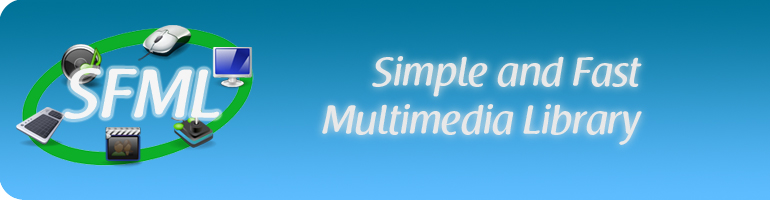
Sprite.cpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00026 // Headers 00028 #include <SFML/Graphics/Sprite.hpp> 00029 #include <SFML/Graphics/Image.hpp> 00030 #include <SFML/Graphics/GraphicsContext.hpp> 00031 00032 00033 namespace sf 00034 { 00038 Sprite::Sprite() : 00039 mySubRect (0, 0, 1, 1), 00040 myIsFlippedX(false), 00041 myIsFlippedY(false) 00042 { 00043 00044 } 00045 00046 00050 Sprite::Sprite(const Image& Img, const Vector2f& Position, const Vector2f& Scale, float Rotation, const Color& Col) : 00051 Drawable (Position, Scale, Rotation, Col), 00052 mySubRect (0, 0, 1, 1), 00053 myIsFlippedX(false), 00054 myIsFlippedY(false) 00055 { 00056 SetImage(Img); 00057 } 00058 00059 00063 void Sprite::SetImage(const Image& Img) 00064 { 00065 // If there was no source image before and the new image is valid, adjust the source rectangle 00066 if (!myImage && (Img.GetWidth() > 0) && (Img.GetHeight() > 0)) 00067 { 00068 SetSubRect(IntRect(0, 0, Img.GetWidth(), Img.GetHeight())); 00069 } 00070 00071 // Assign the new image 00072 myImage = &Img; 00073 } 00074 00075 00079 void Sprite::SetSubRect(const IntRect& SubRect) 00080 { 00081 mySubRect = SubRect; 00082 } 00083 00084 00089 void Sprite::Resize(float Width, float Height) 00090 { 00091 int LocalWidth = mySubRect.GetWidth(); 00092 int LocalHeight = mySubRect.GetHeight(); 00093 00094 if ((LocalWidth > 0) && (LocalHeight > 0)) 00095 SetScale(Width / LocalWidth, Height / LocalHeight); 00096 } 00097 00098 00103 void Sprite::Resize(const Vector2f& Size) 00104 { 00105 Resize(Size.x, Size.y); 00106 } 00107 00108 00112 void Sprite::FlipX(bool Flipped) 00113 { 00114 myIsFlippedX = Flipped; 00115 } 00116 00117 00121 void Sprite::FlipY(bool Flipped) 00122 { 00123 myIsFlippedY = Flipped; 00124 } 00125 00126 00130 const Image* Sprite::GetImage() const 00131 { 00132 return myImage; 00133 } 00134 00135 00139 const IntRect& Sprite::GetSubRect() const 00140 { 00141 return mySubRect; 00142 } 00143 00144 00148 Vector2f Sprite::GetSize() const 00149 { 00150 return Vector2f(mySubRect.GetWidth() * GetScale().x, mySubRect.GetHeight() * GetScale().y); 00151 } 00152 00153 00158 Color Sprite::GetPixel(unsigned int X, unsigned int Y) const 00159 { 00160 if (myImage) 00161 { 00162 unsigned int ImageX = mySubRect.Left + X; 00163 unsigned int ImageY = mySubRect.Top + Y; 00164 00165 if (myIsFlippedX) ImageX = mySubRect.GetWidth() - ImageX - 1; 00166 if (myIsFlippedY) ImageY = mySubRect.GetHeight() - ImageY - 1; 00167 00168 return myImage->GetPixel(ImageX, ImageY) * GetColor(); 00169 } 00170 else 00171 { 00172 return GetColor(); 00173 } 00174 } 00175 00176 00180 void Sprite::Render(RenderTarget&) const 00181 { 00182 // Get the sprite size 00183 float Width = static_cast<float>(mySubRect.GetWidth()); 00184 float Height = static_cast<float>(mySubRect.GetHeight()); 00185 00186 // Check if the image is valid 00187 if (myImage && (myImage->GetWidth() > 0) && (myImage->GetHeight() > 0)) 00188 { 00189 // Use the "offset trick" to get pixel-perfect rendering 00190 // see http://www.opengl.org/resources/faq/technical/transformations.htm#tran0030 00191 GLCheck(glTranslatef(0.375f, 0.375f, 0.f)); 00192 00193 // Bind the texture 00194 myImage->Bind(); 00195 00196 // Calculate the texture coordinates 00197 FloatRect TexCoords = myImage->GetTexCoords(mySubRect); 00198 FloatRect Rect(myIsFlippedX ? TexCoords.Right : TexCoords.Left, 00199 myIsFlippedY ? TexCoords.Bottom : TexCoords.Top, 00200 myIsFlippedX ? TexCoords.Left : TexCoords.Right, 00201 myIsFlippedY ? TexCoords.Top : TexCoords.Bottom); 00202 00203 // Draw the sprite's triangles 00204 glBegin(GL_QUADS); 00205 glTexCoord2f(Rect.Left, Rect.Top); glVertex2f(0, 0); 00206 glTexCoord2f(Rect.Left, Rect.Bottom); glVertex2f(0, Height); 00207 glTexCoord2f(Rect.Right, Rect.Bottom); glVertex2f(Width, Height); 00208 glTexCoord2f(Rect.Right, Rect.Top); glVertex2f(Width, 0) ; 00209 glEnd(); 00210 } 00211 else 00212 { 00213 // Disable texturing 00214 GLCheck(glDisable(GL_TEXTURE_2D)); 00215 00216 // Draw the sprite's triangles 00217 glBegin(GL_QUADS); 00218 glVertex2f(0, 0); 00219 glVertex2f(0, Height); 00220 glVertex2f(Width, Height); 00221 glVertex2f(Width, 0); 00222 glEnd(); 00223 } 00224 } 00225 00226 } // namespace sf
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::