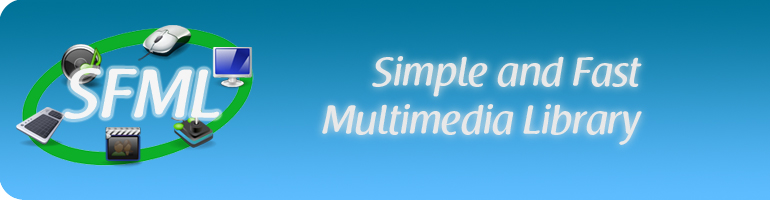
Event.hpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00025 #ifndef SFML_EVENT_HPP 00026 #define SFML_EVENT_HPP 00027 00029 // Headers 00031 #include <SFML/Config.hpp> 00032 00033 00034 namespace sf 00035 { 00039 namespace Key 00040 { 00041 enum Code 00042 { 00043 A = 'a', 00044 B = 'b', 00045 C = 'c', 00046 D = 'd', 00047 E = 'e', 00048 F = 'f', 00049 G = 'g', 00050 H = 'h', 00051 I = 'i', 00052 J = 'j', 00053 K = 'k', 00054 L = 'l', 00055 M = 'm', 00056 N = 'n', 00057 O = 'o', 00058 P = 'p', 00059 Q = 'q', 00060 R = 'r', 00061 S = 's', 00062 T = 't', 00063 U = 'u', 00064 V = 'v', 00065 W = 'w', 00066 X = 'x', 00067 Y = 'y', 00068 Z = 'z', 00069 Num0 = '0', 00070 Num1 = '1', 00071 Num2 = '2', 00072 Num3 = '3', 00073 Num4 = '4', 00074 Num5 = '5', 00075 Num6 = '6', 00076 Num7 = '7', 00077 Num8 = '8', 00078 Num9 = '9', 00079 Escape = 256, 00080 LControl, 00081 LShift, 00082 LAlt, 00083 LSystem, 00084 RControl, 00085 RShift, 00086 RAlt, 00087 RSystem, 00088 Menu, 00089 LBracket, 00090 RBracket, 00091 SemiColon, 00092 Comma, 00093 Period, 00094 Quote, 00095 Slash, 00096 BackSlash, 00097 Tilde, 00098 Equal, 00099 Dash, 00100 Space, 00101 Return, 00102 Back, 00103 Tab, 00104 PageUp, 00105 PageDown, 00106 End, 00107 Home, 00108 Insert, 00109 Delete, 00110 Add, 00111 Subtract, 00112 Multiply, 00113 Divide, 00114 Left, 00115 Right, 00116 Up, 00117 Down, 00118 Numpad0, 00119 Numpad1, 00120 Numpad2, 00121 Numpad3, 00122 Numpad4, 00123 Numpad5, 00124 Numpad6, 00125 Numpad7, 00126 Numpad8, 00127 Numpad9, 00128 F1, 00129 F2, 00130 F3, 00131 F4, 00132 F5, 00133 F6, 00134 F7, 00135 F8, 00136 F9, 00137 F10, 00138 F11, 00139 F12, 00140 F13, 00141 F14, 00142 F15, 00143 Pause, 00144 00145 Count // Keep last -- total number of keyboard keys 00146 }; 00147 } 00148 00149 00153 namespace Mouse 00154 { 00155 enum Button 00156 { 00157 Left, 00158 Right, 00159 Middle, 00160 XButton1, 00161 XButton2, 00162 00163 ButtonCount // Keep last -- total number of mouse buttons 00164 }; 00165 } 00166 00167 00171 namespace Joy 00172 { 00173 enum Axis 00174 { 00175 AxisX, 00176 AxisY, 00177 AxisZ, 00178 AxisR, 00179 AxisU, 00180 AxisV, 00181 AxisPOV, 00182 00183 AxisCount // Keep last -- total number of joystick axis 00184 }; 00185 00186 enum 00187 { 00188 Count = 4, 00189 ButtonCount = 32 00190 }; 00191 } 00192 00193 00197 class Event 00198 { 00199 public : 00200 00204 struct KeyEvent 00205 { 00206 Key::Code Code; 00207 bool Alt; 00208 bool Control; 00209 bool Shift; 00210 }; 00211 00215 struct TextEvent 00216 { 00217 Uint32 Unicode; 00218 }; 00219 00223 struct MouseMoveEvent 00224 { 00225 int X; 00226 int Y; 00227 }; 00228 00232 struct MouseButtonEvent 00233 { 00234 Mouse::Button Button; 00235 int X; 00236 int Y; 00237 }; 00238 00242 struct MouseWheelEvent 00243 { 00244 int Delta; 00245 }; 00246 00250 struct JoyMoveEvent 00251 { 00252 unsigned int JoystickId; 00253 Joy::Axis Axis; 00254 float Position; 00255 }; 00256 00260 struct JoyButtonEvent 00261 { 00262 unsigned int JoystickId; 00263 unsigned int Button; 00264 }; 00265 00269 struct SizeEvent 00270 { 00271 unsigned int Width; 00272 unsigned int Height; 00273 }; 00274 00278 enum EventType 00279 { 00280 Closed, 00281 Resized, 00282 LostFocus, 00283 GainedFocus, 00284 TextEntered, 00285 KeyPressed, 00286 KeyReleased, 00287 MouseWheelMoved, 00288 MouseButtonPressed, 00289 MouseButtonReleased, 00290 MouseMoved, 00291 MouseEntered, 00292 MouseLeft, 00293 JoyButtonPressed, 00294 JoyButtonReleased, 00295 JoyMoved, 00296 00297 Count // Keep last -- total number of event types 00298 }; 00299 00301 // Member data 00303 EventType Type; 00304 00305 union 00306 { 00307 KeyEvent Key; 00308 TextEvent Text; 00309 MouseMoveEvent MouseMove; 00310 MouseButtonEvent MouseButton; 00311 MouseWheelEvent MouseWheel; 00312 JoyMoveEvent JoyMove; 00313 JoyButtonEvent JoyButton; 00314 SizeEvent Size; 00315 }; 00316 }; 00317 00318 } // namespace sf 00319 00320 00321 #endif // SFML_EVENT_HPP
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::