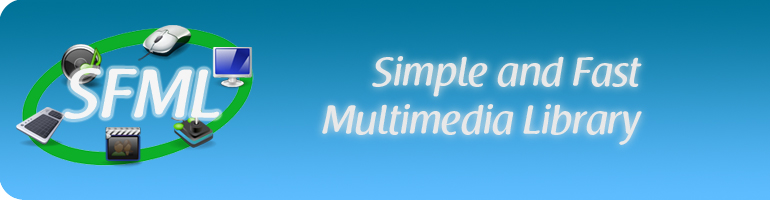
SoundFile.hpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00025 #ifndef SFML_SOUNDFILE_HPP 00026 #define SFML_SOUNDFILE_HPP 00027 00029 // Headers 00031 #include <SFML/System/NonCopyable.hpp> 00032 #include <string> 00033 00034 00035 namespace sf 00036 { 00037 namespace priv 00038 { 00043 class SoundFile : NonCopyable 00044 { 00045 public : 00046 00058 static SoundFile* CreateRead(const std::string& Filename); 00059 00072 static SoundFile* CreateRead(const char* Data, std::size_t SizeInBytes); 00073 00084 static SoundFile* CreateWrite(const std::string& Filename, unsigned int ChannelsCount, unsigned int SampleRate); 00085 00090 virtual ~SoundFile(); 00091 00098 std::size_t GetSamplesCount() const; 00099 00106 unsigned int GetChannelsCount() const; 00107 00114 unsigned int GetSampleRate() const; 00115 00122 bool Restart(); 00123 00133 virtual std::size_t Read(Int16* Data, std::size_t NbSamples); 00134 00142 virtual void Write(const Int16* Data, std::size_t NbSamples); 00143 00144 protected : 00145 00150 SoundFile(); 00151 00152 private : 00153 00165 virtual bool OpenRead(const std::string& Filename, std::size_t& NbSamples, unsigned int& ChannelsCount, unsigned int& SampleRate); 00166 00179 virtual bool OpenRead(const char* Data, std::size_t SizeInBytes, std::size_t& NbSamples, unsigned int& ChannelsCount, unsigned int& SampleRate); 00180 00191 virtual bool OpenWrite(const std::string& Filename, unsigned int ChannelsCount, unsigned int SampleRate); 00192 00194 // Member data 00196 std::size_t myNbSamples; 00197 unsigned int myChannelsCount; 00198 unsigned int mySampleRate; 00199 std::string myFilename; 00200 const char* myData; 00201 std::size_t mySize; 00202 }; 00203 00204 } // namespace priv 00205 00206 } // namespace sf 00207 00208 00209 #endif // SFML_SOUNDFILE_HPP
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::