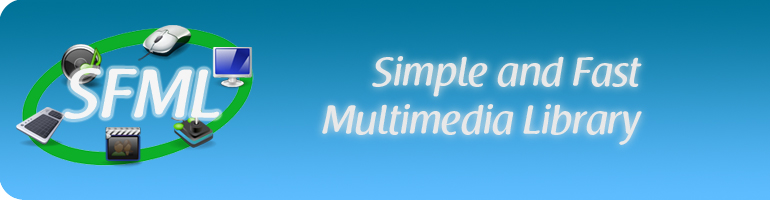
Ftp.hpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00025 #ifndef SFML_FTP_HPP 00026 #define SFML_FTP_HPP 00027 00029 // Headers 00031 #include <SFML/System/NonCopyable.hpp> 00032 #include <SFML/Network/SocketTCP.hpp> 00033 #include <string> 00034 #include <vector> 00035 00036 00037 namespace sf 00038 { 00039 class IPAddress; 00040 00047 class SFML_API Ftp : NonCopyable 00048 { 00049 public : 00050 00054 enum TransferMode 00055 { 00056 Binary, 00057 Ascii, 00058 Ebcdic 00059 }; 00060 00066 class SFML_API Response 00067 { 00068 public : 00069 00074 enum Status 00075 { 00076 // 1xx: the requested action is being initiated, 00077 // expect another reply before proceeding with a new command 00078 RestartMarkerReply = 110, 00079 ServiceReadySoon = 120, 00080 DataConnectionAlreadyOpened = 125, 00081 OpeningDataConnection = 150, 00082 00083 // 2xx: the requested action has been successfully completed 00084 Ok = 200, 00085 PointlessCommand = 202, 00086 SystemStatus = 211, 00087 DirectoryStatus = 212, 00088 FileStatus = 213, 00089 HelpMessage = 214, 00090 SystemType = 215, 00091 ServiceReady = 220, 00092 ClosingConnection = 221, 00093 DataConnectionOpened = 225, 00094 ClosingDataConnection = 226, 00095 EnteringPassiveMode = 227, 00096 LoggedIn = 230, 00097 FileActionOk = 250, 00098 DirectoryOk = 257, 00099 00100 // 3xx: the command has been accepted, but the requested action 00101 // is dormant, pending receipt of further information 00102 NeedPassword = 331, 00103 NeedAccountToLogIn = 332, 00104 NeedInformation = 350, 00105 00106 // 4xx: the command was not accepted and the requested action did not take place, 00107 // but the error condition is temporary and the action may be requested again 00108 ServiceUnavailable = 421, 00109 DataConnectionUnavailable = 425, 00110 TransferAborted = 426, 00111 FileActionAborted = 450, 00112 LocalError = 451, 00113 InsufficientStorageSpace = 452, 00114 00115 // 5xx: the command was not accepted and 00116 // the requested action did not take place 00117 CommandUnknown = 500, 00118 ParametersUnknown = 501, 00119 CommandNotImplemented = 502, 00120 BadCommandSequence = 503, 00121 ParameterNotImplemented = 504, 00122 NotLoggedIn = 530, 00123 NeedAccountToStore = 532, 00124 FileUnavailable = 550, 00125 PageTypeUnknown = 551, 00126 NotEnoughMemory = 552, 00127 FilenameNotAllowed = 553, 00128 00129 // 10xx: SFML custom codes 00130 InvalidResponse = 1000, 00131 ConnectionFailed = 1001, 00132 ConnectionClosed = 1002, 00133 InvalidFile = 1003 00134 }; 00135 00143 Response(Status Code = InvalidResponse, const std::string& Message = ""); 00144 00152 bool IsOk() const; 00153 00160 Status GetStatus() const; 00161 00168 const std::string& GetMessage() const; 00169 00170 private : 00171 00173 // Member data 00175 Status myStatus; 00176 std::string myMessage; 00177 }; 00178 00182 class SFML_API DirectoryResponse : public Response 00183 { 00184 public : 00185 00192 DirectoryResponse(Response Resp); 00193 00200 const std::string& GetDirectory() const; 00201 00202 private : 00203 00205 // Member data 00207 std::string myDirectory; 00208 }; 00209 00210 00214 class SFML_API ListingResponse : public Response 00215 { 00216 public : 00217 00225 ListingResponse(Response Resp, const std::vector<char>& Data); 00226 00233 std::size_t GetCount() const; 00234 00243 const std::string& GetFilename(std::size_t Index) const; 00244 00245 private : 00246 00248 // Member data 00250 std::vector<std::string> myFilenames; 00251 }; 00252 00253 00258 ~Ftp(); 00259 00270 Response Connect(const IPAddress& Server, unsigned short Port = 21, float Timeout = 0.f); 00271 00278 Response Login(); 00279 00289 Response Login(const std::string& UserName, const std::string& Password); 00290 00297 Response Disconnect(); 00298 00305 Response KeepAlive(); 00306 00313 DirectoryResponse GetWorkingDirectory(); 00314 00324 ListingResponse GetDirectoryListing(const std::string& Directory = ""); 00325 00334 Response ChangeDirectory(const std::string& Directory); 00335 00342 Response ParentDirectory(); 00343 00352 Response MakeDirectory(const std::string& Name); 00353 00362 Response DeleteDirectory(const std::string& Name); 00363 00373 Response RenameFile(const std::string& File, const std::string& NewName); 00374 00383 Response DeleteFile(const std::string& Name); 00384 00395 Response Download(const std::string& DistantFile, const std::string& DestPath, TransferMode Mode = Binary); 00396 00407 Response Upload(const std::string& LocalFile, const std::string& DestPath, TransferMode Mode = Binary); 00408 00409 private : 00410 00420 Response SendCommand(const std::string& Command, const std::string& Parameter = ""); 00421 00429 Response GetResponse(); 00430 00435 class DataChannel; 00436 00437 friend class DataChannel; 00438 00440 // Member data 00442 SocketTCP myCommandSocket; 00443 }; 00444 00445 } // namespace sf 00446 00447 00448 #endif // SFML_FTP_HPP
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::