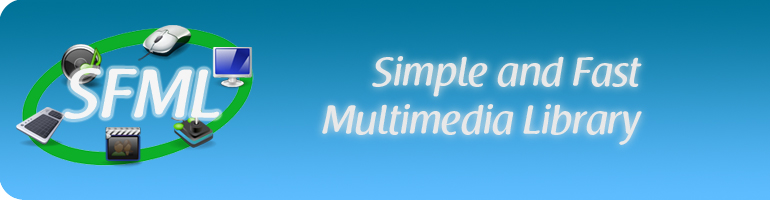
SoundFile.cpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00026 // Headers 00028 #include <SFML/Audio/SoundFile.hpp> 00029 #include <SFML/Audio/SoundFileDefault.hpp> 00030 #include <SFML/Audio/SoundFileOgg.hpp> 00031 #include <iostream> 00032 00033 00034 namespace sf 00035 { 00036 namespace priv 00037 { 00041 SoundFile* SoundFile::CreateRead(const std::string& Filename) 00042 { 00043 // Create the file according to its type 00044 SoundFile* File = NULL; 00045 if (SoundFileOgg::IsFileSupported(Filename, true)) File = new SoundFileOgg; 00046 else if (SoundFileDefault::IsFileSupported(Filename, true)) File = new SoundFileDefault; 00047 00048 // Open it for reading 00049 if (File) 00050 { 00051 std::size_t SamplesCount; 00052 unsigned int ChannelsCount; 00053 unsigned int SampleRate; 00054 00055 if (File->OpenRead(Filename, SamplesCount, ChannelsCount, SampleRate)) 00056 { 00057 File->myFilename = Filename; 00058 File->myData = NULL; 00059 File->mySize = 0; 00060 File->myNbSamples = SamplesCount; 00061 File->myChannelsCount = ChannelsCount; 00062 File->mySampleRate = SampleRate; 00063 } 00064 else 00065 { 00066 delete File; 00067 File = NULL; 00068 } 00069 } 00070 00071 return File; 00072 } 00073 00074 00078 SoundFile* SoundFile::CreateRead(const char* Data, std::size_t SizeInMemory) 00079 { 00080 // Create the file according to its type 00081 SoundFile* File = NULL; 00082 if (SoundFileOgg::IsFileSupported(Data, SizeInMemory)) File = new SoundFileOgg; 00083 else if (SoundFileDefault::IsFileSupported(Data, SizeInMemory)) File = new SoundFileDefault; 00084 00085 // Open it for reading 00086 if (File) 00087 { 00088 std::size_t SamplesCount; 00089 unsigned int ChannelsCount; 00090 unsigned int SampleRate; 00091 00092 if (File->OpenRead(Data, SizeInMemory, SamplesCount, ChannelsCount, SampleRate)) 00093 { 00094 File->myFilename = ""; 00095 File->myData = Data; 00096 File->mySize = SizeInMemory; 00097 File->myNbSamples = SamplesCount; 00098 File->myChannelsCount = ChannelsCount; 00099 File->mySampleRate = SampleRate; 00100 } 00101 else 00102 { 00103 delete File; 00104 File = NULL; 00105 } 00106 } 00107 00108 return File; 00109 } 00110 00111 00115 SoundFile* SoundFile::CreateWrite(const std::string& Filename, unsigned int ChannelsCount, unsigned int SampleRate) 00116 { 00117 // Create the file according to its type 00118 SoundFile* File = NULL; 00119 if (SoundFileOgg::IsFileSupported(Filename, false)) File = new SoundFileOgg; 00120 else if (SoundFileDefault::IsFileSupported(Filename, false)) File = new SoundFileDefault; 00121 00122 // Open it for writing 00123 if (File) 00124 { 00125 if (File->OpenWrite(Filename, ChannelsCount, SampleRate)) 00126 { 00127 File->myFilename = ""; 00128 File->myData = NULL; 00129 File->mySize = 0; 00130 File->myNbSamples = 0; 00131 File->myChannelsCount = ChannelsCount; 00132 File->mySampleRate = SampleRate; 00133 } 00134 else 00135 { 00136 delete File; 00137 File = NULL; 00138 } 00139 } 00140 00141 return File; 00142 } 00143 00144 00148 SoundFile::SoundFile() : 00149 myNbSamples (0), 00150 myChannelsCount(0), 00151 mySampleRate (0) 00152 { 00153 00154 } 00155 00156 00160 SoundFile::~SoundFile() 00161 { 00162 // Nothing to do 00163 } 00164 00165 00169 std::size_t SoundFile::GetSamplesCount() const 00170 { 00171 return myNbSamples; 00172 } 00173 00174 00178 unsigned int SoundFile::GetChannelsCount() const 00179 { 00180 return myChannelsCount; 00181 } 00182 00183 00187 unsigned int SoundFile::GetSampleRate() const 00188 { 00189 return mySampleRate; 00190 } 00191 00192 00196 bool SoundFile::Restart() 00197 { 00198 if (myData) 00199 { 00200 // Reopen from memory 00201 return OpenRead(myData, mySize, myNbSamples, myChannelsCount, mySampleRate); 00202 } 00203 else if (myFilename != "") 00204 { 00205 // Reopen from file 00206 return OpenRead(myFilename, myNbSamples, myChannelsCount, mySampleRate); 00207 } 00208 else 00209 { 00210 // Trying to reopen a file opened in write mode... error 00211 std::cerr << "Warning : trying to restart a sound opened in write mode, which is not allowed" << std::endl; 00212 return false; 00213 } 00214 } 00215 00216 00220 bool SoundFile::OpenRead(const std::string& Filename, std::size_t&, unsigned int&, unsigned int&) 00221 { 00222 std::cerr << "Failed to open sound file \"" << Filename << "\", format is not supported by SFML" << std::endl; 00223 00224 return false; 00225 } 00226 00227 00231 bool SoundFile::OpenRead(const char*, std::size_t, std::size_t&, unsigned int&, unsigned int&) 00232 { 00233 std::cerr << "Failed to open sound file from memory, format is not supported by SFML" << std::endl; 00234 00235 return false; 00236 } 00237 00238 00242 bool SoundFile::OpenWrite(const std::string& Filename, unsigned int, unsigned int) 00243 { 00244 std::cerr << "Failed to open sound file \"" << Filename << "\", format is not supported by SFML" << std::endl; 00245 00246 return false; 00247 } 00248 00249 00253 std::size_t SoundFile::Read(Int16*, std::size_t) 00254 { 00255 std::cerr << "Failed to read from sound file (not supported)" << std::endl; 00256 00257 return 0; 00258 } 00259 00260 00264 void SoundFile::Write(const Int16*, std::size_t) 00265 { 00266 std::cerr << "Failed to write to sound file (not supported)" << std::endl; 00267 } 00268 00269 } // namespace priv 00270 00271 } // namespace sf
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::