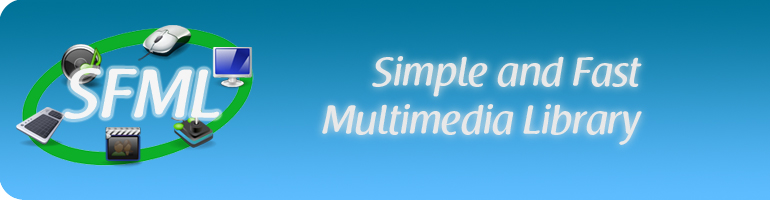
Font.cpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00026 // Headers 00028 #include <SFML/Graphics/Font.hpp> 00029 #include <SFML/Graphics/FontLoader.hpp> 00030 #include <iostream> 00031 00032 00033 namespace sf 00034 { 00036 // Static member data 00038 Uint32 Font::ourDefaultCharset[] = 00039 { 00040 // Printable characters in ASCII range 00041 0x20, 0x21, 0x22, 0x23, 0x24, 0x25, 0x26, 0x27, 0x28, 0x29, 0x2A, 0x2B, 0x2C, 0x2D, 0x2E, 0x2F, 00042 0x30, 0x31, 0x32, 0x33, 0x34, 0x35, 0x36, 0x37, 0x38, 0x39, 0x3A, 0x3B, 0x3C, 0x3D, 0x3E, 0x3F, 00043 0x40, 0x41, 0x42, 0x43, 0x44, 0x45, 0x46, 0x47, 0x48, 0x49, 0x4A, 0x4B, 0x4C, 0x4D, 0x4E, 0x4F, 00044 0x50, 0x51, 0x52, 0x53, 0x54, 0x55, 0x56, 0x57, 0x58, 0x59, 0x5A, 0x5B, 0x5C, 0x5D, 0x5E, 0x5F, 00045 0x60, 0x61, 0x62, 0x63, 0x64, 0x65, 0x66, 0x67, 0x68, 0x69, 0x6A, 0x6B, 0x6C, 0x6D, 0x6E, 0x6F, 00046 0x70, 0x71, 0x72, 0x73, 0x74, 0x75, 0x76, 0x77, 0x78, 0x79, 0x7A, 0x7B, 0x7C, 0x7D, 0x7E, 00047 00048 // Printable characters in extended ASCII range 00049 0xA0, 0xA1, 0xA2, 0xA3, 0xA4, 0x2A, 0xA6, 0xA7, 0xA8, 0xA9, 0xAA, 0xAB, 0xAC, 0xAD, 0xAE, 0xAF, 00050 0xB0, 0xB1, 0xB2, 0xB3, 0xB4, 0xB5, 0xB6, 0xB7, 0xB8, 0xB9, 0xBA, 0xBB, 0xBC, 0xBD, 0xBE, 0xBF, 00051 0xC0, 0xC1, 0xC2, 0xC3, 0xC4, 0xC5, 0xC6, 0xC7, 0xC8, 0xC9, 0xCA, 0xCB, 0xCC, 0xCD, 0xCE, 0xCF, 00052 0xD0, 0xD1, 0xD2, 0xD3, 0xD4, 0xD5, 0xD6, 0xD7, 0xD8, 0xD9, 0xDA, 0xDB, 0xDC, 0xDD, 0xDE, 0xDF, 00053 0xE0, 0xE1, 0xE2, 0xE3, 0xE4, 0xE5, 0xE6, 0xE7, 0xE8, 0xE9, 0xEA, 0xEB, 0xEC, 0xED, 0xEE, 0xEF, 00054 0xF0, 0xF1, 0xF2, 0xF3, 0xF4, 0xF5, 0xF6, 0xF7, 0xF8, 0xF9, 0xFA, 0xFB, 0xFC, 0xFD, 0xFE, 00055 00056 // To make it a valid string 00057 0x00 00058 }; 00059 00060 00064 Font::Font() : 00065 myCharSize(0) 00066 { 00067 00068 } 00069 00070 00074 bool Font::LoadFromFile(const std::string& Filename, unsigned int CharSize, const Unicode::Text& Charset) 00075 { 00076 // Clear the previous character map 00077 myGlyphs.clear(); 00078 00079 // Always add these special characters 00080 Unicode::UTF32String UTFCharset = Charset; 00081 if (UTFCharset.find(L' ') != Unicode::UTF32String::npos) UTFCharset += L' '; 00082 if (UTFCharset.find(L'\n') != Unicode::UTF32String::npos) UTFCharset += L'\n'; 00083 if (UTFCharset.find(L'\v') != Unicode::UTF32String::npos) UTFCharset += L'\v'; 00084 if (UTFCharset.find(L'\t') != Unicode::UTF32String::npos) UTFCharset += L'\t'; 00085 00086 return priv::FontLoader::GetInstance().LoadFontFromFile(Filename, CharSize, UTFCharset, *this); 00087 } 00088 00089 00093 bool Font::LoadFromMemory(const char* Data, std::size_t SizeInBytes, unsigned int CharSize, const Unicode::Text& Charset) 00094 { 00095 // Clear the previous character map 00096 myGlyphs.clear(); 00097 00098 // Check parameters 00099 if (!Data || (SizeInBytes == 0)) 00100 { 00101 std::cerr << "Failed to load font from memory, no data provided" << std::endl; 00102 return false; 00103 } 00104 00105 // Always add these special characters 00106 Unicode::UTF32String UTFCharset = Charset; 00107 if (UTFCharset.find(L' ') != Unicode::UTF32String::npos) UTFCharset += L' '; 00108 if (UTFCharset.find(L'\n') != Unicode::UTF32String::npos) UTFCharset += L'\n'; 00109 if (UTFCharset.find(L'\v') != Unicode::UTF32String::npos) UTFCharset += L'\v'; 00110 if (UTFCharset.find(L'\t') != Unicode::UTF32String::npos) UTFCharset += L'\t'; 00111 00112 return priv::FontLoader::GetInstance().LoadFontFromMemory(Data, SizeInBytes, CharSize, UTFCharset, *this); 00113 } 00114 00115 00120 unsigned int Font::GetCharacterSize() const 00121 { 00122 return myCharSize; 00123 } 00124 00125 00130 const Glyph& Font::GetGlyph(Uint32 CodePoint) const 00131 { 00132 std::map<Uint32, Glyph>::const_iterator It = myGlyphs.find(CodePoint); 00133 if (It != myGlyphs.end()) 00134 { 00135 // Valid glyph 00136 return It->second; 00137 } 00138 else 00139 { 00140 // Invalid glyph -- return an invalid glyph 00141 static const Glyph InvalidGlyph; 00142 return InvalidGlyph; 00143 } 00144 } 00145 00146 00150 const Image& Font::GetImage() const 00151 { 00152 return myTexture; 00153 } 00154 00155 00159 const Font& Font::GetDefaultFont() 00160 { 00161 #if defined(SFML_SYSTEM_WINDOWS) && defined(SFML_DYNAMIC) 00162 00163 // On Windows dynamic build, the default font causes a crash at global exit. 00164 // This is a temporary workaround that turns the crash into a memory leak. 00165 // Note that this bug doesn't exist anymore in SFML 2. 00166 static Font* DefaultFontPtr = new Font; 00167 Font& DefaultFont = *DefaultFontPtr; 00168 00169 #else 00170 00171 static Font DefaultFont; 00172 00173 #endif 00174 00175 // Get the raw data of the Arial font file into an array, so that we can load it into the font 00176 static const char DefaultFontData[] = 00177 { 00178 #include <SFML/Graphics/Arial.hpp> 00179 }; 00180 00181 // Load the default font on first call 00182 static bool DefaultFontLoaded = false; 00183 if (!DefaultFontLoaded) 00184 { 00185 DefaultFont.LoadFromMemory(DefaultFontData, sizeof(DefaultFontData), 30); 00186 DefaultFontLoaded = true; 00187 } 00188 00189 return DefaultFont; 00190 } 00191 00192 } // namespace sf
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::