { 00163 myHalfSize /= Factor; 00164 myNeedUpdate = true; 00165 } 00166 } 00167 00168 00172 const Matrix3& View::GetMatrix() const 00173 { 00174 // Recompute the matrix if needed 00175 if (myNeedUpdate) 00176 const_cast<View*>(this)->RecomputeMatrix(); 00177 00178 return myMatrix; 00179 } 00180 00181 00185 void View::RecomputeMatrix() 00186 { 00187 // Compute the 4 corners of the view 00188 float Left = myCenter.x - myHalfSize.x; 00189 float Top = myCenter.y - myHalfSize.y; 00190 float Right = myCenter.x + myHalfSize.x; 00191 float Bottom = myCenter.y + myHalfSize.y; 00192 00193 // Update the view rectangle - be careful, reversed views are allowed ! 00194 myRect.Left = std::min(Left, Right); 00195 myRect.Top = std::min(Top, Bottom); 00196 myRect.Right = std::max(Left, Right); 00197 myRect.Bottom = std::max(Top, Bottom); 00198 00199 // Update the projection matrix 00200 myMatrix(0, 0) = 2.f / (Right - Left); 00201 myMatrix(1, 1) = 2.f / (Top - Bottom); 00202 myMatrix(0, 2) = (Left + Right) / (Left - Right); 00203 myMatrix(1, 2) = (Bottom + Top) / (Bottom - Top); 00204 00205 myNeedUpdate = false; 00206 } 00207 00208 } // namespace sf
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::